This content originally appeared on DEV Community 👩‍💻👨‍💻 and was authored by Bhavik Gevariya
In today's web development world, RESTful APIs are essential in building modern web applications. In this blog post, we will explore how to create a RESTful API using Node.js and Express.
Prerequisites
Before we begin, you should have the following installed on your machine:
Node.js
NPM (Node Package Manager)
Step 1: Create a new Node.js project
First, create a new directory for your project and navigate to it in your terminal. Then, run the following command to create a new Node.js project:
npm init -y
This will create a new package.json file in your project directory.
Step 2: Install required dependencies
Next, we need to install the necessary dependencies for our project. Run the following command to install express, body-parser, and cors:
npm install express body-parser cors
Step 3: Create a server using Express
Now that we have the necessary dependencies installed, we can create a server using Express. Create a new file called index.js in your project directory and add the following code:
const express = require('express');
const bodyParser = require('body-parser');
const cors = require('cors');
const app = express();
app.use(bodyParser.json());
app.use(cors());
const port = 5000;
app.listen(port, () => {
console.log(`Server is running on port: ${port}`);
});
This will create a new Express server with CORS support and JSON body parsing middleware.
Step 4: Add routes to your server
Next, we need to add some routes to our server. Add the following code to your index.js file:
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.post('/api/users', (req, res) => {
const { name, email } = req.body;
res.json({ message: `User ${name} with email ${email} was created.` });
});
This will create two routes - one for a simple "Hello World" message, and another for creating a new user.
Step 5: Test your API
To test your API, run the following command in your terminal:
node index.js
This will start your server on port 5000. You can then use a tool like curl or Postman to make requests to your API.
For example, to create a new user, you can send a POST request to http://localhost:5000/api/users with a JSON body like this:
{
"name": "John Doe",
"email": "john.doe@example.com"
}
This will return a JSON response with a message indicating that the user was created.
Conclusion
In this blog post, we explored how to create a RESTful API using Node.js and Express. We covered the necessary dependencies, creating a server using Express, adding routes to the server, and testing the API. With this knowledge, you can start building your own RESTful APIs and take your web development skills to the next level.
Small support comes a long way!
This content originally appeared on DEV Community 👩‍💻👨‍💻 and was authored by Bhavik Gevariya
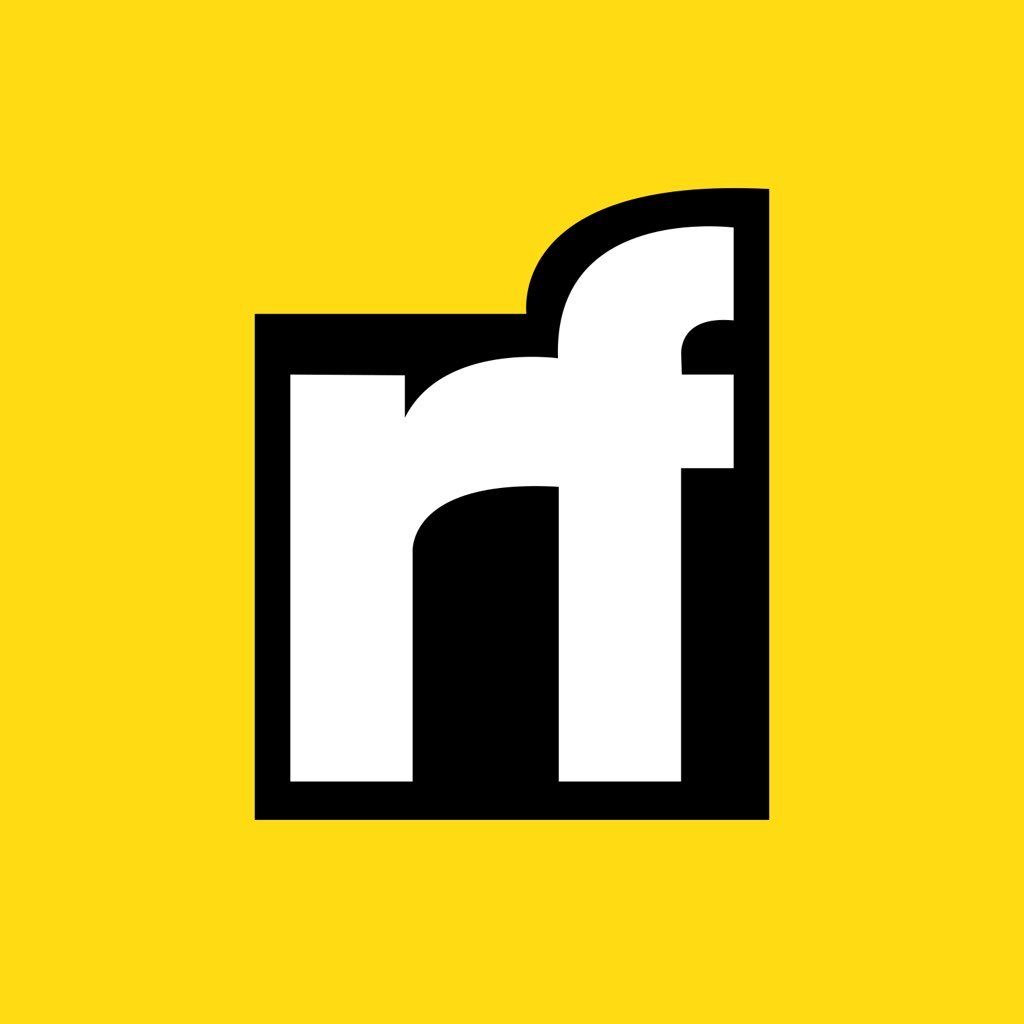
Bhavik Gevariya | Sciencx (2023-02-16T00:38:39+00:00) Building a RESTful API with Node.js and Express: A Step-by-Step Guide. Retrieved from https://www.scien.cx/2023/02/16/building-a-restful-api-with-node-js-and-express-a-step-by-step-guide/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.