This content originally appeared on Bits and Pieces - Medium and was authored by Teslenko Oleh
Part 2: Understanding the Open-Close Principle (OCP)
Hey there! From the previous article, we already know about SOLID and Single Responsibility Principle (SRP) and today let’s talk about the second one — Open-Closed principle. This principle is a fancy name for a simple idea — when you’re adding new functionality to your code, don’t modify existing code. Instead, create new code that extends the existing code.
So, how does this apply to Angular?
Let’s consider the same example of an application that displays a list of products.
Suppose we want to add a new feature that allows users to sort products by category.
💡 Pro Tip: Get the most out of your Angular components by using tools such as Bit. With Bit you can “harvest” them from your codebase and share them on Bit Cloud. You can then reuse these components across all your projects.
Learn more:
Scenario 1: Not using the Open-Closed principle
In this scenario, we might modify the existing ProductComponent to add the sorting functionality. This means we would need to modify the existing code and add new properties and methods to the component. This approach violates the Open-Closed principle because we’re changing existing code instead of extending it.
Here’s what the code might look like:
In this code, we added two new properties (sortBy and sortAscending) and a new method sortProducts() to the ProductComponent.
This approach has a few drawbacks:
- The ProductComponent now has too many responsibilities — displaying products and sorting by category. This violates the Single Responsibility principle.
- If we want to add more sort types in the future, we will have to keep modifying the ProductComponent, which violates the Open-Closed principle.
- If we have other components that display products, we will have to duplicate the sorting code in those components as well.
Scenario 2: Using the Open-Closed Principle
In this scenario, we can create a new component called ProductSorterComponent that handles the sorting functionality. This component will extend the behavior of the existing ProductComponent without modifying it.
Here’s what the code might look like:
In this code, we created a new component called ProductSorterComponent that handles the sorting functionality. The ProductComponent now only displays the products and does not handle the sorting logic. We passed the products, sortBy, sortAscending data from the ProductComponent to the ProductSorterComponent using the @Input() decorator.
This approach has a few advantages:
- The ProductComponent and the ProductSorterComponent each have a single responsibility, which follows the Single Responsibility principle. The ProductComponent displays products, and the ProductSorterComponent sorts products by param.
- If we want to change the sort option, we can pass one to ProductSorterComponent components without modifying ProductComponent. This follows the Open-Closed principle.
- We can reuse the ProductSorterComponent in other components that display products. This avoids duplicating the sorting code in multiple components.
- The ProductComponent and the ProductSorterComponent can be developed and tested independently of each other. This improves the maintainability of the codebase.
In summary, the Open-Closed principle is an important software design principle that encourages code extensibility and maintainability. In Angular, we can apply this principle by creating new components that extend the behavior of existing components without modifying them. This approach helps us to write cleaner, more modular, and more reusable code.
Thanks for reading, if you like this article you can 👏 or write a comment.
