This content originally appeared on DEV Community and was authored by Inder Rajoriya
Radhe Radhe Guy's
JavaScript Promises can sometimes feel like that one friend who says, "Bhai, kaam ho jayega!" but leaves you waiting forever. This post will break down promises in a fun and easy way with relatable Indian examples.
1. What is a Promise in JavaScript?
A Promise in JavaScript is like ordering biryani online. It will either:
Resolve – Biryani arrives and you're happy 😋.
Reject – Delivery guy cancels, and you're left starving 😭.
Example:
const biryaniOrder = new Promise((resolve, reject) => {
let biryaniReady = true;
if (biryaniReady) {
resolve('Biryani is here!');
} else {
reject('No biryani for you today.');
}
});
2. Consuming a Promise (Then and Catch)
To handle a Promise, we use .then() and .catch().
Example:
biryaniOrder
.then((message) => {
console.log(message); // Biryani is here!
})
.catch((error) => {
console.log(error); // No biryani for you today.
});
Relatable? Imagine this:
"When Zomato says 'Arriving in 10 mins' but it's been 30 mins..."
3. Promise Chaining (One Thing After Another)
Promise chaining allows sequential execution. Like ordering dessert after biryani.
Example:
biryaniOrder
.then((message) => {
console.log(message);
return 'Let’s order gulab jamun!';
})
.then((dessert) => {
console.log(dessert); // Let's order gulab jamun!
})
.catch((error) => {
console.log(error);
});
when the Gulab jamun is coming😋
4. Async/Await (The VIP Treatment)
Async/await simplifies working with promises, like being a VVIP at a wedding.
Example:
async function enjoyMeal() {
try {
let food = await biryaniOrder;
console.log(food);
console.log('Gulab jamun coming next...');
} catch (error) {
console.log(error);
}
}
enjoyMeal();
5. Why Use Promises?
- Better readability (No callback hell 😈)
- Easier error handling
- Clean asynchronous operations
When your relatives promise shaadi ki laddoo but ghost you later.
Thanks for reading! 🤍✌️
This content originally appeared on DEV Community and was authored by Inder Rajoriya
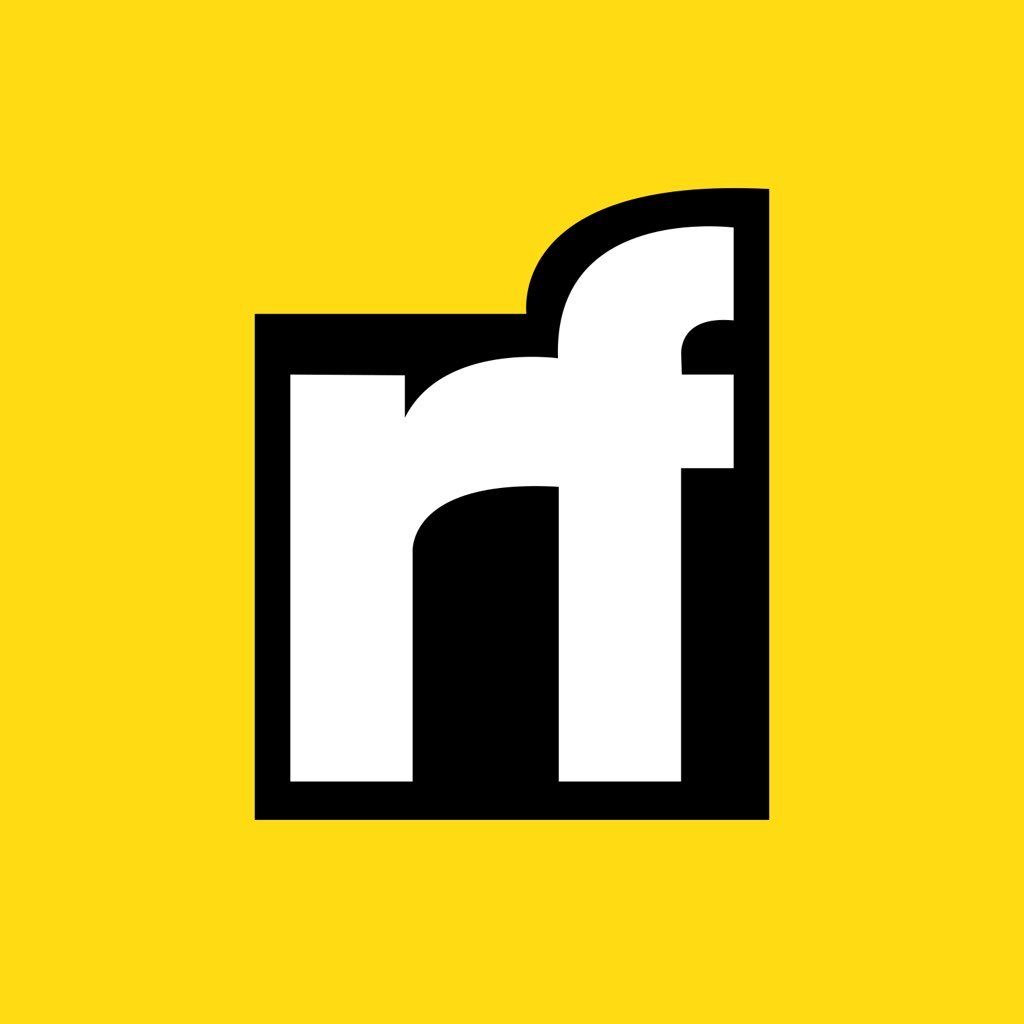
Inder Rajoriya | Sciencx (2025-01-08T18:07:06+00:00) Understanding JavaScript Promises (With a Desi Twist 🌟). Retrieved from https://www.scien.cx/2025/01/08/understanding-javascript-promises-with-a-desi-twist-%f0%9f%8c%9f/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.