This content originally appeared on Level Up Coding - Medium and was authored by Aman Saxena
Concurrency is a fundamental aspect of Go, and channels are a core feature that facilitates communication between goroutines. This article dives into the mechanics of channels, provides practical examples, discusses common use cases, highlights potential pitfalls, and explains how to handle errors effectively, including creating dedicated error channels. Continuing from the previous article.
Introduction to Channels
Channels in Go allow goroutines to communicate with each other and synchronize their execution. They are typed conduits through which you can send and receive values. Channels are created using the make function and can be either buffered or unbuffered.
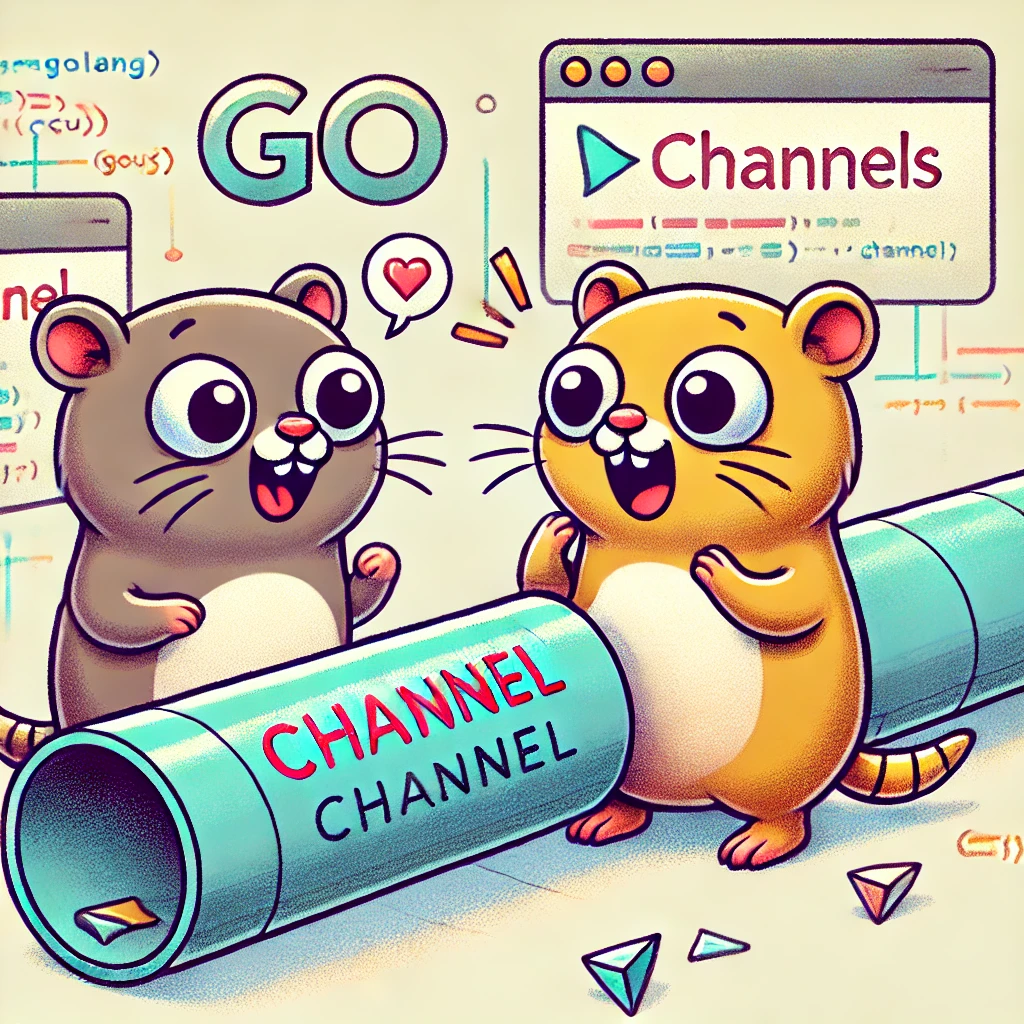
Basic Example of Channels
Let’s start with a simple example of how channels work:
package main
import (
"fmt"
)
func main() {
ch := make(chan int) // Create an unbuffered channel
go func() {
ch <- 42 // Send value to the channel
}()
val := <-ch // Receive value from the channel
fmt.Println(val) // Output: 42
}
In this example, a goroutine sends the value 42 to the channel ch, and the main function receives it. This demonstrates basic send-and-receive operations using an unbuffered channel.
