This content originally appeared on Level Up Coding - Medium and was authored by Liz Catoe
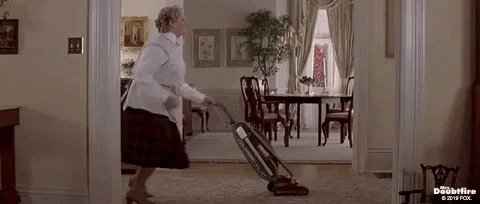
As they say in the restaurant industry, “Make it nice or make it twice”. Or in the case of controlled components, “Make it nice or make it for every input value you need to keep track of”. Not quite so catchy…
Consolidating your forms in react is not an intuitive skill at first. Often the easiest route seems to be creating a state variable for each input, throwing those specified key value pairs into a new object, and moving on. You can probably see how this could get tedious with multiple inputs.
So, time to learn to make our code work for us. Doesn’t that sound nice?
Let’s start with an example: a simple app to list items for sale.
Our goal is to be able to add our own item to the marketplace with a name, category, price, and contact information (let’s stick with an e-mail address for now).
First things first. We need to know how our data is structured in order to post a new object to the database. Take a look at what we’re working with:
Then it’s time to tackle our form. With this type of data, we are likely to have a text input for our name and email, a dropdown for our category, and just to keep us on our toes, an input with a “step” attribute for price. Something like this:
This code will give us a form that lets us select an option for category and type some text, but beyond that we aren’t doing much. We need access to the information that the user is providing. How do we do that? Well we could….
set the value of the name input to name, make a state variable for name, set it to an empty string, make a specific event listener for name, use the setName function to set the name to the value of the input, then set a key value pair of name:name inside of a new obj in our submit handler, set the value of the price input to price, make a state variable for price, set it to an empty string, make a specific event listener for price, use the setPrice function to set the price to the value of the input, then set a key value pair of price:price inside of a new obj in our submit handler, set the value of the email input to email, make a state variable for email, set it to an empty string, make a specific event listener for email, use the setEmail function to set the email to the value of the input, then set a key value pair of email:email inside of a new obj in our submit handler, make a state variable for category, set it to an empty string, make a specific event listener for the category select, use the setCategory function to set the category to the value of the input, then set a key value pair of category:category inside of a new obj in our submit handler, and add that new object to the array we want to display,
like so…..
Do you want to do that? I don’t want to do that.
Let’s do this instead.
First off, let’s create a state variable for our form data. We will need to set this to an object that includes all of our keys (except id - the Computer Gods take care of id.) with a values of empty strings.
Now, let’s set up some event handlers to handle our data when we access it. a simple ‘handleChange’ will do fine for our purposes. The purpose of this ONE function is to build out our new objects with the values that the user gives us. One way to do this would be to give each input tag a name attribute assigned to the name of the key it is associated with. The name attribute of our email input would be equal to email, for example.
You’ll notice we have also set a value attribute for each input to correspond with our formData’s appropriate value.
Now we can set our handle function to take care of all of our user input in one fell swoop using the values of the target’s name and value. We want to use the our name attributes as the keys in our formData object, and our value attributes as the value.
Then we simply set our formData with that key value pair.
Pop a console.log(formData) underneath your handleChange function and watch how the user builds out our new object as they type.
At this point we have our new object built and ready to go as soon as our user hits submit, but that isn’t worth much unless we actually do something when the form is submitted.
Ultimately we would like to add the user’s new object to our existing list of objects for sale. To do this, we need to trigger an event when the user clicks the “List Item” button. onSubmit seems like a safe bet here.
Our handleSubmit function should take care of adding our new object to the array (ok, technically a copy of that array) we are already displaying on the page. Likely, this array will defined in a separate component, one higher up in your control flow, so that it can be passed around your app to be displayed, filtered, etc. In order to give our “higher” component (let’s call it App) access to our handleSubmit function, we can use a callback function. We will define our callback function in App, which will look something like this:
Our FormExample component will accept the function as props and use it in our onSubmit handler, taking our formData as a parameter. Now we have built our object and given it a pathway to be displayed onto our app. While our form is admittedly slightly longer due to our new attributes, we are now achieving our goal without repetitive logic in a nice clean package that we can continue to use without adding more logic if we decide we need more information from our user.
