This content originally appeared on Level Up Coding - Medium and was authored by Emmanuel Tejeda
A thorough breakdown of data types and variables for people proficient in Java.
In this guide we're going to break down data types and variables to their bones and show you how they work. A large portion of this guide will contain programming and mathematical jargon, if you are not familiar with programming or just started, I would not recommend this guide to you. If you are a student or studying for an interview, this will be useful information to become familiar with.
Fields vs. Local variables
Fields
- Fields are declared at the class level, as opposed to the method or block level.
- They can be an instance variable, meaning that every instance of the class has a unique instance of this variable. Or they can be a class/static variable, meaning there is only on instance in memory for this variable that any instance of the class can access and change. Static variables can be invoked with the class name rather than creating an instance. For example className.variablename
If you want more information on static vs instance, check out this Oracle tutorial - Fields will automatically be initialized with a default value. Here are the default values for all the primitive data types. String is the only outlier since it is an object (Primitive data types are classified as such because they only contain raw values).
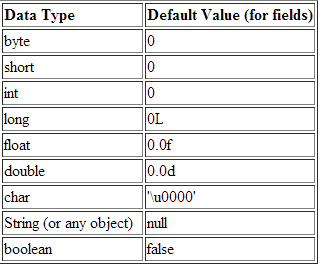
Local variables
- Local variables are declared in code blocks or methods
- They have to be assigned a value/initialized
- They can never be static
- If not defined, will cause an undefined error in the console
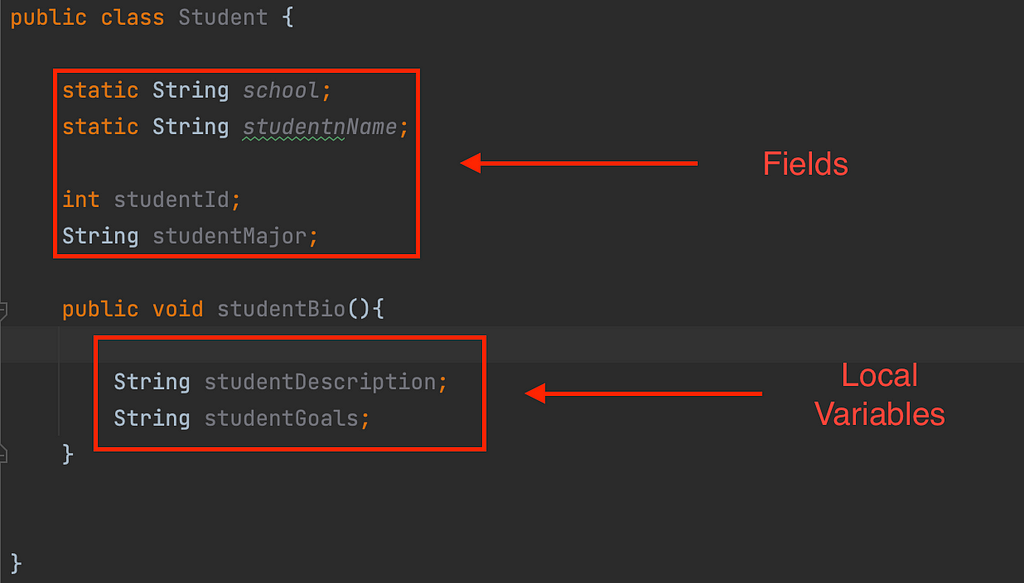
The difference between Primitive types and Object references
Primitive data types
- Store raw values.
