This content originally appeared on Bits and Pieces - Medium and was authored by FardaKarimov
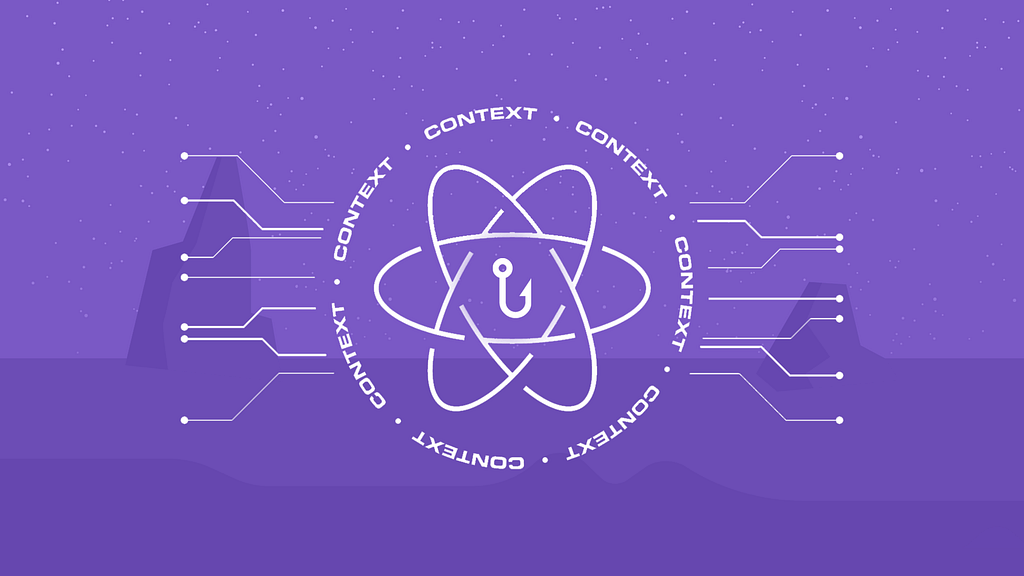
Explore the Context API in React and learn how you can use it in your own applications.
The Context API in React is a feature that allows for sharing data between components without the need for passing props down through multiple levels of the component tree. This can be especially useful when you have data that needs to be shared between many components or when you have deeply nested components.
In this article, we will explore the Context API in React and provide some code examples to demonstrate how you can use it in your own applications.
💡 As an aside, if you want to get the most out of your React components, you could consider using tools such as Bit. With Bit you can pack your React components into packages and use them across multiple projects with a simple npm i @bit/your-username/your-component.
Learn more:
How to reuse React components across your projects
What is the Context API?
The Context API is a way of passing data down through the component tree without the need for props. It allows you to share data between components that are not directly related, such as a parent and a child that are several levels apart.
The Context API is built on two React components: the Provider and the Consumer. The Provider component is used to wrap the components that need to access the shared data, and the Consumer component is used to access that data.
Creating a Context
To create a new Context, you can use the createContext() method from the React library. This method returns a new Context object that can be used to share data across components.
Here is an example of creating a new Context:
import React from 'react';
const MyContext = React.createContext();
This creates a new Context object called MyContext.
Providing Data to the Context
To provide data to the Context, you need to use the Provider component. The Provider component can be used to wrap the components that need to access the shared data.
Here is an example of providing data to the Context:
import React, { useState } from 'react';
const MyContext = React.createContext();
function App() {
const [myData, setMyData] = useState('Hello World');
return (
<MyContext.Provider value={myData}>
<Child />
</MyContext.Provider>
);
}
In this example, the MyContext.Provider component is used to wrap the Child component. The value prop is used to pass data to the Child component. In this case, we are passing the string 'Hello World'.
Accessing Data from the Context
To access data from the Context, you need to use the Consumer component. The Consumer component can be used to access the shared data.
