This content originally appeared on HackerNoon and was authored by Nikolai Babkin
Python is a highly popular programming language, widely used in various fields like software development, data analysis, AI, and machine learning. Its simple syntax and structure make it easy to learn and work with. With such a broad applications, code optimization – ensuring efficient and effective functioning of software – is a critical requirement.
\ As a Software Engineer who has developed resource-intensive applications in various sectors, from banking to social media platforms, I see code optimization as a critical aspect of Python development. In this article, we will go through some optimization strategies, covering everything from profiling to JIT compilation and concurrency. The goal is to provide you with practical tools and methods for improving your Python code's performance, so that you can be certain that your Python code is optimized for today's fast-paced digital environment.
Understanding Python's Performance Characteristics
Let’s review the key factors that affect Python’s performance before we turn to actual optimization techniques.
\ Python's performance is deeply influenced by its interpreter architecture. Unlike compiled languages that convert code into machine language beforehand, Python processes code line-by-line.
\ This line-by-line execution offers advantages in rapid prototyping and development flexibility but comes with trade-offs in speed, especially in tasks requiring intensive computation. It is important to recognize and address this issue, choosing more efficient data structures, algorithms, and external libraries for performance-critical sections.
Common Performance Bottlenecks in Python
The typical bottleneck areas described below can significantly impact runtime efficiency, and understanding them is key to enhancing overall performance.
\
- I/O Bound Bottlenecks: I/O operations with external sources like files, databases, or networks, often slow down Python applications. E.g., a Python script that reads large files line-by-line can become I/O bound, as the system spends too much time waiting for I/O operations to complete, rather than executing code. Optimizing I/O bound processes often involves techniques like asynchronous programming or employing more efficient data access methods.
\
CPU Bound Bottlenecks: CPU-bound processes are another common bottleneck, particularly in data processing and complex calculations. A typical example is a Python script performing processing large datasets in a loop. Such tasks can be optimized by vectorisation, using libraries that perform computations in compiled code (like NumPy), or leveraging parallel processing techniques.
\
By identifying whether a bottleneck is I/O or CPU-bound, developers can apply targeted optimization strategies, ensuring that Python code runs efficiently and effectively in various scenarios.
Profiling Python Applications 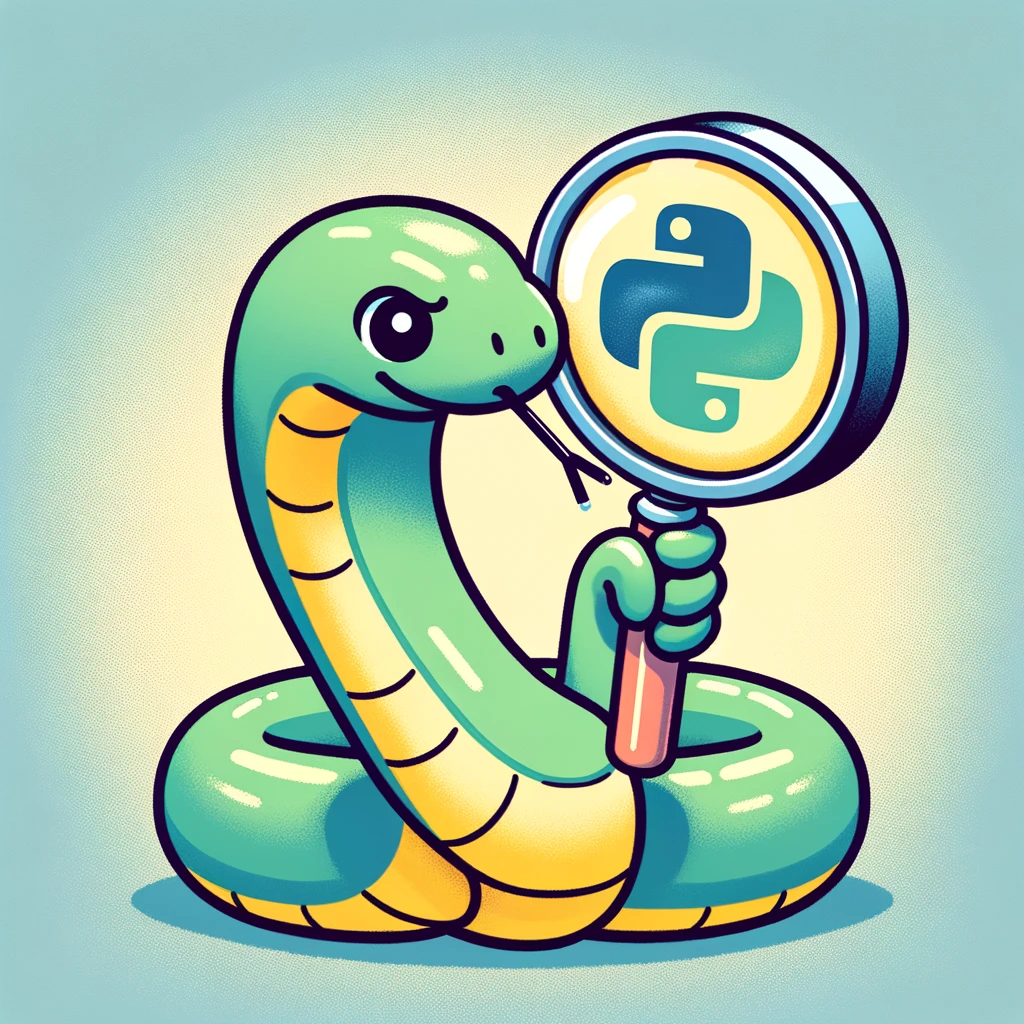
Profiling involves assessing the behavior of the code, identifying areas that are inefficient or slow, and providing insights for performance improvements. This could include identifying functions that take the longest to execute or pinpointing lines of code that are the most memory-intensive.
