This content originally appeared on Envato Tuts+ Tutorials and was authored by Jemima Abu
In this tutorial, we’ll be implementing a timer to countdown to a specific date or time using vanilla JavaScript. We’ll also implement a feature to display content on a webpage once the countdown is done.
A countdown timer on a webpage is usually used to indicate the end or beginning of an event. It can be used to let customers know when a price offer expires, or feature on a “coming soon” landing page to indicate when the site will be published. It can also be used to let a user know if an action needs to be carried out soon; for example, some websites will log users out of a page if there has been no activity within a certain period of time.
So, back to what we’re making—here’s a look at the final product. Press Rerun on the demo to start the countdown function:
Let’s get started!
1. Creating the Markup
We’ll be using two sections for this demo: a timer section and a content section. The timer section will contain the elements for displaying the time left in the countdown and the content section will be the element shown after the countdown is up.
<section id="timer"> <p>This content will be displayed in</p> <div class="timer-container"> <span id="days">0 days</span> <span id="hours">0 hours</span> <span id="minutes">0 minutes</span> and <span id="seconds">0 seconds</span> </div> </section> <section id="content"> <h1>Hello, World</h1> </section>
Considering Accessibility
Since we’re building a section that has constantly changing information, we should consider how this information will be presented to assistive technologies. In this demo, we’ll use the timer
role to represent the elements that have regularly updated text.
<section id="timer"> <p>This content will be displayed in</p> <div class="timer-container"> <span id="days" role="timer">0 days</span> <span id="hours" role="timer">0 hours</span> <span id="minutes" role="timer">0 minutes</span> and <span id="seconds" role="timer">0 seconds</span> </div> </section> <section id="content"> <h1>Hello, World</h1> </section>
2. Styling the Sections
The timer container has the most priority when the page is first loaded so we’ll make it a fixed container that’s the full width and height of the page so no other content is visible till the countdown is up. We’ll also use an opacity styling on our content to hide it.
#timer { position: fixed; top: 0; bottom: 0; display: flex; flex-direction: column; min-height: 100vh; justify-content: center; align-items: center; width: 100%; z-index: 2; } #content { opacity: 0; }
3. Building the Countdown Timer
This is the logic we’ll use to handle the countdown timer:
- Define a countdown value based on a specific date or time
- Get the current date and subtract the value from our countdown
- Carry out the subtraction function at an interval of 1s
- If the countdown date is less than or equal to the current date, end the countdown
Let’s start with defining our countdown value. There are two possible methods we can use:
1. Defining the Countdown Value as a Specific Date and Time
We can initialise a countdown value as a specific date using the Date()
constructor. This method is useful for displaying special offers or discounts as the countdown end time will always be constant. For example, you can use a countdown timer to display a discount offer until the New Year.
let countdownDate = new Date('01 January 2023 00:00')
2. Defining the Countdown Value as an Added Unit of Time to the Current Date
We can also initialise a countdown timer by adding time to the current date. This method is useful for handling user-based timer interactions. We use the Date get and set methods for this. For example, you can set a timer to display content 30 seconds after a user has landed on a webpage.
let countdownDate = new Date().setSeconds(new Date().getSeconds() + 30);
We can also modify this function for minutes or hours:
let countdownDate = new Date().setMinutes(new Date().getMinutes() + 5);
let countdownDate = new Date().setHours(new Date().getHours() + 1)
Once we’ve setup our countdown value, we can define our constants:
const daysElem = document.getElementById("days"), hoursElem = document.getElementById("hours"), minutesElem = document.getElementById("minutes"), secondsElem = document.getElementById("seconds"), timer = document.getElementById("timer"), content = document.getElementById("content");
Then we can work on our countdown function.
4. Creating the startCountdown() Function
We’ll create a new function called startCountdown()
where we get the current date and subtract it from the countdown date. We’ll use the Date.getTime()
method to convert both values into milliseconds then we divide the difference by 1,000 to convert to seconds.
const startCountdown = () => { const now = new Date().getTime(); const countdown = new Date(countdownDate).getTime(); const difference = (countdown - now) / 1000; }
We’ll need to convert the difference value to days, hours, minutes and seconds to determine our timer value.
