This content originally appeared on Level Up Coding - Medium and was authored by Besarion Turmanauli
This is the third article in a series of tutorials: Build AI for generating quant trading strategies automatically, for the previous article, please, check this link.
Where are we exactly? Well, we’ve built a basic MetaTrader 5 robot, refined it, and made it more robust following the best practices, which means, in short, that the robot shall perform reasonably well in real market conditions with the least amount of surprises we don’t want.
There are additional data points we can use to improve the logic further but this is not the purpose of this article, that’s what AI shall do, therefore we’ll build an AI which at a minimum will replicate everything we did in the second article, and that will give us opportunities to make the AI core robust enough for it to employ additional data points automatically and generate better automated trading systems than we did.
The next step is to create a Tester class in C#, the tester will be able to take all the data points in, including the trading logic, replicate MetaTrader 5 strategy tester processes and output the results. The goal is for our Tester class to output exactly the same results as MetaTrader 5 strategy tester.
Why do we need this? because AI shall be able to test and confirm the profitability of the trading logic it generates and make relevant decisions of its own, the final product shall already be tested and verified.
In general, I can realistically divide the whole journey into the following levels of complexity:
- Testing of the automated trading strategy — manual testing we performed in the second article with predefined input values;
- Optimization — automatically generating different input values and testing them all, in order to select the best, more profitable combination, which we also did, but manually.
- Genetic optimization — genetic optimization algorithms do not test all possible combinations but only the most promising ones based on previous tests, it’s mainly used to save time on unnecessary input combinations particularly when there are a lot of them.
- Self-optimization — self-optimization algorithms are usually embedded in robots themselves, they run optimizations of their own, selecting the best settings automatically to adapt to changing market conditions.
- Automated strategy building— a mix of strategy coding from scratch and optimization, in this case, we improve not only the input values but the core logic itself, it’s a form of source code generating AI adapted for a basic amount of specific tasks that are needed for building, optimizing and verifying the strategy from the ground up.
- Quant AI — while an automated strategy builder algorithm is able to generate a more robust strategy source code than a human can, in a fraction of a time (5 minutes vs weeks of manual research, coding, testing, and optimization), pure machine learning applied to quantitative research is whole another beast. To give you a better perspective, all the items in this list have fewer and fewer limitations of what they’re able to accomplish, this item has none.
If I’m not wildly overexaggerating anything and with the knowledge and skills, you’ll acquire in this series of tutorials you’ll be able to make more money than any university graduate can make in his or her career on average (I haven’t made such a promise yet and I’m still not making any but that’s kind of what I’m trying to do), why am I spending my time to teach you all of this for free? After all, you’ve probably heard the saying:
Those who can, do; those who can’t, teach.
Here’s my version:
Those who can, do; those who can’t, teach; those who teach, can.
Anyone can do something, that’s not really a problem, the question is what you can’t do YET, the best way to master an area of competence is to learn, apply, and explain, this is how our brains work best.
Imagine you teach others the very best skills and knowledge you’ve got so far, whatever that is, something you’re really really good at, you provide the best possible value to society, your city, your country, the whole world, and your brain becomes exponentially more competent.
As long as you keep learning new things, applying that knowledge, and teaching… well, you get the idea!
The deal is, research shows that people are usually more motivated to learn and apply the knowledge if they pay money for that education…
Sorry.
Back to our Tester class. Create a new project in Visual Studio or an IDE of your choice and create a new class called Tester.cs.
We’ll need the following properties:
- totalPips (double)
- profitFactor (double)
- totalTrade (int)
- buyLogic (string)
- sellLogic (string)
- slDistance (double)
- tpDistance (double)
- buyOpenPrices (List<double>)
- buyStopPrices (List<double>)
- buyLimitPrices (List<double>)
- sellOpenPrices (List<double>)
- sellStopPrices (List<double>)
- sellLimitPrices (List<double>)
Here’s the result:
buyLogic and sellLogic are JSON strings which will be recognized by the tester as a trading logic, kind of executing a source code as a binary code in a compiled program, as long as we can modify the value of these properties, we can basically modify the running (already compiled) program without having to change the source code and recompile it, on-the-fly, that’s not something that has never been done before but still really cool!
