This content originally appeared on Level Up Coding - Medium and was authored by bitbug
Preface
In our daily development, it is very common to take screenshots and paste pictures into IM or rich text editors. Are you curious about how to copy and paste images via JavaScript.
In this article, we will look at how to accomplish these features with plain JavaScript. There may one demo and the final effect looks like below:
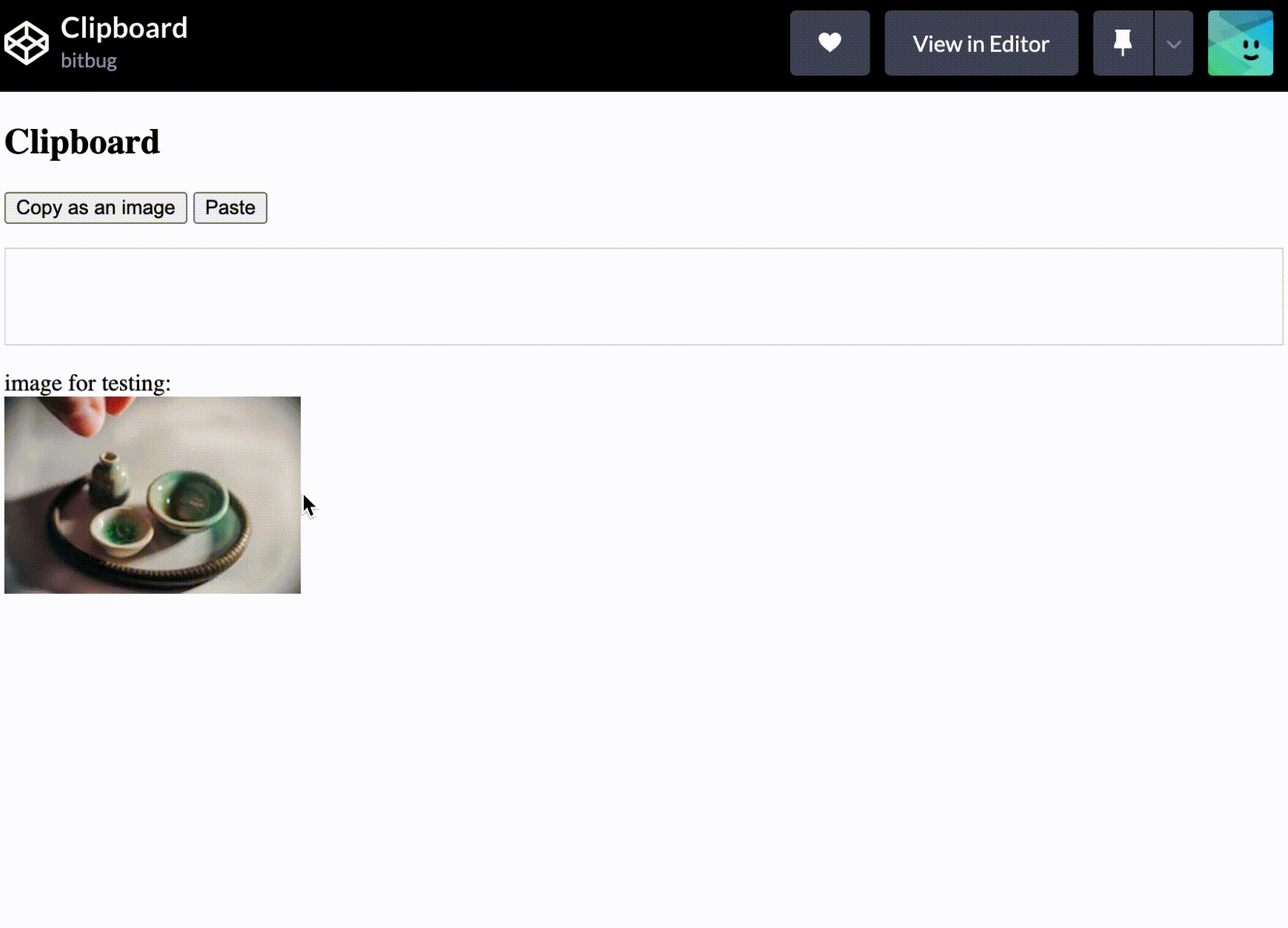
Clipboard API
When we want to get data or write data to clipboard, we can use the Clipboard API — Web APIs | MDN (mozilla.org), that provides the ability to access to clipboard. And we can access the system clipboard through the global navigator.clipboard instead of creating a Clipboard object instance:
navigator.clipboard
.readText()
.then((clipText) => {
console.log('clipboard text:', clipText);
});
Tips: copy this code into Devtool console may occur an error DOMException: Document is not focused, the solution is to wrap this code by setTimeout and then click the page to make it focused, like below way:
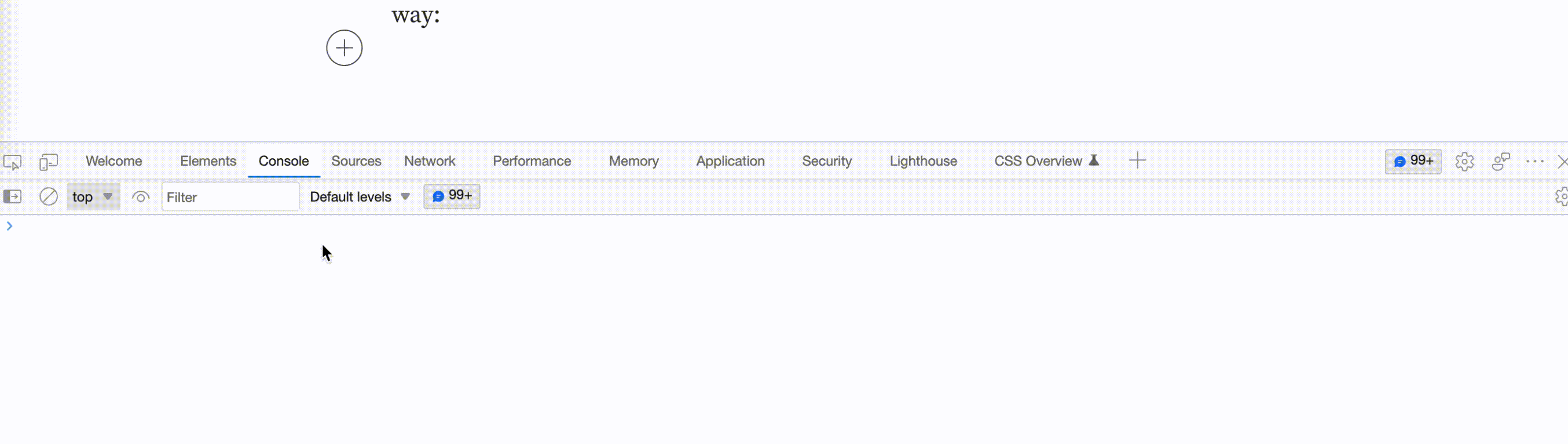
The Clipboard API includes four methods, all of methods will return a Promise.
- read(): requests arbitrary data from the system clipboard.
- readText(): requests text from the system clipboard, and returns a Promise which is resolved with a text string.
- write(): writes arbitrary data to the system clipboard.
- writeText(): writes text data to the system clipboard.
When we need to handle image data, we can use the read and write method.
Paste Image
Paste image means that we have to consume data from system clipboard, in this case, we can use read method, which will return a Promise that resolves with an array of ClipboardItem objects. The ClipboardItem has a types property which can be used to determine the type of data and also defines getType method which returns a Promise that resolves with a Blob of requested MIME type. We want to request the image data from system clipboard as the below code:
async function parseClipboardData() {
const items = await navigator.clipboard.read().catch((err) => {
console.error(err);
});
for (let item of items) {
for (let type of item.types) {
if (type.startsWith("image/")) {
clipboardItem.getType(type).then((imageBlob) => {
const image = `<img src="${window.URL.createObjectURL(
imageBlob
)}" />`;
$container.innerHTML = image;
});
return true;
}
}
}
}
Every clipboardItem may have several type, such as when we copy an image in chrome by right click some picture on web page, the types will be like [‘text/html’, ‘image/png’] .
And you you can test it on Codepen.io that also support paste text data:
Copy Image
When we want to copy html tag as image, suggest to use html-to-image that is promise-based and supports convert html to image, svg and blob data.
