This content originally appeared on DEV Community and was authored by 101samovar
Suppose we have two code snippets that do the same thing.
How to check which one is faster?
Let we have an array of numbers.
And we have to do mathematical evaluation for each item.
There is more than one way to do it.
One way is to use a map method and an arrow function.
The other way is to use a for loop and a push method.
So we have two code snippets for one task.
Which one is faster?
Let’s define the benchmark function.
Function has one parameter: code snippet.
In that function we will repeat code snippet execution multiple times.
const array = [1, 2, 3, 4, 5, 6];
const snippetA = () => {array.map(x => Math.sin(x))};
const snippetB = () => {
let result = [];
for (let i = 0; i < array.length; i++) {
result.push(Math.sin(array[i]));
}
return result;
};
const benchmark = snippet => {
const count = 1000000;
let start = new Date();
for (let i = 0; i < count; i++) {
snippet();
}
return new Date() - start;
};
console.log('map: ', benchmark(snippetA));
console.log('for: ', benchmark(snippetB));
And we check how much time it requires.
The less time period the faster the code snippet.
OK.
Let’s check it out.
The snippet A with a map method.
And the snippet B with a for loop.
The snippet B has required less time.
The for snippet is faster.
I hope you found this article useful, if you need any help please let me know in the comment section.
? See you next time. Have a nice day!
Subscribe to our channel:
https://www.youtube.com/c/Samovar101
This content originally appeared on DEV Community and was authored by 101samovar
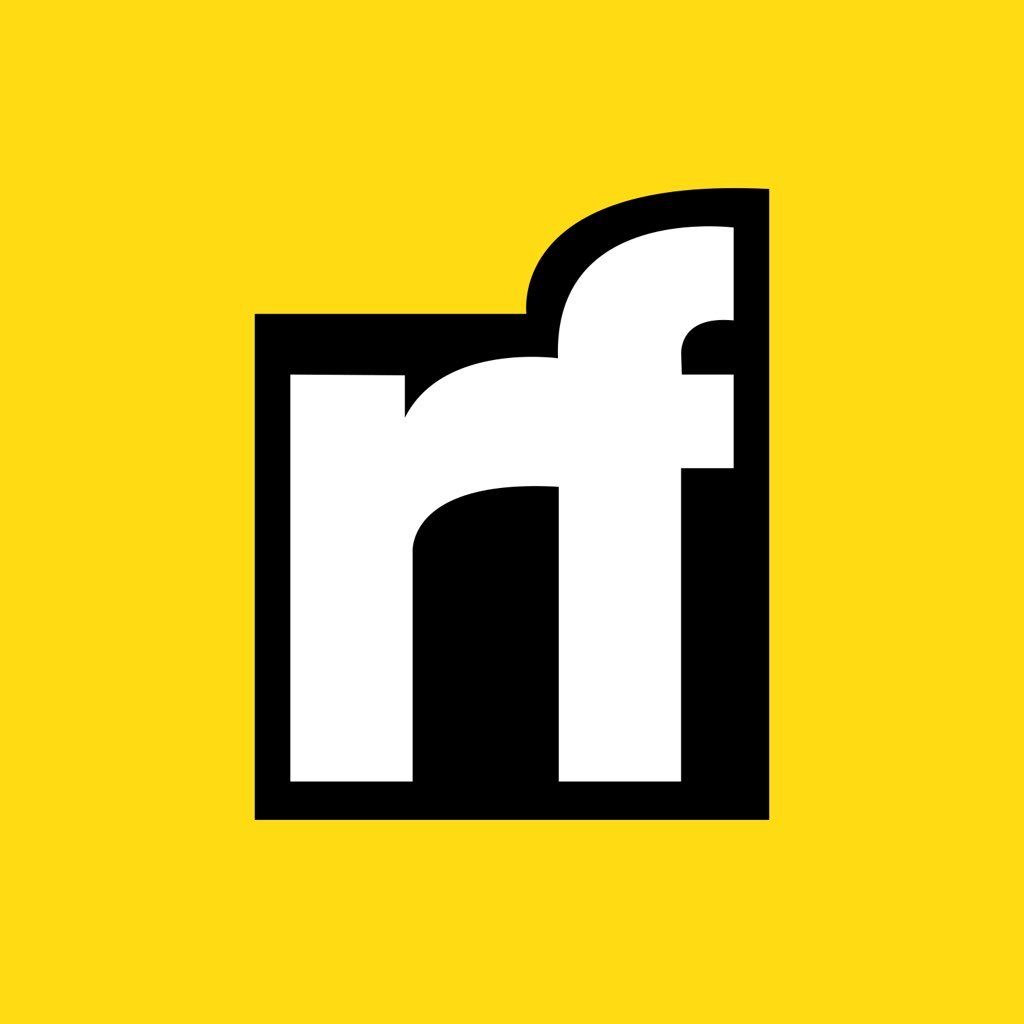
101samovar | Sciencx (2021-09-26T17:57:30+00:00) How fast your code is?. Retrieved from https://www.scien.cx/2021/09/26/how-fast-your-code-is/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.