This content originally appeared on Envato Tuts+ Tutorials and was authored by George Martsoukos
In today’s tutorial, we’ll learn three different techniques for creating a “wipe fill” CSS hover effect. We’ll even go one step further and give ourselves the flexibility to select the direction of the animation.
CSS Hover Effects
Here’s what we’ll be creating:
As you can see, when you hover over the text a fill is applied, wiping across from one side to the other. The upper menu links have no apparent fill, to begin with, but instead have a text stroke which then appears to fill up on hover.
Using CSS transitions you can alter the speed and easing of the wiping effect to whatever suits your project.
Jump to techniques in this section
CSS Text Effect: Technique #1
Let’s walk through the first technique, in which we use a bit of extra markup to create each link’s “wipe fill” element.
Specify the Page Markup
We’ll start with a plain unordered list which will represent a typical page menu. Each menu link will include a text node and a span
element. The text inside the span
will match the list item’s text. We’ll give this element aria-hidden="true"
because we want to hide it from any accessibility devices. In actual fact, the only reason for its existence is to help us build the desired hover effect.
As a bonus, we’ll give ourselves the ability to set the direction of the hover effect animation. We can pass the data-animation
custom attribute to the list items which will determine the starting position of their animation. Possible attribute values are to-left
, to-bottom
, and to-top
. By default, the effect will appear from left to right.
Here’s the required markup:
1 |
<ul class="menu"> |
2 |
<li>
|
3 |
<a href="#0"> |
4 |
About Us |
5 |
<span class="outer" aria-hidden="true"> |
6 |
<span class="inner">About Us</span> |
7 |
</span>
|
8 |
</a>
|
9 |
</li>
|
10 |
<li data-animation="to-left"> |
11 |
<a href="#0"> |
12 |
Projects |
13 |
<span class="outer" aria-hidden="true"> |
14 |
<span class="inner">Projects</span> |
15 |
</span>
|
16 |
</a>
|
17 |
</li>
|
18 |
<li data-animation="to-bottom"> |
19 |
<a href="#0"> |
20 |
Clients |
21 |
<span class="outer" aria-hidden="true"> |
22 |
<span class="inner">Clients</span> |
23 |
</span>
|
24 |
</a>
|
25 |
</li>
|
26 |
<li data-animation="to-top"> |
27 |
<a href="#0"> |
28 |
Contact |
29 |
<span class="outer" aria-hidden="true"> |
30 |
<span class="inner">Contact</span> |
31 |
</span>
|
32 |
</a>
|
33 |
</li>
|
34 |
</ul>
|
Specify the Basic Styles
Let’s continue with the CSS styles.
We’ll add a stroke to the menu links by using the native text-stroke
property. Nowadays, this property has great browser support. However, in case you need to support Internet Explorer or Microsoft Edge 12-14, you can use the text-shadow
property to simulate a stroke.
If you don’t like the stroke around the text, I’ve created an alternative layout which will append a background color to the menu along with its links. To enable it, just attach the has-bg
class to the menu.
The associated styles are as follows:
1 |
/*CUSTOM VARIABLES HERE*/
|
2 |
|
3 |
.has-bg { |
4 |
background: var(--stroke-color); |
5 |
padding: 40px 0 60px 0; |
6 |
margin-top: 20px; |
7 |
}
|
8 |
|
9 |
.menu a { |
10 |
position: relative; |
11 |
display: inline-block; |
12 |
font-weight: bold; |
13 |
font-size: 60px; |
14 |
line-height: 1.4; |
15 |
overflow: hidden; |
16 |
color: var(--white); |
17 |
-webkit-text-stroke: 1px var(--stroke-color); |
18 |
/*text-shadow: -1px -1px 0 var(--stroke-color), 1px -1px 0 var(--stroke-color), -1px 1px 0 var(--stroke-color), 1px 1px 0 var(--stroke-color);*/
|
19 |
}
|
20 |
|
21 |
.has-bg a { |
22 |
color: var(--secondary-color); |
23 |
text-shadow: none; |
24 |
}
|
And Now for the Animation Styles
The outer span
inside the links will be absolutely positioned. Additionally we’ll give it a dark cyan background color and set its overflow
property to hidden
. By default, we’ll move it 100% of its original position to the left and its inner span
100% of its original position to the right. Then, each time we hover over a link, we’ll remove their initial transform
values. That simple trick will produce a fill text hover effect which will go from left to right.
