This content originally appeared on Adam Silver and was authored by Adam Silver
ECMAScript doesn’t have an inherit function. But you can mimic the functionality yourself if you need to.
Here’s a ready made inherit function you can use in your project:
// namespace
var lib = {};
// cloneObject function
// For browsers that have Object.create
if(Object.create) {
lib.cloneObject = function(o) {
return Object.create(o);
};
} else {
lib.cloneObject = (function() {
var Fn = function() {};
return function(o) {
Fn.prototype = o;
return new Fn();
};
})();
}
// innherit function
lib.inherit = function(Sub, Super) {
// Clone parent's prototype to child's prototype
Sub.prototype = lib.cloneObject(Super.prototype);
// Assign super constructor to child constructor
Sub.superConstructor = Super;
// Assign child as the child constructor
Sub.prototype.constructor = Sub;
};
As an example you might want a superhero to inherit the features of a regular person. A person constructor might look like this:
function Person(name) {
this.name = name;
}
Person.prototype.sayName = function() {
return "My name is:" + this.name;
};
var bob = new Person('Bob');
bob.sayName(); // "My name is Bob"
A superhero has some differences. They won’t tell you their identity. Instead they will tell you their alias. The code for this is as follows:
function Superhero(name, alias) {
// call parent constructor so Superheros have name
Superhero.superConstructor.call(this, name);
this.alias = alias;
}
// Inherit the features of a Person
lib.inherit(Superhero, Person);
// Override method so that Superheros only tell you their alias
Superhero.prototype.sayName = function() {
return "Can't tell you that but my alias is: " + this.alias;
}
// Call parent method if you need to
Superhero.prototype.revealIdentity = function() {
return Superhero.superConstructor.prototype.sayName();
};
var batman = new Superhero("Bruce Wayne", "Batman");
batman.sayName(); // "Can't tell you that but my alias is Batman"
batman.revealIdentity(); // "My name is Bruce Wayne"
This content originally appeared on Adam Silver and was authored by Adam Silver
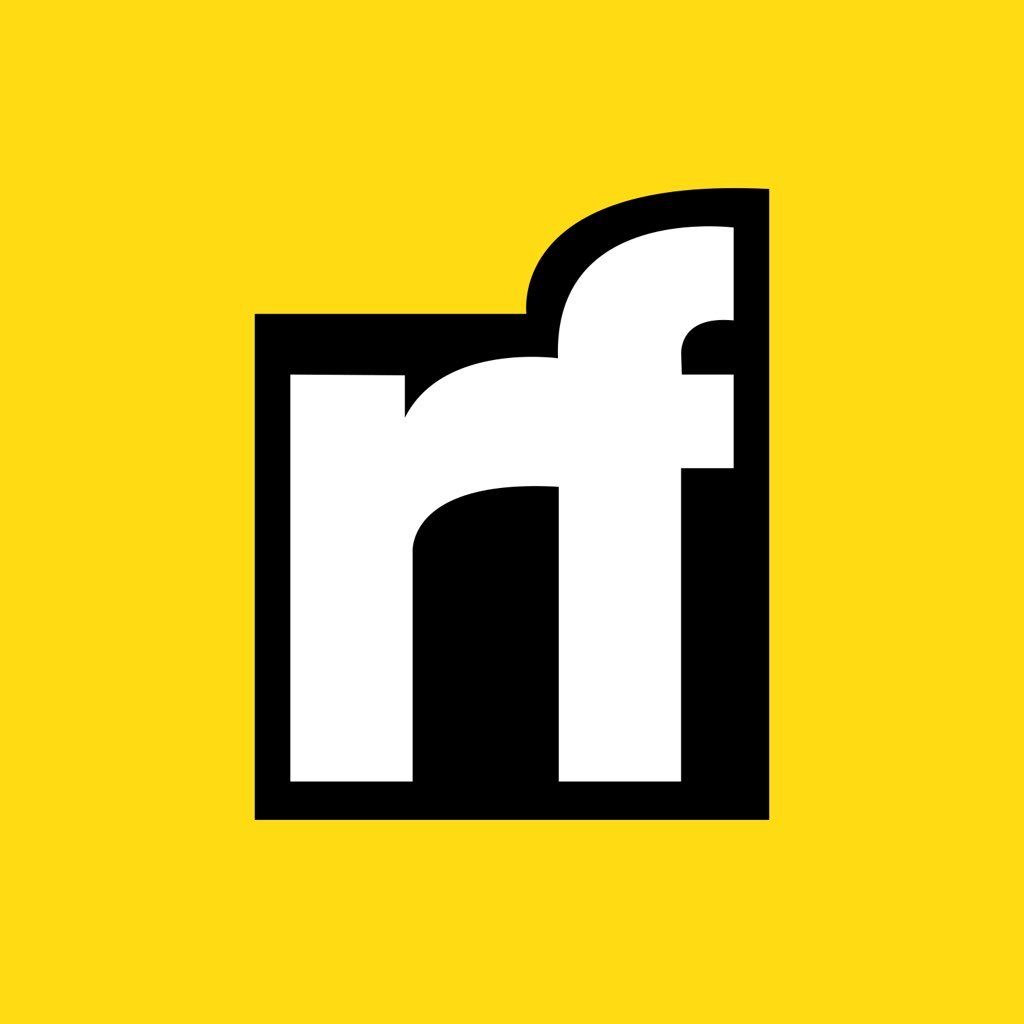
Adam Silver | Sciencx (2014-09-09T09:00:59+00:00) JavaScript inheritance. Retrieved from https://www.scien.cx/2014/09/09/javascript-inheritance/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.