This content originally appeared on DEV Community and was authored by Santhosh Balasa
Binary Tree:
(4)
/ \
/ \
(2) (7)
/ \ / \
/ \ (6) (9)
(1) (3)
Inverting a Binary tree means mirroring the tree, i.e, swapping the children from left to right and vice versa.
Inverted Binary Tree:
(4)
/ \
/ \
(7) (2)
/ \ / \
/ \ (3) (1)
(9) (6)
This can be easily achieved using recursion,
Code:
tree = {
4: [2, 7],
2: [1, 3],
7: [6, 9],
1: [],
3: [],
6: [],
9: []
}
def invert_tree(tree, node, path=[]):
if tree[node]:
path.extend(tree[node][::-1])
invert_tree(tree, tree[node][1], path)
invert_tree(tree, tree[node][0], path)
return [node] + path
This content originally appeared on DEV Community and was authored by Santhosh Balasa
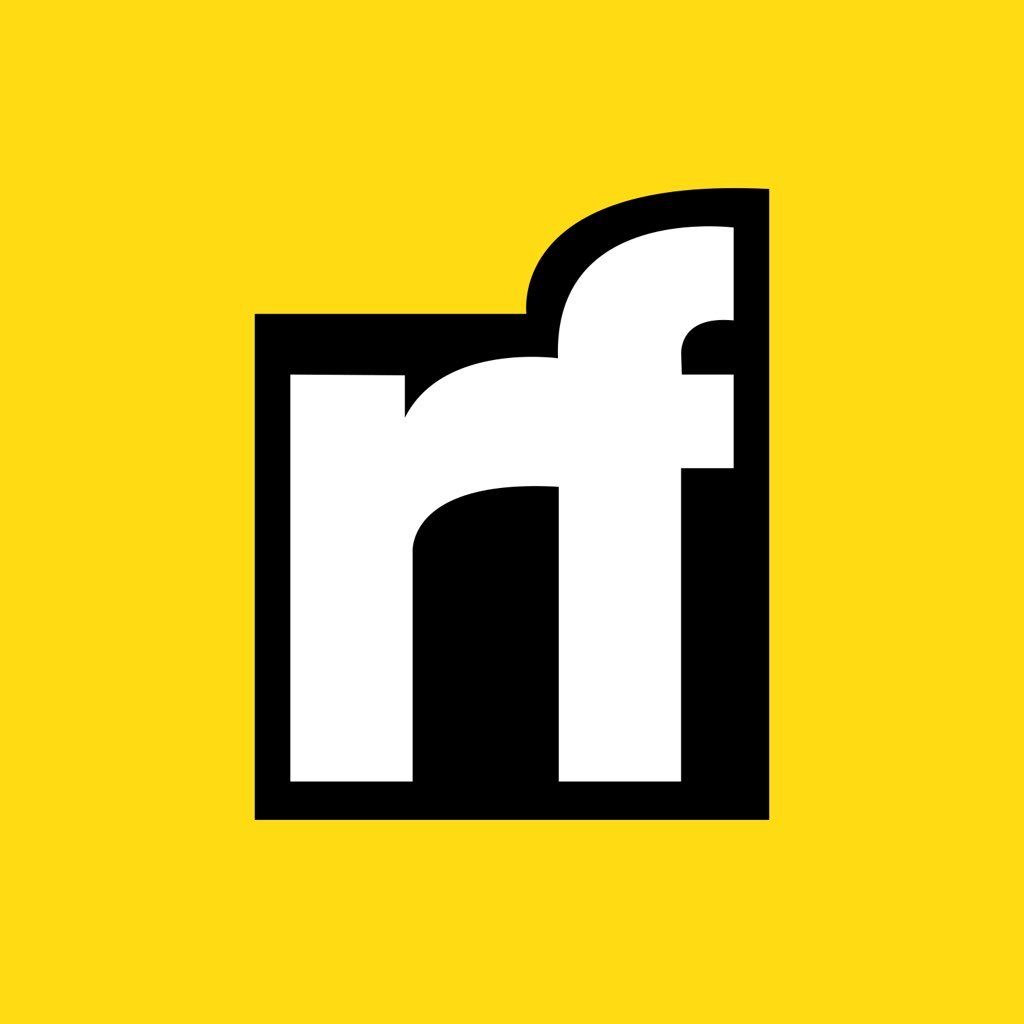
Santhosh Balasa | Sciencx (2021-12-23T11:06:41+00:00) Inverting a Binary Tree. Retrieved from https://www.scien.cx/2021/12/23/inverting-a-binary-tree/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.