- Can’t store null
Object References
- Stores a pointer to its value
- Can store null
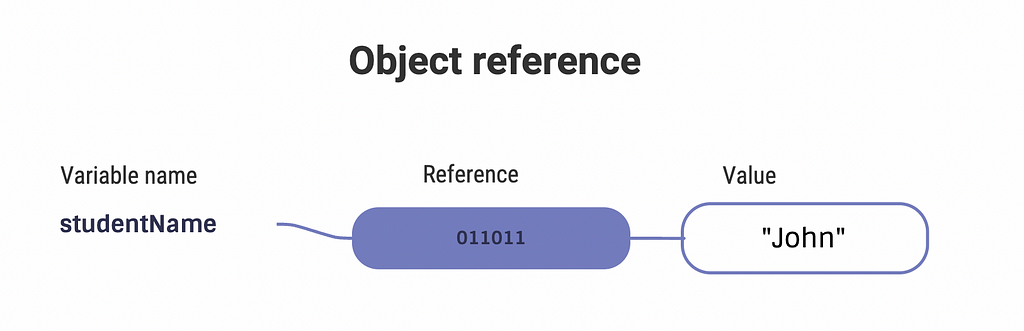
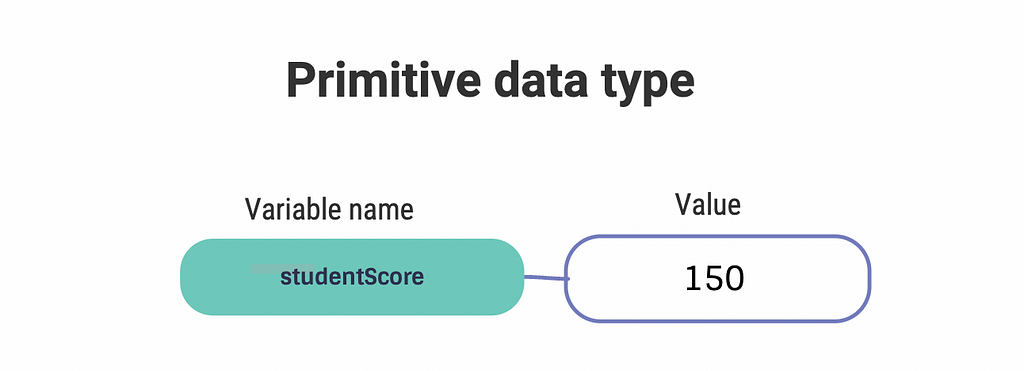
Primitive Integral types
Integral types are values that cannot be expressed in decimal format and can hold a certain range of values depending on which one is used. They are all initialized by the value 0. The list of Integral types in ascending order by size is as follows.
- byte. A byte is a sequence of 8 bits. A byte can hold numerical values from -128 to 127. It is best used when the numbers that will be stored do not require much memory.
- short. A short is twice the size of a byte at 16 bits. It can store values from -32,768 to 32,767.
- int. An int is probably what most people who program are familiar with. An int is a sequence of 32 bits and can hold values from -2.1 billion to 2.1 billion. An int in most situations is not the ideal data type to use because of how much memory it takes. Most people assigning values to an int will almost never get close to its upper or lower limit.
- long. A long is the largest of the integral types. At 64 bits it can hold values between -9.2 Con trillion and 9.2 Con trillion. Longs are most often used to measure time in milliseconds. When you have a number that an int can’t handle, a long is usually your go-to. When writing a long value, you need to add the letter “L” at the end to signify it is a long. The L can be either upper case or lower case. Example long bigNum = 9,233,186,123L.
Float and Double Primitives
There are not many differences between a float and a double. They both behave very similarly and share a lot of features. So, for this section, we are going to list what they share, then afterward list their individual properties.
Both floats and doubles
- Can store integral types such as long, int, short, etc.
- Represent data in a decimal format.
- Have a wider range of values than all the integrals.
- They both round the number after the decimal point if the value outputted to the console has too many digits.
- If the value outputted to the console is too large it will be represented in scientific notation.
Now for the differences
Float
- Is a sequence of 32 bits.
- Must always include the letter “F” either lower or uppercase at the end of the value. Otherwise, the system will recognize it as a double.
For example, float aFloat = 21.22f . If you do not add trailing “f” you will get an error.
Double
- Is a sequence of 64 bits
The char Primitive
Most people familiar with the data type char, know that it can hold one character as we see here char letter = 'B', but that is just the surface of how the char primitive works.
- The char is actually a 16-bit integral type that can hold a value from 0 to 65,535.
- A char like all integral types cannot be assigned a decimal value
- A char can also be represented as a Unicode literal. For example 'A' and the Unicode '\u0041' are the same and will both output the letter “A” to the console when run. There are many characters in different languages that are represented in Unicode. You can explore the wiki on Unicode if you are interested.
- Under the hood, a character is represented as an integral number. For example, if you were to assign a char a number like this and print it to the consolechar letterA = 65 you would get the letter ‘A’. This means that these three values 'A' = '\u0041' = 65 are all the same when assigned to a char.
- A char is initialized by the value 0 represented in Unicode as '\u0000' .
