This content originally appeared on DEV Community and was authored by Mohammed Dawood
As React developers, we often face the challenge of maintaining a clean and scalable codebase. The SOLID principles—five foundational guidelines in object-oriented programming—can help us create React applications that are not only efficient but also easy to maintain. In this article, we'll explore how to implement each SOLID principle within a React context, ensuring your codebase stays robust and flexible.
1. Single Responsibility Principle (SRP)
The Single Responsibility Principle states that a class or module should have only one reason to change. In React, this means each component should have a single responsibility, separating concerns between UI rendering and logic.
- Example:
const useFetchData = (url: string) => {
const [data, setData] = useState(null);
useEffect(() => {
fetch(url)
.then((response) => response.json())
.then(setData);
}, [url]);
return data;
};
const DataDisplay: React.FC<{ url: string }> = ({ url }) => {
const data = useFetchData(url);
return <div>{data ? JSON.stringify(data) : 'Loading...'}</div>;
};
In this example, the data fetching logic is separated into a custom hook (useFetchData
), allowing the DataDisplay
component to focus solely on rendering the UI.
2. Open/Closed Principle (OCP)
The Open/Closed Principle states that software entities should be open for extension but closed for modification. In React, this can be achieved by designing components that can be extended via props or composition rather than modifying the existing code.
- Example:
const Button: React.FC<ButtonProps> = ({ variant, ...props }) => (
<button className={`btn btn-${variant}`} {...props} />
);
const IconButton: React.FC<IconButtonProps> = ({ icon, ...props }) => (
<Button {...props}>
<i className={`icon-${icon}`} />
</Button>
);
Here, the Button
component is open for extension (e.g., by adding an icon) without modifying its core structure, demonstrating the Open/Closed Principle.
3. Liskov Substitution Principle (LSP)
The Liskov Substitution Principle asserts that objects of a superclass should be replaceable with objects of a subclass without affecting the program's correctness. In React, this means ensuring extended components can replace base components without breaking the app.
- Example:
interface ShapeProps {
color: string;
}
const Shape: React.FC<ShapeProps> = ({ color }) => (
<div style={{ backgroundColor: color }} />
);
const Circle: React.FC<ShapeProps> = (props) => (
<Shape {...props} style={{ borderRadius: '50%' }} />
);
const Square: React.FC<ShapeProps> = (props) => (
<Shape {...props} style={{ width: '100px', height: '100px' }} />
);
In this example, both Circle
and Square
components can replace the Shape
component without altering the application's behavior.
4. Interface Segregation Principle (ISP)
The Interface Segregation Principle suggests that no client should be forced to depend on methods it does not use. In React, this principle can be applied by creating smaller, more focused interfaces or prop types that are specific to the needs of a component.
- Example:
interface CommonProps {
id: string;
}
interface ButtonProps extends CommonProps {
onClick: () => void;
}
interface LinkProps extends CommonProps {
href: string;
}
const ActionButton: React.FC<ButtonProps> = ({ id, onClick }) => (
<button id={id} onClick={onClick}>
Click Me
</button>
);
const NavigationLink: React.FC<LinkProps> = ({ id, href }) => (
<a id={id} href={href}>
Go to Link
</a>
);
Here, ButtonProps
and LinkProps
interfaces are segregated based on the specific needs of the ActionButton
and NavigationLink
components, following the ISP.
5. Dependency Inversion Principle (DIP)
The Dependency Inversion Principle states that high-level modules should not depend on low-level modules; both should depend on abstractions. In React, this principle can be implemented using dependency injection and context to decouple components from their dependencies.
- Example:
const ApiServiceContext = React.createContext<ApiService | null>(null);
const App: React.FC = () => {
const apiService = new ApiService();
return (
<ApiServiceContext.Provider value={apiService}>
<MyComponent />
</ApiServiceContext.Provider>
);
};
const MyComponent: React.FC = () => {
const apiService = useContext(ApiServiceContext);
useEffect(() => {
apiService?.fetchData().then(console.log);
}, [apiService]);
return <div>Check the console for data.</div>;
};
In this example, MyComponent
depends on the ApiService
through context, following the Dependency Inversion Principle by relying on abstractions.