\ Profiling equips developers with the necessary data to make informed decisions about where to focus optimization efforts.
\ In Python, several tools and techniques can be used for profiling, each with its strengths and ideal use cases. Let’s explore some of the most powerful ones.
\ cProfile is a widely used profiler in Python. It provides a comprehensive overview of function calls, measuring the time spent in each function. This tool is useful for identifying time-consuming parts of the code.
\ For more detailed analysis, line_profiler breaks down the execution time line by line, offering a granular view of the code's performance. Line Profiler is quite straightforward to use even inside Jupyter or IPython session.
\
Let’s look at process_data
function as an example of line_profiler
usage:
\
# example.py
import numpy as np
def process_data(n, m):
data = np.random.rand(n, m)
output = np.zeros((n, m))
for i in range(n):
for j in range(m):
output[i, j] = np.sin(data[i, j]) + np.cos(data[i, j])
return output
def main(n, m):
_ = process_data(n, m)
\
Let’s now profile process_data
function in ipython
session. It is worth mentioning that any function can be profiled and to profile a function, it should be imported in the session:
>>> from example import process_data, main
>>> %load_ext line_profiler
>>> %lprun -f process_data main(1000, 1000)
Timer unit: 1e-09 s
Total time: 1.09929 s
File: /Users/nikolai_babkin/PycharmProjects/demo_py/example.py
Function: process_data at line 4
Line # Hits Time Per Hit % Time Line Contents
==============================================================
4 def process_data(n, m):
5 1 10683000.0 1e+07 1.0 data = np.random.rand(n, m)
6 1 1538000.0 2e+06 0.1 output = np.zeros((n, m))
7
8 1001 104000.0 103.9 0.0 for i in range(n):
9 1001000 88985000.0 88.9 8.1 for j in range(m):
10 1000000 997978000.0 998.0 90.8 output[i, j] = np.sin(data[i, j]) + np.cos(data[i, j])
11 1 0.0 0.0 0.0 return output
\
We can see that line 10 is being extensively accessed (1000000 hits) and is overall responsible for 90% of the runtime. This should be a good target for improvements. Let’s try to rewrite process_data
function utilizing vectorization this time:
# example.py
def process_data_improved(n, m):
data = np.random.rand(n, m)
output = np.sin(data) + np.cos(data) # Vectorized operation
return output
\ Now, we can see a 30x improvement in speed as well as we no longer observe inefficient iterations over the same part of the code:
>>> from example import process_data_improved, main
>>> %load_ext line_profiler
>>> %lprun -f process_data_improved main(1000, 1000)
Timer unit: 1e-09 s
Total time: 0.032837 s
File: /Users/nikolai_babkin/PycharmProjects/demo_py/example.py
Function: process_data_improved at line 13
Line # Hits Time Per Hit % Time Line Contents
==============================================================
13 def process_data_improved(n, m):
14 1 10338000.0 1e+07 31.5 data = np.random.rand(n, m)
15 1 22499000.0 2e+07 68.5 output = np.sin(data) + np.cos(data)
16 1 0.0 0.0 0.0 return output
\ I would recommend using line_profiler even when you don't have concerns about code performance bottlenecks. It's easy to use, and the potential benefits of discovering inefficiencies in the code could save considerable time and computational resources.
