This content originally appeared on Bits and Pieces - Medium and was authored by Andrii Drozdov
How Enums Actually Work in TypeScript
A deep dive into the world of Enums — what happens to them after compilation and how they work.
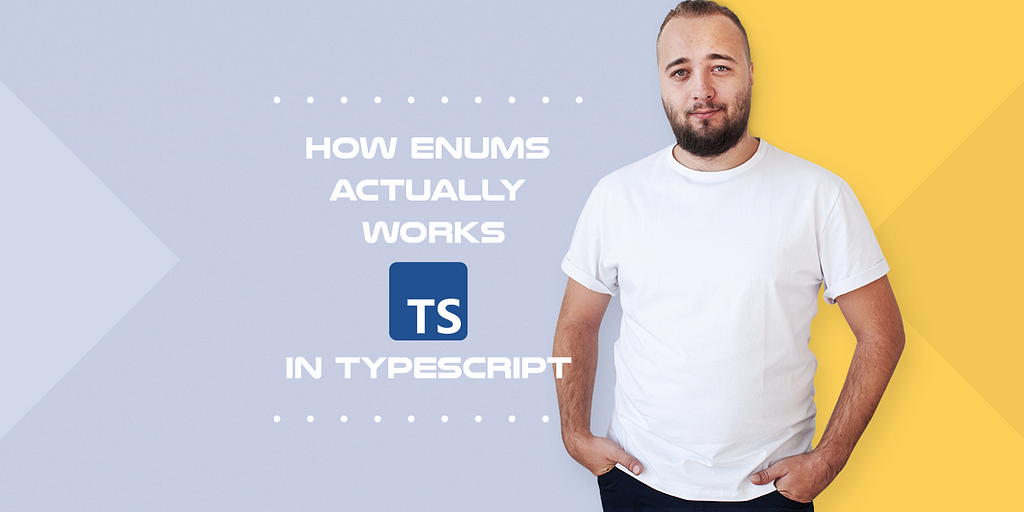
Hello, my favourite bit lovers.
Did you know, that Enum is a function after compilation to JavaScript!?
At some point, you need to ask yourself how things work under the hood.
Today we will dive deeply into the magic of what happens to our Enum after compilation and how it works.
Definition
We will start with an academic definition of Enum.
Enum — is a set of defined key/value pairs. This approach allows developers to reference a single source of truth when using pre-defined values.
TypeScript allows only to use Number or String as values in enums, it is not allowed to use other data types.
How to define it (models/Roles.ts):
How to use it in Interface (models/User.ts):
How to use Enum values (example/Usage.ts):
Nah, boring!
So, how it actually works?
To answer this question we need to take a look at what Enum is compiled to.
So, let's compile the example above and see what we will receive. We will compile to ES2022.
I will ignore models/User.ts because it will be empty since interfaces are not compiled into JavaScript.
Roles.ts -> Roles.js
This is our former models/Roles.ts file, which is compiled into models/Roles.js file:
You see that our Enum compiled into function. Let's walk through the file.
Line 2
Object.defineProperty(exports, “__esModule”, { value: true }); — this helps correctly export files to CommonJS/AMD/UMD module format. It’s not related to our Enum.
Line 3
exports.Roles = void 0; — a fancy way to set undefined
Line 9
This one needs some description. Since the first thing after var Roles; what will be executed is Roles = exports.Roles || (exports.Roles = {}), let us take a look a bit closer at what is happening here.
In JavaScript, if you will try to console.log assignment operation the output will be variable content. For example, console.log(Roles = 1) will result in 1.
In our models/Roles.js file at first run, when our exports.Roles is undefined assignment will return undefined what makes the first part of the expression falsy and then we fall back to the right-hand part of the expression. On the right part, we assign an object to our exports.Roles and pass this object as an argument.
In the second run, our exports.Roles already will be populated, so we will code will assign the Roles variable and pass it as an argument.
Line 6
The code base from line:6 to line:8 follows the same logic that was described above. If we will look closer at this line: Roles[Roles[“Default”] = 0] = “Default”; we will notice that TypeScript define two keys at the same time:
- The first key will be the value of our Enum, in our case 0
- The second key will be the key of Enum, in our case Default
Results
And in the end, when this function will be executed it will populate our variable with two types of keys for each Enum entry. In our example the object will look like this:
{
'0': 'Default',
'1': 'Admin',
'2': 'SuperAdmin',
Default: 0,
Admin: 1,
SuperAdmin: 2
}
Usage.ts -> Usage.js
There is nothing fancy here, it’s pretty clear to understand what is happening here. The only thing that is important for us is that our Enum name is not being randomised and has the name as in our TypeScript files.
Do you find yourself creating the same Enums over and over again? It might be time to consider packaging them up with Bit so that you can use and reuse!
Does TypeScript configuration somehow change this behaviour?
In the example above our tsconfig.json looks like this:
As you see — we are compiling to es2022 and commonjs.
Does the target change anything?
Short answer — NO.
Long answer the only thing that is being changed is the line with export helpers, Object.defineProperty(exports, “__esModule”, { value: true }); to exports.__esModule = true; if we will compile to es3.
Does the module property change anything?
