This content originally appeared on Level Up Coding - Medium and was authored by Goldin Evgeny
Seamlessly Transition Your Web Development Skills to Build High-Performance Mobile Apps
Transitioning from web development to mobile development can seem daunting, especially if you’re coming from a ReactJS background. However, with React Native and Expo, this transition can be smooth, efficient, and, most importantly, fast. Let’s dive into how you can take your web skills and explore into the wild world of mobile development, and why it’s not as scary as it seems.
React Native: Your Old Friend in a New Costume
React Native is essentially React for mobile platforms. If you’re familiar with React, you’re already halfway there.
React Native lets you write most of your code in TypeScript (or JavaScript for the masochists out there), but instead of rendering to the DOM, after the reconciliation process, it renders to native components. That’s right, real native components on iOS and Android. No web wrappers or weird magic tricks — this is the real deal.
The Basics: Components and Rendering
Remember how you used to wrap your content in a <div>? Say hello to <View>. Miss your <p> tags? They’re now <Text>. And your trusty old <img> becomes <Image>. It’s like learning a new dialect of the same language.
Let’s break it down with some simple examples:
// ReactJS (Web)
export function MyWebComponent() {
return (
<div>
<p>Hello, web!</p>
<img src="path/to/image.jpg" alt="Example" />
</div>
);
}
// React Native (Mobile)
import { View, Text, Image } from 'react-native';
export function MyMobileComponent() {
return (
<View>
<Text>Hello, native!</Text>
<Image source={{ uri: 'https://example.com/image.jpg' }} />
</View>
);
}
You can see how similar the structure is. You’re still thinking in components, but you’re speaking the native language of mobile devices. And yes, your brain might do a double-take when you realize div is now View, but trust me, it’s all good!
The “It’s Really Native” Part
When I say native, I mean native. Your React Native code gets converted into platform-specific code for iOS and Android, using real native components under the hood. This means you’re getting performance and UI that feels right at home on any device. No janky webview wrappers here — this is the real deal. And there’s more to it. Mobile development has its quirks, like dealing with device-specific features, managing app states like a parent wrangling toddlers, and mastering the black art of build signing. We’ll get to those. And just before we continue to talk about the magic word: Expo, lets talk about how our components are rendered on mobile.
How Does It Work Under the Hood?
Here’s where it gets interesting: React Native leverages a JavaScript engine like JavaScriptCore on iOS and Hermes (or V8) on Android to execute your JavaScript code. These engines run your code in a separate thread, and then communicate with native components through a bridge.
This bridge is the magic that allows your JavaScript code to talk to native modules and components. It’s an asynchronous, bidirectional communication channel where serialized data is passed back and forth between the JavaScript code and the native environment. This means you’re not just building a mobile app with web technologies — you’re creating a truly native experience, optimized for performance and fully integrated with the underlying platform.
Expo: Your Trusty Sidekick
Imagine you’re Batman. Expo is your Robin, Alfred, and Batmobile all rolled into one. Expo takes away a lot of the heavy lifting that comes with mobile development — no need to mess with XCode or Android Studio for most of your development. It’s like someone handed you a mobile app sandbox and said, “Go have fun.”
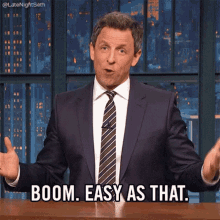
With Expo, you can start building a mobile app in minutes — literally. It gives you access to a abundance of APIs that you’ll need, like camera access, push notifications, and more. Plus, Expo Go lets you run your app on your device almost instantly, which is awesome and gives you immediate feedback.
Here’s how simple it is to get started:
npx create-expo-app MyFirstApp
cd MyFirstApp
npx expo start
But here’s where it gets really cool: When you’re ready to graduate from the sandbox to the real playground, Expo doesn’t just kick you out. Instead, it introduces you to Development Builds. Think of these as your personalized version of Expo Go, but with your choice of custom native modules. You get the best of both worlds: the ease of Expo with the flexibility of React Native.
Gotchas and Goodies: The Stuff You Need to Know
Let’s talk about some of the common “Oh, no!” moments you’ll likely encounter, and how to avoid them.
