This content originally appeared on Level Up Coding - Medium and was authored by Anas Reza
As a front-end developer, in every React project, we must be calling API’s in multiple components to get their respective data. In addition to it, we also want to handle error scenarios and set loaders in the background.
Suppose we have multiple components where we want to fetch some data from the server and display it on UI. Writing the same code in every component is definitely not recommended. To avoid such cases, we can use axios as a custom hook.
Let’s create a useAxios (Custom Hook)
import { useEffect, useState } from "react";
import axios from 'axios';
const useAxios = (configParams) => {
axios.defaults.baseURL = 'https://jsonplaceholder.typicode.com';
const [res, setRes] = useState('');
const [err, setErr] = useState('');
const [loading, setLoading] = useState(true);
useEffect(() => {
fetchDataUsingAxios(configParams);
}, []);
const fetchDataUsingAxios = async() => {
await axios.request(configParams)
.then(res => setRes(res)
.catch(err => setErr(err))
.finally(() => setLoading(false));
}
return [res, err, loading];
}
export default useAxios;
- We’ve set the default base URL so that any kind of request(get/post/..) will be made to this end point(https://jsonplaceholder.typicode.com) only.
- We’ve created three states(response, error, and loading).
- We’ve used async and await to make asynchronous HTTP requests so that it won’t block any other thread.
- axios.request is very useful for making any kind of HTTP request since it only expects config{url, method, body, headers} object and rest will be handled automatically.
- We could’ve also used axios.get or axios.post instead. The choice is yours.
Let’s move to the second part, how to use useAxios in any of your component
import { useEffect, useState } from "react/cjs/react.development";
import useAxios from "./useAxios";
const YourComponent = () => {
const [data, setData] = useState(null);
const [todo, isError, isLoading] = useAxios({
url: '/todos/2',
method: 'get',
body: {...},
headers: {...}
});
use Effect(() => {
if(todo && todo.data) setData(todo.data)
}, [todo]);
return (
<> {isLoading ? (
<p>isLoading...</p>
) : (
<div>
{isError && <p>{isError.message}</p>}
{data && <p>{data.title}</p>}</div>
</div>
)} </>
)
}
export default YourComponent;
Here we have created a config object consisting of {url, method, body, and headers} and passed it to the useAxios({….}) which will make the HTTP call and return the array of values [res, err, loading] and we’re storing those in [todo, isError, isLoading] through destructuring.
useEffect has a dependency check on “todo” variable, if any changes happen it will update the local state followed by UI.
Share your thoughts in the comments. Keep learning !!
Thanks for the read. Follow for more.
React Custom Hook: useAxios() was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Anas Reza
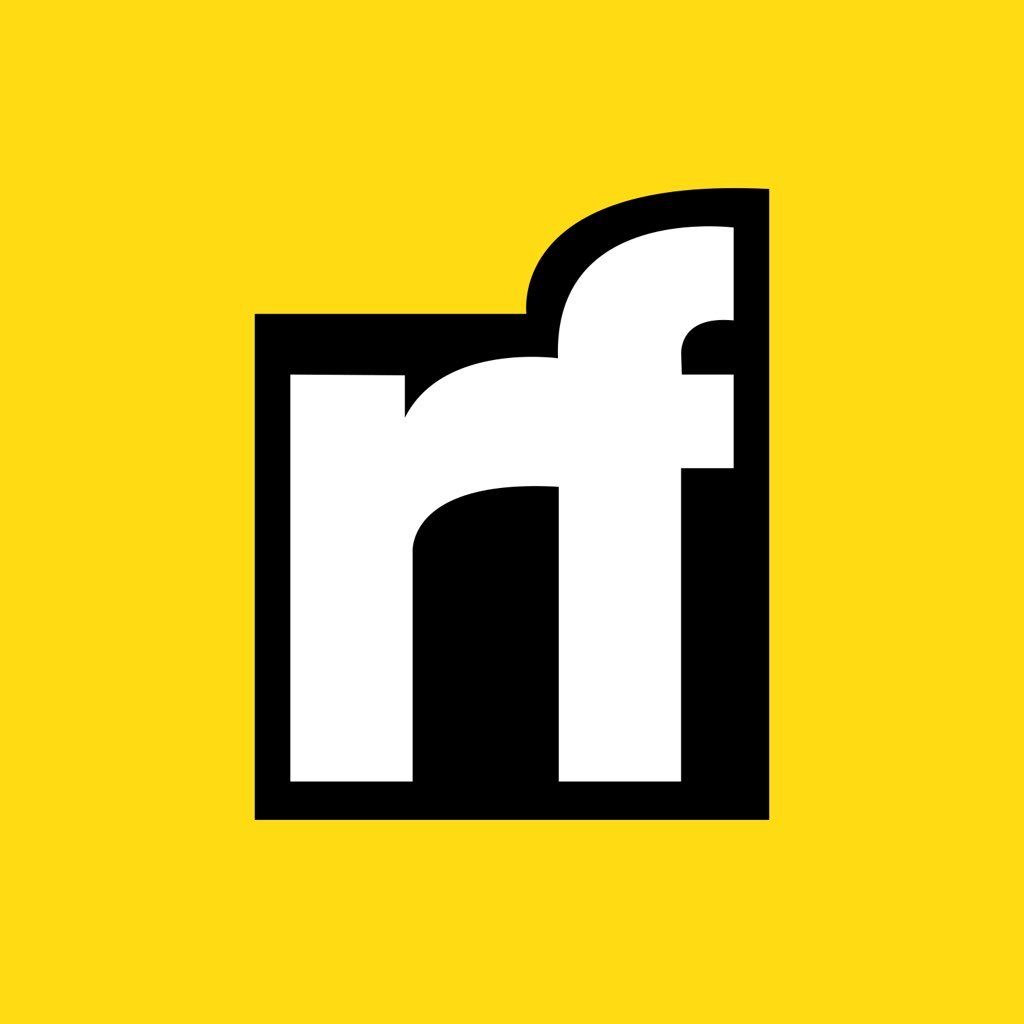
Anas Reza | Sciencx (2022-02-05T01:16:54+00:00) React Custom Hook: useAxios(). Retrieved from https://www.scien.cx/2022/02/05/react-custom-hook-useaxios/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.