Here are the associated styles:
1 |
/*CUSTOM VARIABLES HERE*/
|
2 |
|
3 |
.menu .outer { |
4 |
position: absolute; |
5 |
top: 0; |
6 |
left: 0; |
7 |
overflow: hidden; |
8 |
color: var(--fill-color); |
9 |
transform: translateX(-100%); |
10 |
}
|
11 |
|
12 |
.menu .inner { |
13 |
display: inline-block; |
14 |
transform: translateX(100%); |
15 |
}
|
16 |
|
17 |
.menu a .outer, |
18 |
.menu a .inner { |
19 |
transition: transform 0.15s cubic-bezier(0.29, 0.73, 0.74, 1.02); |
20 |
}
|
21 |
|
22 |
.menu a:hover .outer, |
23 |
.menu a:hover .inner { |
24 |
transform: none; |
25 |
}
|
Specifying Direction
Lastly, as discussed in the markup section, we can customize the direction of our hover effect by passing the data-animation
attribute to a menu item. Depending on the effect direction (horizontal or vertical), we have to define reversed transform values for the .outer
and .inner
elements:
1 |
[data-animation="to-left"] .outer { |
2 |
transform: translateX(100%); |
3 |
}
|
4 |
|
5 |
[data-animation="to-left"] .inner { |
6 |
transform: translateX(-100%); |
7 |
}
|
8 |
|
9 |
[data-animation="to-top"] .outer { |
10 |
transform: translateY(100%); |
11 |
}
|
12 |
|
13 |
[data-animation="to-top"] .inner { |
14 |
transform: translateY(-100%); |
15 |
}
|
16 |
|
17 |
[data-animation="to-bottom"] .outer { |
18 |
transform: translateY(-100%); |
19 |
}
|
20 |
|
21 |
[data-animation="to-bottom"] .inner { |
22 |
transform: translateY(100%); |
23 |
}
|
The resulting demo:
CSS Text Effect: Technique #2
Let’s now examine the second technique, in which we keep our markup cleaner by using pseudo-elements to provide the “wipe fill”.
Specify the Page Markup
We’ll start again with a plain unordered list. Each menu link will include the data-text
custom attribute. The value of this attribute will match the text of the related link.
Similarly to the previous example, we’ll be able to customize the starting position of an animation via the data-animation
attribute. Possible attribute values are to-left
, to-bottom
, and to-top
. By default, the effect will appear from left to right.
Here’s the required markup to get us started:
1 |
<ul class="menu"> |
2 |
<li>
|
3 |
<a data-text="About Us" href="#0">About Us</a> |
4 |
</li>
|
5 |
<li data-animation="to-left"> |
6 |
<a data-text="Projects" href="#0">Projects</a> |
7 |
</li>
|
8 |
<li data-animation="to-bottom"> |
9 |
<a data-text="Clients" href="#0">Clients</a> |
10 |
</li>
|
11 |
<li data-animation="to-top"> |
12 |
<a data-text="Contact" href="#0">Contact</a> |
13 |
</li>
|
14 |
</ul>
|
Specify the Animation Styles
The basic CSS styles for this are the same as in the previous technique. For the animation, we’ll define the ::before
pseudo-element of each menu link and absolutely position it. Its content will come from the data-text
attribute value of the parent link. Initially, its width will be 0, but then, when we hover over the target link, it will be set to 100%. Also, we’ll give it white-space: nowrap
, so the text will never wrap to a new line.
The related styles for this are as follows:
1 |
/*CUSTOM VARIABLES HERE*/
|
2 |
|
3 |
.menu a::before { |
4 |
content: attr(data-text); |
5 |
position: absolute; |
6 |
top: 0; |
7 |
left: 0; |
8 |
width: 0; |
9 |
overflow: hidden; |
10 |
color: var(--fill-color); |
11 |
white-space: nowrap; |
12 |
transition: all 0.15s cubic-bezier(0.29, 0.73, 0.74, 1.02); |
13 |
}
|
14 |
|
15 |
.menu a:hover::before { |
16 |
width: 100%; |
17 |
}
|
Add the Hover Effect Styles
Next, we’ll add the styles responsible for customizing the direction of our hover effect. So first, we have to check the direction of the desired animation (horizontal or vertical), then animate accordingly the width
or the height
property of the target pseudo-element. In addition, in case we’re interested in the “to left” or “to top” animation, we should swap the text color values of the target pseudo-element and its parent link.
