This content originally appeared on Telerik Blogs and was authored by Nikolay Iliev
Fiddler Everywhere can help you pin down syntax, logistic, execution and runtime errors and more. Learn about five debugging issues Fiddler can help solve.
Fiddler Everywhere comes with features that allow you to modify, mock, filter and automate how HTTP(S) traffic is processed. Developers can simulate various scenarios by altering requests and responses dynamically. Fiddler’s rule system is highly customizable and provides great flexibility when working with network traffic. This article will demonstrate the top five debugging issues you can solve using Progress Telerik Fiddler Everywhere.
1. Syntax Errors
Syntax errors are one of the main reasons bugs are introduced in the development process. While there are complex IDE tools for maintaining manually written codebase, the syntax errors are harder to pinpoint when appearing within calls to online API or are part of received HTTPS responses.
Fiddler headers and body inspectors are neat places to track these kinds of errors. Let’s demonstrate this through a sample app that calls the public NASA API for an Astronomical Picture of the Day (APOD).
The initial source we use is as follows:
<html>
<head>
<script>
let nasa_endpoint = "https://api.nasa.gov/planetary/apod?1&api_key=";
let api_key = "DEMO_KEY";
let apod = fetch(nasa_endpoint + api_key)
.then((response) => response.json())
.then(data => {
document.getElementById('img').src = data['url'];
})
</script>
</head>
<body>
<img id="img"/>
</body>
</html>
The above page runs on a local https server with the address 192.168.100.4:8080 for demonstration.
While using the Fiddler’s browser capturing mode, we can immediately see that our application loads, but the fetch request to the NASA APIs fails with status code 400 (Bad Request)
.
Double-clicking on the session further reveals the server’s response message, which indicates that we have used incorrect fields. We can now use the Params inspector in the Request tab to further analyze the query parameters passed. Here, our API endpoint contains an additional key named “1” with no value—which causes the issue.
While status code 400 is not so descriptive, sometimes the API will help us identify an issue with a more specific status code. Let’s rewrite the above snippet, so that now it uses the proper endpoint. However, this time the syntax error will be intentionally introduced in the value of the required API key.
<script>
let nasa_endpoint = "https://api.nasa.gov/planetary/apod?api_key=";
let api_key = "WRONG_KEY"
let apod = fetch(nasa_endpoint + api_key)
.then((response) => response.json())
.then(data => {
document.getElementById('img').src = data['url'];
})
</script>
This time, the NASA API returns status code 403 (Unauthorized)
. By default, Fiddler Everywhere is configured to display and highlight the sessions with status codes 4xx and 5xx to detect problematic sessions easier (if needed, you can show and hide different columns in the traffic grid).
The above immediately shows that the issue is within the value of the used API key, which developers can quickly fix.
2. Logical Errors
Like syntax errors, an issue can appear and break our web application when there is a logical error within our codebase or the API itself.
Let’s slightly modify the above example as follows:
<script>
let nasa_endpoint = "https://api.nasa.gov/planetary/apod?api_key=";
let api_key = "DEMO_KEY"
let apod = fetch(nasa_endpoint + api_key)
.then((response) => response.json())
.then(data => {
document.getElementById('img').src = data['title'];
})
</script>
Have you noticed the logical error? It is not immediately obvious. The issue here is based on results from the NASA endpoint, which returns an object with multiple keys, including URLs to images and thumbnails. In this specific case, our JS code uses the value of the title key and assigns it as a source for an HTML image. The error might not be detected immediately—the initial call to the NASA API works as expected. However, running the above in Fiddler shows the following:
We know we explicitly ran our app on http://192.168.100.4:8080, so the first session is expected. We also know that internally, our application makes a call to https://api.nasa.gov/planetary/apod, so that is also another expected session.
However, we also know that our application has an image that has not loaded properly, and we see a third session that returned status 404 (Not Found)
. This is the session that should be constructed through the URL contained in the JSON key-value pair, but we can immediately see that the added query parameter is not the expected HTTP address (that points to the JPG image) but rather a string that contains other information.
