This content originally appeared on Bits and Pieces - Medium and was authored by Ewumesh
How to retrieve a nested value from an object in JavaScript
Retrieving data from nested and deeply nested data structures can feel like a bit of a pain at times. So let’s look at ways that we can make this process easier.
Imagine we have the following data structure:
let data =
[
{
"testingNewValue": {
"en-US": "hello"
}
},
{
"testingNewValue": {
"hi-IN": "hello "
}
},
{
"testingNewValue": {
"us": "dummy"
}
},
{
"testingNewValue": {
"xp-op-as": "value for testing"
},
"locationField": {
"en-US": {
"lat": 19.28563,
"lon": 72.8691
}
}
}
]
const value = Object.fromEntries(Object.entries(data).map(el=>(el[1]=Object.values(el[1])[0], el)))
console.log(value);
We could try to use methods such as object.fromEntries and object.entries, but they’re not returning the data in the format that I want.
Ideally, I want something such as the following to be returned:
{
{
testingNewValue:"hello"
},
{
testingNewValue:"hello"
},
{
testingNewValue:"dummy"
},
{
testingNewValue:"value for testing",
locationField:{
lat: 19.28563,
lon: 72.8691
}
}
}
Providing that you’re actually after an array (as your current expected result isn’t a valid structure), you should be taking the entries of each inner objects and then building your new object with fromEntries()after mapping each entry, rather then taking the entries of your entire data array which is causing the indexes to appear in your current result.
The below code assumes you have one value within each inner object, and that each value is an object:
let data =
[
{
"testingNewValue": {
"en-US": "hello"
}
},
{
"testingNewValue": {
"hi-IN": "hello "
}
},
{
"testingNewValue": {
"us": "dummy"
}
},
{
"testingNewValue": {
"xp-op-as": "value for testing"
},
"locationField": {
"en-US": {
"lat": 19.28563,
"lon": 72.8691
}
}
}
]
const value = data.map(el=>
Object.fromEntries(Object.entries(el).map(([k, v]) => [k, Object.values(v)[0]]))
);
console.log(value);
Object.fromEntries()
The Object.fromEntries() method transforms a list of key-value pairs into an object.
const entries = new Map([
['foo', 'bar'],
['baz', 42]
]);
const obj = Object.fromEntries(entries);
console.log(obj);
// expected output: Object { foo: "bar", baz: 42 }
Object.values()
The Object.values() method returns an array of a given object's own enumerable property values, in the same order as that provided by a for...in loop.
The only difference is that a for...in loop enumerates properties in the prototype chain as well.
const object1 = {
a: 'somestring',
b: 42,
c: false
};
console.log(Object.values(object1));
// expected output: Array ["somestring", 42, false]
And that’s just a couple of ways that we can take a deeply nested data structure, and bring back something closer to the formatting we want. I hope you have found this useful. Be sure to let us know your thoughts in the comments.
Build anything from independent components
Say goodbye to monolithic applications and leave behind you the tears of their development.
The future is components; modular software that is faster, more scalable, and simpler to build together. OSS Tools like Bit offer a great developer experience for building independent components and composing applications. Many teams start by building their Design Systems or Micro Frontends, through shared components. Give it a try →
Get Deeply Nested Value in JavaScript was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Ewumesh
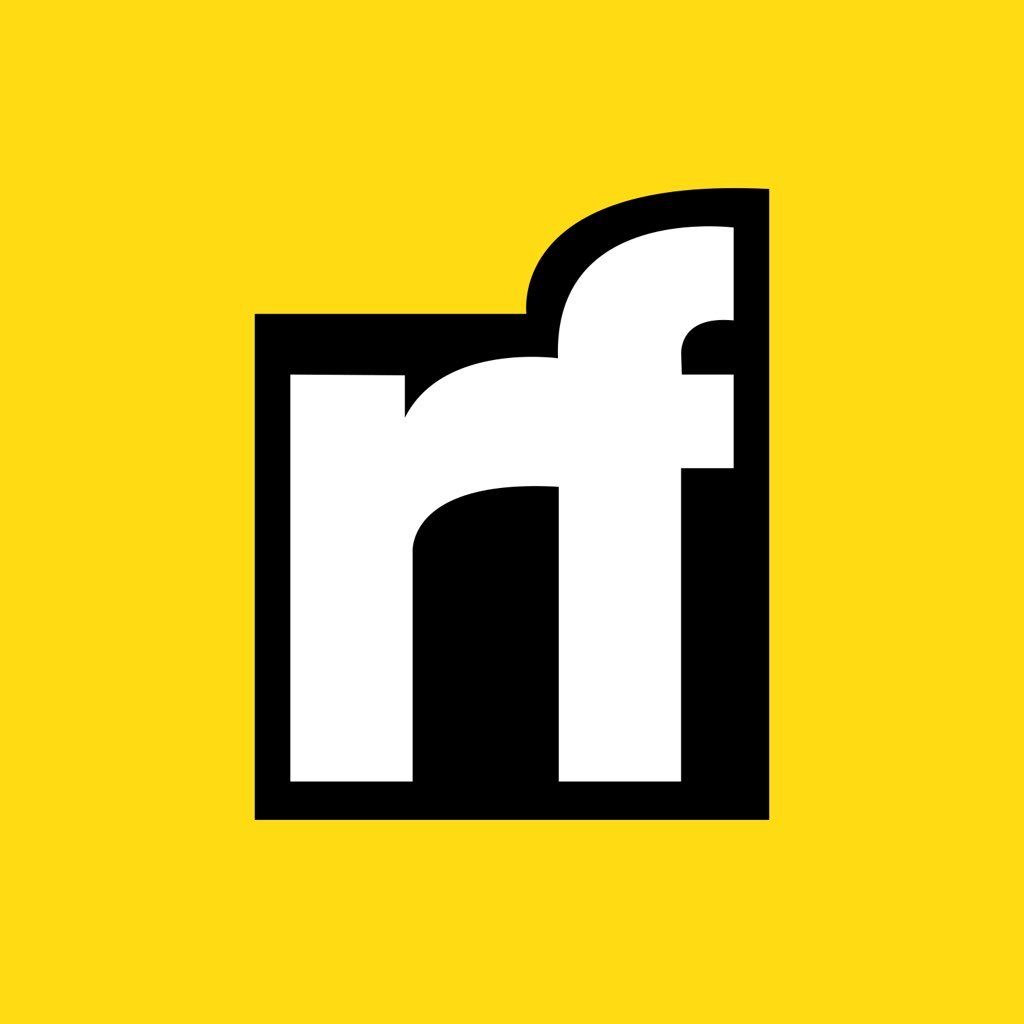
Ewumesh | Sciencx (2022-02-07T09:13:33+00:00) Get Deeply Nested Value in JavaScript. Retrieved from https://www.scien.cx/2022/02/07/get-deeply-nested-value-in-javascript/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.