This content originally appeared on DEV Community and was authored by RajeshKumarYadav.com
Step 1 - Install dependancies for toastr and animation in your project.
Open your terminal in project folder and run below command -
npm install ngx-toastr --save
@angular/animations package is a required dependency for the default toast
npm install @angular/animations --save
Step 2 - Add Toastr Styles in your project
Add few styles based on your requirements -
/ regular style toast
@import '~ngx-toastr/toastr';
// bootstrap style toast
// or import a bootstrap 4 alert styled design (SASS ONLY)
// should be after your bootstrap imports, it uses bs4 variables, mixins, functions
@import '~ngx-toastr/toastr-bs4-alert';
// if you'd like to use it without importing all of bootstrap it requires
@import '~bootstrap/scss/functions';
@import '~bootstrap/scss/variables';
@import '~bootstrap/scss/mixins';
@import '~ngx-toastr/toastr-bs4-alert';
If you are using angular-cli you can add it to your angular.json
"styles": ["styles.scss", "node_modules/ngx-toastr/toastr.css" // try adding '../' if you're using angular cli before 6]
Step 3 - add ToastrModule
to app NgModule
, make sure you have BrowserAnimationsModule
as well
app.module.ts
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { BrowserAnimationsModule } from '@angular/platform-browser/animations';
import { ToastrModule, ToastContainerModule } from 'ngx-toastr'; import { AppComponent } from './app.component';
@NgModule({
declarations: [AppComponent],
imports: [
BrowserModule, BrowserAnimationsModule,
ToastrModule.forRoot({ positionClass: 'inline' }),
ToastContainerModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule {}
Step 4 - Add a div with toastContainer directive on it.
CODE:
import { Component, OnInit, ViewChild } from '@angular/core'; import { ToastContainerDirective, ToastrService } from 'ngx-toastr';
@Component({
selector: 'app-root',
template: ` <h1><a (click)="onClick()">Click</a></h1> <div toastContainer></div>`
})
export class AppComponent implements OnInit {
@ViewChild(ToastContainerDirective, {static: true}) toastContainer: ToastContainerDirective;
constructor(private toastrService: ToastrService) {}
ngOnInit() {
this.toastrService.overlayContainer = this.toastContainer;
}
onClick() {
this.toastrService.success('in div');
}
}
Extra Bits-
How to handle toastr click/tap action?
showToaster() {
this.toastr.success('Hello world!', 'Toastr fun!').onTap.pipe(take(1)).subscribe(() => this.toasterClickedHandler());
}
toasterClickedHandler() {
console.log('Toastr clicked');
}
With all that being said, I highly recommend you keep learning!
Thank you for reading this article. Please feel free to connect with me on LinkedIn and Twitter.
This content originally appeared on DEV Community and was authored by RajeshKumarYadav.com
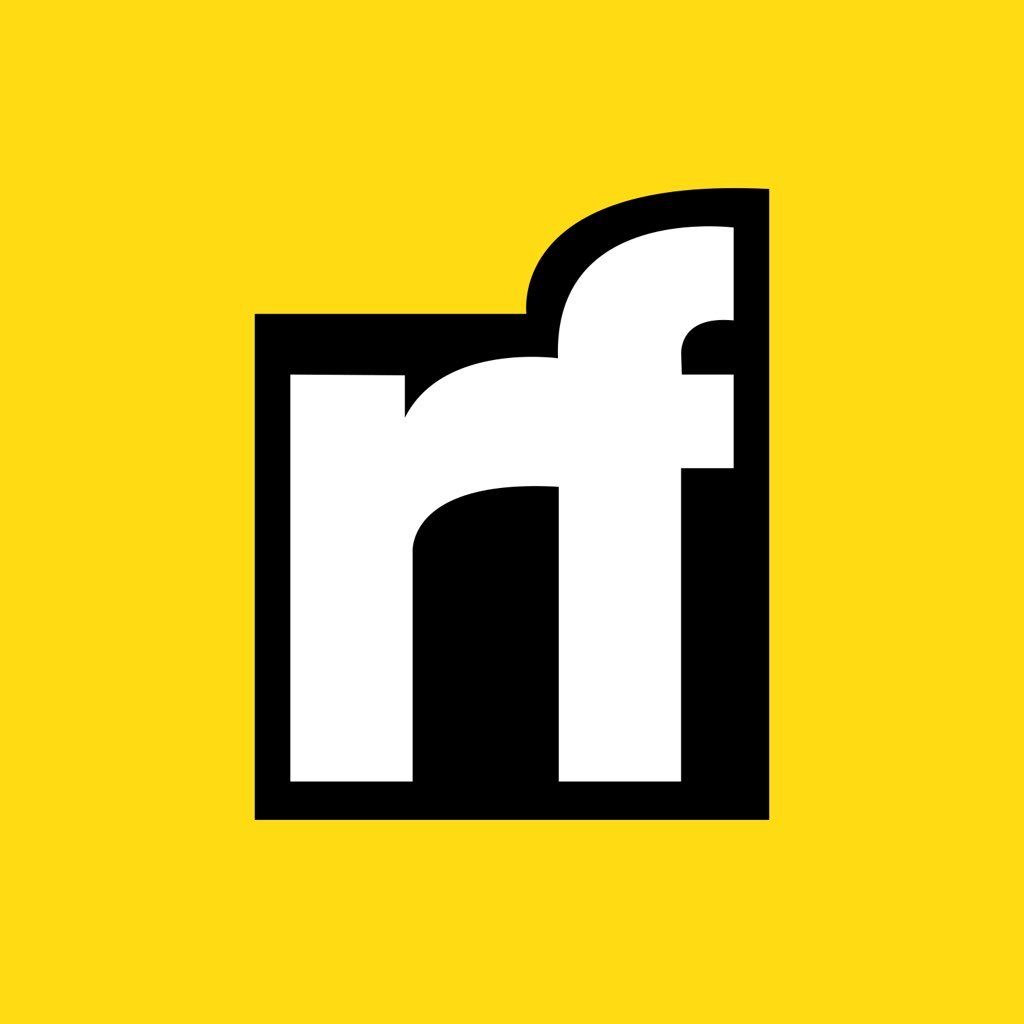
RajeshKumarYadav.com | Sciencx (2021-06-10T05:16:44+00:00) Angular : How to add Toastr in your angular project just in 15 minutes?. Retrieved from https://www.scien.cx/2021/06/10/angular-how-to-add-toastr-in-your-angular-project-just-in-15-minutes/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.