This content originally appeared on DEV Community and was authored by Aya Bouchiha
Sending a PUT request using then & catch
const putTodo = (todo) => {
const method = 'PUT';
const headers = {
'Content-type': 'application/json; charset=UTF-8',
'header-name': 'header-value',
};
fetch('https://jsonplaceholder.typicode.com/posts/1', {
method,
headers,
body: JSON.stringify(todo),
})
.then((response) => response.json())
.then((data) => console.log(data))
.catch((e) => console.log('something went wrong :(', e));
};
const data = {
id: 1,
title: 'this is a title',
body: 'body!',
userId: 13,
};
putTodo(data);
Console:
{id: 1, title: "this is a title", body: "body!", userId: 13}
Sending a PUT request using async & await
const putTodo = async (todo) => {
const method = 'PUT';
const headers = {
'Content-type': 'application/json; charset=UTF-8',
'header-name': 'header-value',
};
try {
const response = await fetch(
'https://jsonplaceholder.typicode.com/posts/1',
{ method, headers, body: JSON.stringify(todo) },
);
const data = await response.json();
console.log(data);
} catch (e) {
console.log('something went wrong :(', e);
}
};
const data = {
id: 1,
title: 'this is a title',
body: 'body!',
userId: 13,
};
putTodo(data);
Console:
{id: 1, title: "this is a title", body: "body!", userId: 13}
Have a nice day!
This content originally appeared on DEV Community and was authored by Aya Bouchiha
Print
Share
Comment
Cite
Upload
Translate
Updates
There are no updates yet.
Click the Upload button above to add an update.
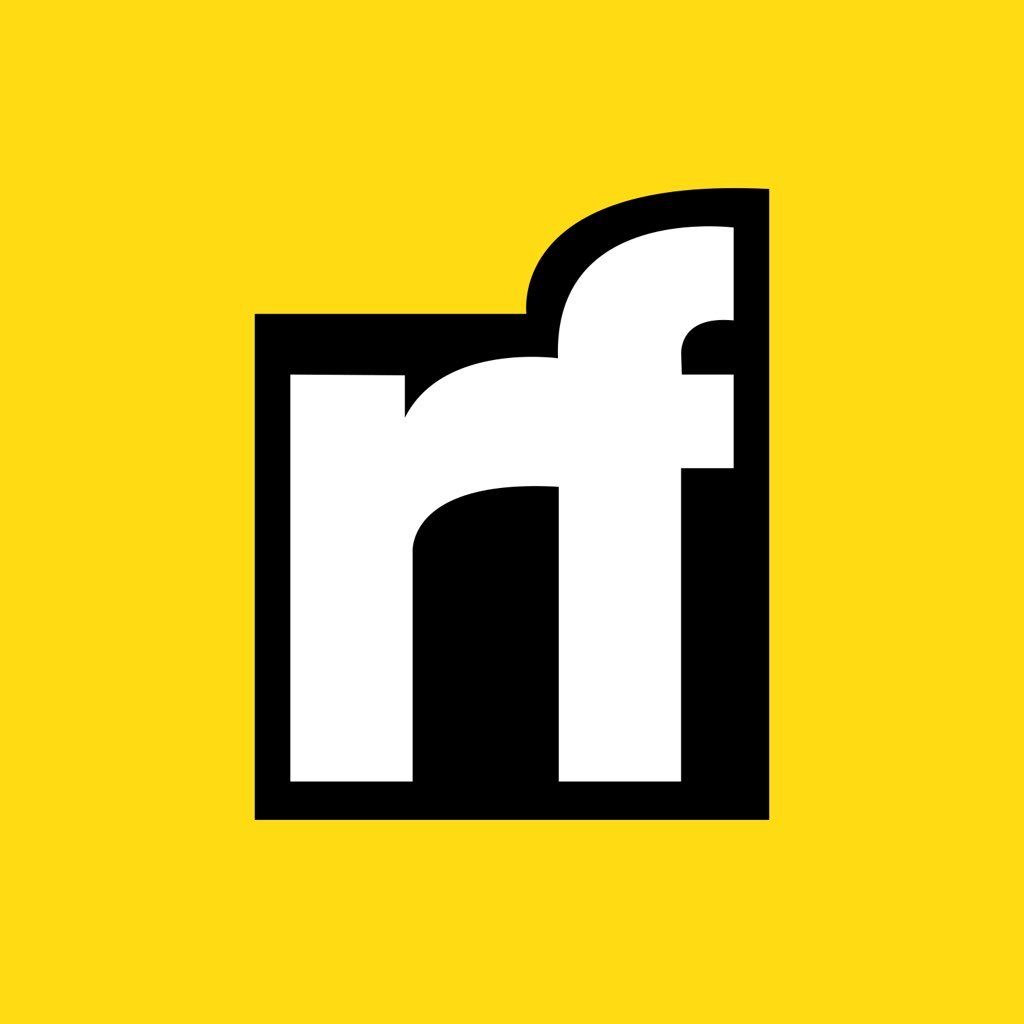
APA
MLA
Aya Bouchiha | Sciencx (2021-08-19T23:39:59+00:00) Sending PUT Request Using fetch. Retrieved from https://www.scien.cx/2021/08/19/sending-put-request-using-fetch/
" » Sending PUT Request Using fetch." Aya Bouchiha | Sciencx - Thursday August 19, 2021, https://www.scien.cx/2021/08/19/sending-put-request-using-fetch/
HARVARDAya Bouchiha | Sciencx Thursday August 19, 2021 » Sending PUT Request Using fetch., viewed ,<https://www.scien.cx/2021/08/19/sending-put-request-using-fetch/>
VANCOUVERAya Bouchiha | Sciencx - » Sending PUT Request Using fetch. [Internet]. [Accessed ]. Available from: https://www.scien.cx/2021/08/19/sending-put-request-using-fetch/
CHICAGO" » Sending PUT Request Using fetch." Aya Bouchiha | Sciencx - Accessed . https://www.scien.cx/2021/08/19/sending-put-request-using-fetch/
IEEE" » Sending PUT Request Using fetch." Aya Bouchiha | Sciencx [Online]. Available: https://www.scien.cx/2021/08/19/sending-put-request-using-fetch/. [Accessed: ]
rf:citation » Sending PUT Request Using fetch | Aya Bouchiha | Sciencx | https://www.scien.cx/2021/08/19/sending-put-request-using-fetch/ |
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.