This content originally appeared on DEV Community and was authored by Nadim Chowdhury
Role-Based Access Control (RBAC) is a security model that restricts system access based on user roles. Implementing RBAC in a MERN (MongoDB, Express, React, Node.js) application enhances security by ensuring users have appropriate permissions based on their roles.
1. Setting Up User Roles
Common user roles include:
- Admin: Full access to the system.
- Editor: Can create and modify content but lacks administrative privileges.
- User: Can only view content and perform limited actions.
2. Defining User Roles in MongoDB
In your user model, add a role field:
const mongoose = require('mongoose');
const UserSchema = new mongoose.Schema({
username: { type: String, required: true, unique: true },
password: { type: String, required: true },
role: { type: String, enum: ['admin', 'editor', 'user'], default: 'user' }
});
module.exports = mongoose.model('User', UserSchema);
3. Implementing Role-Based Middleware in Express
Create middleware to check user roles:
const checkRole = (roles) => {
return (req, res, next) => {
if (!roles.includes(req.user.role)) {
return res.status(403).json({ message: 'Access denied' });
}
next();
};
};
4. Applying Middleware to Routes
Secure routes based on roles:
const express = require('express');
const router = express.Router();
const { checkRole } = require('../middleware/authMiddleware');
router.post('/create-post', checkRole(['admin', 'editor']), (req, res) => {
res.json({ message: 'Post created successfully' });
});
router.delete('/delete-user', checkRole(['admin']), (req, res) => {
res.json({ message: 'User deleted successfully' });
});
module.exports = router;
5. Handling RBAC in React Frontend
Control UI elements based on user roles:
const Dashboard = ({ user }) => {
return (
<div>
<h1>Dashboard</h1>
{user.role === 'admin' && <button>Manage Users</button>}
{(user.role === 'admin' || user.role === 'editor') && <button>Create Post</button>}
</div>
);
};
Conclusion
RBAC is crucial for securing a MERN application by controlling access to different parts of the system. By defining user roles in MongoDB, implementing role-based middleware in Express, and controlling frontend UI elements, you can ensure a secure and structured access control system.
If you enjoy my content and would like to support my work, you can buy me a coffee. Your support is greatly appreciated!
Disclaimer: This content has been generated by AI.
This content originally appeared on DEV Community and was authored by Nadim Chowdhury
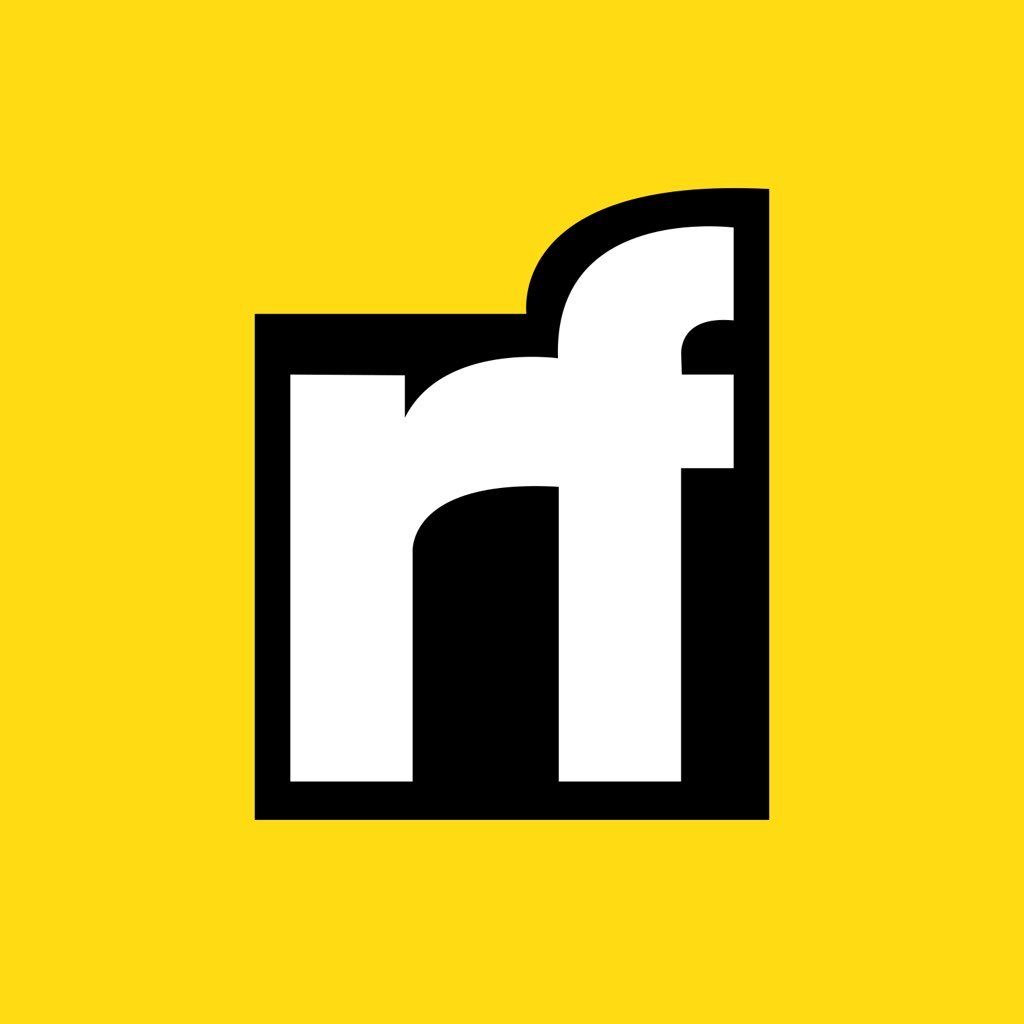
Nadim Chowdhury | Sciencx (2025-02-01T12:38:08+00:00) Role-Based Access Control (RBAC) in a MERN Application. Retrieved from https://www.scien.cx/2025/02/01/role-based-access-control-rbac-in-a-mern-application/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.