This content originally appeared on DEV Community and was authored by Noah
The basic of readonly property
In Type Script, you can make the object of the properties of an object read-only.
const person: { readonly name: string } = { name: 'Mike' }
person.name = 21;
// → Cannot assign to 'name' because it is a read-only property.
⚠️① readonly is only at compile-time
In the compiled JavaScript code, the readonly declaration is removed, so it will not be detected as an error at runtime.
⚠️② readonly is not recursive.
const person: {
readonly name: string;
readonly academicBackground: {
primarySchool: string
}
} = {
name: 'Mike',
academicBackground: {
primarySchool: 'School A'
}
}
person.academicBackground.primarySchool = 'School B'
// You can change `person.academicBackground.primarySchool`
If you want to make it read-only, also you need to put readonly to primarySchool
.
const person: {
readonly name: string;
readonly academicBackground: {
readonly primarySchool: string
}
} = {
name: 'Mike',
academicBackground: {
primarySchool: 'School A'
}
}
person.academicBackground.primarySchool = 'School B'
// → Cannot assign to 'primarySchool' because it is a read-only property.
Readonly
When the number of properties increases, adding readonly to each one becomes cumbersome and increases the amount of code.
You can refactor by using Readonly.
const obj: {
readonly a : string;
readonly b: string;
readonly c: string;
readonly d: string;
} = {
a: 'a',
b: 'b',
c: 'c',
d: 'd'
}
// ↓
const obj: Readonly<{
a : string;
b: string;
c: string;
d: string;
}> = {
a: 'a',
b: 'b',
c: 'c',
d: 'd'
}
Happy Coding☀️
This content originally appeared on DEV Community and was authored by Noah
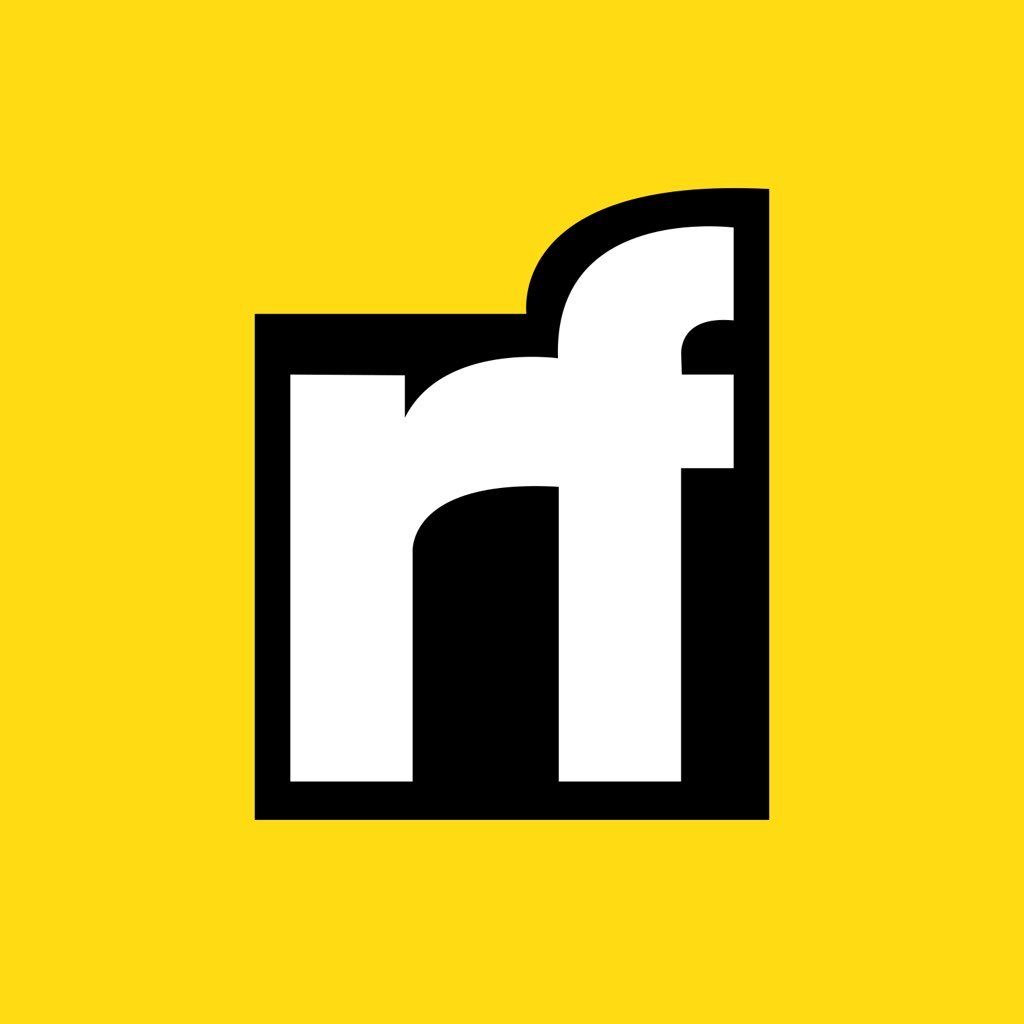
Noah | Sciencx (2024-08-10T12:56:16+00:00) Cautions When Using readonly in TypeScript. Retrieved from https://www.scien.cx/2024/08/10/cautions-when-using-readonly-in-typescript/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.