This content originally appeared on DEV Community and was authored by CodeCadim by Brahim Hamdouni
Svelte's component system features a specific approach to reactivity and component initialization. This article explores a common misconception about how props, variables, and component initialization work in Svelte.
The Misconception
Developers new to Svelte might assume that when a prop changes, the entire component script runs again, like if the component was a function and the prop a parameter: if the prop changes, the function
will give another result, right?
This isn't how Svelte operates, and understanding this distinction is important for building functional applications.
Analyzing the Example
The example demonstrates this concept with two components:
- app.svelte: A parent component with buttons to select different names
- name.svelte: A child component that receives a name prop and tracks modifications
The Parent Component (app.svelte)
<script>
// This shows that changing the prop does not rerun the component
// script.
import InputName from "./name.svelte";
let names = ["John", "Mila", "Ali"];
let selected = $state();
</script>
<!-- This selector trigger the prop change in child component -->
{#each names as name}
<button onclick={() => selected=name}>{name}</button>
{/each}
<!-- This section call the child component with the prop -->
{#if selected}
<InputName name={selected}/>
{/if}
The parent component:
- Maintains a list of names and a selected name state
- Renders buttons for each name that update the
selected
variable - Also renders the
InputName
component with the selected name
The Child Component (name.svelte)
<script>
// This is a simple component that implements change detection
let { name } = $props();
// this is executed only once at component mount
let original = name;
let changed = $derived(original != name);
</script>
Name : <input type="text" bind:value={name}>
{#if changed}
modified
{/if}
The child component:
- Receives a
name
prop using$props()
- Stores the initial value in an
original
variable - Uses
$derived
to create a reactivechanged
variable that compares current and original values - Displays "modified" when the input value differs from the original
The Problem
When we click on a name and start updating the field, it shows the 'modified' indicator. This appears to work correctly.
However, when we click on another name, the indicator remains visible. We can also trigger this issue by clicking on the first name without modifying it, then clicking a second name: the 'modified' indicator appears despite not updating anything.
The key insight is that the component's script block runs only once when the component is initially mounted. When props change, Svelte updates the reactive dependencies without re-running the entire script.
This means that let original = name;
is executed only once during component initialization. When the name
prop changes later:
- The
name
value updates - The
changed
derived value recalculates - But the variable
original
still holds the first value it received
The Solution
To force the child component to re-execute its script when the name
prop changes, we use a {#key}
block in the parent component.
The {#key var}
block triggers at var
updates by destroying and recreating the components it surrounds.
For our example, we need to modify our code like this:
<!-- This section call the child component with the prop -->
{#if selected}
{#key selected}
<InputName name={selected}/>
{/key}
{/if}
When selected
changes:
- Without
{#key}
, the same component instance would remain and the variableoriginal
would never update - With
{#key}
, Svelte destroys and recreates theInputName
component, causing the script to run again and the variableoriginal
to be reset
Conclusion
- Be aware of one-time initialization: Variables assigned directly in the script block are initialized only once.
-
Use reactive declarations: Leverage
$derived
for values that need to react to prop changes. -
Understand when to use
{#key}
: Use it when you need to completely reset a component state, like in our example for change detection patterns.
This content originally appeared on DEV Community and was authored by CodeCadim by Brahim Hamdouni
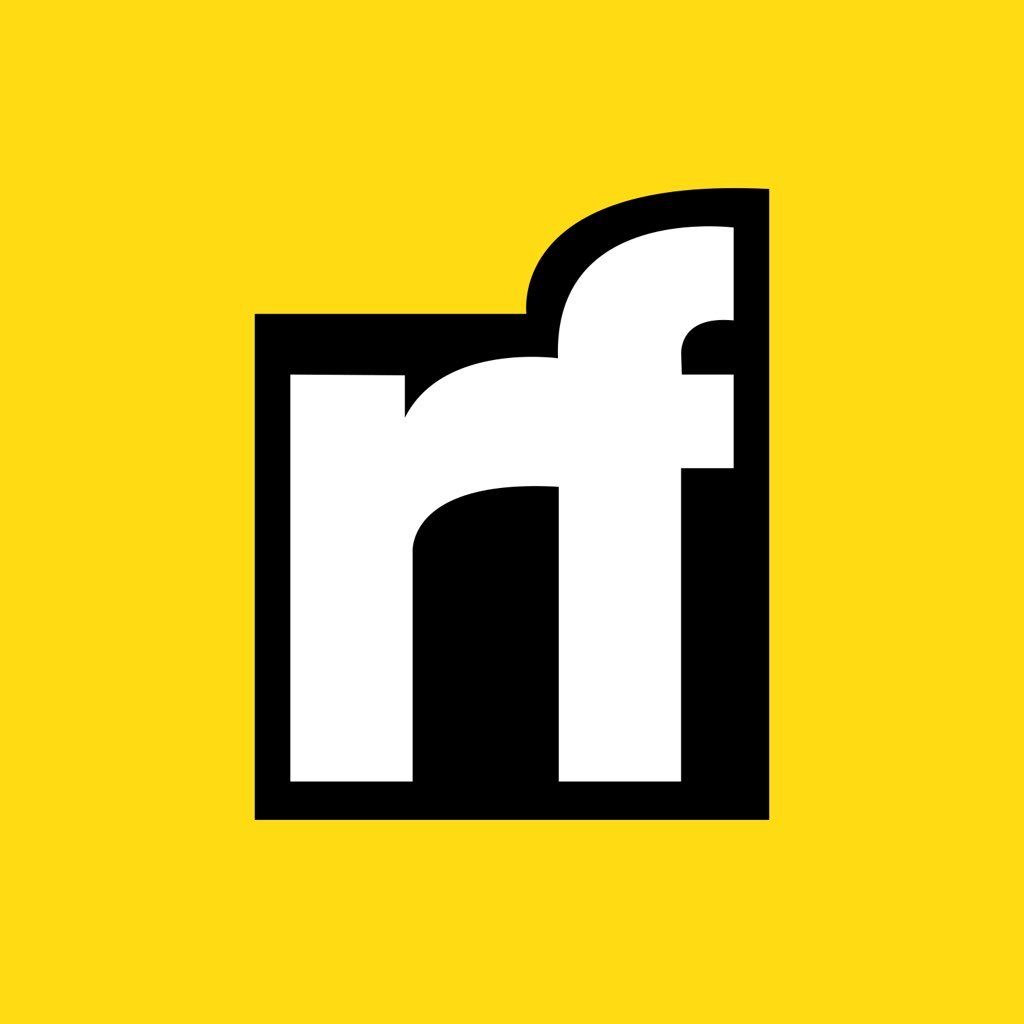
CodeCadim by Brahim Hamdouni | Sciencx (2025-03-01T21:50:19+00:00) Understanding Svelte Component Lifecycle and Reactivity. Retrieved from https://www.scien.cx/2025/03/01/understanding-svelte-component-lifecycle-and-reactivity/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.