This content originally appeared on Bits and Pieces - Medium and was authored by André Skjelin Ottosen
Reasons why you should consider using pipes in Angular.
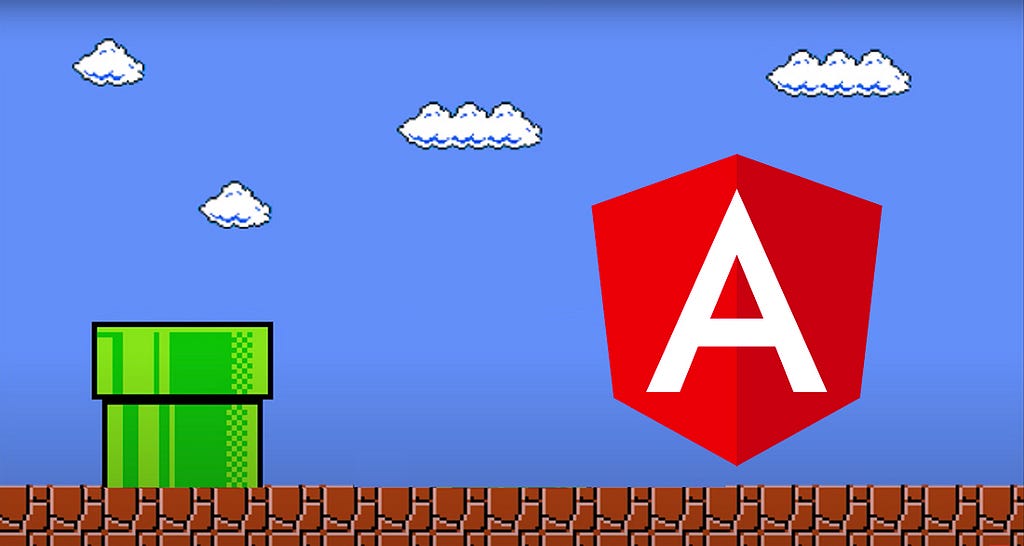
Angular pipes are a versatile feature that simplifies data transformation and formatting within Angular templates. They are used to modify the output of a value before it is displayed to the user. Pipes are represented by the | symbol in template expressions and can be applied to variables, property bindings, and interpolation.
<p>{{ data | myPipe }}</p>
Here are some reasons why you should use pipes in Angular:
- Data transformation: Pipes provide a convenient way to transform data without modifying the underlying values. You can use built-in pipes like DatePipe, UpperCasePipe, CurrencyPipe, or create custom pipes to perform operations such as formatting dates, converting text case, filtering arrays, or applying mathematical calculations.
- Code reusability: Pipes allow you to encapsulate common transformations into reusable components. Once defined, pipes can be used across different components and templates, promoting code reusability and reducing code duplication.
- Readability and maintainability: By using pipes, you can make your templates more readable and expressive. Pipes provide a declarative way to describe the desired data transformation, making it easier for developers to understand the intent of the code. Additionally, encapsulating transformations within pipes enhances maintainability by centralizing the logic in a single location.
- Performance optimization: Angular’s built-in pipes are designed to be efficient and optimized for performance. They use change detection mechanisms to only recalculate the transformed value if the input changes, minimizing unnecessary calculations and improving rendering performance.
- Customizability: Angular allows you to create custom pipes to suit your specific needs. Custom pipes enable you to define and reuse data transformations that are specific to your application’s requirements, providing flexibility and customization options.
Overall, pipes in Angular offer a powerful way to transform and format data in templates. They enhance code readability, reusability, and maintainability, and provide performance optimizations. By leveraging pipes, you can create more expressive and efficient Angular applications.
Demo
UtcToLocalTimePipe
Code: https://stackblitz.com/edit/github-narc4d?file=src/main.ts
The UtcToLocalTimePipe is responsible for transforming a given UTC date into a local date and time format. It utilizes the UtcToLocalTimeService to perform the conversion. The transform method of the pipe takes a Date object as input and an optional argument, and it calls the convertUtcToLocalTime method of the service to obtain the converted local date and time as a string.
utc-to-local-time.pipe.ts
import { Pipe, PipeTransform } from '@angular/core';
import { UtcToLocalTimeService } from '../services/utc-to-local-time.service';
@Pipe({
name: 'utcToLocalTime',
})
export class UtcToLocalTimePipe implements PipeTransform {
constructor(private utcToLocalTimeService: UtcToLocalTimeService) {}
transform(date: Date, args?: any): string {
return this.utcToLocalTimeService.convertUtcToLocalTime(date, args);
}
}
The UtcToLocalTimeService is an injectable service that provides two methods for date and time conversions. The convertUtcToLocalTime method takes a UTC Date object and an optional UtcToLocalTimeFormat argument. It converts the UTC date to a local date and time format based on the specified format or the default format (DateTime) if no format is provided. The conversion is performed using the toLocaleDateString and toLocaleTimeString methods, which take into account the browser's language settings.