While in the simplified scenario, the issue is caused by a logical error in our own codebase (because we used data["title"]
instead of data["url"]
), the very same type of issues could be the result of a fault within the backend server. For example, we could have the right property called in our codebase, but its value might be deformed by the server API. In both cases, Fiddler allows you to quickly filter and detect that information and apply the needed fix.
Finally, let’s run the proper API call.
<script>
let nasa_endpoint = "https://api.nasa.gov/planetary/apod?api_key=";
let api_key = "DEMO_KEY"
let apod = fetch(nasa_endpoint + api_key)
.then((response) => response.json())
.then(data => {
document.getElementById('img').src = data['url'];
})
</script>
Now, we can see all API calls made as expected, and Fiddler can even preview images or HTML pages.
3. Execution Errors (Error Simulation with Rules)
In the previous sections, we discussed how you can use Fiddler to detect syntax and logical errors within our web applications.
However, you don’t have to wait for your application to crash (especially in production) to test that you have recovery mechanisms or if you are passing the proper information to the end users.
Here is where you can use Fiddler to debug various issues, mock status codes and load alternative responses to fully test the ability of your web application to handle different scenarios.
Perhaps one of the most powerful features of Fiddler is its Rules tab. Simply put, this application section enables you to debug, modify, filter and/or entirely mock sessions.
While still using our little astronomical application, we can use the Rules to mock various status codes. You don’t need to access the backend—the modifications of the requests and responses happens in the middle which is why tools like Fiddler are called MITM proxies (man-in-the-middle).
For example:
- Capture the traffic from our APOD application.
- Open the context menu and use the Add New Rule feature.
- Within the Fiddler’s Rules Builder, create new rule that uses the Return Predefined Responses action.
With the above steps, you can mock a server behavior or test different scenarios on-the-fly and within few seconds. No need to modify the backend—no need to even have access to the server! Fiddler stands in the middle and allows you to mock the production or staging environments of your web applications.
And things are getting better. While the above example shows how to use a list of predefined HTTP responses (that are mocking different status codes), you can also create entirely custom responses. This enables you to test potential fixes or new designs—again without having to make changes within your application or server.
For example:
- Capture the traffic from the targeted page.
- Open the context menu and use the Add New Rule feature.
- Within the Fiddler’s Rules Builder, create a new rule that uses the Return Manual Response or Return File actions.
4. Runtime Changes and Errors
One of the most powerful features of Fiddler Everywhere is its ability to “pause” the traffic and modify it during the HTTP Sessions execution. Similarly to Neo dodging the bullets in the iconic Matrix scene, you can use Fiddler to pause the execution of a targeted API call and then to modify its behavior with the idea of fixing an error or to demonstrate an entirely new reality. The feature is well-known to the Fiddler community—Fiddler breakpoints.
In Fiddler Everywhere, you can create two types of breakpoints. The first one will pause the session before the HTTP Request is sent to the server, while the second one will pause before the HTTP Response is sent to the client.
The immediate effect of the above feature is that you can modify live traffic before it reaches the targeted destination. The possibilities here are endless—you can test any API call on how it will behave with different headers, cookies and bodies. You can “hack” a page without having access to either the client or the server. All you need is Fiddler to stand in the middle as a proxy and to use a breakpoint.
Let’s visualize the process through a quick demo. We will use a well-known online service that mocks a cached endpoint.
- Start Fidder Everywhere and use the browser capturing mode.
- Open an endpoint that mocks cached responses like https://httpbin.org/cache.
The above returns a 304 if an If-Modified-Since header or If-None-Match is present within the HTTP request. Otherwise, it returns the same as a GET (200). If this is your first time accessing the page, it will show 200. Refresh the page, and you will receive status 304
We will use Fiddler to pause the request execution and explicitly add or remove the mentioned headers before they reach the server.
