This content originally appeared on DEV Community and was authored by Salvietta150x40
Part 2: Advanced Features and Best Practices
In the first part of this series, we explored the fundamentals of the Payment Request API and how it simplifies the payment experience. Now, let's delve deeper into advanced features and best practices to further optimize the integration of this powerful API.
1. Customizing Payment Request UI
The Payment Request API provides flexibility in customizing the user interface (UI) to match the branding and design of your website. You can modify the look and feel of the payment sheet, including colors, fonts, and layout, to provide a consistent user experience.
// Customize payment sheet styles paymentRequest.addEventListener('shippingaddresschange', (event) => { const paymentRequest = event.target; // Modify UI elements based on the updated shipping address // ... });
2. Handling Events and Callbacks
The Payment Request API offers various events and callbacks to handle different stages of the payment process. You can utilize these hooks to display additional information to users, update the UI based on user actions, or handle errors gracefully.
// Listen for payment request events paymentRequest.addEventListener('shippingoptionchange', (event) => { const paymentRequest = event.target; // Update payment details based on the selected shipping option // ... });
3. Address and Shipping Information
To streamline the checkout process, you can leverage the Payment Request API to request address and shipping information from users. This enables a seamless flow where users can provide their shipping details without having to fill in lengthy forms manually.
// Request shipping information const shippingOptions = [ { id: 'standard', label: 'Standard Shipping', amount: { currency: 'USD', value: '5.00' }, selected: true, }, { id: 'express', label: 'Express Shipping', amount: { currency: 'USD', value: '10.00' }, }, ]; const paymentDetails = { total: { label: 'Total', amount: { currency: 'USD', value: '100.00' }, }, shippingOptions, }; const paymentRequest = new PaymentRequest(supportedPaymentMethods, paymentDetails);
4. Handling Payment Method Changes
Users may change their preferred payment method during the checkout process. The Payment Request API provides the necessary mechanisms to detect and handle these changes dynamically. You can update the payment details or display relevant information based on the selected payment method.
// Listen for payment method change event paymentRequest.addEventListener('paymentmethodchange', (event) => { const paymentRequest = event.target; // Update payment details based on the selected payment method // ... });
5. Supporting Digital Wallets and Mobile Payments
In addition to traditional payment methods, the Payment Request API supports digital wallets and mobile payment platforms. By integrating with popular digital wallets like Apple Pay, Google Pay, or Samsung Pay, you can offer users a fast and convenient payment experience on their mobile devices.
// Support digital wallets const supportedPaymentMethods = [ { supportedMethods: ['basic-card'], data: { supportedNetworks: ['visa', 'mastercard'], }, }, { supportedMethods: ['https://apple.com/apple-pay'], }, { supportedMethods: ['https://google.com/pay'], data: { merchantIdentifier: '0123456789', allowedPaymentMethods: ['TOKENIZED_CARD // CARD'], }, ]; const paymentRequest = new PaymentRequest(supportedPaymentMethods, paymentDetails);
6. Enhanced Security with Payment Handlers
To further enhance security, you can utilize payment handlers, which are secure payment intermediaries, to process the payment request. Payment handlers provide an additional layer of protection by encrypting sensitive payment information and ensuring secure communication between the website and the payment gateway.
// Create a payment handler const paymentHandler = paymentRequest.createPaymentHandler('https://example.com/payment-handler');
7. Testing and Debugging
During the integration process, it is crucial to thoroughly test and debug your Payment Request API implementation. Use browser developer tools and testing environments to simulate various scenarios, handle errors effectively, and ensure a smooth payment flow.
// Test and debug your payment request implementation console.log(paymentRequest.supportedMethods); // Log supported payment methods console.log(paymentDetails.total.amount.value); // Log the total amount
By implementing these advanced features and following best practices, you can unlock the full potential of the Payment Request API and provide a seamless, secure, and user-friendly payment experience to your customers.
In conclusion, the Payment Request API revolutionizes online payments by simplifying the checkout process, supporting multiple payment methods, and prioritizing security. With advanced features and best practices, you can optimize the integration and take full advantage of this powerful API.