There’s no change related to Enum behaviour, but there are a lot of other changes related to code structure. But this is completely another story…
Conclusion
As an engineer, you have to know how the tools that you are using work and obviously, if you are only learning TypeScript, is totally fine not to know how some aspects of your technology works, but at some point, as you grow, you need to dive deeper into how a thing works under the hood.
That’s it for today. Hope you had a fun day.
Don’t forget to follow me for more!
Your bit. 01101100 01101111 01110110 01100101
Build Apps with reusable components, just like Lego
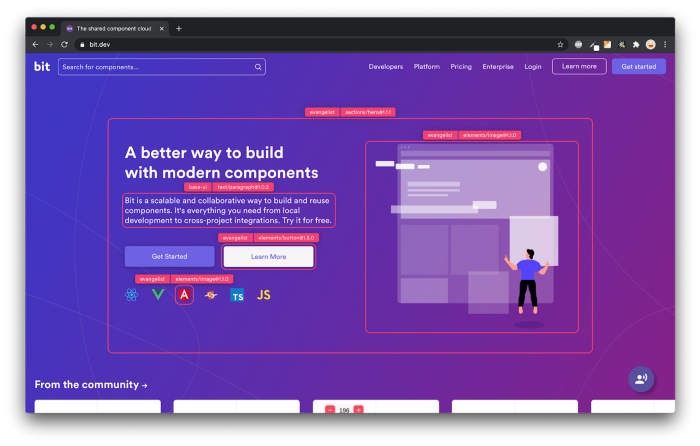
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Learn more
- How We Build Micro Frontends
- How we Build a Component Design System
- How to reuse React components across your projects
- 5 Ways to Build a React Monorepo
- How to Create a Composable React App with Bit
How Enums actually works in TypeScript was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Andrii Drozdov
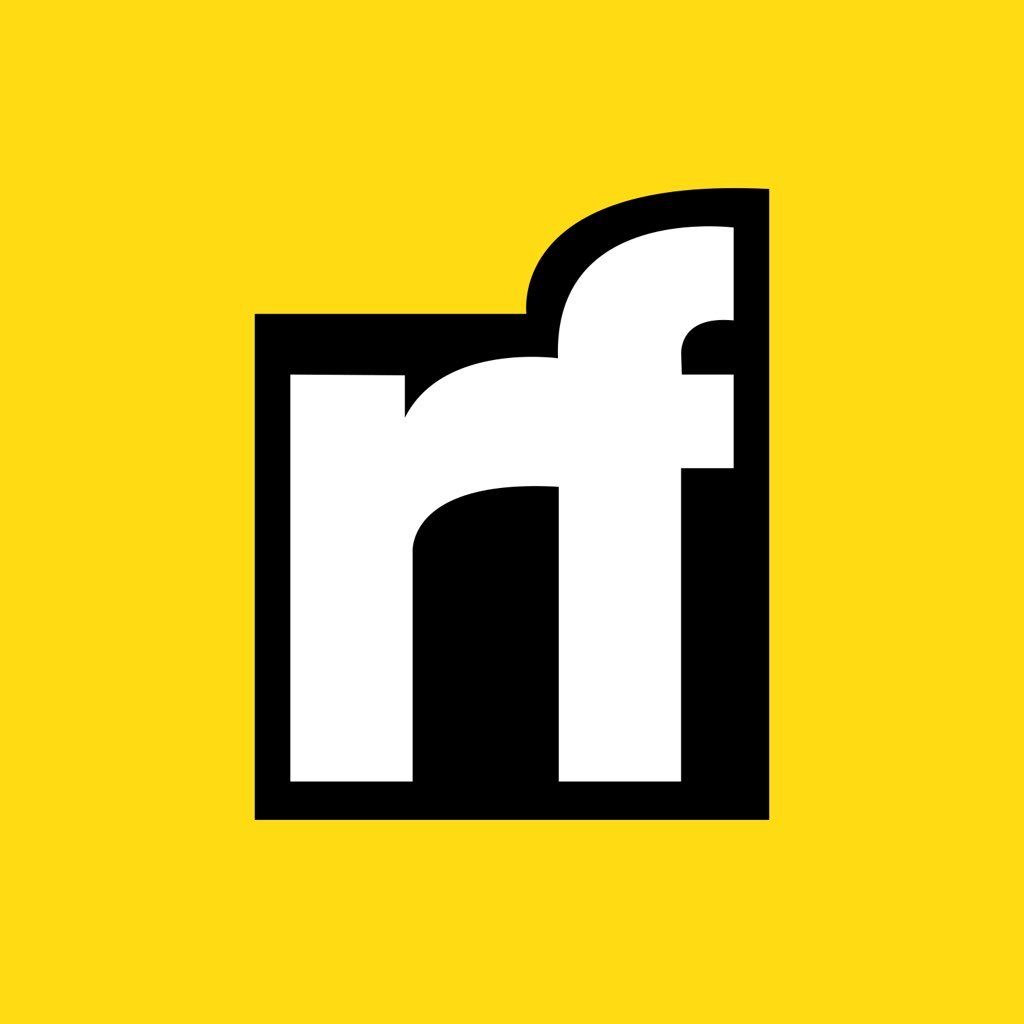
Andrii Drozdov | Sciencx (2023-02-08T17:04:26+00:00) How Enums actually works in TypeScript. Retrieved from https://www.scien.cx/2023/02/08/how-enums-actually-works-in-typescript/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.