1. No More Map Madness
On the web, you might be used to rendering long lists with array.map(). But in the land of React Native, this could lead to performance issues. Instead, you’ll want to use FlatList or SectionList for those Instagram-worthy feeds. They’re optimized for mobile, ensuring you don’t send your users on a scroll lag adventure.
Example:
import { FlatList, Text, View } from 'react-native';
const data = [{ key: 'Devin' }, { key: 'Dan' }, { key: 'Dominic' }];
function MyListComponent() {
return (
<FlatList
data={data}
renderItem={({ item }) => <Text>{item.key}</Text>}
keyExtractor={(item) => item.key}
/>
);
}
FlatList handles rendering efficiently by only drawing what’s on screen, making your app buttery smooth.
2. Touchable, Pressable, and Everything in Between
React Native has its own way of handling buttons and touch events. The old faithful button won’t work. Enter TouchableOpacity and Pressable. These components give you control over how your elements respond to touch, ensuring your app feels smooth.
Example:
import { TouchableOpacity, Text } from 'react-native';
function MyButtonComponent() {
const handlePress = () => alert('Button pressed!');
return (
<TouchableOpacity onPress={handlePress} style={{ padding: 10, backgroundColor: 'blue' }}>
<Text style={{ color: 'white' }}>Press Me</Text>
</TouchableOpacity>
);
}
TouchableOpacity gives you that subtle feedback when a button is pressed by reducing the opacity of the wrapped element, providing a visual feedback and making your app feel more interactive. For more control or different feedback styles, consider using Pressable or TouchableHighlight, depending on the interaction you’re designing.
3. Styling Like a Pro
Styling in React Native isn’t just a copy-paste from your CSS files. It’s inline, and you’ll need to get cozy with Flexbox. But there’s a twist — everything defaults to flexDirection: column. If you’ve been laying out websites like a pro, get ready for a slight brain rewiring.
Example:
import { View, Text, StyleSheet } from 'react-native';
function MyStyledComponent() {
return (
<View style={styles.container}>
<Text style={styles.header}>Hello, Styled World!</Text>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#f0f0f0',
},
header: {
fontSize: 24,
color: '#333',
},
});
No more global CSS classes — styles are scoped and defined within the component, making them easier to manage and maintain.
4. Conditional Rendering Can Be Tricky
React developers love using short-circuit evaluation for conditional rendering. You know, the classic {condition && <Component />} pattern. But when you bring this habit into React Native, things can get a little problematic.
Here’s how different values behave:
export const Example = () => {
return (
<>
{0 && <Something />}
{/* React: renders 0 */}
{/* React Native: crashes */}
{'' && <Something />}
{/* React: renders nothing */}
{/* React Native: crashes */}
{NaN && <Something />}
{/* React: renders NaN */}
{/* React Native: crashes */}
{false && <Something />}
{/* React: renders nothing */}
{/* React Native: renders nothing */}
{null && <Something />}
{/* React: renders nothing */}
{/* React Native: renders nothing */}
{undefined && <Something />}
{/* React: renders nothing */}
{/* React Native: renders nothing */}
</>
);
};
The takeaway? Stick to using conditional ternaries (condition ? <Component /> : null) in React Native. It’ll save you from unexpected crashes and endless debugging sessions.
The Dreaded Deployment (But Not Really)
Deploying a web app is easy — you just push to your server, and voilà, the whole world gets the update. Native apps? Not so fast, cowboy.
Deploying to the App Store or Google Play is a different beast. You’ll need signing credentials, provisioning profiles, and a deep breath before you hit that upload button. But here’s the good news: Expo’s EAS (Expo Application Services) has your back. It handles the heavy lifting, so you can focus on what you do best — coding.
Here’s the magic command:
eas build --platform ios
Before running `eas build — platform ios`, ensure you’ve set up Expo Application Services (EAS) properly. EAS streamlines the build process by handling tasks like generating signing credentials and uploading your app to the store, all from the command line.
Expo takes care of the rest, including generating the signing credentials, provisioning profiles, and even uploading your app to the store. It’s like having a deployment wizard on your team.