Use Cases for Channels
- Pipeline Pattern: Channels can be used to connect stages in a pipeline, where each stage processes data and passes it to the next stage via a channel.
package main
import (
"fmt"
)
func main() {
numbers := make(chan int)
squares := make(chan int)
go func() {
for x := 0; x < 5; x++ {
numbers <- x
}
close(numbers)
}()
go func() {
for x := range numbers {
squares <- x * x
}
close(squares)
}()
for square := range squares {
fmt.Println(square)
}
}
2. Worker Pools: Channels facilitate the creation of worker pools, where multiple workers consume tasks from a shared channel.
package main
import (
"fmt"
"sync"
)
func worker(id int, jobs <-chan int, results chan<- int) {
for j := range jobs {
fmt.Printf("Worker %d started job %d\n", id, j)
results <- j * 2
}
}
func main() {
const numJobs = 5
jobs := make(chan int, numJobs)
results := make(chan int, numJobs)
for w := 1; w <= 3; w++ {
go worker(w, jobs, results)
}
for j := 1; j <= numJobs; j++ {
jobs <- j
}
close(jobs)
for a := 1; a <= numJobs; a++ {
fmt.Printf("Result: %d\n", <-results)
}
}
Pitfalls of Using Channels
- Deadlocks: Deadlocks occur when goroutines are waiting indefinitely for messages that are never sent. Properly structuring your channel operations and using select statements can help avoid deadlocks.
- Resource Leaks: Forgetting to close channels can lead to resource leaks. Always ensure channels are closed when no longer needed, especially in producer-consumer patterns.
- Panic on Closed Channels: Sending on a closed channel causes panic. Make sure to handle channel closure carefully and signal completion correctly.
Error Handling in Channels
Error handling in channels can be achieved by creating dedicated error channels. This allows you to separate regular data flow from error messages, making your code more robust and easier to debug.
package main
import (
"errors"
"fmt"
)
func worker(id int, jobs <-chan int, results chan<- int, errs chan<- error) {
for j := range jobs {
if j%2 == 0 { // Simulate an error for even numbers
errs <- errors.New(fmt.Sprintf("worker %d: error processing job %d", id, j))
} else {
results <- j * 2
}
}
}
func main() {
const numJobs = 5
jobs := make(chan int, numJobs)
results := make(chan int, numJobs)
errs := make(chan error, numJobs)
for w := 1; w <= 3; w++ {
go worker(w, jobs, results, errs)
}
for j := 1; j <= numJobs; j++ {
jobs <- j
}
close(jobs)
for a := 1; a <= numJobs; a++ {
select {
case res := <-results:
fmt.Printf("Result: %d\n", res)
case err := <-errs:
fmt.Printf("Error: %s\n", err)
}
}
}
In this example, a dedicated error channel errs is used to handle errors. The worker function sends errors to this channel when it encounters an issue. The select statement in the main function listens for messages on both the results and errs channels, ensuring that errors are properly handled.
Conclusion
Channels are a powerful feature in Go that enables concurrent communication between goroutines. By understanding their mechanics, common use cases, and potential pitfalls, you can leverage channels to write efficient and reliable concurrent programs. Additionally, by incorporating error handling with dedicated error channels, you can further enhance the robustness of your applications.
Feel free to connect with me on LinkedIn!
