This content originally appeared on DEV Community and was authored by Nelson Adonis Hernandez
For this example we will use Authlib which is the ultimate Python library in building OAuth and OpenID Connect servers
Installation
pip3 install Flask Authlib requests python-dotenv
Configuration
from flask import Flask, url_for, redirect
from dotenv import load_dotenv
from os import getenv
from authlib.integrations.flask_client import OAuth
app = Flask(__name__)
app.secret_key = "mysecretkey"
oauth = OAuth(app)
github = oauth.register(
name='github',
client_id=getenv("CLIENT_ID"),
client_secret=getenv("SECRET_ID"),
access_token_url='https://github.com/login/oauth/access_token',
access_token_params=None,
authorize_url='https://github.com/login/oauth/authorize',
authorize_params=None,
api_base_url='https://api.github.com/',
client_kwargs={'scope': 'user:email'},
)
@app.route("/")
def saludo():
return "Hello"
if __name__ == '__main__':
load_dotenv()
app.run(debug=True, port=4000, host="0.0.0.0")
Route for Authorization
authorize_redirect indicates the url to redirect to the "Callback URL"
@app.route("/login")
def login():
redirect_url = url_for("authorize", _external=True)
return github.authorize_redirect(redirect_url)
Callback URL
@app.route("/authorize")
def authorize():
token = github.authorize_access_token()
resp = github.get('user', token=token)
profile = resp.json()
# do something with the token and profile
print(profile, token)
return redirect('/')
Settings in Github
This content originally appeared on DEV Community and was authored by Nelson Adonis Hernandez
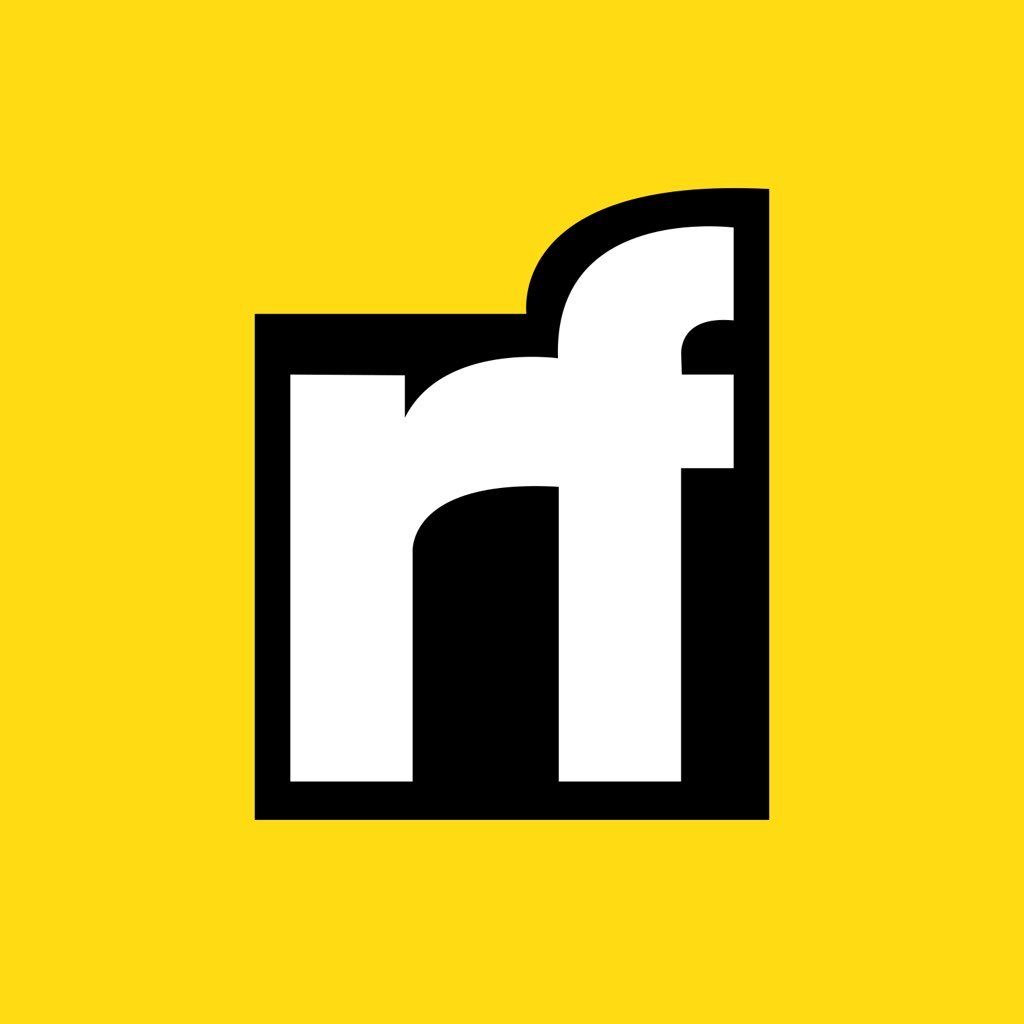
Nelson Adonis Hernandez | Sciencx (2021-08-10T02:09:08+00:00) Authentication with Flask and Github || Authlib. Retrieved from https://www.scien.cx/2021/08/10/authentication-with-flask-and-github-authlib/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.