This content originally appeared on DEV Community and was authored by Saoud
Database Schema and Relationship Types
Terminology
- Database schema: The structure that represents the way the database is built. It defines how data is stored in the database tables and how the relations among tables are associated.
- One-to-one Relationship: Two tables have a one-to-one relationship when a row on one table is related to only one row on the other table.
- One-to-many relationship: Two tables share a one-to-many relationship when a single row on one table can be related to many rows on another table.
- Many-to-many relationship: Two tables share a many-to-many relationship when each row on each table can have many rows on the other table. This relationship requires use of a join table.
- Join table: Used to manage all possible relationships in a many-to-many relationship, a join table contains individual entries.
Configuration for Entity Framework Core
ToDoList.csproj
<Project Sdk="Microsoft.NET.Sdk.Web">
<PropertyGroup>
<TargetFramework>net5.0</TargetFramework>
</PropertyGroup>
<ItemGroup>
<PackageReference Include="Microsoft.EntityFrameworkCore" Version="5.0.0" />
<PackageReference Include="Pomelo.EntityFrameworkCore.MySql" Version="5.0.0-alpha.2" />
</ItemGroup>
</Project>
appsettings.json
{
"ConnectionStrings": {
"DefaultConnection": "Server=localhost;Port=3306;database=to_do_list;uid=root;pwd=[YOUR-PASSWORD-HERE];"
}
}
Make sure your appsettings.json
file is in your .gitignore
!
Startup.cs
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Http;
using Microsoft.EntityFrameworkCore;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.DependencyInjection;
using ToDoList.Models;
namespace ToDoList
{
public class Startup
{
public Startup(IWebHostEnvironment env)
{
var builder = new ConfigurationBuilder()
.SetBasePath(env.ContentRootPath)
.AddJsonFile("appsettings.json");
Configuration = builder.Build();
}
public IConfigurationRoot Configuration { get; set; }
public void ConfigureServices(IServiceCollection services)
{
services.AddMvc();
services.AddEntityFrameworkMySql()
.AddDbContext<ToDoListContext>(options => options
.UseMySql(Configuration["ConnectionStrings:DefaultConnection"], ServerVersion.AutoDetect(Configuration["ConnectionStrings:DefaultConnection"])));
}
public void Configure(IApplicationBuilder app)
{
app.UseDeveloperExceptionPage();
app.UseRouting();
app.UseEndpoints(routes =>
{
routes.MapControllerRoute("default", "{controller=Home}/{action=Index}/{id?}");
});
app.UseStaticFiles();
app.Run(async (context) =>
{
await context.Response.WriteAsync("Hello World!");
});
}
}
}
Program.cs
using System.IO;
using Microsoft.AspNetCore.Hosting;
namespace ToDoList
{
public class Program
{
public static void Main(string[] args)
{
var host = new WebHostBuilder()
.UseKestrel()
.UseContentRoot(Directory.GetCurrentDirectory())
.UseIISIntegration()
.UseStartup<Startup>()
.Build();
host.Run();
}
}
}
Models/ToDoListContext.cs
using Microsoft.EntityFrameworkCore;
namespace ToDoList.Models
{
public class ToDoListContext : DbContext
{
public DbSet<Item> Items { get; set; }
public ToDoListContext(DbContextOptions options) : base(options) { }
}
}
EF Core with an Existing Database
Models/Item.cs
namespace ToDoList.Models
{
public class Item
{
public int ItemId { get; set; }
public string Description { get; set; }
}
}
Controllers/ItemsController.cs
using Microsoft.AspNetCore.Mvc;
using ToDoList.Models;
using System.Collections.Generic;
using System.Linq;
namespace ToDoList.Controllers
{
public class ItemsController : Controller
{
private readonly ToDoListContext _db;
public ItemsController(ToDoListContext db)
{
_db = db;
}
public ActionResult Index()
{
List<Item> model = _db.Items.ToList();
return View(model);
}
}
}
Controllers/HomeController.cs
using Microsoft.AspNetCore.Mvc;
namespace ToDoList.Controllers
{
public class HomeController : Controller
{
[HttpGet("/")]
public ActionResult Index()
{
return View();
}
}
}
Views/Items/Index.cshtml
@{
Layout = "_Layout";
}
@using ToDoList.Models;
<h1>Items</h1>
@if (@Model.Count == 0)
{
<h3>No items have been added yet!</h3>
}
@foreach (Item item in Model)
{
<li>@item.Description</li>
}
Create and Read with EF Core
Controllers/ItemsController.cs
using Microsoft.AspNetCore.Mvc;
using ToDoList.Models;
using System.Collections.Generic;
using System.Linq;
namespace ToDoList.Controllers
{
public class ItemsController : Controller
{
private readonly ToDoListContext _db;
public ItemsController(ToDoListContext db)
{
_db = db;
}
public ActionResult Index()
{
List<Item> model = _db.Items.ToList();
return View(model);
}
public ActionResult Create()
{
return View();
}
[HttpPost]
public ActionResult Create(Item item)
{
_db.Items.Add(item);
_db.SaveChanges();
return RedirectToAction("Index");
}
}
}
This content originally appeared on DEV Community and was authored by Saoud
Print
Share
Comment
Cite
Upload
Translate
Updates
There are no updates yet.