Mastering Channels in Golang: Examples, Use Cases, Pitfalls, and Error Handling was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Aman Saxena
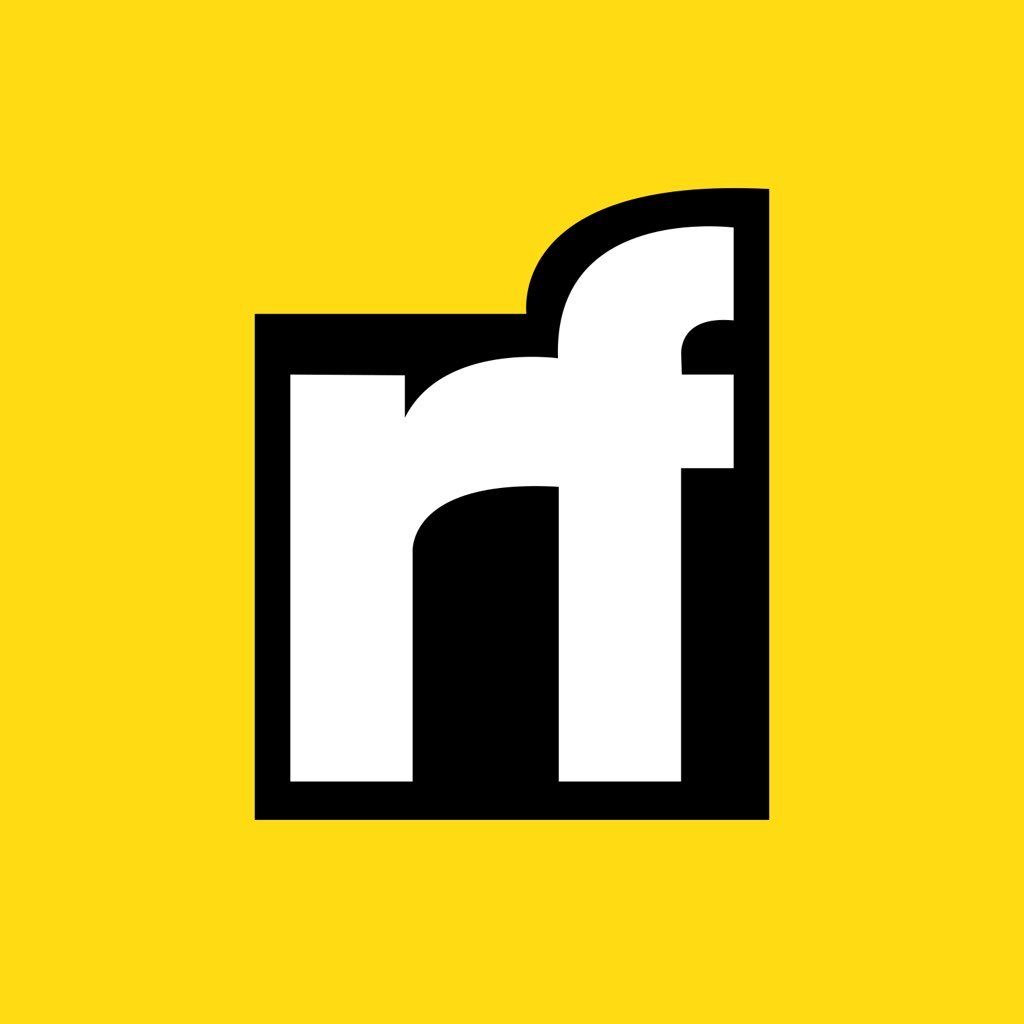
Aman Saxena | Sciencx (2024-06-30T18:08:47+00:00) Mastering Channels in Golang: Examples, Use Cases, Pitfalls, and Error Handling. Retrieved from https://www.scien.cx/2024/06/30/mastering-channels-in-golang-examples-use-cases-pitfalls-and-error-handling/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.