This content originally appeared on DEV Community and was authored by Super Kai (Kazuya Ito)
*Memos:
-
My post explains how to create
nan
andinf
in PyTorch. -
My post explains the arithmetic operations with
nan
andinf
in PyTorch.
The comparisons with torch.nan
and 100.0
, torch.nan
or torch.inf
as shown below:
*Memos:
- The comparisons(except
!=
) withnan
are alwaysFalse
. -
complex
type cannot use>
,<
,>=
and<=
.
import torch
""" `float` type """
torch.nan == 100.0 # False
torch.nan == torch.nan # False
torch.nan == -torch.nan # False
torch.nan == torch.inf # False
torch.nan == -torch.inf # False
torch.nan != 100.0 # True
torch.nan != torch.nan # True
torch.nan != -torch.nan # True
torch.nan != torch.inf # True
torch.nan != -torch.inf # True
torch.nan > 100.0 # False
torch.nan > torch.nan # False
torch.nan > -torch.nan # False
torch.nan > torch.inf # False
torch.nan > -torch.inf # False
torch.nan < 100.0 # False
torch.nan < torch.nan # False
torch.nan < -torch.nan # False
torch.nan < torch.inf # False
torch.nan < -torch.inf # False
torch.nan >= 100.0 # False
torch.nan >= torch.nan # False
torch.nan >= -torch.nan # False
torch.nan >= torch.inf # False
torch.nan >= -torch.inf # False
torch.nan <= 100.0 # False
torch.nan <= torch.nan # False
torch.nan <= -torch.nan # False
torch.nan <= torch.inf # False
torch.nan <= -torch.inf # False
""" `complex` type """
complex(torch.nan, torch.nan) == (100.0+0.j) # False
complex(torch.nan, torch.nan) == complex(torch.nan, torch.nan) # False
complex(torch.nan, torch.nan) == complex(torch.nan, -torch.nan) # False
complex(torch.nan, torch.nan) == complex(-torch.nan, torch.nan) # False
complex(torch.nan, torch.nan) == complex(-torch.nan, -torch.nan) # False
complex(torch.nan, torch.nan) == complex(torch.inf, torch.inf) # False
complex(torch.nan, torch.nan) == complex(torch.inf, -torch.inf) # False
complex(torch.nan, torch.nan) == complex(-torch.inf, torch.inf) # False
complex(torch.nan, torch.nan) == complex(-torch.inf, -torch.inf) # False
complex(torch.nan, torch.nan) != (100.0+0.j) # True
complex(torch.nan, torch.nan) != complex(torch.nan, torch.nan) # True
complex(torch.nan, torch.nan) != complex(torch.nan, -torch.nan) # True
complex(torch.nan, torch.nan) != complex(-torch.nan, torch.nan) # True
complex(torch.nan, torch.nan) != complex(-torch.nan, -torch.nan) # True
complex(torch.nan, torch.nan) != complex(torch.inf, torch.inf) # True
complex(torch.nan, torch.nan) != complex(torch.inf, -torch.inf) # True
complex(torch.nan, torch.nan) != complex(-torch.inf, torch.inf) # True
complex(torch.nan, torch.nan) != complex(-torch.inf, -torch.inf) # True
The comparisons with torch.inf
and 100.0
or torch.inf
as shown below. *complex
type cannot use >
, <
, >=
and <=
:
import torch
""" `float` type """
torch.inf == 100.0 # False
torch.inf == torch.inf # True
torch.inf == -torch.inf # False
torch.inf != 100.0 # True
torch.inf != torch.inf # False
torch.inf != -torch.inf # True
torch.inf > 100.0 # True
torch.inf > torch.inf # False
torch.inf > -torch.inf # True
torch.inf < 100.0 # False
torch.inf < torch.inf # False
torch.inf < -torch.inf # False
torch.inf >= 100.0 # True
torch.inf >= torch.inf # True
torch.inf >= -torch.inf # True
torch.inf <= 100.0 # False
torch.inf <= torch.inf # True
torch.inf <= -torch.inf # False
""" `complex` type """
complex(torch.inf, torch.inf) == (100.0+0.j) # False
complex(torch.inf, torch.inf) == complex(torch.inf, torch.inf) # True
complex(torch.inf, torch.inf) == complex(torch.inf, -torch.inf) # False
complex(torch.inf, torch.inf) == complex(-torch.inf, torch.inf) # False
complex(torch.inf, torch.inf) == complex(-torch.inf, -torch.inf) # False
complex(torch.inf, torch.inf) != (100.0+0.j) # True
complex(torch.inf, torch.inf) != complex(torch.inf, torch.inf) # False
complex(torch.inf, torch.inf) != complex(torch.inf, -torch.inf) # True
complex(torch.inf, torch.inf) != complex(-torch.inf, torch.inf) # True
complex(torch.inf, torch.inf) != complex(-torch.inf, -torch.inf) # True
Additionally, the comparisons with nan
and 100.0
, nan
or inf
using float() and complex() which are Python's built-in functions as shown below:
*Memos:
- The comparisons(except
!=
) withnan
are alwaysFalse
. -
complex
type cannot use>
,<
,>=
and<=
.