Stay tuned for the next part of this series, where we will explore real-world examples and case studies showcasing the successful implementation of the Payment Request API.
This content originally appeared on DEV Community and was authored by Salvietta150x40
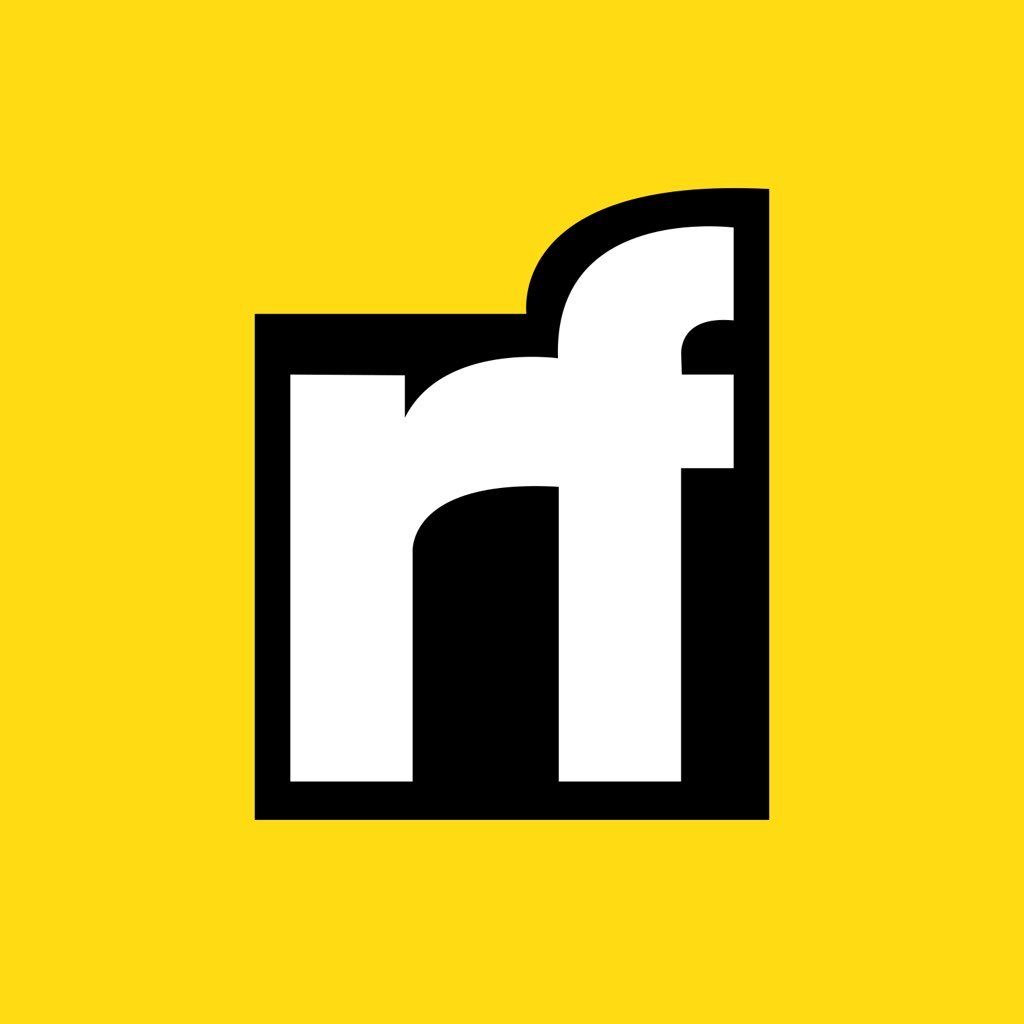
Salvietta150x40 | Sciencx (2023-06-05T15:31:00+00:00) The Payment Request API: Revolutionizing Online Payments (Part 2). Retrieved from https://www.scien.cx/2023/06/05/the-payment-request-api-revolutionizing-online-payments-part-2/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.