This content originally appeared on DEV Community and was authored by Md Nazmus Sakib
Introduction to ES6
JavaScript ES6 (ECMAScript 2015) marked a significant update to the language, introducing many powerful features that improved developer productivity and code readability. Released in June 2015, ES6 brought a host of enhancements that streamlined the development process and made JavaScript a more robust language for building complex applications.
Key Features of ES6
Here are the top 20 features introduced in ES6, complete with code examples:
-
Arrow Functions
- Syntax:
const add = (a, b) => a + b; console.log(add(2, 3)); // 5
-
Template Literals
- Syntax:
const name = 'John'; console.log(`Hello, ${name}!`); // Hello, John!
-
Destructuring Assignment
- Syntax:
const obj = { x: 1, y: 2 }; const { x, y } = obj; console.log(x, y); // 1 2
-
Default Parameters
- Syntax:
function multiply(a, b = 1) { return a * b; } console.log(multiply(5)); // 5
-
Rest and Spread Operators
- Rest:
function sum(...numbers) { return numbers.reduce((a, b) => a + b, 0); } console.log(sum(1, 2, 3)); // 6
-
Spread:
const arr = [1, 2, 3]; const newArr = [...arr, 4, 5]; console.log(newArr); // [1, 2, 3, 4, 5]
-
Let and Const
- Syntax:
let x = 10; const y = 20; x = 15; // Works // y = 25; // Error: Assignment to constant variable
-
Modules
- Syntax:
// module.js export const greet = (name) => `Hello, ${name}!`; // main.js import { greet } from './module.js'; console.log(greet('John')); // Hello, John!
-
Promises
- Syntax:
const myPromise = new Promise((resolve, reject) => { setTimeout(() => resolve("Success!"), 1000); }); myPromise.then(result => console.log(result)); // Success!
-
Classes
- Syntax:
class Animal { constructor(name) { this.name = name; } speak() { console.log(`${this.name} makes a noise.`); } } const dog = new Animal('Dog'); dog.speak(); // Dog makes a noise.
-
Enhanced Object Literals
- Syntax:
const x = 1; const y = 2; const obj = { x, y, greet() { return 'Hello'; } }; console.log(obj.greet()); // Hello
-
Iterators and Generators
- Iterators:
const myArray = [1, 2, 3]; const iterator = myArray[Symbol.iterator](); console.log(iterator.next().value); // 1
- **Generators**:
```javascript
function* generatorFunction() {
yield 1;
yield 2;
}
const gen = generatorFunction();
console.log(gen.next().value); // 1
```
-
Map and Set Data Structures
- Map:
const myMap = new Map(); myMap.set('key', 'value'); console.log(myMap.get('key')); // value
- **Set**:
```javascript
const mySet = new Set();
mySet.add(1);
mySet.add(1);
console.log(mySet.size); // 1
```
-
Symbol Data Type
- Syntax:
const sym = Symbol('description'); console.log(sym); // Symbol(description)
-
Binary and Octal Literals
- Syntax:
const binary = 0b1010; // 10 in decimal const octal = 0o755; // 493 in decimal console.log(binary, octal); // 10 493
-
New Built-in Methods
- Example:
const arr = Array.from('hello'); console.log(arr); // ['h', 'e', 'l', 'l', 'o']
-
String Methods
- Example:
const str = 'Hello World'; console.log(str.startsWith('Hello')); // true console.log(str.endsWith('World')); // true
-
Math and Number Enhancements
- Example:
console.log(Math.trunc(4.9)); // 4 console.log(Math.sign(-5)); // -1
-
Array Iteration Methods
- Example:
const numbers = [5, 12, 8, 130, 44]; const found = numbers.find(element => element > 10); console.log(found); // 12
-
Proxies and Reflection
- Example:
const target = {}; const handler = { get: function(target, prop) { return prop in target ? target[prop] : 37; } }; const proxy = new Proxy(target, handler); console.log(proxy.someProperty); // 37
-
WeakMap and WeakSet
- WeakMap:
const weakMap = new WeakMap(); const obj = {}; weakMap.set(obj, 'value'); console.log(weakMap.get(obj)); // value
- **WeakSet**:
```javascript
const weakSet = new WeakSet();
const obj1 = {};
weakSet.add(obj1);
console.log(weakSet.has(obj1)); // true
```
Conclusion
JavaScript ES6 brought a significant evolution in the language, making it more powerful, easier to use, and more enjoyable for developers. By understanding and utilizing these features, developers can write cleaner, more efficient, and more maintainable code.
