This content originally appeared on flaviocopes.com and was authored by flaviocopes.com
This post is part of a new series where we build a clone of Airbnb with Next.js. See the first post here.
- Part 1: Let’s start by installing Next.js
- Part 2: Build the list of houses
- Part 3: Build the house detail view
- Part 4: CSS and navigation bar
- Part 5: Start with the date picker
- Part 6: Add the sidebar
- Part 7: Add react-day-picker
- Part 8: Add the calendar to the page
- Part 9: Configure the DayPickerInput component
- Part 10: Sync the start and end dates
- Part 11: Show the price for the chosen dates
- Part 12: Login and signup forms
- Part 13: Activate the modal
- Part 14: Send registration data to the server
- Part 15: Add postgres
- Part 16: Implement model and DB connection
- Part 17: Create a session token
- Part 18: Implement login
- Part 19: Determine if we are logged in
- Part 20: Change state after we login
- Part 21: Log in after registration
- Part 22: Create the models and move data to the db
- Part 23: Use the database instead of the file
- Part 24: Handle bookings
- Part 25: Handle booked dates
- Part 26: Prevent booking if already booked
- Part 27: Adding Stripe for payments
- Part 28: Airbnb clone, handling Stripe webhooks
- Part 29: Airbnb clone, view bookings
Clearing unpaid bookings
Now we have a little issue. Since we book dates before paying, some people might not complete the payment (it will happen!) and this leaves us with booked dates that are not really confirmed.
To solve this, we need to clear out bookings from time to time.
I made a POST endpoint to do this, in api/clean.js
:
server.js
import { Booking } from '../../model.js'
export default async (req, res) => {
if (req.method !== 'POST') {
res.status(405).end() //Method Not Allowed
return
}
Booking.destroy({
where: {
paid: false
}
})
res.writeHead(200, {
'Content-Type': 'application/json'
})
res.end(
JSON.stringify({
status: 'success',
message: 'ok'
})
)
}
It calls Booking.destroy
to remove all unpaid bookings.
Ideally you’d remove all bookings that have been created, say, more than 1 hour ago, and are still unpaid.
This needs some refinement, but it’s good for us now.
I added an Insomnia POST request to help me clean the database, manually:
This content originally appeared on flaviocopes.com and was authored by flaviocopes.com
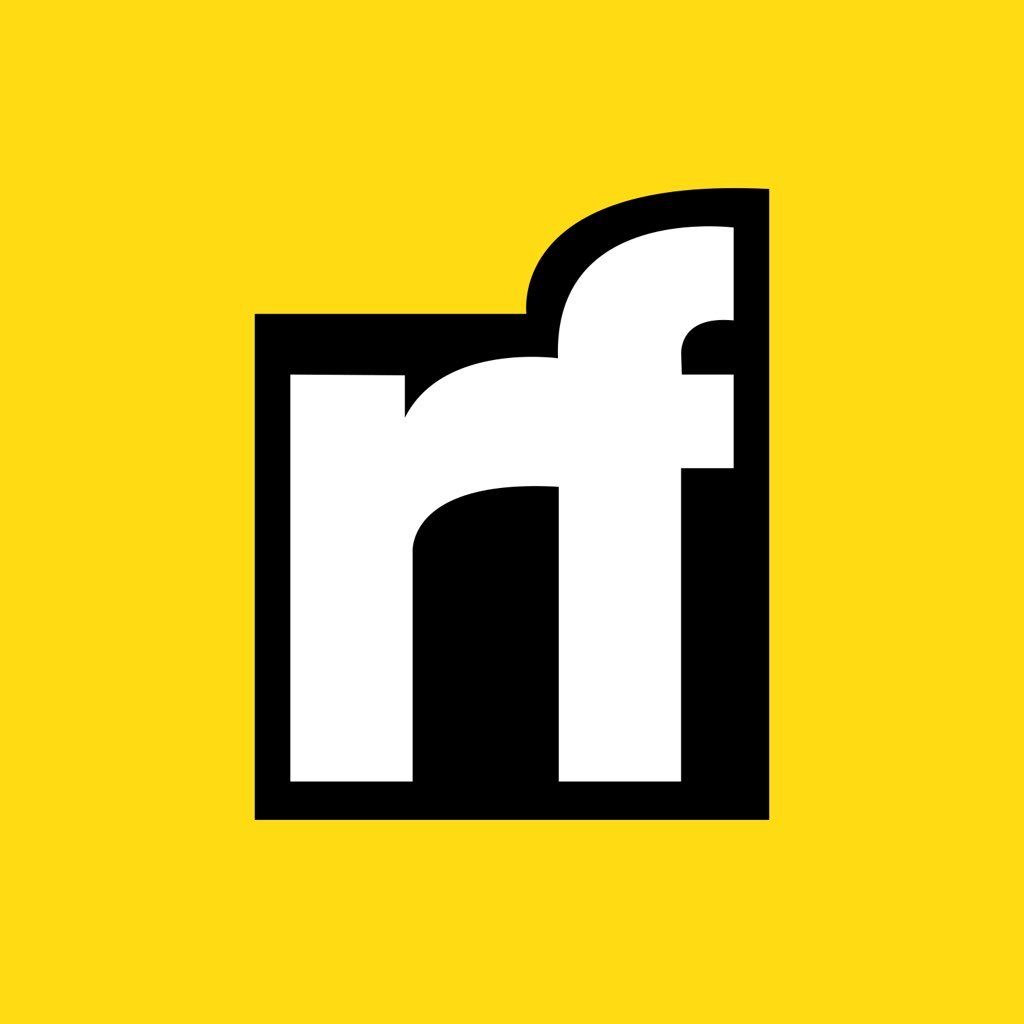
flaviocopes.com | Sciencx (2021-12-30T05:00:00+00:00) Airbnb clone, clean bookings. Retrieved from https://www.scien.cx/2021/12/30/airbnb-clone-clean-bookings/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.