The use of a number when using char is very obscure and not something you will see every day. But it is very useful to know that it is a possibility.
Hope you guys found this informative. If you have any questions or comments, leave them and I will get to them as soon as I can.
Have a great day!
Resources
List of Unicode's and their values
Oracle tutorial. class/static vs. instance variable
Level Up Coding
Thanks for being a part of our community! More content in the Level Up Coding publication.
Follow: Twitter, LinkedIn, Newsletter
Level Up is transforming tech recruiting 👉 Join our talent collective
Advanced Java Tutorial. Primitive Types and Variables. was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Emmanuel Tejeda
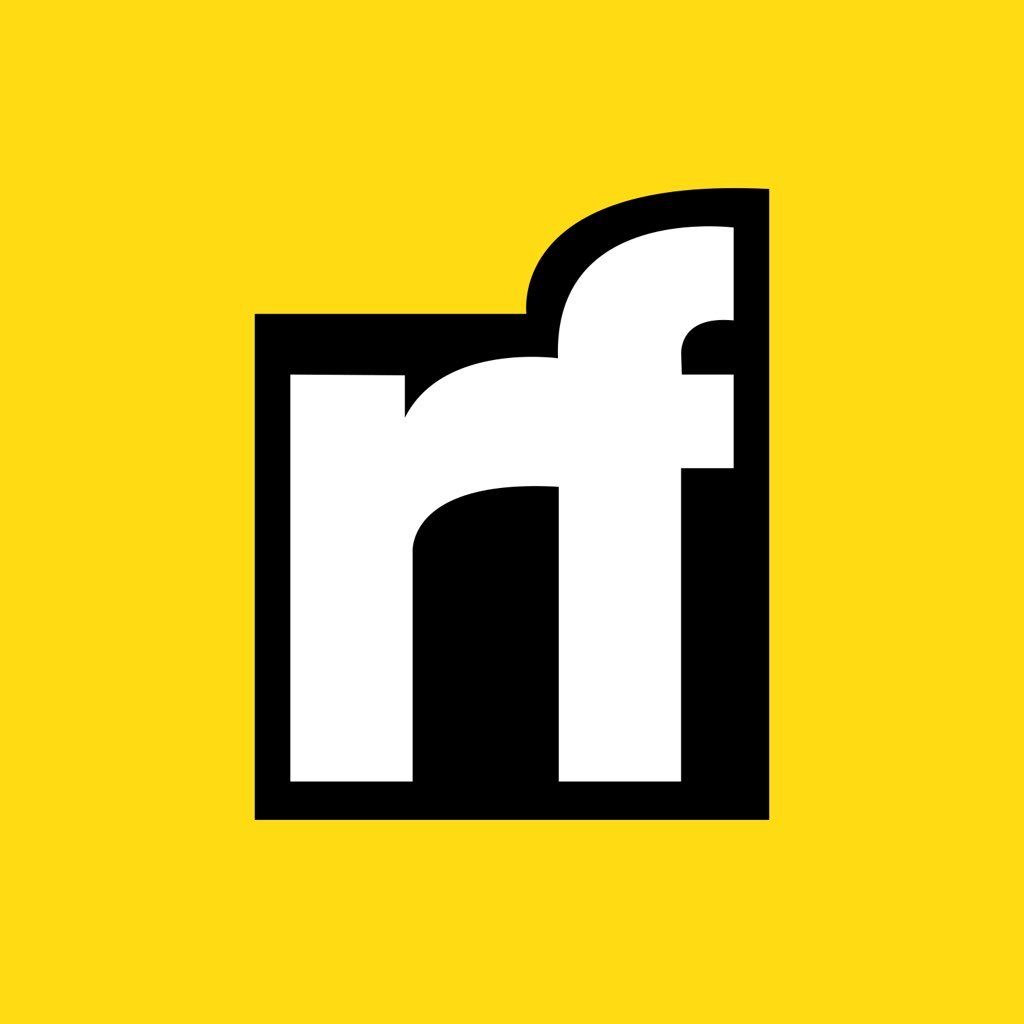
Emmanuel Tejeda | Sciencx (2022-07-16T01:01:16+00:00) Advanced Java Tutorial. Primitive Types and Variables.. Retrieved from https://www.scien.cx/2022/07/16/advanced-java-tutorial-primitive-types-and-variables/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.