This content originally appeared on DEV Community and was authored by Walter Nascimento
[Clique aqui para ler em português]
Let’s create an autocomplete so that clicking on an input displays a suggestion list
Code
First let’s create the interface, we’ll do something simple, using just HTML.
<div>
<label for="input">Input</label>
<input type="text" id="input" />
<ul id="suggestions"></ul>
</div>
We have a div and inside we have a label, an input and a ul, this input will be where we will type the information and when the information matches the list we have it will be displayed in ul
(function () {
"use strict";
let inputField = document.getElementById('input');
let ulField = document.getElementById('suggestions');
inputField.addEventListener('input', changeAutoComplete);
ulField.addEventListener('click', selectItem);
function changeAutoComplete({ target }) {
let data = target.value;
ulField.innerHTML = ``;
if (data.length) {
let autoCompleteValues = autoComplete(data);
autoCompleteValues.forEach(value => { addItem(value); });
}
}
function autoComplete(inputValue) {
let destination = ["Italy", "Spain", "Portugal", "Brazil"];
return destination.filter(
(value) => value.toLowerCase().includes(inputValue.toLowerCase())
);
}
function addItem(value) {
ulField.innerHTML = ulField.innerHTML + `<li>${value}</li>`;
}
function selectItem({ target }) {
if (target.tagName === 'LI') {
inputField.value = target.textContent;
ulField.innerHTML = ``;
}
}
})();
We have four functions:
- changeAutoComplete = In this function we will have the input values, we check if there is any text, we call the autocomplete function, with the return of the autocomplete function we do a loop and add the item using the additem() function;
- autoComplete = In this function we have a target array and with the data passed we check if the typed text exists in some value of the target array, if it exists it is returned;
- addItem = Here the received value is added directly to ul;
- selectItem = This function is activated by clicking on the item list, thus directly choosing the selected item;
ready simple like that.
Demo
See below for the complete working project.
Youtube
If you prefer to watch it, see the development on youtube.
References:
Thanks for reading!
If you have any questions, complaints or tips, you can leave them here in the comments. I will be happy to answer!
😊😊 See you later! 😊😊
This content originally appeared on DEV Community and was authored by Walter Nascimento
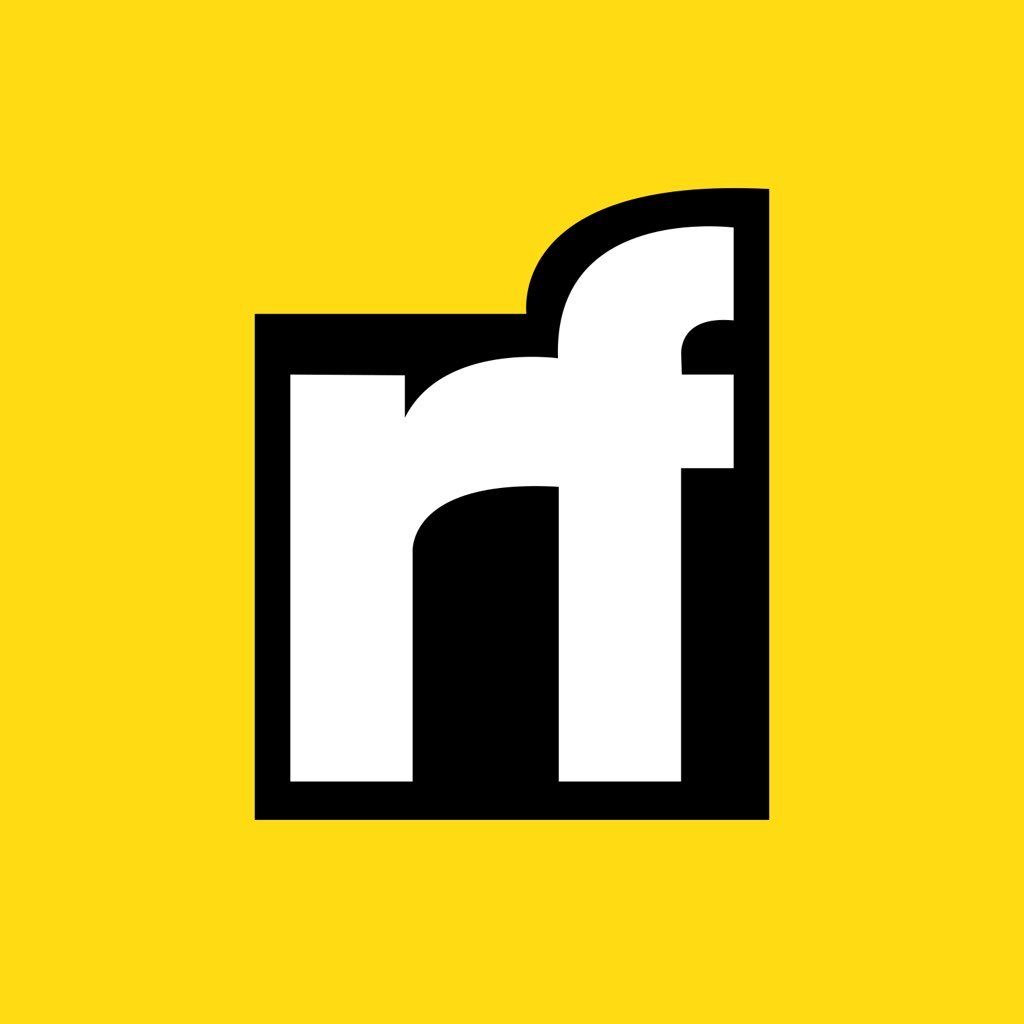
Walter Nascimento | Sciencx (2021-11-19T19:39:55+00:00) AutoComplete with JS. Retrieved from https://www.scien.cx/2021/11/19/autocomplete-with-js/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.