Here is an example of accessing data from the Context:
import React, { useContext } from 'react';
const MyContext = React.createContext();
function Child() {
const myData = useContext(MyContext);
return (
<div>{myData}</div>
);
}
In this example, the useContext hook is used to access the data from the Context. The MyContext object is passed as an argument to the useContext hook. This returns the data that was passed to the MyContext.Provider component in the parent component.
Passing Functions to the Context
In addition to passing data to the Context, you can also pass functions. This can be useful when you want to update the data in the Context.
Here is an example of passing a function to the Context:
import React, { useState } from 'react';
const MyContext = React.createContext();
function App() {
const [myData, setMyData] = useState('Hello World');
function updateData() {
setMyData('Goodbye World');
}
return (
<MyContext.Provider value={{ data: myData, update: updateData }}>
<Child />
</MyContext.Provider>
);
}
In this example, we are passing an object with two properties: data and update. data contains the string 'Hello World' and update is a function that updates the data in the Context to ‘Goodbye World’. This object is passed as the value prop to MyContext.Provider component.
To access the data and function from the Context, we can modify the Child component like this:
import React, { useContext } from "react"
const MyContext = React.createContext()
function Child() {
const { data, update } = useContext(MyContext)
return (
<div>
<div>{data}</div>
<button onClick={update}>Update Data</button>
</div>
)
}
In this example, we are using object destructuring to extract the data and update function from the Context. We are then rendering the data and a button that calls the update function when clicked.
Updating Data in the Context
To update the data in the Context, you need to call the update function that was passed to the Context. You can do this by calling the function directly or passing it down through a prop to a child component.
Here is an example of updating the data in the Context:
import React, { useState } from "react"
const MyContext = React.createContext()