Next, we need basic functions to assemble MetaTrader 5 strategy tester logic, like checking if Stop Loss or Take Profit have been hit:
These functions are very basic but reliable: they take a specific price from historical price data as an argument, and if that price is above/below any stop loss or take profit, relevant changes are made to the list of open trades.
If we want to make them more realistic, they all have to take a second argument: spread — the difference between Ask and Bid prices, basically how it works is when stop loss is triggered, you lose stop loss amount + spread and when take profit is triggered, you gain take profit amount, but the price has to reach take profit + spread, in other words, spread always works against you.
MetaTrader 5 gives us the ability to perform tests on real historical spreads for higher accuracy. When we export OHLC (Open, High, Low, Close) price data, it also exports real spreads (among others), we’re going to use this to our advantage, in the end, for now, let’s keep moving…
In the beginning, we created buyLogic and sellLogic properties, which store buy/sell trading logics (criteria for opening buy/sell trades) as JSON strings, here’s how they work:
We start with an AND property, the value of which is an array listing the criteria, all criteria listed in this [AND] array should be met for a buy/sell position to open, some items, however, aren’t just strings, they’re yet other objects with an OR property, the value of which is also an array, listing the criteria. At least one of these [OR] criteria should be met for a position to open.
To recap, the elementary parts of logic are stored as strings, they could be part of an AND or OR array, and all or one of the array items should be satisfied respectively in order for a position to open.
For more information on how to work with these kinds of JSON strings, please check the articles listed below:
- A complete guide for deserializing JSON to dynamic objects On The Fly in C#
- Iterating through a dynamic object in C#
Once we retrieve the basic string logic, we need to distinguish and process its components: comparison operators (>, <, ≥, ≤, ==, !=), comparison sides, math operators (+, -, /, *) and its sides:
These functions take raw strings as well as operator variables as a reference, they additionally output an array of sides with 2 items inside, here’s a function that wraps the whole thing up:
PriceEngine.priceData is a property of PriceEngine class storing all the price data imported from MetaTrader 5 in a DataTable format, looks like this:

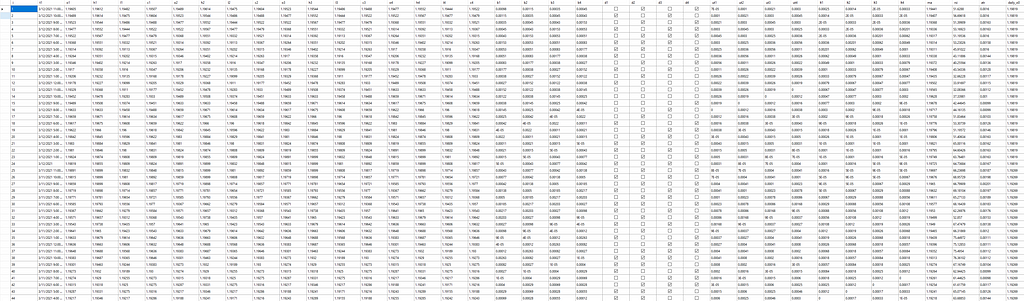
The idea here is to convert (deserialize) JSON strings into objects using Newtonsoft.json NuGet package, iterate through it, and output a final true or false boolean value for opening or not opening a position respectively.
The principle behind this is simple: buyLogic and sellLogic JSON strings basically contain a source code, which we should be able to modify, add new parts, or remove without having to change the actual source code and recompile the program. As we can’t modify the source code of an already compiled and launched program, we do this instead.
We use a couple of functions in order to iterate through the object we generated from those buyLogic and sellLogic JSON strings:
Finally, here’s the function that wraps everything up:
Strategy builder will use the test function multiple times, therefore we need to reset some of the properties to their initial values:
Source code of a complete Tester class (everything is 100% verified and working):
RISK DISCLAIMER:
For the purposes of this tutorial, I’m not a qualified licensed investment advisor, nor do I provide personal investment advice, all information provided in this article is for informational and educational purposes only.
Conduct your own due diligence and consult a licensed financial advisor before making any and all investment decisions. Any investments, trades, speculations, or decisions made on the basis of any information found in this article, expressed or implied herein, are committed at your own risk, financial or otherwise.
Trading (both manual and algorithmic) involves substantial risk of loss and is not suitable for everyone. The valuation of financial securities may fluctuate, and, as a result, you may lose more than you original investment. The impact of seasonal and geopolitical events is already factored into market prices.