We can convert seconds to days by dividing the difference in seconds by the value of one day (60seconds * 60minutes * 24hours) and rounding up to the nearest value.
let days = Math.floor(difference / (60 * 60 * 24));
Since our countdown is an additive value, we’ll need to take into consideration the preceding values when calculating. When converting to hours, we’ll first need to know how many days are in the difference and then convert the remainder to hours.
For example, if we have 90,000 seconds, this will be converted to 25 hours. However, we can’t display a countdown as 1 day and 25 hours as that will be an incorrect time. Instead we’ll first calculate how many days are in the seconds as 90000 / (60 * 60 * 24) = 1 day with a remainder of 3600 seconds. Then we can convert this remainder to hours by dividing by (60seconds * 60minutes) which gives 1 hour.
let hours = Math.floor((difference % (60 * 60 * 24)) / (60 * 60));
Applying the same cumulative division for minutes and seconds:
let minutes = Math.floor((difference % (60 * 60)) / 60); let seconds = Math.floor(difference % 60);
Then we’ll pass our calculated values into the HTML elements. Taking grammar into consideration, we can define a function to format the unit of time text as singular or plural based on the value of time.
const formatTime = (time, string) => { return time == 1 ? `${time} ${string}` : `${time} ${string}s`; };
Our startCountdown()
function now looks like this:
const startCountdown = () => { const now = new Date().getTime(); const countdown = new Date(countdownDate).getTime(); const difference = (countdown - now) / 1000; let days = Math.floor(difference / (60 * 60 * 24)); let hours = Math.floor((difference % (60 * 60 * 24)) / (60 * 60)); let minutes = Math.floor((difference % (60 * 60)) / 60); let seconds = Math.floor(difference % 60); daysElem.innerHTML = formatTime(days, "day"); hoursElem.innerHTML = formatTime(hours, "hour"); minutesElem.innerHTML = formatTime(minutes, "minute"); secondsElem.innerHTML = formatTime(seconds, "second"); };
5. Running our Function at Intervals
Now we have our countdown function, we’ll create a function that runs the countdown function at an interval of 1s.
First, we’ll create our timerInterval value.
let timerInterval
Then we define the timerInterval as a setInterval function when the page loads
window.addEventListener("load", () => { startCountdown(); timerInterval = setInterval(startCountdown, 1000); });
In this method, we’ve called the startCountdown()
function immediately as the page loads to update the countdown values and then we call the countdown function every 1s after the page loads.
We’ll need to also define a function to handle when the countdown timer ends. We’ll know the timer has ended when the difference in our startCountdown
function is less than 1 second.
const startCountdown = () => { const now = new Date().getTime(); const countdown = new Date(countdownDate).getTime(); const difference = (countdown - now) / 1000; if (difference < 1) { endCountdown(); } ... };
This is what we have so far!
6. When the Countdown Timer Ends
What we currently have keeps counting down indefinitely, which clearly isn’t very useful, so let’s remedy that.
In our endCountdown()
function, we’ll stop the interval function, delete the timer and display the content section.
const endCountdown = () => { clearInterval(timerInterval); timer.remove(); content.classList.add("visible"); };
We’ll target the visible class in CSS to handle displaying the content. In this demo, we have a text scale and background colour change animation when the content is displayed that’s also handled with CSS. Of course, this is purely for demonstrative purposes—you can style things however you want.
#content h1 { font-size: 10vmax; transform: scale(1.25); } #content.visible { opacity: 1; animation: colorChange 1s ease-in-out 0.5s forwards; } #content.visible h1 { animation: scaleOut 1s ease-in-out 0.5s forwards; } @keyframes colorChange { from { color: #fcdf00; background-color: #0d67ad; } to { color: white; background-color: black; } } @keyframes scaleOut { from { transform: scale(1.25); } to { transform: scale(1); } }
Conclusion
And, with that, we’ve successfully created a countdown timer to display content on a webpage!