The convertLocalTimeToUtc method of the service accepts a local Date object and returns its equivalent UTC representation as a string, using the toUTCString method.
Overall, this code allows for easy conversion between UTC and local date and time formats in an Angular application.
💡 You can now extract this code from your codebase into a package and share it across multiple projects with the help of a component hub like Bit. Bit comes with a component development environment for Angular that provides proper tools, configurations and runtime environment for your components, providing versioning, testing, and dependency management features to simplify the process.
Learn more here:
utc-to-local-time.service.ts
import { Injectable } from '@angular/core';
export enum UtcToLocalTimeFormat {
DateTime = 'datetime', // 01.12.2023 12:00
Date = 'date', // 01.12.2023
Time = 'time', // 12:00
}
@Injectable({
providedIn: 'root',
})
export class UtcToLocalTimeService {
constructor() {}
public convertUtcToLocalTime(
utcDate: Date,
format: UtcToLocalTimeFormat = UtcToLocalTimeFormat.DateTime
): string {
const browserLanguage = 'no-NO';
const date = new Date(utcDate).toLocaleDateString(browserLanguage, {
month: '2-digit',
day: '2-digit',
year: 'numeric',
});
const time = new Date(utcDate).toLocaleTimeString(browserLanguage, {
hour: '2-digit',
minute: '2-digit',
});
if (format === UtcToLocalTimeFormat.Date) {
return `${date}`;
} else if (format === UtcToLocalTimeFormat.Time) {
return `${time}`;
} else {
return `${date} ${time}`;
}
}
public convertLocalTimeToUtc(localDate: Date): string {
const date = new Date(localDate);
return date.toUTCString();
}
}
app.component
<div class="container">
<p>{{ date | utcToLocalTime : "datetime" }}</p>
<p>{{ date | utcToLocalTime : "date" }}</p>
<p>{{ date | utcToLocalTime : "time" }}</p>
</div>
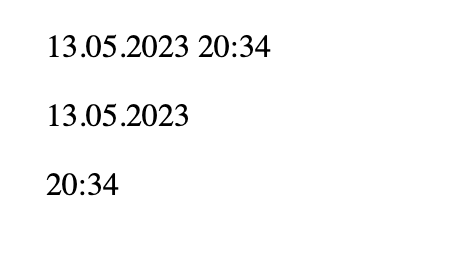
That’s it. I hope this helped you. Feel free to follow me on Medium.
If you would like to connect with me on LinkedIn, please feel free to visit my LinkedIn profile at https://www.linkedin.com/in/skjelin-ottosen/
Build Angular Apps with reusable components, just like Lego
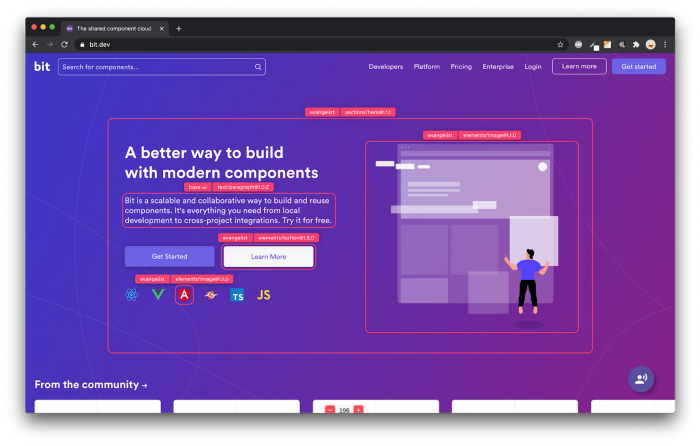
Bit’s open-source tool help 250,000+ devs to build apps with components.
Turn any UI, feature, or page into a reusable component — and share it across your applications. It’s easier to collaborate and build faster.