Leveraged trading means that small market movements will have a great impact on your trading account and this can work against you, leading to large losses or can work for you, leading to large gains.
If the market moves against you, you may sustain a total loss greater than the amount you deposited into your account.
If you enjoyed this article, please, hit that clap button 50 times, this way more people will be able to find it!
Thank you and have a great day, see you in the next one!
Build AI for Generating Quant Trading Strategies in C# (Part 3) was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Besarion Turmanauli
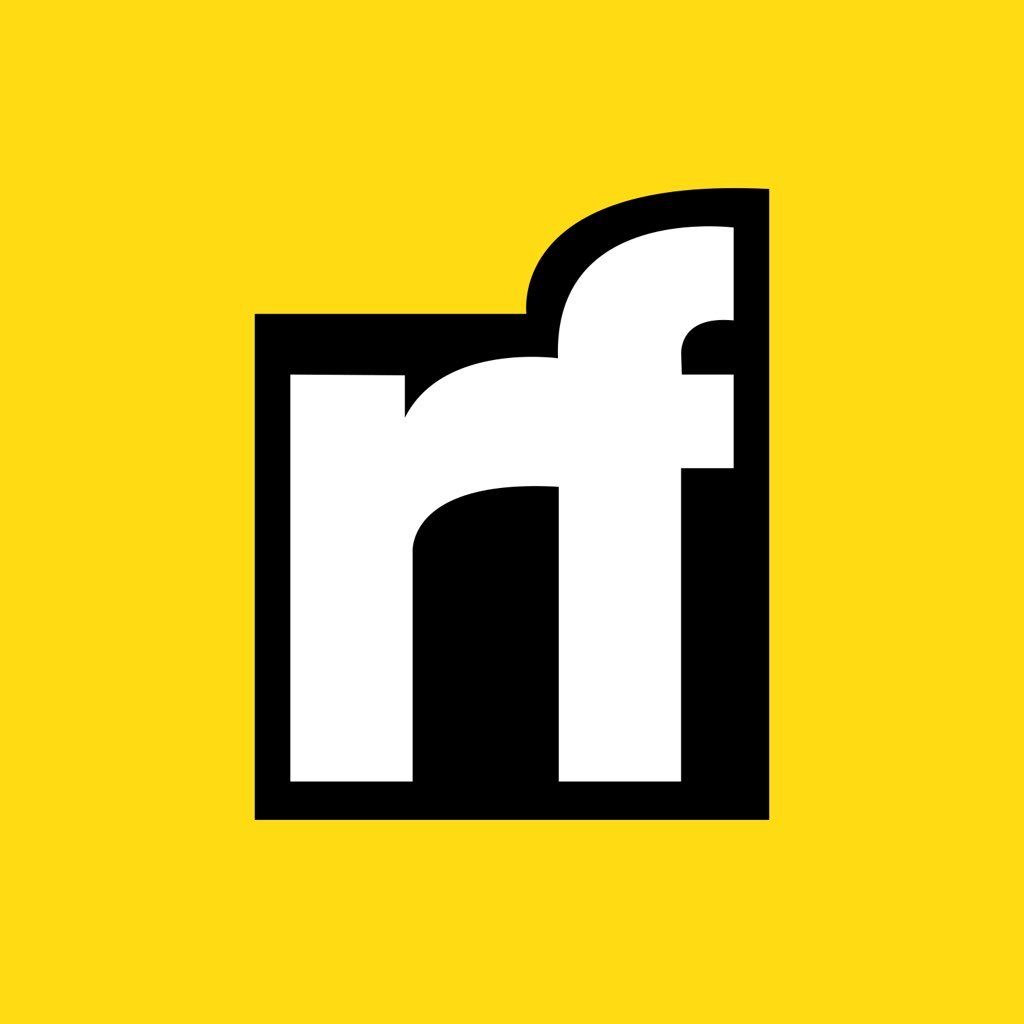
Besarion Turmanauli | Sciencx (2021-04-13T12:52:03+00:00) Build AI for Generating Quant Trading Strategies in C# (Part 3). Retrieved from https://www.scien.cx/2021/04/13/build-ai-for-generating-quant-trading-strategies-in-c-part-3/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.