This content originally appeared on Envato Tuts+ Tutorials and was authored by Jemima Abu
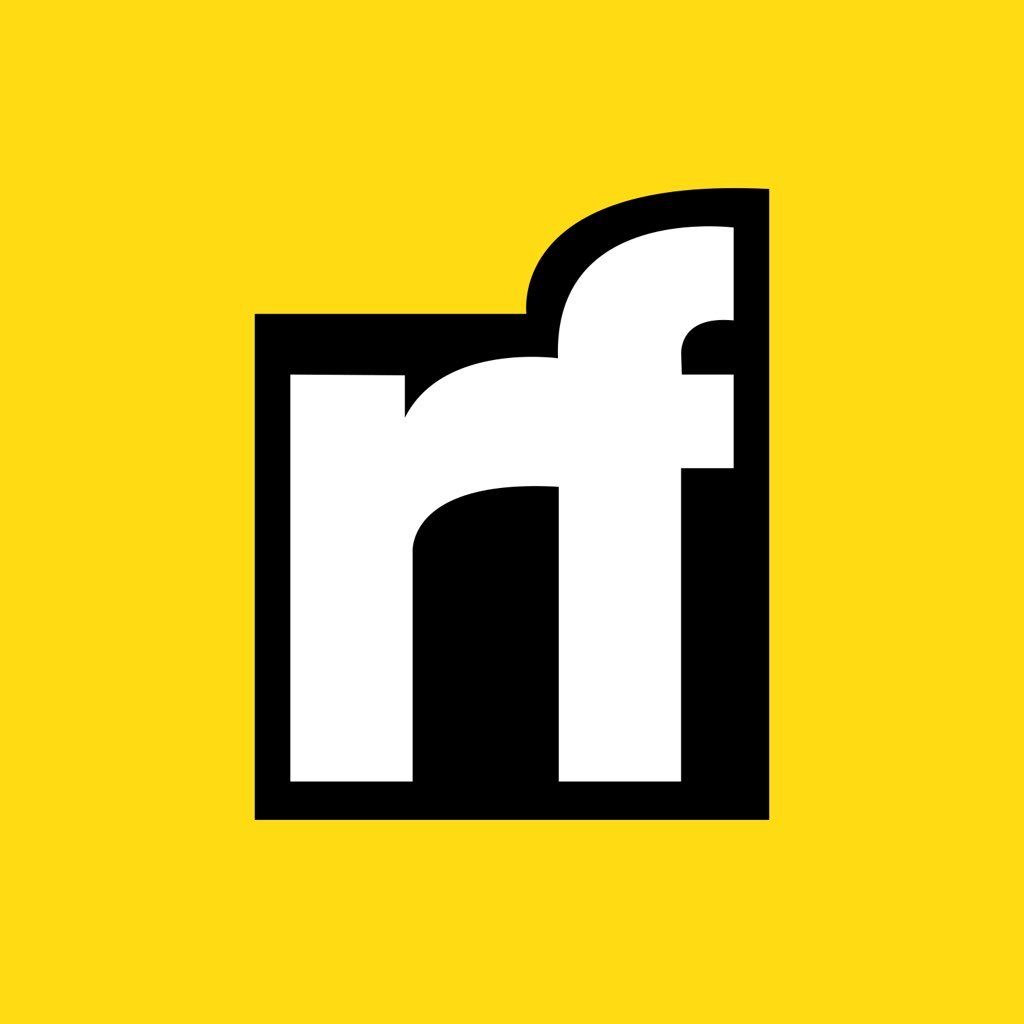
Jemima Abu | Sciencx (2022-08-29T07:59:09+00:00) How to Build A JavaScript Countdown Timer. Retrieved from https://www.scien.cx/2022/08/29/how-to-build-a-javascript-countdown-timer/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.