Whether you are a novice or an experienced developer, mastering these ES6 features will enhance your programming skills and allow you to leverage the full potential of JavaScript in modern web development.
This guide serves as a comprehensive overview of JavaScript ES6 release notes and its top features, complete with code examples, providing you with valuable insights to navigate the modern JavaScript landscape. Happy coding!
This content originally appeared on DEV Community and was authored by Md Nazmus Sakib
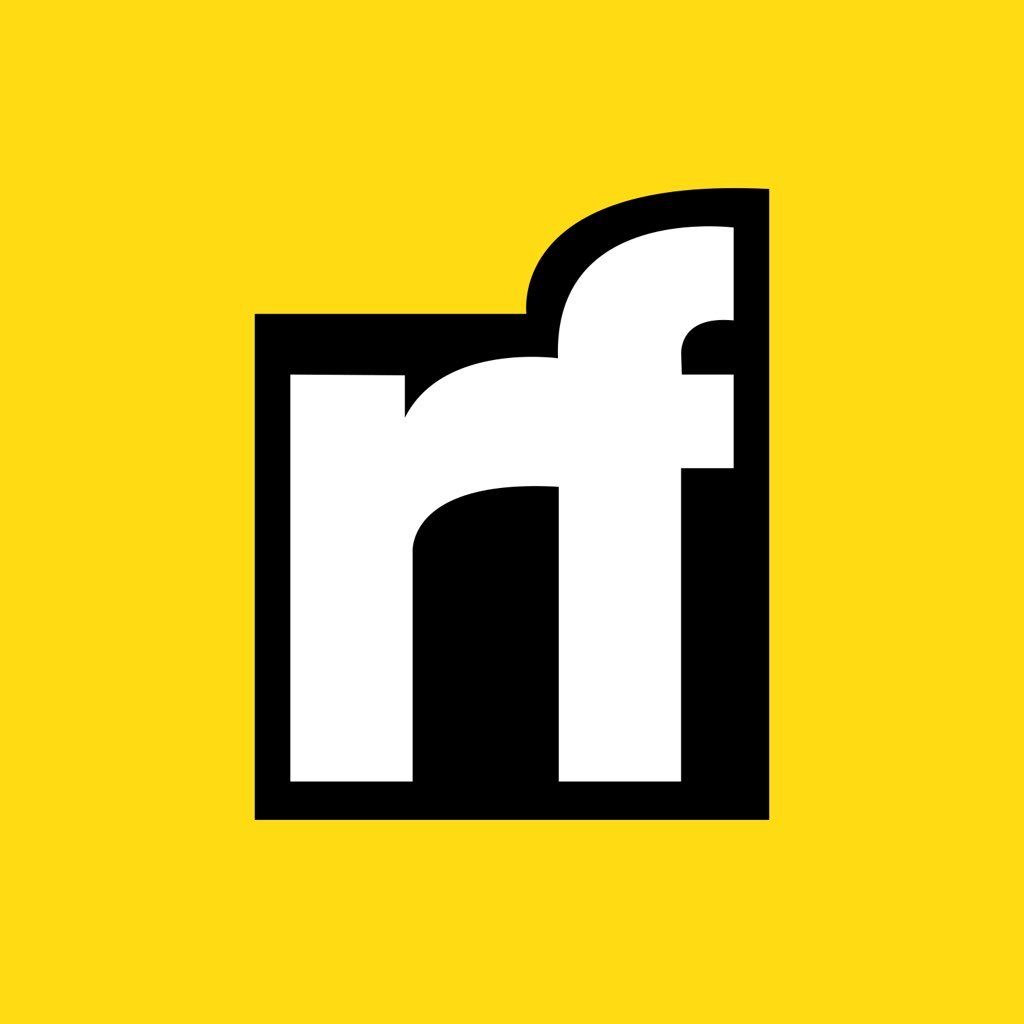
Md Nazmus Sakib | Sciencx (2024-10-09T19:08:46+00:00) Unlocking the Power of JavaScript ES6: A Comprehensive Guide to Its Top 20 Features. Retrieved from https://www.scien.cx/2024/10/09/unlocking-the-power-of-javascript-es6-a-comprehensive-guide-to-its-top-20-features/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.