Click the Upload button above to add an update.
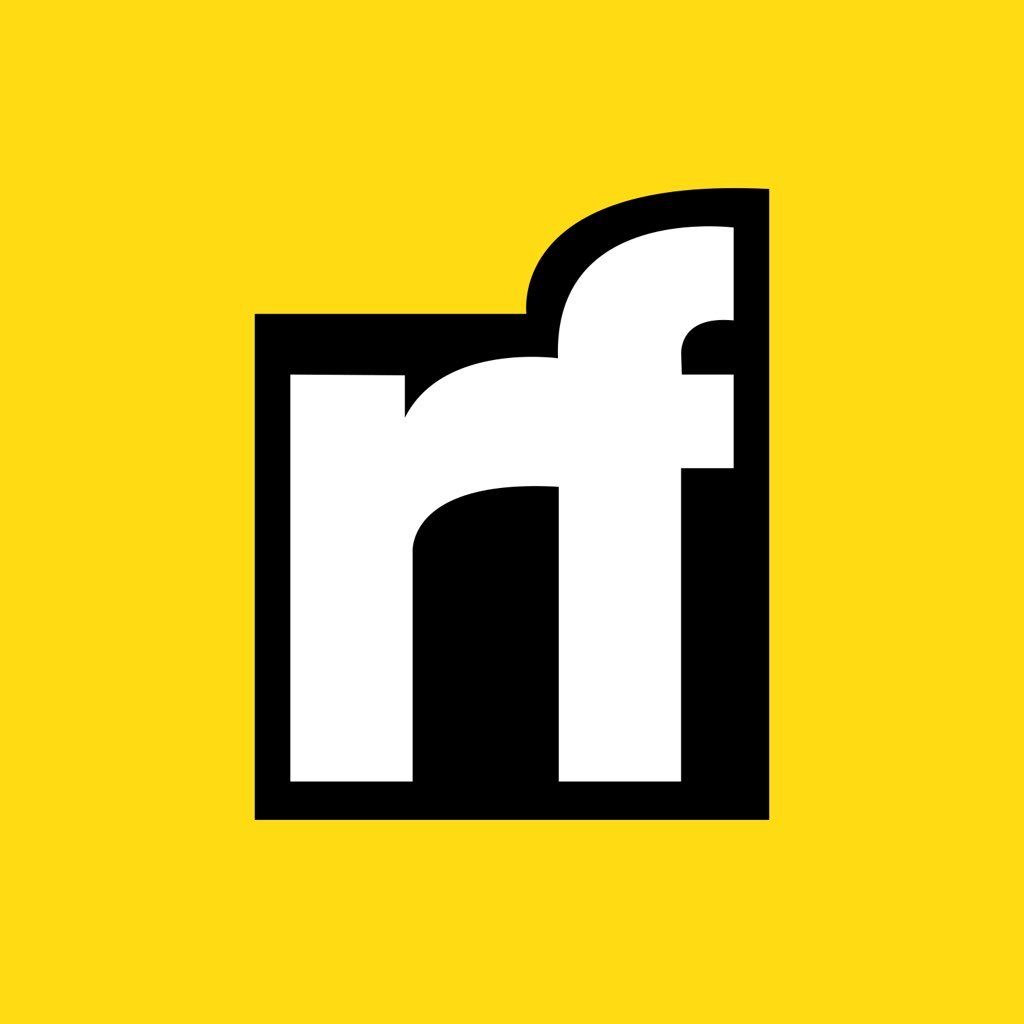
APA
MLA
Saoud | Sciencx (2021-05-09T22:14:43+00:00) Database Basics. Retrieved from https://www.scien.cx/2021/05/09/database-basics/
" » Database Basics." Saoud | Sciencx - Sunday May 9, 2021, https://www.scien.cx/2021/05/09/database-basics/
HARVARDSaoud | Sciencx Sunday May 9, 2021 » Database Basics., viewed ,<https://www.scien.cx/2021/05/09/database-basics/>
VANCOUVERSaoud | Sciencx - » Database Basics. [Internet]. [Accessed ]. Available from: https://www.scien.cx/2021/05/09/database-basics/
CHICAGO" » Database Basics." Saoud | Sciencx - Accessed . https://www.scien.cx/2021/05/09/database-basics/
IEEE" » Database Basics." Saoud | Sciencx [Online]. Available: https://www.scien.cx/2021/05/09/database-basics/. [Accessed: ]
rf:citation » Database Basics | Saoud | Sciencx | https://www.scien.cx/2021/05/09/database-basics/ |
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.