This content originally appeared on DEV Community and was authored by TheYoungestCoder
As you may know, many programming languages have the ability to generate random numbers, while not letters (at least in javascript). Today, I will walk you through how you can make a random password/code generator.
Firstly, we need to know how to create a random number. In javascript it's pretty simple:
Math.random()
It returns a random number—but only between 0-1. Since there are 26 numbers in the alphabet but many possibilities from Math.random
, we need to use a little logic to get a random number that has 26 possibilities:
Math.floor(Math.random() * 25)
At this stage our code will return a random from 0 to 25. Since these are only numbers—we need to get the character associated with that number. Luckily, javascript has a function called String.fromCharCode
. Since the character code of "a" is 97, we need to offset our random number by 97:
String.fromCharCode(
Math.round(Math.random() * 25) + 97
)
Now that we have our random letter, time to repeat the process using a for loop—or my personal favorite, creating an empty array followed by mapping it. Putting it all together, this is what it would look like:
function randomPassword(length) {
// initialize an array of the specified length
var charArr = [...Array(length)]
// map each item in the array to a random char
charArr = charArr.map(
_ => String.fromCharCode(
Math.round(Math.random() * 25) + 97
)
)
// convert the array into a string by joining it
return charArr.join("")
}
This content originally appeared on DEV Community and was authored by TheYoungestCoder
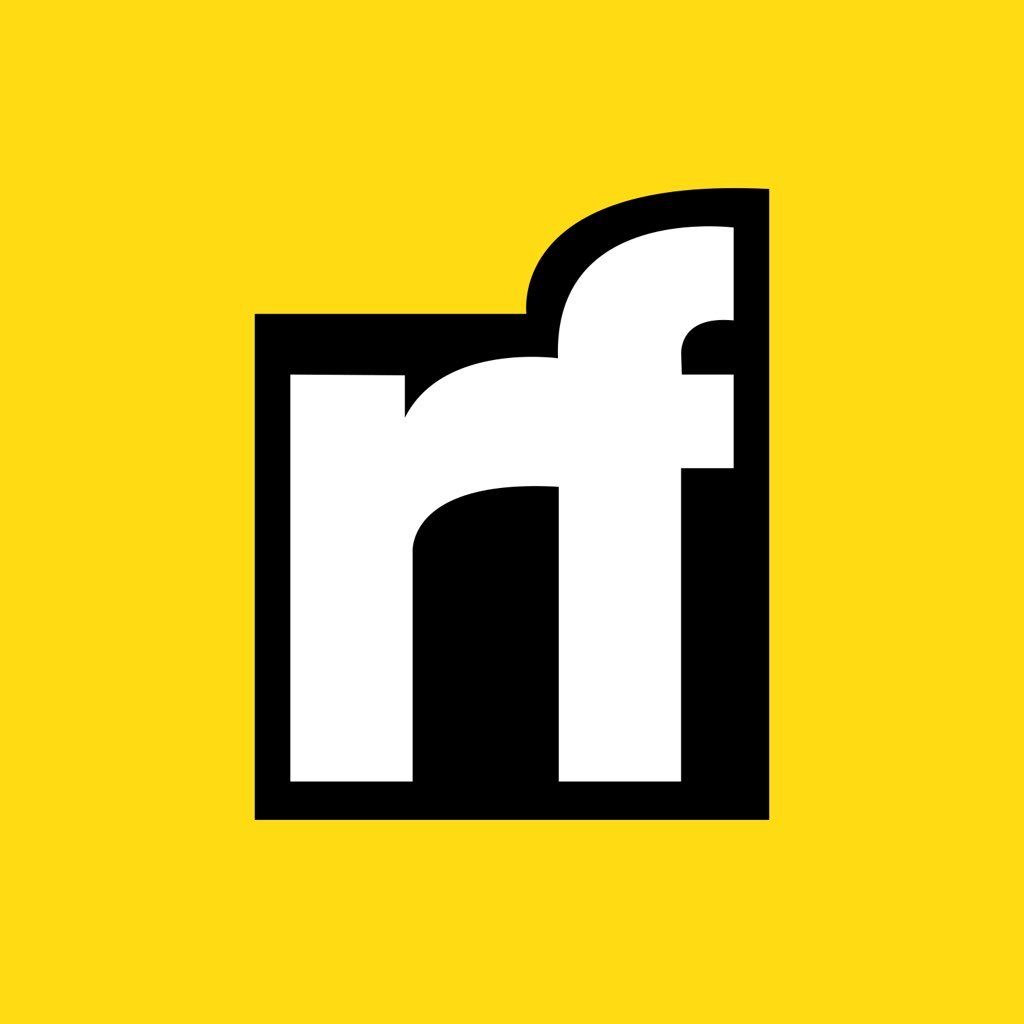
TheYoungestCoder | Sciencx (2021-04-15T20:52:00+00:00) Generating Random Passwords. Retrieved from https://www.scien.cx/2021/04/15/generating-random-passwords/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.