This content originally appeared on Telerik Blogs and was authored by Hassan Djirdeh
The use API in React 19 allows for conditional and loop-based access to context values, offering enhanced flexibility and potential performance optimizations.
Context in React is a powerful tool that allows us to share data between components without having to pass props down every level of a component tree. We’ve written content before on how and when to use React Context and the React useContext hook. In this article, we’ll briefly explore what React Context is and how it works with the new use API now available in React.
React Context
When managing data between parent and child components, React gives us the ability to use something known as props to pass data down from parent to child. Props can only flow in one direction, from parent components to child components (and further down). When state changes occur on parent elements, React will re-render components that depend on those values.
Context in React is a way to pass data down through a component tree without having to pass props down through every level. This can be very helpful since this allows us to share data between components that are not directly related to each other (e.g., between sibling components).
React Context works by creating a context object that holds the data we want to share between components. We create this context object with the createContext() function:
import { createContext } from "react";
const MyContext = createContext();
Once we’ve created the context object, we can use it in our components by first wrapping the components in a Context provider:
<MyContext value={/* some value */}>
{/* Your components */}
</MyContext>
In React 19, we’re able to render
<MyContext>
as a provider instead of<MyContext.Provider>
. See React Documentation | Context as a provider.
The New Use API
Previously, we’ve had to rely primarily on the useContext hook to access context values within components. React 19 introduces a versatile addition to our toolkit: the use function, which enhances how values, such as those from contexts or promises, are read within a component.
Any component that is a child of the Context Provider can then access the data in the context using the use()
function:
import { useContext } from 'react';
import MyContext from './MyContext';
function MyComponent() {
const value = use(MyContext);
// ...
}
Compared to the traditional useContext()
hook, the use()
function stands out by offering the flexibility to be called within conditionals and loops. This is a significant improvement as it allows developers to optimize performance and resource utilization by conditionally accessing contexts based on the component’s state or props.
Here’s an example of using the use()
function within a conditional:
import { use } from 'react';
import FeatureContext from './FeatureContext';
function FeatureComponent({ featureName }) {
let featureEnabled = false;
if (featureName) {
featureEnabled = use(FeatureContext);
}
return featureEnabled ? <div>Feature Enabled!</div> : <div>Feature Disabled.</div>;
}
In the above example, we have a feature toggle context that determines whether certain features should be enabled in our application. We can use the use()
function to conditionally access the relevant context only when needed, thus potentially reducing unnecessary re-renders.
Here’s an example of using the use()
function within a loop:
import { use } from 'react';
import SettingsContext from './SettingsContext';
function SettingsDisplay({ settingKeys }) {
const settingElements = settingKeys.map(key => {
const settings = use(SettingsContext);
return <div key={key}>{key}: {settings[key]}</div>;
});
return <div>{settingElements}</div>;
}
In the above example, each call to use(SettingsContext)
within the loop demonstrates the new flexibility of the hypothetical use()
function, allowing each iteration to access the context independently.
Similar to useContext
, the use(context)
function consistently searches for the nearest context provider located above the calling component. It scans upward through the component tree, ignoring any context providers within the component from which use(context)
is invoked.
import { use, createContext } from 'react';
const ThemeContext = createContext('light');
function ThemedComponent() {
// This will use the value from the nearest ThemeContext.Provider
// above this component in the tree, not the one defined below
const theme = use(ThemeContext);
return (
<div>
<p>Current theme: {theme}</p>
{/* This Provider is ignored by the use(ThemeContext) call above */}
<ThemeContext value="dark">
<NestedComponent />
</ThemeContext>
</div>
);
}
function NestedComponent() {
// This will use the "dark" theme from the Provider in ThemedComponent
const theme = use(ThemeContext);
return <p>Nested component theme: {theme}</p>;
}
function App() {
return (
<ThemeContext value="light">
<ThemedComponent />
</ThemeContext>
);
}
Wrap-up
The introduction of the use API in React 19 represents a significant advancement in how we interact with context and other resources in React applications. This new approach allows for conditional and loop-based access to context values, offering us enhanced flexibility and potential performance optimizations.
