This content originally appeared on DEV Community and was authored by Jamilah Foucher
Manipulating 2d, 3d, or 4d arrays in JavaScript are necessary for AI model training and image/audio/video analysis.
Below are a few useful functions for array manipulation using pure JavaScript without matrix packages like Tensorflow.js.
Transpose of a 2d array
function transpose_2d_array(arr) {
var transpose_colNum = arr.length;
var transpose_rowNum = arr.at(0).length;
var transpose_arr = [];
for (var i=0; i<transpose_rowNum ; i++) {
const col = Array.from({ length: transpose_colNum }, (_, i) => 0);
transpose_arr.push(col);
}
for (var i=0; i<arr.length; i++) {
for (var j=0; j<arr.at(i).length; j++) {
transpose_arr[j][i] = arr[i][j];
}
}
return transpose_arr;
}
Print the Shape of an array
async function recur_func(arr) {
if (arr != undefined) {
return [arr[0], arr.length];
} else {
return arr;
}
}
async function shape(arr) {
var out = await recur_func(arr);
var shap = [out[1]];
var c = 0; // typically work with 4D arrays or less
while (out != undefined && c < 4) {
out = await recur_func(out[0]);
if (out != undefined) {
shap.push(out[1]);
}
c = c + 1;
}
return shap.slice(0, shap.length-1);
}
I hope these array utility functions will be helpful to someone.
Happy practicing! 👋
This content originally appeared on DEV Community and was authored by Jamilah Foucher
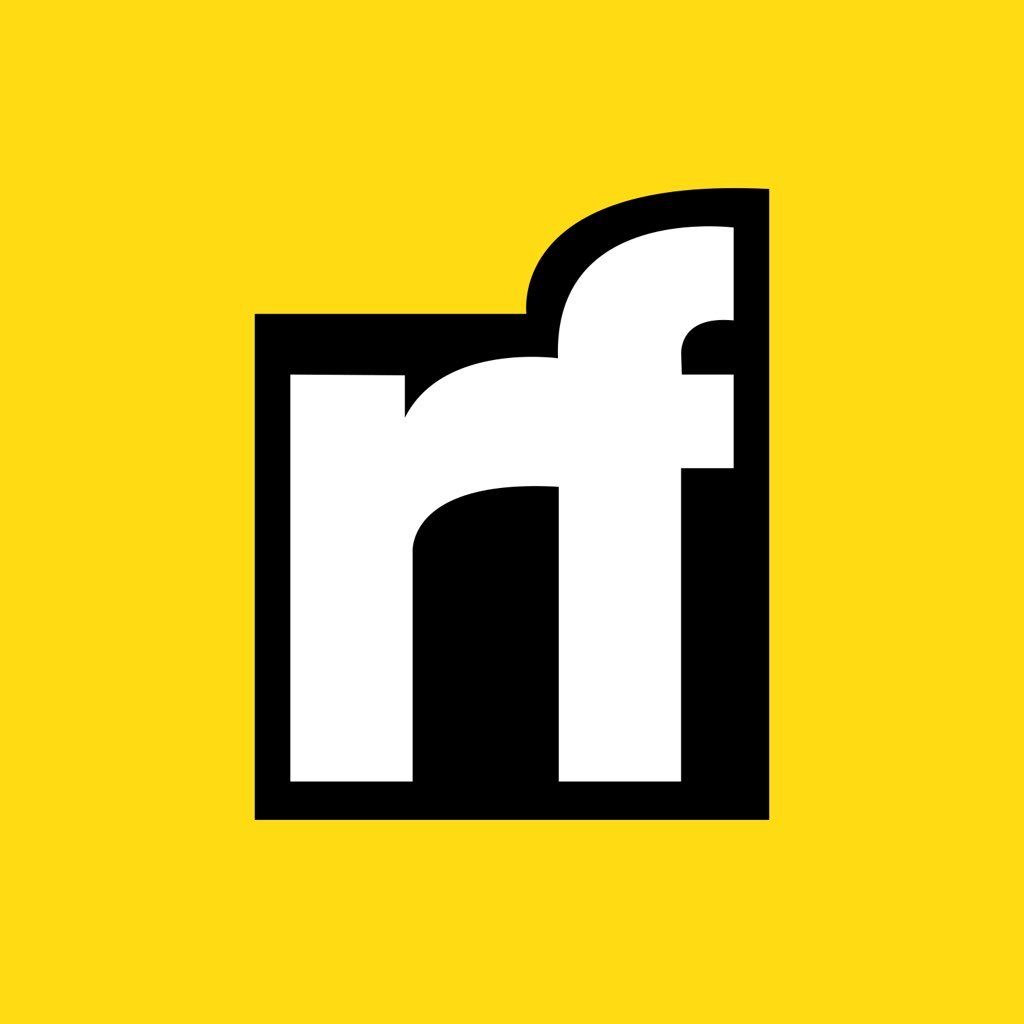
Jamilah Foucher | Sciencx (2024-08-31T14:05:52+00:00) JavaScript Array Manipulations. Retrieved from https://www.scien.cx/2024/08/31/javascript-array-manipulations/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.