This content originally appeared on Level Up Coding - Medium and was authored by Evgeni Gomziakov
Simple tips that improved my coding experience
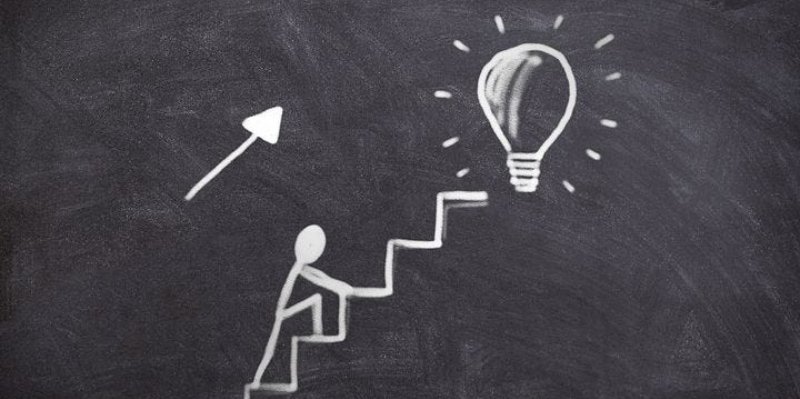
1. Keep it Simple
In my coding journey, I’ve found that simplicity often leads to better results. If I’m spending too much time trying to figure out a piece of code, it’s usually a sign that I need to simplify. Here’s how I approach it:
- Break Down Problems: try to divide complex problems into smaller, more manageable pieces.
- Avoid Over-Engineering: focus on what’s needed now rather than adding complexity for future “what ifs.”
- Refactor Regularly: keep an eye out for opportunities to simplify my code, even after it’s working.
I’ve often started with something overly complex for a basic task, like this:
type Numeric = number | string;
interface AddOptions {
checkTypes?: boolean;
round?: boolean;
precision?: number;
}
function addComplex<T extends Numeric, U extends Numeric>(a: T, b: U, options: AddOptions = {}): number | string {
if (options.checkTypes) {
const isANumeric = typeof a === 'number' || (typeof a === 'string' && !isNaN(Number(a)));
const isBNumeric = typeof b === 'number' || (typeof b === 'string' && !isNaN(Number(b)));
if (!isANumeric || !isBNumeric) {
throw new Error('Invalid type: only numeric values or numeric strings are allowed');
}
}
let result = Number(a) + Number(b);
if (options.round) {
result = Math.round(result);
} else if (typeof options.precision === 'number') {
result = parseFloat(result.toFixed(options.precision));
}
return result;
}
But often, a simple version is all that’s needed:
const addSimple = (a,b) => (a + b)
Starting simple and only adding complexity when absolutely necessary has saved me a lot of headaches.
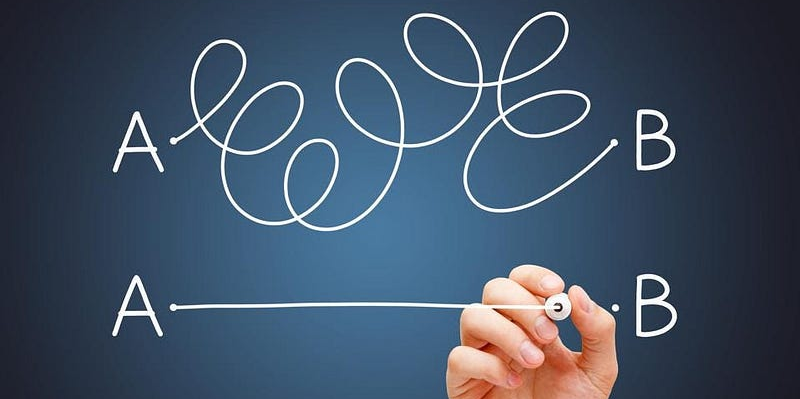
2. Keep Up with Tech Stuff
One habit that’s really helped me grow is setting aside a little time each week to learn something new. Whether it’s a new framework, a coding challenge, or just following a tech blog, it all adds up over time.
