This content originally appeared on DEV Community and was authored by Rakshit
It was during the initial days when I was learning Javascript, I always used to be struck at a point when Objects are involved which is iterating over them. It also used to be so confused whenever I encountered a problem where I had to create a hashmap
using an empty Object.
This article will give you a clear picture about how you can and how you cannot iterate over Objects in Javascript.
You cannot iterate over Javascript objects using the regular methods like map()
, forEach()
or for..of
loop.
Let there be an object:
const object = {
name: 'Rakshit',
age: 23,
gender: 'Male'
}
Using map()
object.map(obj => {})
You will get an error saying TypeError: object.map is not a function
Using forEach()
object.forEach(obj => {})
You will again arrive at an error saying TypeError: object.forEach is not a function
Using for..of
for (const obj of objects) {}
This will give you TypeError: object not iterable
So, how exactly can you iterate an object in Javascript?
Here are some of the ways you can do that:
One of the most simplest way is to use for..in
loop
for(const obj in object) {}
But, personally I love to iterate over an object using the Object.entries()
method. This method generates an array of all the enumerable properties of the object passed into it as an argument.
Since, it returns an array you can use any of the methods you use to iterate over an array.
Using map()
Object.entries(object).map(obj => console.log(obj))
Using forEach()
Object.entries(object).forEach(obj => console.log(obj))
Using for..of
for (const obj of Object.entries(object)) {
console.log(obj)
}
That's it! Eureka! With this article I am sure you will never forget how to iterate over Objects in Javascript.
I hope the article helps.
Reach me out on Github and LinkedIn.
Follow me on Twitter
Have a nice day :)
This content originally appeared on DEV Community and was authored by Rakshit
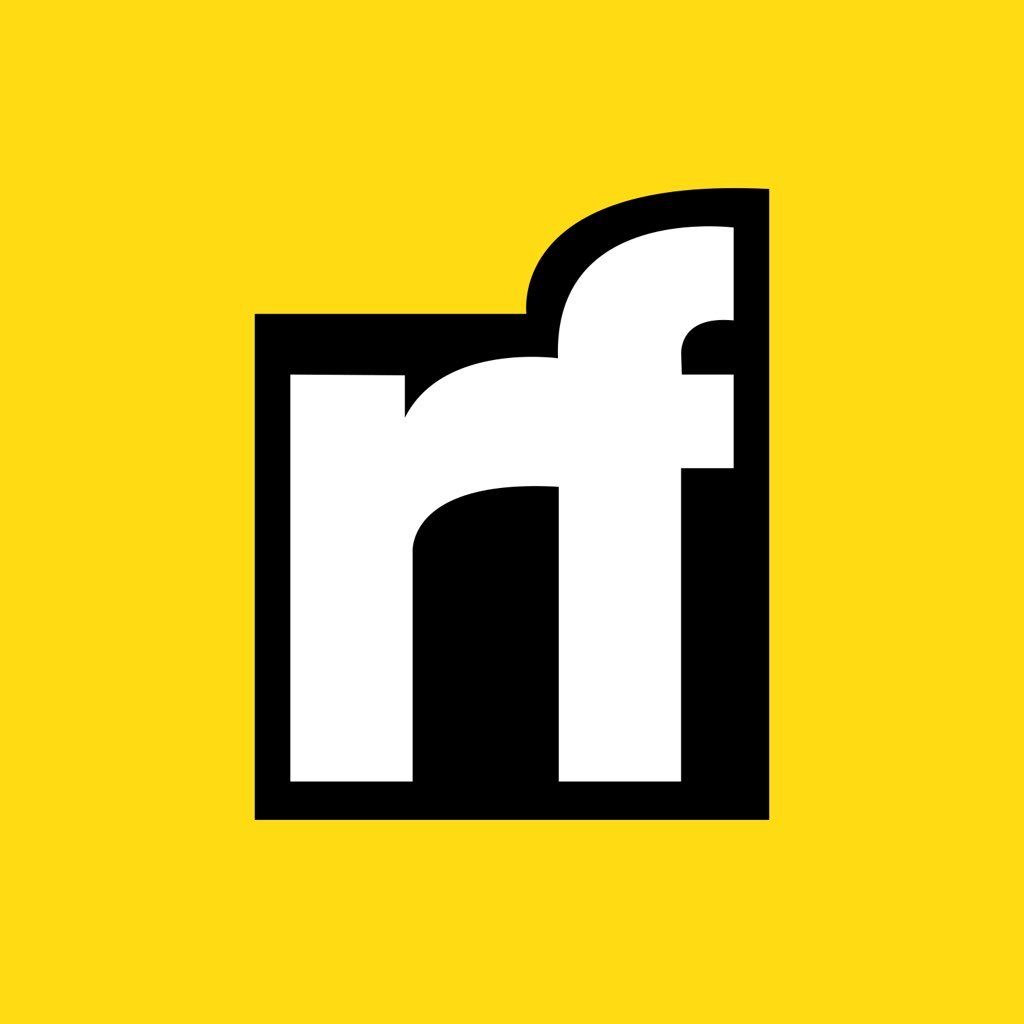
Rakshit | Sciencx (2022-07-02T21:49:56+00:00) How to iterate over an object in Javascript?. Retrieved from https://www.scien.cx/2022/07/02/how-to-iterate-over-an-object-in-javascript/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.