function App() {
const [myData, setMyData] = useState("Hello World")
function updateData() {
setMyData("Goodbye World")
}
return (
<MyContext.Provider value={{ data: myData, update: updateData }}>
<Child updateData={updateData} />
</MyContext.Provider>
)
}
function Child({ updateData }) {
return <button onClick={updateData}>Update Data</button>
}
In this example, we are passing the updateData function down to the Child component through a prop. The Child component renders a button that calls the updateData function when clicked.
Conclusion
The Context API in React is a powerful tool that allows for sharing data between components without the need for passing props down through multiple levels of the component tree. With the Provider and Consumer components, you can easily provide and access data from the Context.
By passing functions to the Context, you can also update the shared data in a simple and efficient way. This makes the Context API a great choice when you have data that needs to be shared across many components or when you have deeply nested components.
I hope this article has helped you understand the Context API in React and how you can use it in your own applications. Remember to check out the React documentation for more information and examples.
Build React Apps with reusable components, just like Lego
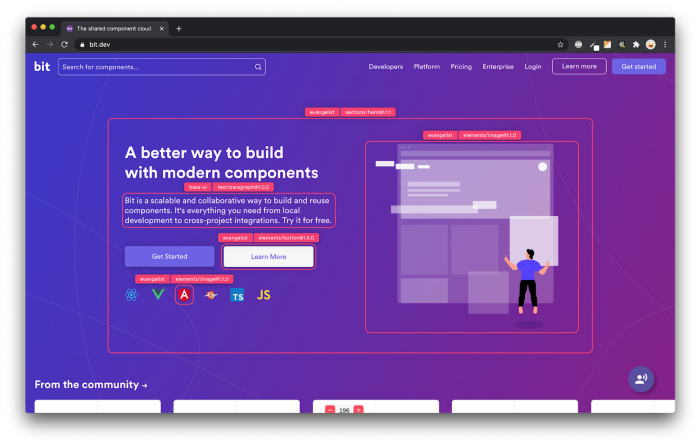
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Learn more:
- Creating a Developer Website with Bit components
- How We Build Micro Frontends
- How we Build a Component Design System
- How to reuse React components across your projects
- 5 Ways to Build a React Monorepo
- How to Create a Composable React App with Bit
- How to Reuse and Share React Components in 2023: A Step-by-Step Guide
Understanding the Power of Context API in React was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by FardaKarimov
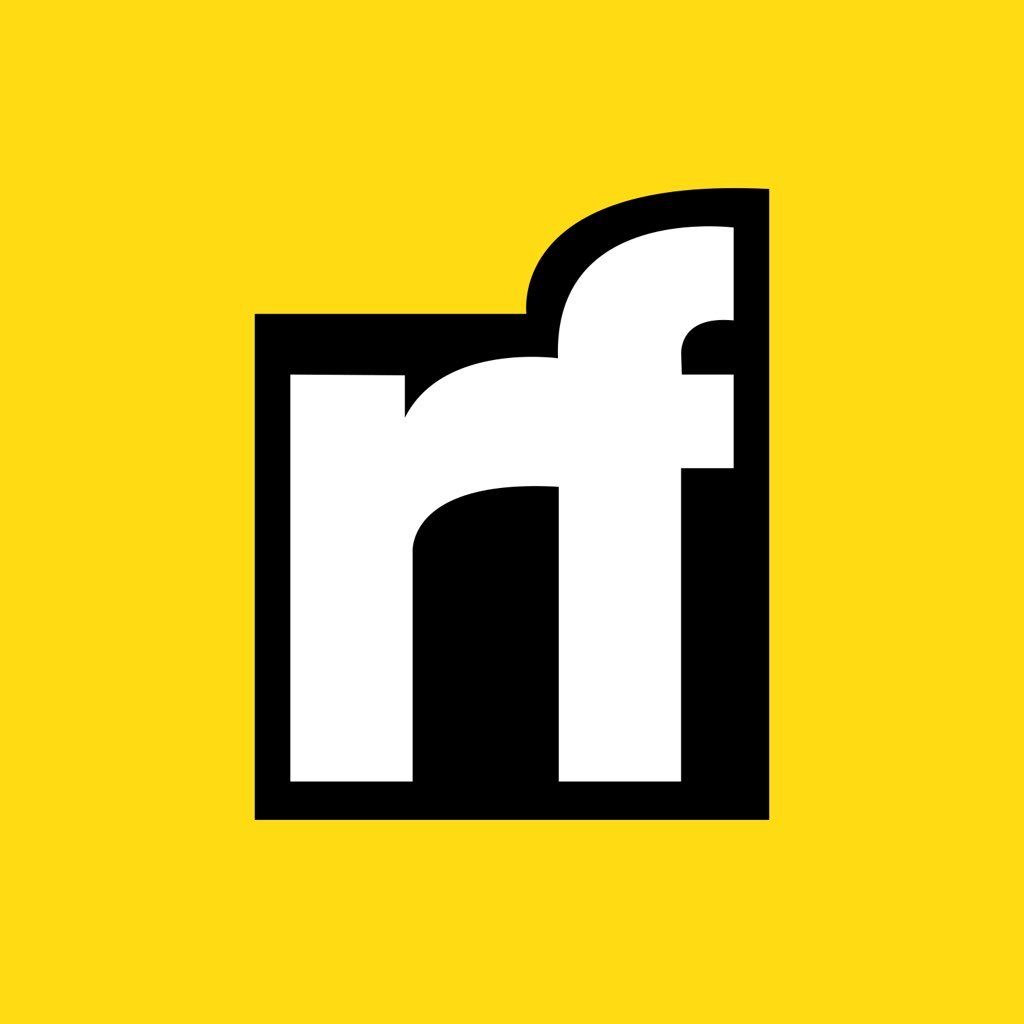
FardaKarimov | Sciencx (2023-05-02T05:53:59+00:00) Understanding the Power of Context API in React. Retrieved from https://www.scien.cx/2023/05/02/understanding-the-power-of-context-api-in-react/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.