Open the context menu from the captured session and use “Add New Rule.”
Within the Rules Builder, add the action called “Set Breakpoint”, and specific that to use “Before Sending a Request.”
- Return to the browser and refresh the page.
At this moment, Fiddler will pause the execution of the HTTP Request. Once the request pauses, you can load the Request inspector and use the Raw tab to make any modifications.
- Finally, unpause the request and the session will proceed with its execution.
With the above we have just made a runtime modification that changed the behavior of the client and the server. As said before, no actual changes are made in either destination—we simply made a Matrix-style hack move that opens a ton of testing possibilities!
5. Testing Performance and Security
Many modern web applications use various features that can cause performance or security issues. One typical example is authentication, which is typically linked to a backend server that needs to be operational for uninterrupted service for clients. Testing the server’s vulnerabilities and weak points to help prevent potential attacks, such as DDoS, is a significant task for developers.
Fiddler Everywhere allows you to test your server endpoints for security issues, such as the following example. You can test if your application has a secure authentication process by following best practices, like creating exponential retries for consecutive requests.
Consider a scenario where a web application attempts to fail authentication requests. The server (in this example, HTTPBin) consistently returns a status 500, prompting our application to retry continuously.
Using Fiddler, we can capture the traffic from our app (with process name node:16176) and identify several issues immediately:
- The retried requests appear to be never-ending.
- The retried requests are executed after a specific period, lacking an exponential backoff strategy.
You can notice this from the Request Time column or by selecting all sessions in Fiddler Everywhere and then switching to the Overview tab, confirming the absence of exponential timing between each request.
These issues could lead to a significant problem. For instance, if the server is unavailable for an extended period, the application’s users will continually trigger many requests, potentially resulting in a self-inflicted DDoS that renders the server unresponsive.
After obtaining information from Fiddler, we can review our application. The demo app used a well-known NPM library called retry (which sets the number and type of retries) alongside axios (for executing the requests). A quick code review revealed that the retry operation was configured with specific parameters.
const retry = require('retry');
function faultTolerantHttpRequest(URL, cb) {
// Initialize a retry operation with custom settings
const operation = retry.operation({
forever: true, // Retries forever
factor: 0, // Exponential backoff factor
minTimeout: 100, // Minimum timeout (in milliseconds)
maxTimeout: 10 * 100, // Maximum timeout (in milliseconds)
randomize: false, // Randomize the timeouts
});
The configuration confirmed our findings—the retry was explicitly set to “forever,” and the exponential backoff factor was set to 0, meaning no exponential timings. To address this, we removed the “forever” setting, set a strict limit for the maximum possible number of retries, implemented an exponential backoff strategy and adjusted the timeout values.
const operation = retry.operation({
retries: 5, // Limits the number of retries to 5
factor: 2, // Sets an exponential timing that adds two additional times after each retry
minTimeout: 1000
maxTimeout: 60 * 1000
randomize: false
});
With these changes in place, the retried requests in Fiddler now appear as follows:
The captured traffic reveals that our application makes only five retries and accepts and ends all requests on the sixth attempt. The Overview tab visualizes the change in the factor attribute, indicating that our exponential backoff strategy is operational and protects us from a potential DDoS attack.
This demonstration illustrates how you can use Fiddler’s capabilities, like the Overview tab, to diagnose your web app within seconds without handling complex applications or codebases. Similar approaches can be applied to test refresh tokens, check for invalid or expiring API certificates, create rules to mimic unexpected client behavior and more.
Fiddler Is a Swiss Army Knife for Web Debugging
Fiddler is a versatile tool with expanded functionalities and good testing capabilities. Whether you would like to debug an error, test a fallback mechanism, try a new user interface or check the ability of your web app and backend server to withstand unexpected scenarios, Fiddler offers all the right tools.
Do you have another scenario in mind? Please share your ideas and suggestions in the Fiddler Everywhere public GitHub repository or the Feedback Portal, and help us improve Fiddler Everywhere.