Conclusion:
By applying the SOLID principles in your React applications, you can ensure that your code is not only easy to understand but also flexible and scalable. These principles help in creating a robust architecture that can adapt to changing requirements without compromising on quality or performance.
This content originally appeared on DEV Community and was authored by Mohammed Dawood
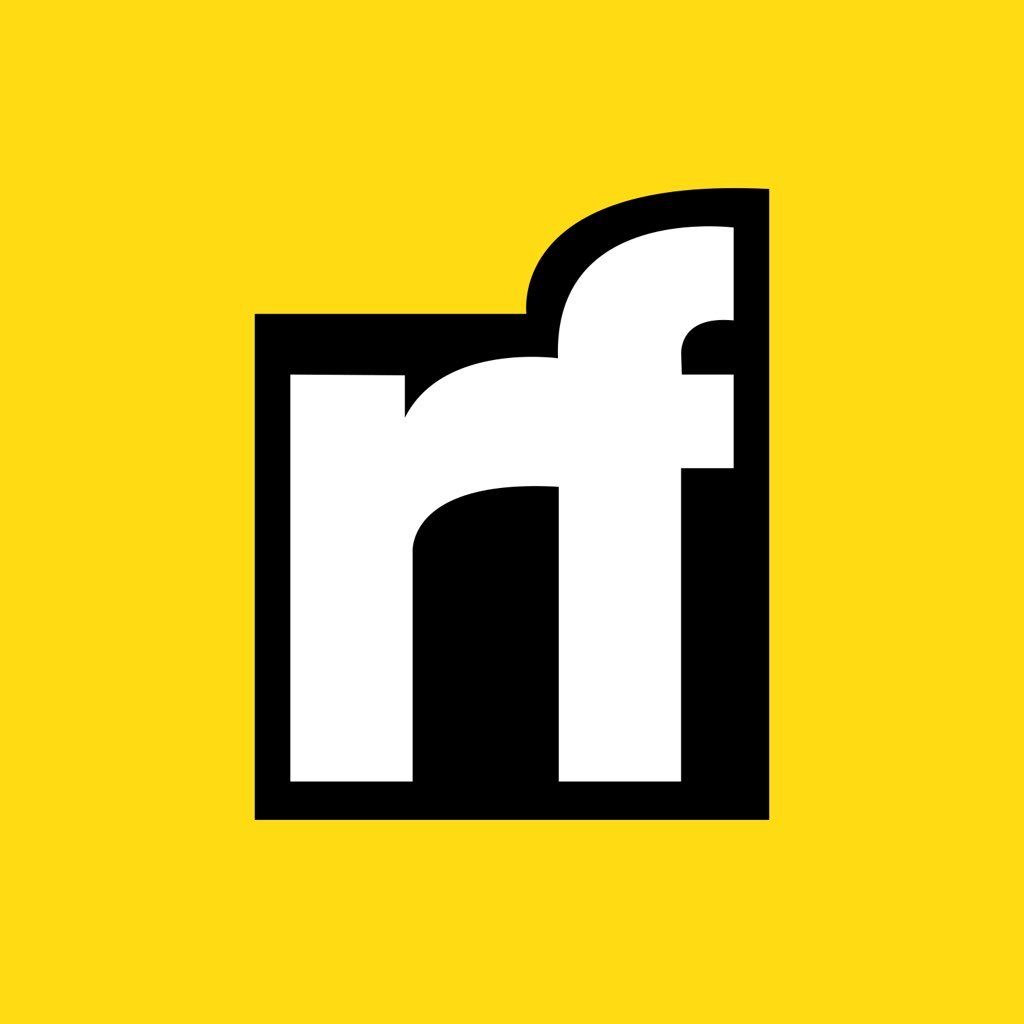
Mohammed Dawood | Sciencx (2024-08-26T21:26:51+00:00) Implementing SOLID Principles in React: A Guide for Scalable Development. Retrieved from https://www.scien.cx/2024/08/26/implementing-solid-principles-in-react-a-guide-for-scalable-development/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.