\ Memory usage is another critical aspect of optimization. Tools like memory_profiler monitor the memory consumption of a Python application, helping to identify memory leaks or inefficient memory usage.
\
pyinstrument
offers a different approach, using a statistical method to record the call stack at regular intervals. This method is less intrusive and can be more accurate for programs with many short function calls, providing a high-level view of where the program spends most of its time. The key to effective profiling lies in correctly interpreting the data these tools provide.
\ Profiling data should guide decisions such as whether to refactor code for efficiency, optimize certain functions, or even rewrite parts of the application using more efficient algorithms.
\ One of the general suggestions would be to make profiling an iterative process, with each round of changes followed by re-profiling to measure the impact and guide further optimization.
Techniques for Code Optimization 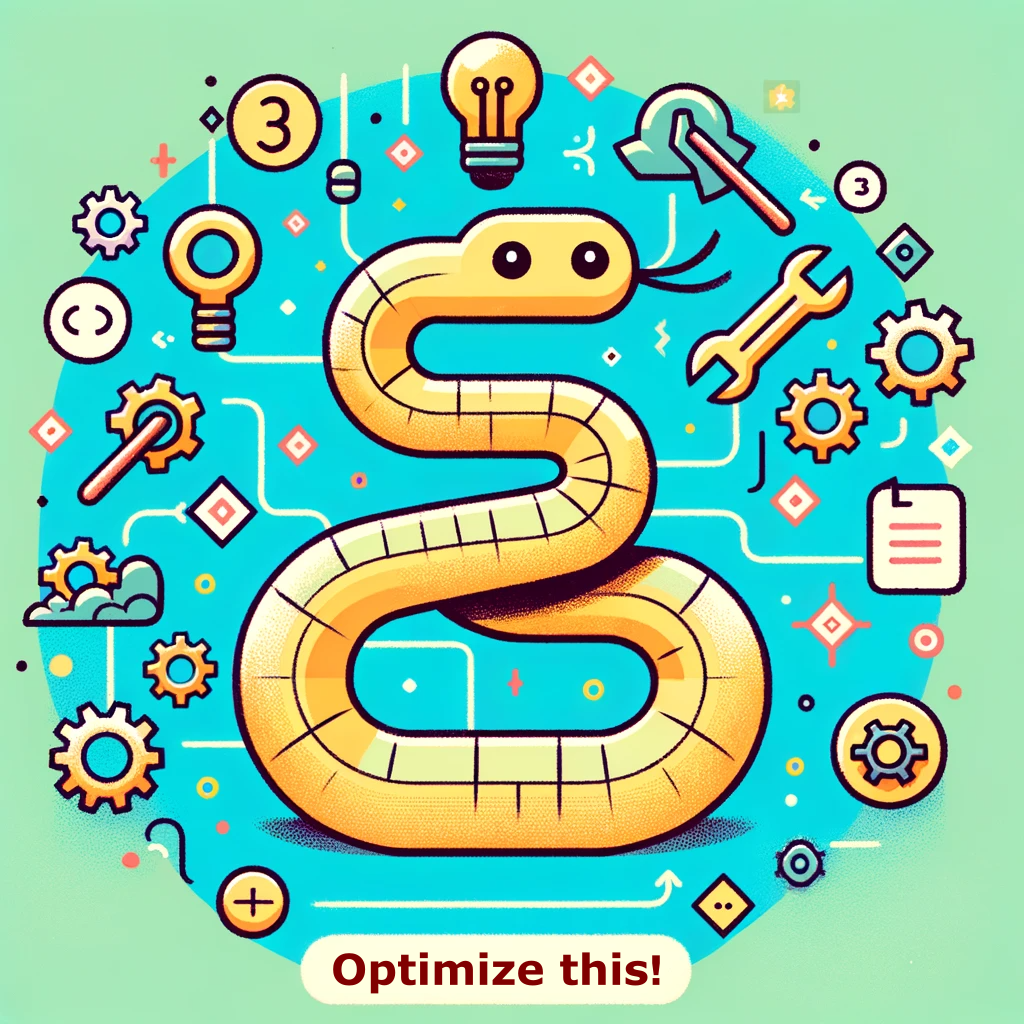
Optimising Python code involves a variety of techniques. Let’s explore several key strategies that can significantly improve the execution speed and resource utilization of your Python code.