import torch
""" `float` type """
float('nan') == 100.0 # False
float('nan') == float('nan') # False
float('nan') == float('-nan') # False
float('nan') == float('inf') # False
float('nan') == float('-inf') # False
float('nan') != 100.0 # True
float('nan') != float('nan') # True
float('nan') != float('-nan') # True
float('nan') != float('inf') # True
float('nan') != float('-inf') # True
float('nan') > 100.0 # False
float('nan') > float('nan') # False
float('nan') > float('-nan') # False
float('nan') > float('inf') # False
float('nan') > float('-inf') # False
float('nan') < 100.0 # False
float('nan') < float('nan') # False
float('nan') < float('-nan') # False
float('nan') < float('inf') # False
float('nan') < float('-inf') # False
float('nan') >= 100.0 # False
float('nan') >= float('nan') # False
float('nan') >= float('-nan') # False
float('nan') >= float('inf') # False
float('nan') >= float('-inf') # False
float('nan') <= 100.0 # False
float('nan') <= float('nan') # False
float('nan') <= float('-nan') # False
float('nan') <= float('inf') # False
float('nan') <= float('-inf') # False
""" `complex` type """
complex('nan+nanj') == (100.0+0.j) # False
complex('nan+nanj') == complex('nan+nanj') # False
complex('nan+nanj') == complex('nan-nanj') # False
complex('nan+nanj') == complex('-nan+nanj') # False
complex('nan+nanj') == complex('-nan-nanj') # False
complex('nan+nanj') == complex('inf+infj') # False
complex('nan+nanj') == complex('inf-infj') # False
complex('nan+nanj') == complex('-inf+infj') # False
complex('nan+nanj') == complex('-inf-infj') # False
complex('nan+nanj') != (100.0+0.j) # True
complex('nan+nanj') != complex('nan+nanj') # True
complex('nan+nanj') != complex('nan-nanj') # True
complex('nan+nanj') != complex('-nan+nanj') # True
complex('nan+nanj') != complex('-nan-nanj') # True
complex('nan+nanj') != complex('inf+infj') # True