By using this npm module, it is very easy to copy html tag as an image:
const $body = document.querySelector("body");
$body.addEventListener("click", () => {
htmlToImage
.toBlob($body)
.then(function (blob) {
return navigator.clipboard.write([
new ClipboardItem({
[blob.type]: blob
})
]);
})
.then(
() => {
console.log("copy success");
},
(err) => {
console.error(err);
}
);
});
And you can test it on Codepen.io:
Conclusion
In this article, we learn about how to use navigator.clipboard to accomplish paste and copy image. By using navigator.clipboard.read() method, we can get arbitrary data from system clipboard. And we can write arbitrary data to system clipboard via navigator.clipboard.write() method.
Hope this article can help you, if you want to known more clipboard tips, you may refer this article:
Javascript How to Copy Text to Clipboard
How to Copy or Paste Image by Plain Javascript was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by bitbug
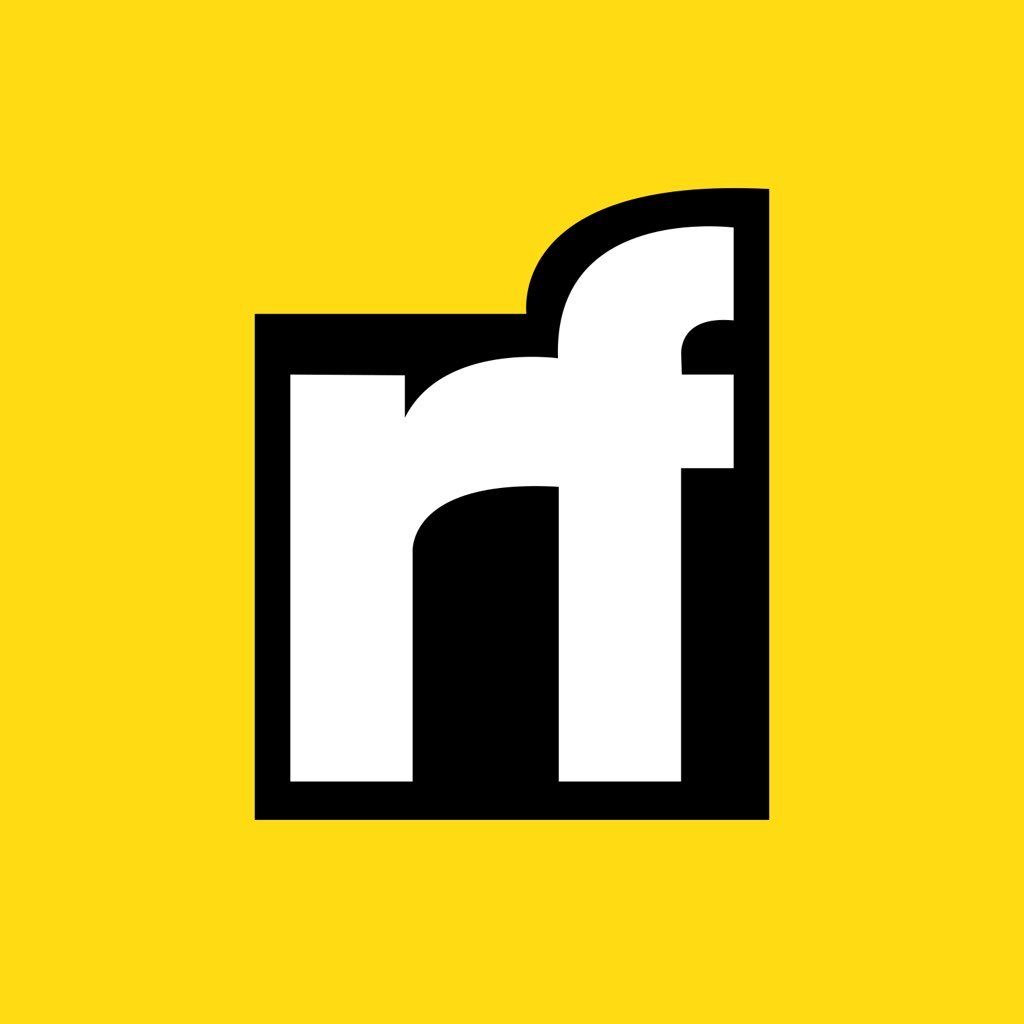
bitbug | Sciencx (2022-10-14T12:24:05+00:00) How to Copy or Paste Image by Plain Javascript. Retrieved from https://www.scien.cx/2022/10/14/how-to-copy-or-paste-image-by-plain-javascript/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.