Algorithm Optimisation in Python
Algorithm efficiency directly impacts the execution speed and resource utilization of an application.
\ Reducing Time Complexity: The concept of time complexity relates to the growth of an algorithm's execution time as input data expands.
\ Opting for algorithms with lesser time complexity can notably enhance the speed of our applications.
\ For instance, a search for an element in an unsorted list has linear time complexity O(n). In the case of performing at least 10 searches in the same considerably long list, it might be more efficient to sort the list first and then perform a binary search on a sorted list. The resulting time complexity will be O(k * log n) + O(n * log n), in most cases less than O(k * n).
\ By carefully considering time and space complexity in algorithm selection, we can optimize our applications for better performance and resource utilization.
Optimizing Data Structures and Memory Usage
Choosing the Right Data Structures: Different data structures offer various benefits for specific tasks. E.g., using lists for operations that frequently insert or delete elements can be less efficient compared to linked lists. However, lists are more efficient for indexed access.
\ Similarly, sets are ideal for membership testing as they provide O(1) complexity, while dictionaries are perfect for key-value pair data with fast lookups.
\ Memory Management Techniques: Memory management in Python is largely handled by the Python memory manager, but developers can optimize memory usage. This includes understanding object mutability, using generators for large data iterations, and utilizing inbuilt methods like slots to reduce the size of objects. Avoiding memory leaks by ensuring that references to objects are removed when no longer needed is also crucial.
\ Efficient Use of Arrays and Collections: For numerical data, arrays (such as those provided by NumPy) are more memory-efficient than lists. They provide contiguous memory storage, leading to better cache utilization and performance. The collections module in Python also offers specialized data structures like defaultdict, Counter, and deque, optimized for specific use cases.
Optimization of I/O-Bound Tasks
One effective approach to optimize I/O-bound tasks is asynchronous programming. This method entails crafting code that manages I/O operations while allowing other parts of the program to run uninterrupted. Tools such as the asyncio
library offers a framework for creating asynchronous Python code, enabling simultaneous handling of several I/O tasks.
\ For file I/O tasks, employing efficient data reading and writing techniques is key. Utilizing methods like buffered I/O or memory-mapped files can significantly improve performance over processing data in smaller, more fragmented pieces. Utilizing libraries like pandas for handling large data files can also lead to better performance.
\ For applications that interact with databases, optimizing query execution and data retrieval is key. This includes writing efficient SQL queries, using connection pooling, and leveraging database caching. ORM (Object-Relational Mapping) frameworks like SQLAlchemy can help in writing efficient database interactions in Python.
\
Network operations can be optimized using asynchronous requests, optimizing data payloads, and managing connections effectively can reduce waiting times. Tools like requests for synchronous operations and aiohttp
for asynchronous operations can be used to optimize network requests in Python applications.
CPU-Bound Tasks Optimization
The distinction between multithreading and multiprocessing is vital for optimizing CPU-intensive tasks In Python: the presence of the Global Interpreter Lock means that multithreading might not always enhance performance for CPU-bound operations. In contrast, multiprocessing involves running parallel processes that bypass the GIL, which increases performance for CPU-intensive tasks.
\ For compute-intensive operations, using C extensions can be highly beneficial. Libraries like NumPy and SciPy, which perform heavy computations in optimized C code, can offer significant speedups. Additionally, tools like Cython allow Python code to be compiled into C extensions, enabling a closer performance to that of pure C.
\
Certain CPU-bound tasks that also involve I/O can be optimized by combining CPU processing with asyncio
for concurrent I/O. This approach is particularly effective when the CPU-intensive part of the task can be split into smaller parts that can be interleaved with I/O operations.
JIT Compilation
Just-In-Time compilation enhances Python performance by converting code into machine code at runtime, especially benefiting CPU-bound tasks.
\ One of the most popular JIT compilers is PyPy. It's particularly effective for long-running applications, adapting to specific usage patterns to improve speed.
\ Numba focuses on numerical functions, using decorators to compile Python functions into optimized machine code. This approach is beneficial for computation-heavy tasks, often yielding performance close to C or Fortran.
\ While JIT can significantly boost performance, it may increase memory usage and introduce startup delays. Its effectiveness varies across different types of Python code, so don’t forget about using profiling!
Optimizing Python code involves a diverse range of strategies, from refining algorithms and data structures to employing sophisticated methods like JIT compilation and concurrency. Each technique outlined above contributes to boosting the performance of Python applications. By applying these techniques, you can transform your Python code into more efficient, faster, and resource-optimized versions, suitable for handling complex and demanding tasks.