Here are a few ways I try to stay in the loop:
- Try Coding Challenges
- Follow Tech Blogs and Podcasts
- Join Developer Communities
- Experiment with New Tools
If you’re looking for places to start, here are some of my go-tos:
- Fireship — quick tutorials and insights into the latest technologies
- Dev.to — community where developers share knowledge
- Trending GitHub Repositories — great way to keep an eye on what’s new
- Daily.dev — extension that turns new tabs into a collection of tech news
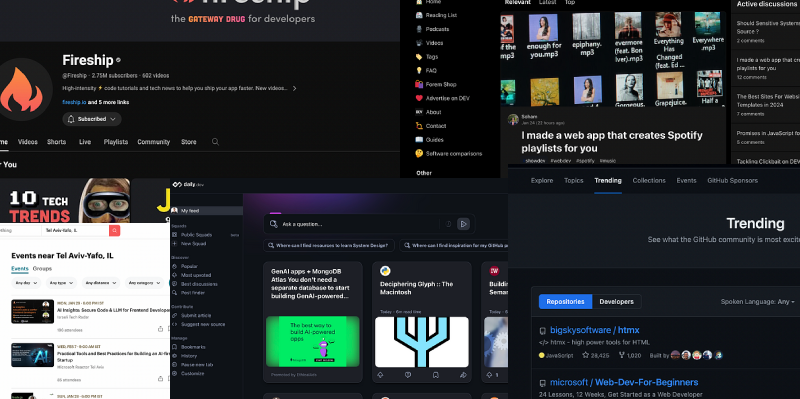
3. Document Your Learnings
One of the most effective ways I’ve found to solidify what I learn is by documenting it. Writing things down not only helps me understand concepts better but also creates a resource that I can refer back to and share with others.
Keep a Dev Journal — I try to sum up key takeaways from what I learn each day in Notion. It could be a new concept, a tricky bug I solved, or just reflections on my progress.
Create Tutorials or Guides — Sometimes, I find that the best way to fully grasp a concept is by teaching it to others. Creating tutorials forces me to break down complex ideas into simple, understandable steps.
4. Focus on Code Quality
It’s easy to rush through coding tasks, but I’ve learned that taking the time to focus on code quality can save a lot of trouble down the line.
Adopt Best Practices — I regularly check whether my code adheres to established best practices, such as Clean Code, SOLID principles, DRY (Don’t Repeat Yourself), and proper error handling.
Code Reviews — I learn a lot by reviewing others’ code and getting feedback on mine. It’s a two-way street that benefits everyone.
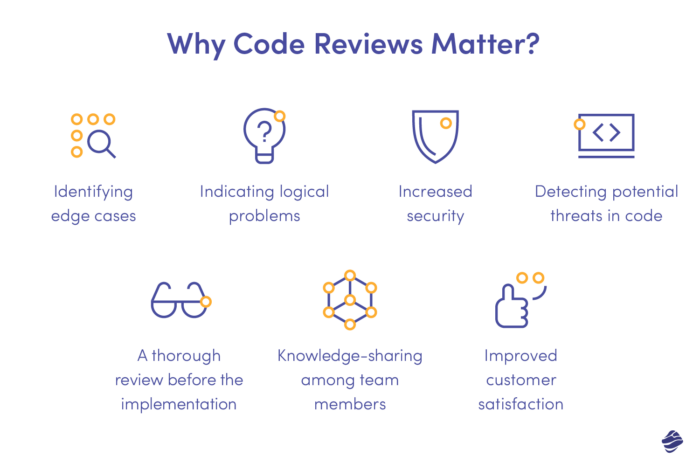
5. Prioritize Mental and Physical Health
I’ve found that taking care of my mental and physical well-being is crucial to my performance and creativity.
Take Regular Breaks — I make it a point to step away from the screen every now and then. Whether it’s a short walk or just a moment to stretch, breaks help me stay focused and reduce burnout.
Stay Active — Regular exercise keeps energy levels up and helps maintain a positive mindset, which is essential for tackling challenging coding problems.