Split apps into components to make app development easier, and enjoy the best experience for the workflows you want:
→ Micro-Frontends
→ Design System
→ Code-Sharing and reuse
→ Monorepo
Learn more:
- Introducing Angular Component Development Environment
- 10 Useful Angular Features You’ve Probably Never Used
- Top 8 Tools for Angular Development in 2023
- Getting Started with a New Angular Project in 2023
- How We Build Micro Frontends
- How to Share Angular Components Between Projects and Apps
- How we Build a Component Design System
- Creating a Developer Website with Bit components
What are Pipes in Angular and Why Should We Use Them? was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by André Skjelin Ottosen
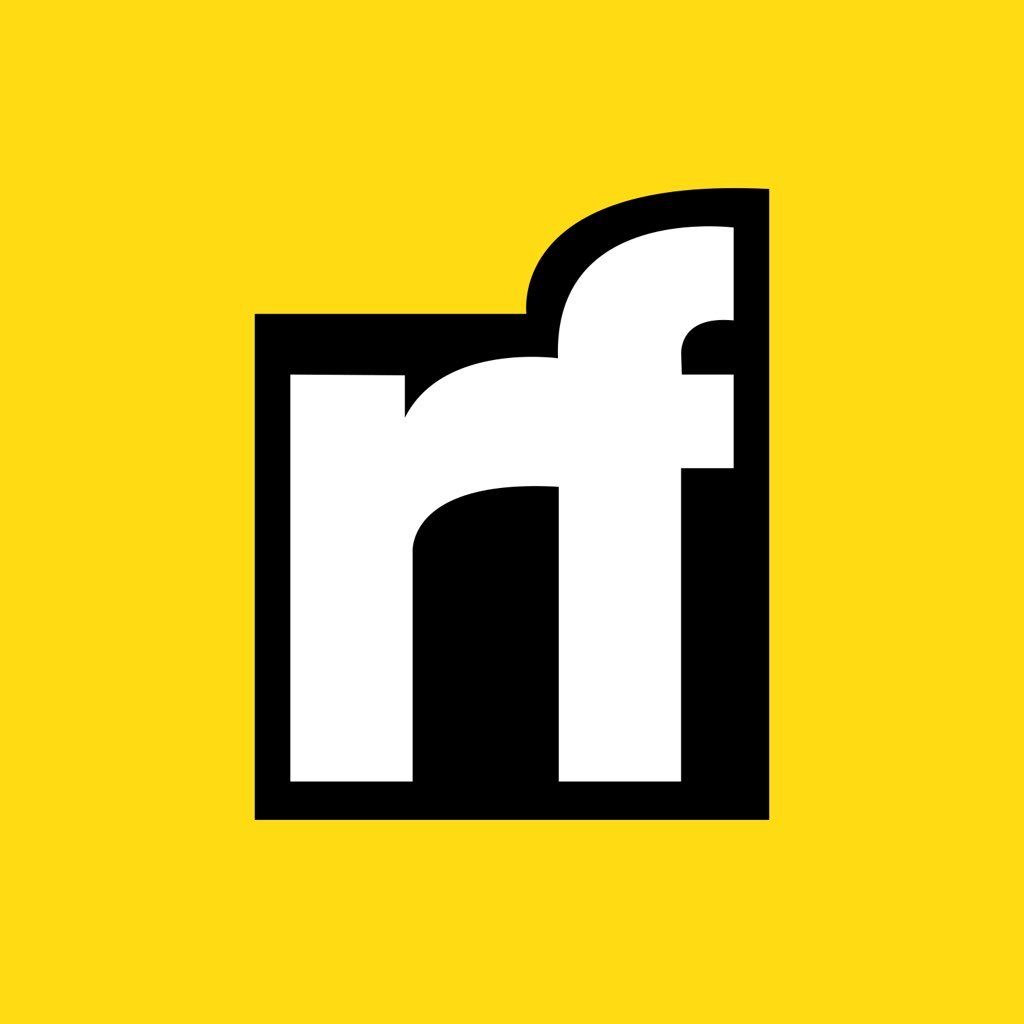
André Skjelin Ottosen | Sciencx (2023-05-16T08:36:26+00:00) What are Pipes in Angular and Why Should We Use Them?. Retrieved from https://www.scien.cx/2023/05/16/what-are-pipes-in-angular-and-why-should-we-use-them/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.