Let’s admire our work.
Consolidating Controlled Components in React was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Liz Catoe
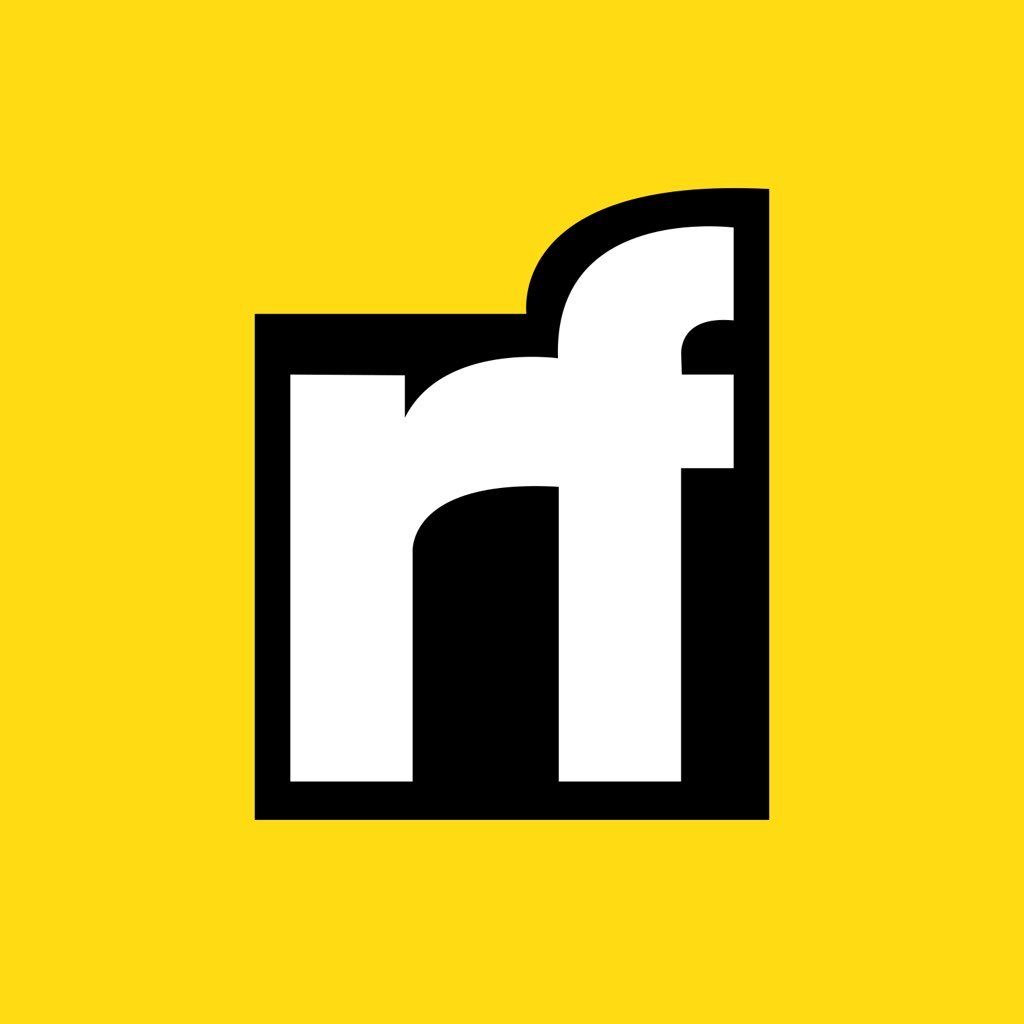
Liz Catoe | Sciencx (2022-03-13T01:58:26+00:00) Consolidating Controlled Components in React. Retrieved from https://www.scien.cx/2022/03/13/consolidating-controlled-components-in-react/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.