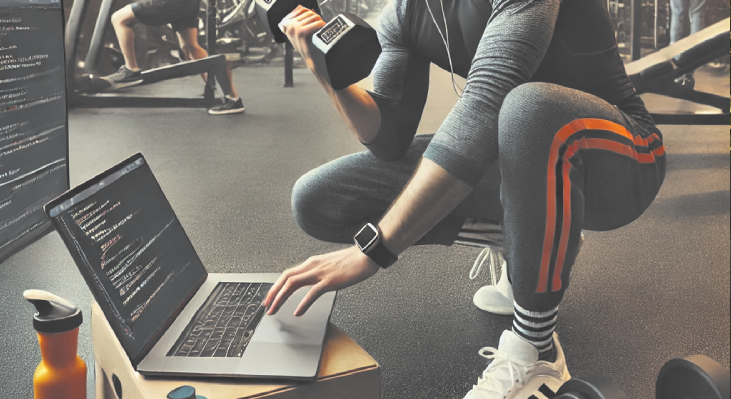
Thanks for reading! I hope you found these tips useful. Feel free to share your thoughts or pass this article along to others who might find it helpful. Happy coding!
Practical Habits for Better Coding was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Evgeni Gomziakov
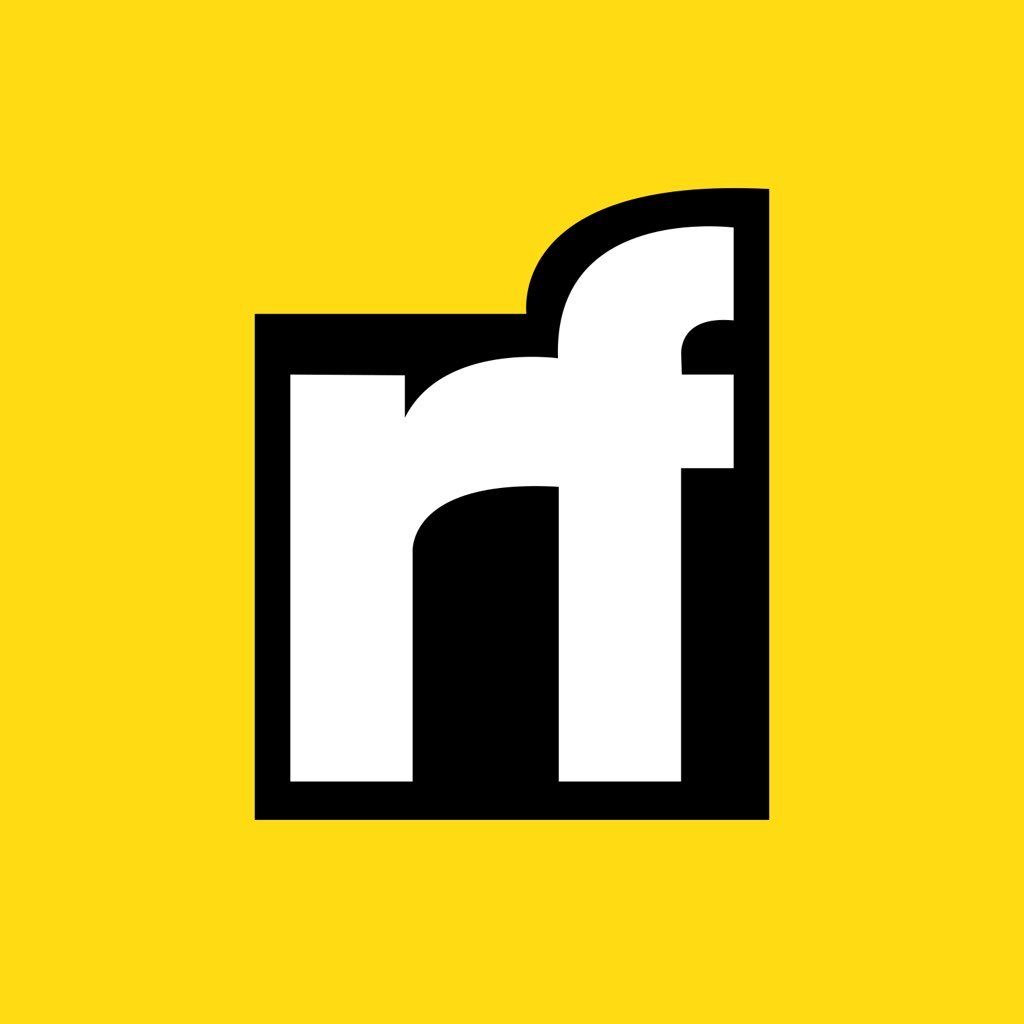
Evgeni Gomziakov | Sciencx (2024-08-27T11:22:04+00:00) Practical Habits for Better Coding. Retrieved from https://www.scien.cx/2024/08/27/practical-habits-for-better-coding/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.