In an upcoming article, we’ll investigate how the use API can also be used to read promise-based data from server to client in a React Server Components setting. For more additional reading on the use API, be sure to check out the official React documentation.
This content originally appeared on Telerik Blogs and was authored by Hassan Djirdeh
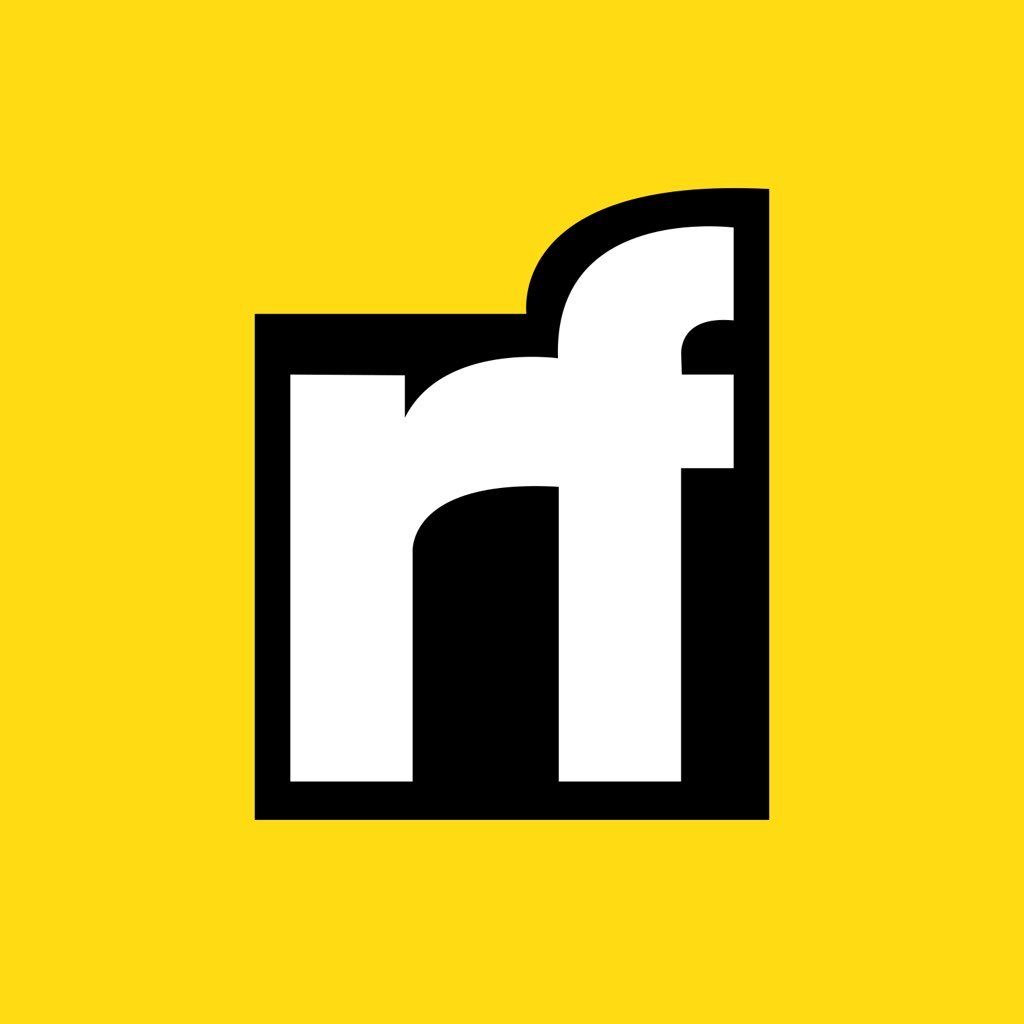
Hassan Djirdeh | Sciencx (2024-08-19T08:29:20+00:00) React Context with the New Use API. Retrieved from https://www.scien.cx/2024/08/19/react-context-with-the-new-use-api/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.