Final Thoughts: Welcome to the Mobile Party 🎉
If you’ve made it this far, you’re not just a React developer — you’re an adventurer, ready to learn. So what are you waiting for? Fire up that terminal, npx create-expo-app, and start building something awesome.
From Web to Mobile: Fast-Track Your Journey with React Native and Expo was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Goldin Evgeny
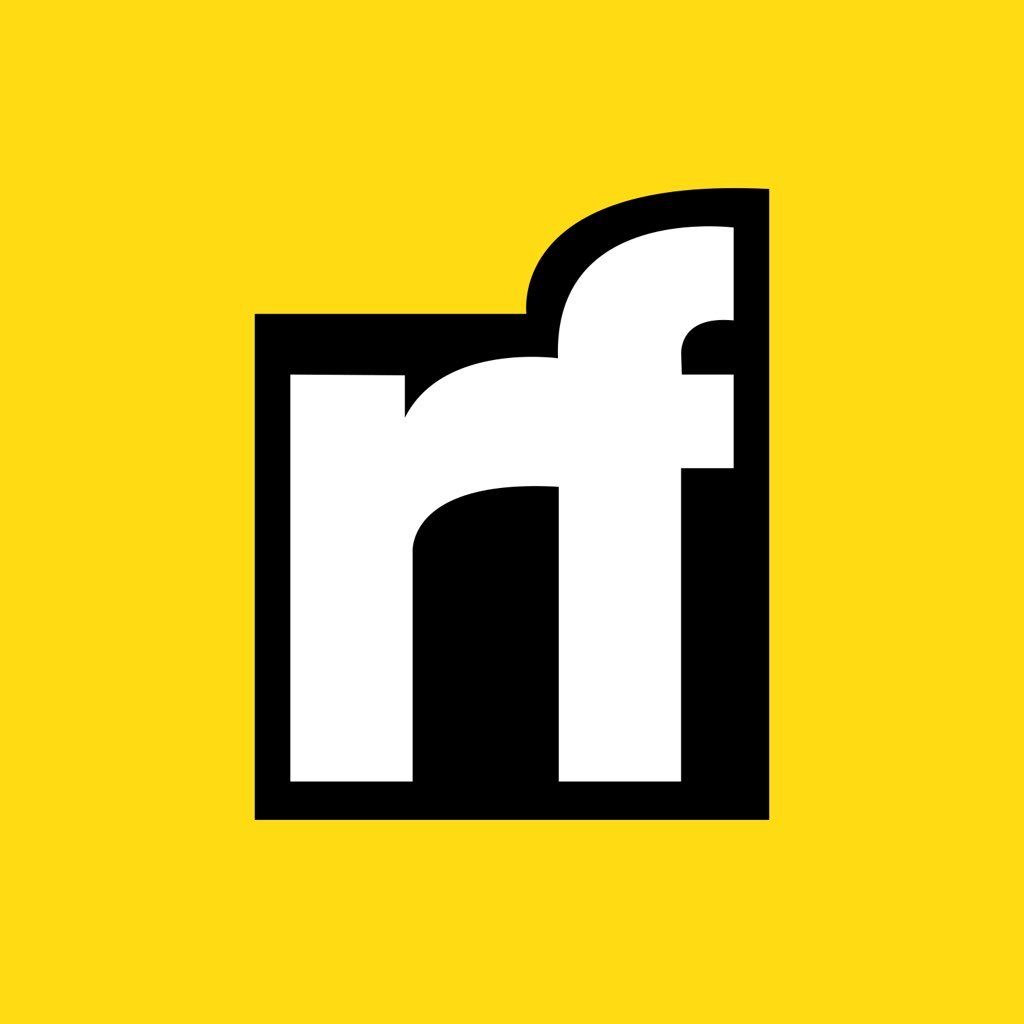
Goldin Evgeny | Sciencx (2024-08-06T22:22:29+00:00) From Web to Mobile: Fast-Track Your Journey with React Native and Expo. Retrieved from https://www.scien.cx/2024/08/06/from-web-to-mobile-fast-track-your-journey-with-react-native-and-expo/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.