\ The journey toward mastering Python optimization requires a combination of theoretical understanding and practical application. As Python continues to evolve and find its place in new and diverse domains, the need for optimization becomes increasingly important. I encourage you to explore and experiment with different methods, always striving for the perfect balance between code efficiency and functionality.
\ Remember, the skills and knowledge you cultivate in optimization today will shape the quality and performance of your Python applications tomorrow!
\ Useful links:
- AsyncIO guide: https://realpython.com/async-io-python/
- Concurrency in Python: https://realpython.com/python-concurrency/
\
This content originally appeared on HackerNoon and was authored by Nikolai Babkin
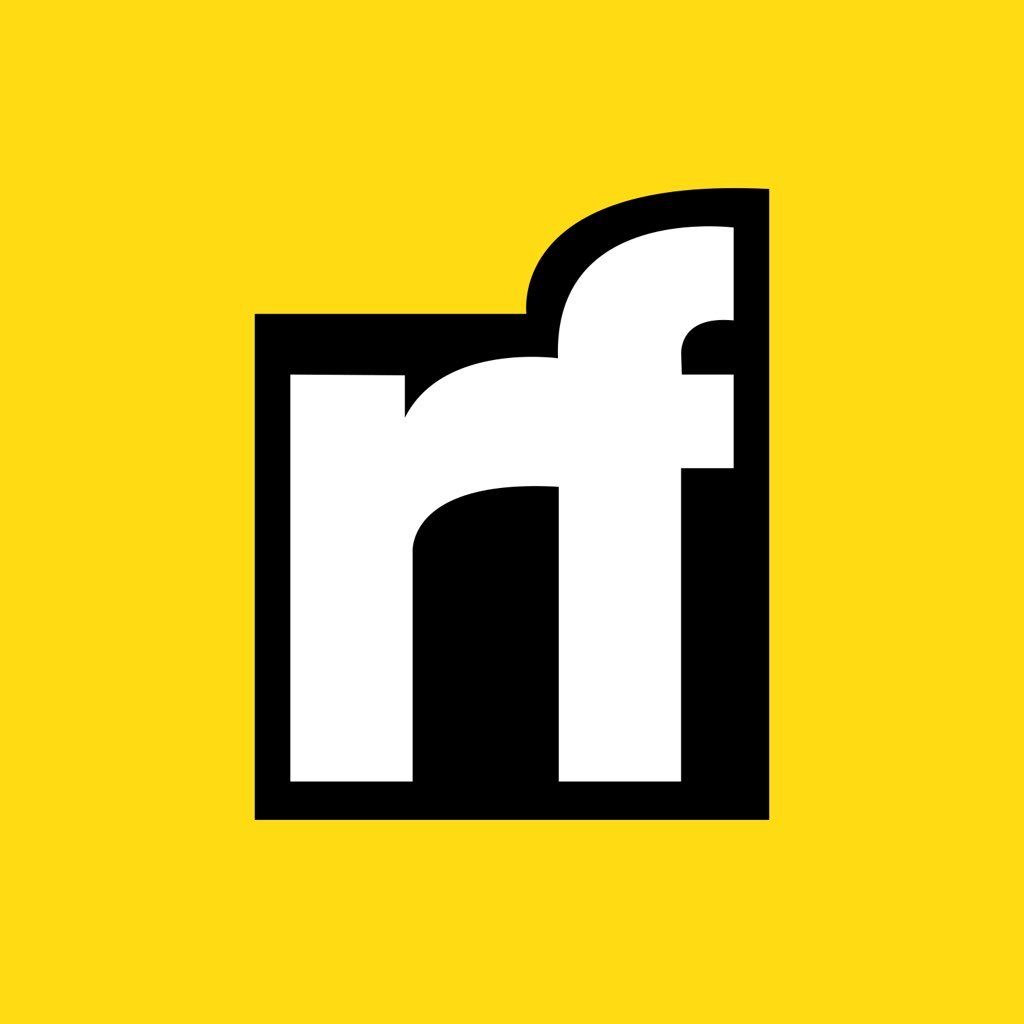
Nikolai Babkin | Sciencx (2024-07-02T00:19:43+00:00) Elevate Your Python: Advanced Techniques for Code Optimization. Retrieved from https://www.scien.cx/2024/07/02/elevate-your-python-advanced-techniques-for-code-optimization/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.