complex('nan+nanj') != complex('inf-infj') # True
complex('nan+nanj') != complex('-inf+infj') # True
complex('nan+nanj') != complex('-inf-infj') # True
Additionally, the comparisons with inf
and 100.0
or inf
using float() and complex() which are Python's built-in functions as shown below. *complex
type cannot use >
, <
, >=
and <=
:
import torch
""" `float` type """
float('inf') == 100.0 # False
float('inf') == float('inf') # True
float('inf') == float('-inf') # False
float('inf') != 100.0 # True
float('inf') != float('inf') # False
float('inf') != float('-inf') # True
float('inf') > 100.0 # True
float('inf') > float('inf') # False
float('inf') > float('-inf') # True
float('inf') < 100.0 # False
float('inf') < float('inf') # False
float('inf') < float('-inf') # False
float('inf') >= 100.0 # True
float('inf') >= float('inf') # True
float('inf') >= float('-inf') # True
float('inf') <= 100.0 # False
float('inf') <= float('inf') # True
float('inf') <= float('-inf') # False
""" `complex` type """
complex('inf+infj') == (100.0+0.j) # False
complex('inf+infj') == complex('inf+infj') # True
complex('inf+infj') == complex('inf-infj') # False
complex('inf+infj') == complex('-inf+infj') # False
complex('inf+infj') == complex('-inf-infj') # False
complex('inf+infj') != (100.0+0.j) # True
complex('inf+infj') != complex('inf+infj') # False
complex('inf+infj') != complex('inf-infj') # True
complex('inf+infj') != complex('-inf+infj') # True
complex('inf+infj') != complex('-inf-infj') # True
This content originally appeared on DEV Community and was authored by Super Kai (Kazuya Ito)
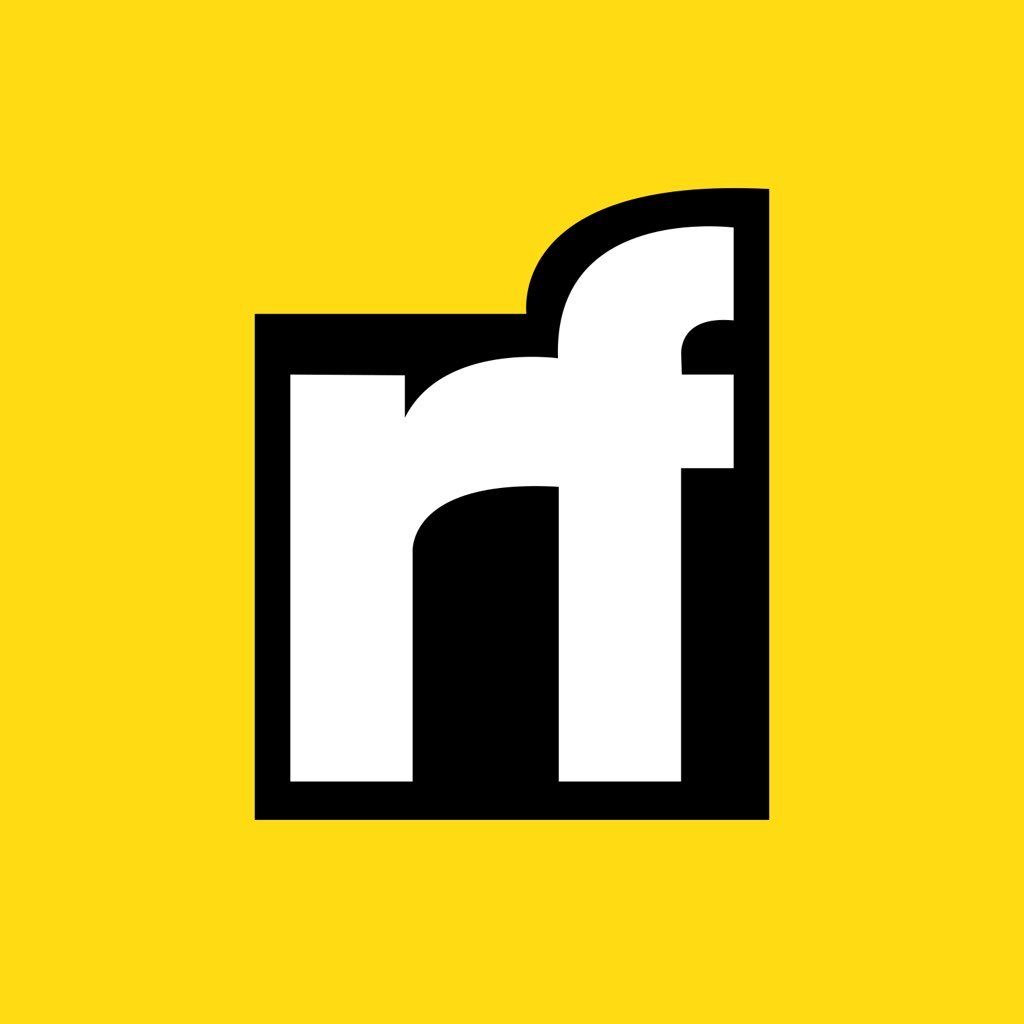
Super Kai (Kazuya Ito) | Sciencx (2024-07-22T05:17:30+00:00) The comparisons with `nan` and `inf` in PyTorch. Retrieved from https://www.scien.cx/2024/07/22/the-comparisons-with-nan-and-inf-in-pytorch/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.