See also other articles about design principles:
Best Design Principles in Angular
Build Angular Apps with reusable components, just like Lego
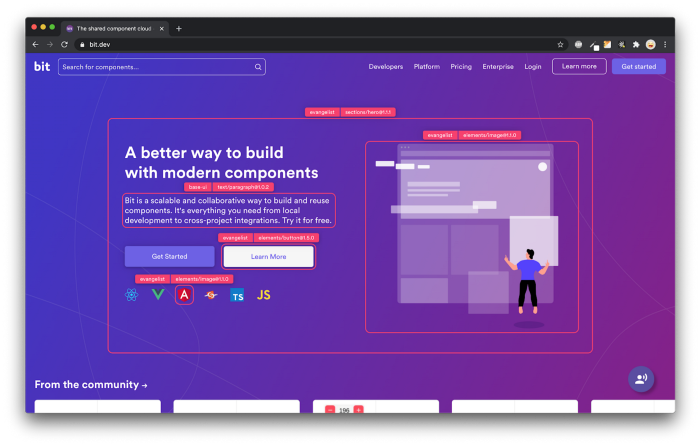
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Learn more:
- Introducing Angular Component Development Environment
- 10 Useful Angular Features You’ve Probably Never Used
- Top 8 Tools for Angular Development in 2023
- Getting Started with a New Angular Project in 2023
- How We Build Micro Frontends
- How to Share Angular Components Between Projects and Apps
- How we Build a Component Design System
- Creating a Developer Website with Bit components
Best Design Principles in Angular was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Teslenko Oleh
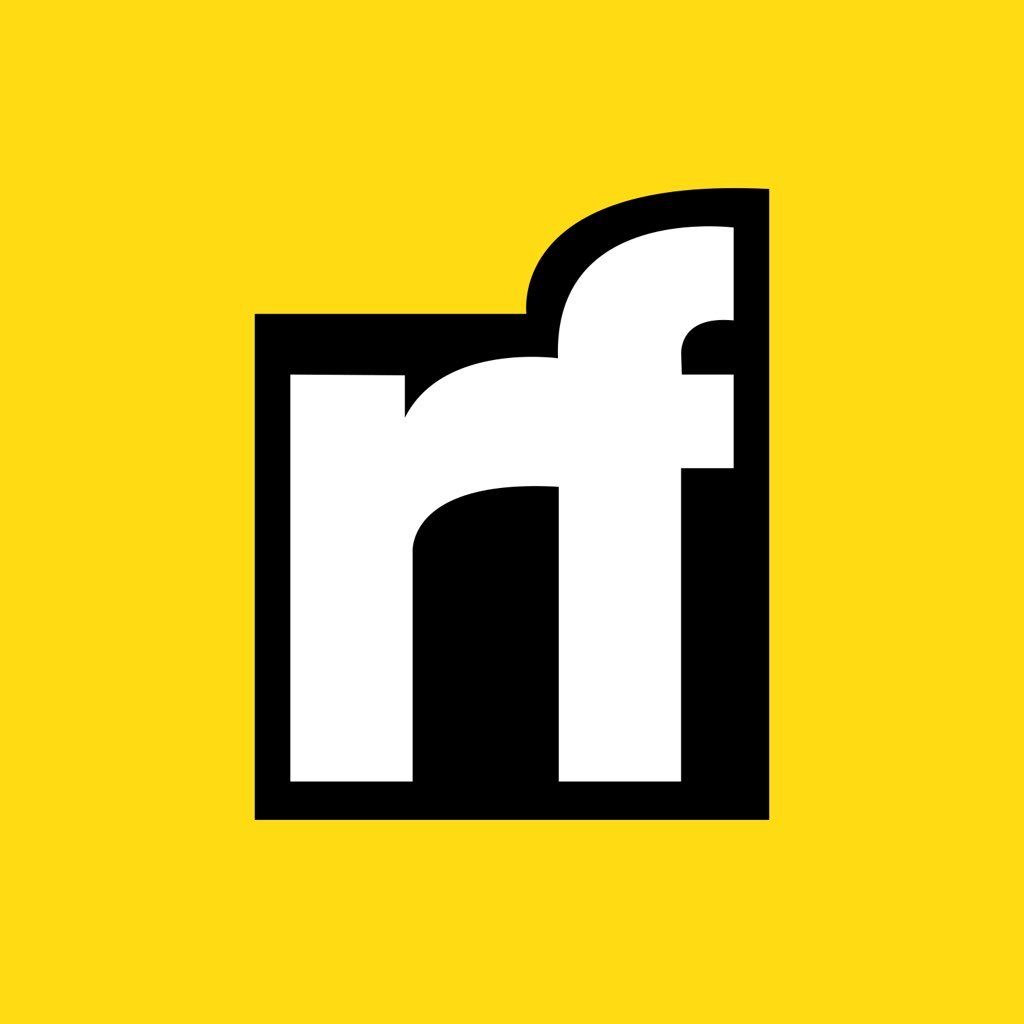
Teslenko Oleh | Sciencx (2023-05-08T03:39:10+00:00) Best Design Principles in Angular. Retrieved from https://www.scien.cx/2023/05/08/best-design-principles-in-angular-2/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.