This content originally appeared on DEV Community and was authored by JoelBonetR
What is a struct?
A struct is used for building data structures. These structures are used to group related data together.
There are differences on how they work behind the scenes depending on the programming language but the fun thing is that there are no structs in JavaScript.
When you want to create a custom data structure in JS you usually do a single thing. Creating an Object.
const user = {
id: 0,
name: '',
country: '',
pets: [],
}
But is there any other way to do that?
Let's try to create a function to dynamically generate "structs" in JavaScript!
/**
* @constructor Generates a constructor for a given data structure
* @param {string} keys separated by a comma + whitespace. struct('id, name, age')
* @returns {constructor} Constructor for the new struct
*/
function struct(keys) {
const k = keys.split(', ');
const count = k.length;
/** @constructor */
function constructor() {
for (let i = 0; i < count; i++) {
this[k[i]] = arguments[i];
}
}
return constructor;
}
Usage:
const user = struct("id, name, country");
const johnny = new user(1, 'John', 'UK');
console.log(johnny.name); // john
We can also assign complex structures at our properties!
const user = struct("id, name, country, pets");
const pepe = new user(1, 'Pepe', 'ES', ['Baxter', 'Flurfrils']);
console.log(pepe.pets); // ['Baxter', 'Flurfrils']
Or adding a struct inside the other:
/**
* @typedef UserInfo
* @property {string} phone
* @property {number} age
* @property {string} hairColor
* @constructor
*/
/** @type {ObjectConstructor|any} */
const UserInfo = new struct('phone, age, hairColor');
/** @type {UserInfo} */
const extraInfo = new UserInfo('555-777-888', 31, 'blonde');
/**
* @typedef User
* @property {string} phone
* @property {number} age
* @property {string} hairColor
* @constructor
*/
/** @type {ObjectConstructor|any} */
const User = new struct('id, name, country, info');
/** @type {User} */
const John = new User(1, 'John', 'US', extraInfo);
Now we can do:
John.country // 'US'
John.info.phone // '555-777-888'
Okay okay it's creating an object at the end but JavaScript is an object-based language just like Python and others, so in truth everything is an object.
And you can't say that this isn't funny!
Disclaimer: This code was meant to instruct on how you can play with Objects in JavaScript and may not be suitable for a production build. Not because it won't work but because you can make code that is hard to maintain. Please, don't forget to create typedefs (either you use TS or JSDoc with TS Pragma) and always remember to document your code properly.
This content originally appeared on DEV Community and was authored by JoelBonetR
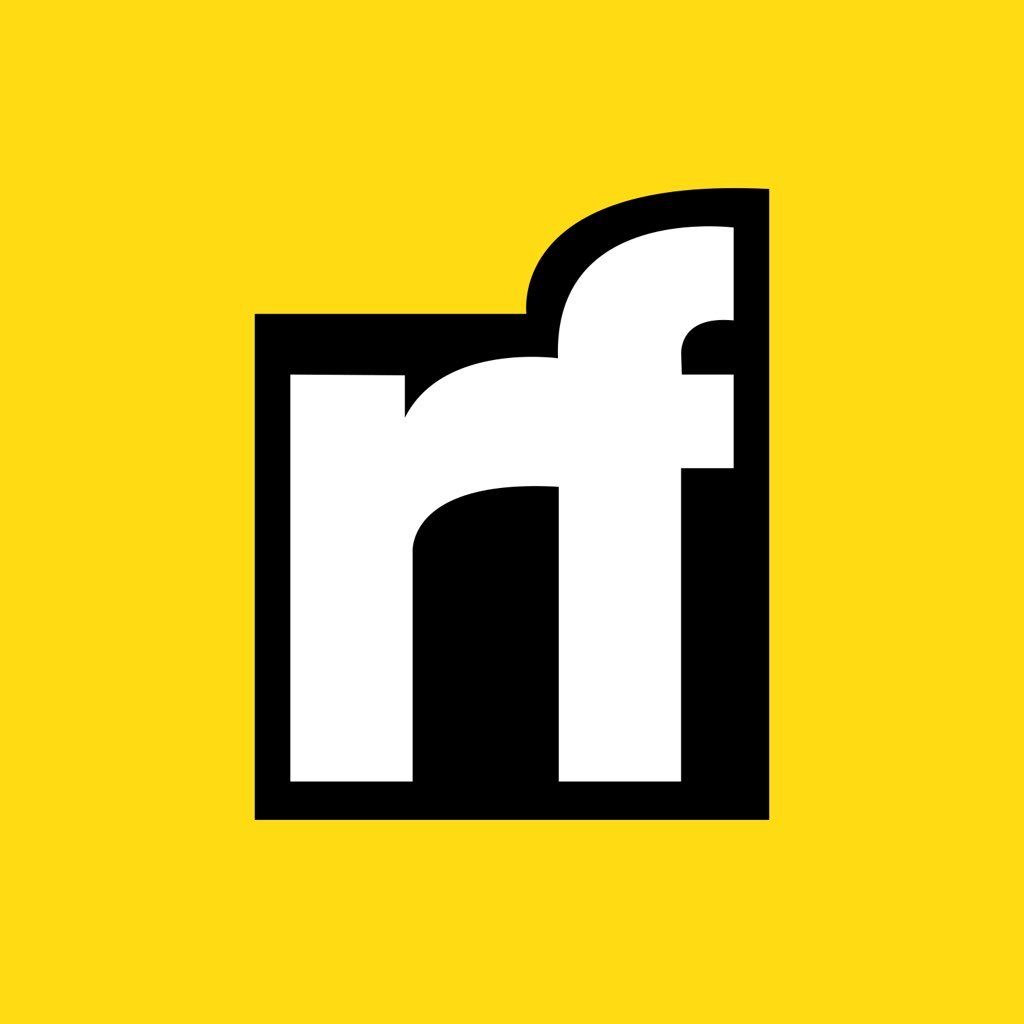
JoelBonetR | Sciencx (2022-05-07T23:59:19+00:00) Structs in JavaScript. Retrieved from https://www.scien.cx/2022/05/07/structs-in-javascript/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.