If you aren’t yet using Fiddler Everywhere, test it out with a free trial.
This content originally appeared on Telerik Blogs and was authored by Nikolay Iliev
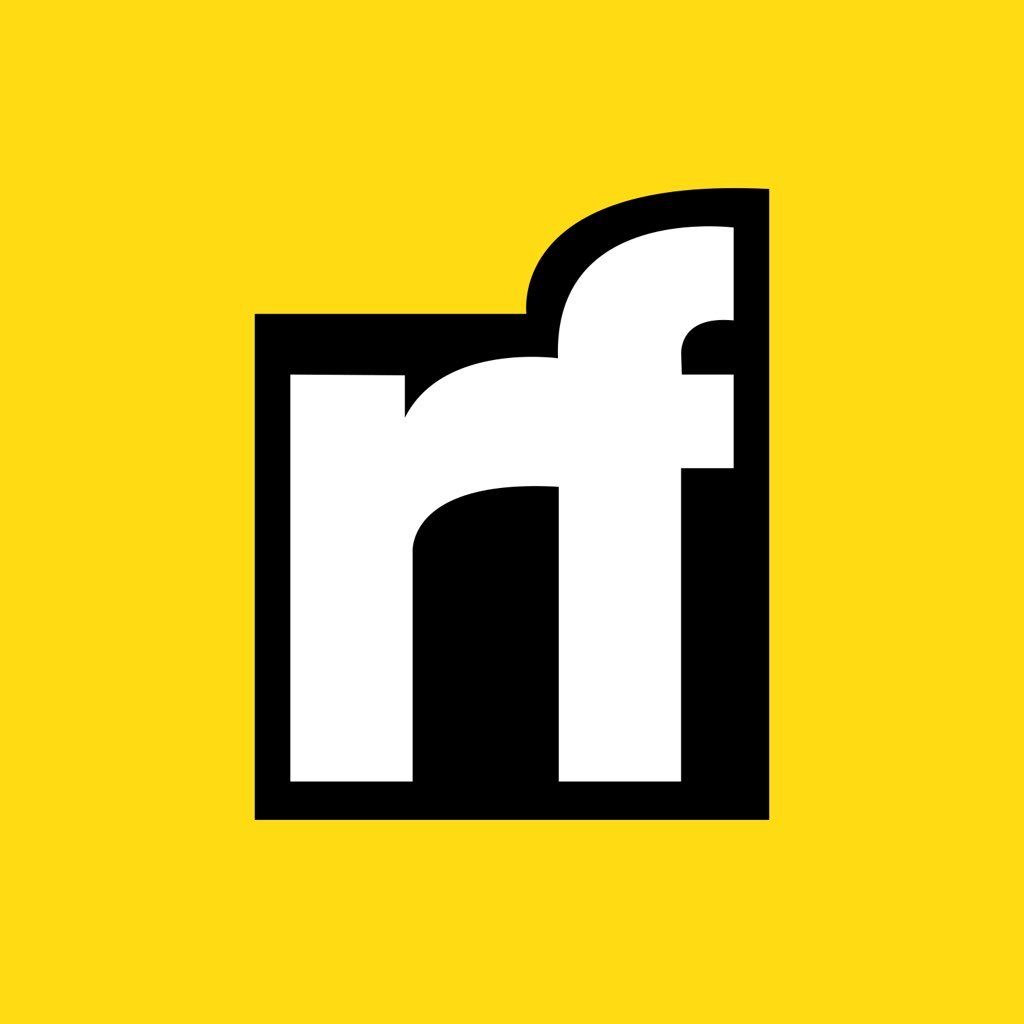
Nikolay Iliev | Sciencx (2024-10-24T14:16:57+00:00) The Top 5 Debugging Issues to Solve with Fiddler Everywhere. Retrieved from https://www.scien.cx/2024/10/24/the-top-5-debugging-issues-to-solve-with-fiddler-everywhere/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.