The styles that handle these animations are as follows:
1 |
/*CUSTOM VARIABLES HERE*/
|
2 |
|
3 |
.menu [data-animation="to-left"] a, |
4 |
.menu [data-animation="to-top"] a { |
5 |
color: var(--fill-color); |
6 |
}
|
7 |
|
8 |
.menu [data-animation="to-left"] a::before, |
9 |
.menu [data-animation="to-top"] a::before { |
10 |
color: var(--white); |
11 |
}
|
12 |
|
13 |
.menu [data-animation] a::before { |
14 |
width: 100%; |
15 |
}
|
16 |
|
17 |
.menu [data-animation="to-left"] a:hover::before { |
18 |
width: 0; |
19 |
}
|
20 |
|
21 |
.menu [data-animation="to-top"] a::before { |
22 |
height: 100%; |
23 |
}
|
24 |
|
25 |
.menu [data-animation="to-top"] a:hover::before { |
26 |
height: 0; |
27 |
}
|
28 |
|
29 |
.menu [data-animation="to-bottom"] a::before { |
30 |
height: 0; |
31 |
}
|
32 |
|
33 |
.menu [data-animation="to-bottom"] a:hover::before { |
34 |
height: 100%; |
35 |
}
|
The resulting demo:
CSS Text Effect: Technique #3
This third CSS hover effect technique (which is the cleanest and quickest) takes advantage of the clip-path
property.
Specify the Page Markup
The markup will be exactly as the second technique’s markup; there will be a list with links with the data-text
attribute attached to each one. Again, the existence of the data-animation
attribute to a menu item can customize the animation direction which will be from left to right by default.
1 |
<ul class="menu"> |
2 |
<li>
|
3 |
<a data-text="About Us" href="#0">About Us</a> |
4 |
</li>
|
5 |
<li data-animation="to-left"> |
6 |
<a data-text="Projects" href="#0">Projects</a> |
7 |
</li>
|
8 |
<li data-animation="to-bottom"> |
9 |
<a data-text="Clients" href="#0">Clients</a> |
10 |
</li>
|
11 |
<li data-animation="to-top"> |
12 |
<a data-text="Contact" href="#0">Contact</a> |
13 |
</li>
|
14 |
</ul>
|
Specify the Animation Styles
The styles will look like the previous example. We'll keep having an absolutely positioned ::before
pseudo-element which will be invisible by default. This time though, we won't give it width: 0
or height: 0
, but instead, we'll make it invisible via the clip-path
property. Then, on hover, we'll animate this property and show the pseudo-element.
If you want to learn in greater detail about the clip-path
property and how to use it to achieve eye-catching scroll effects, have a look at the tutorials below.
How to Build a Grayscale to Color Effect on Scroll (CSS & JavaScript)
Create Beautiful Scrolling Animations With the CSS Clip-Path Property
Here are the main styles for this method to work:
1 |
/*CUSTOM VARIABLES HERE*/
|
2 |
|
3 |
.menu a::before { |
4 |
content: attr(data-text); |
5 |
position: absolute; |
6 |
top: 0; |
7 |
left: 0; |
8 |
width: 100%; |
9 |
height: 100%; |
10 |
color: var(--fill-color); |
11 |
clip-path: inset(0 100% 0 0); |
12 |
transition: clip-path 0.15s cubic-bezier(0.29, 0.73, 0.74, 1.02); |
13 |
}
|
14 |
|
15 |
.menu [data-animation] a:hover::before, |
16 |
.menu a:hover::before { |
17 |
clip-path: inset(0); |
18 |
}
|
19 |
|
20 |
/* ANIMATIONS
|
21 |
–––––––––––––––––––––––––––––––––––––––––––––––––– */
|
22 |
.menu [data-animation="to-left"] a::before { |
23 |
clip-path: inset(0 0 0 100%); |
24 |
}
|
25 |
|
26 |
.menu [data-animation="to-top"] a::before { |
27 |
clip-path: inset(100% 0 0 0); |
28 |
}
|
29 |
|
30 |
.menu [data-animation="to-bottom"] a::before { |
31 |
clip-path: inset(0 0 100% 0); |
32 |
}
|
The associated demo:
Conclusion
And, we’re done! In this exercise, we discussed three different ways for creating a CSS-only wipe-fill hover effect. Hopefully, these have inspired you to build something similar, or at least, incorporate the effect in an existing project.
As always, thanks for reading!
Learn CSS With These Projects
Few things are as fun in the world of front end coding as messing around with CSS; take a look at these CSS projects on Tuts+ and learn while playing!
This content originally appeared on Envato Tuts+ Tutorials and was authored by George Martsoukos
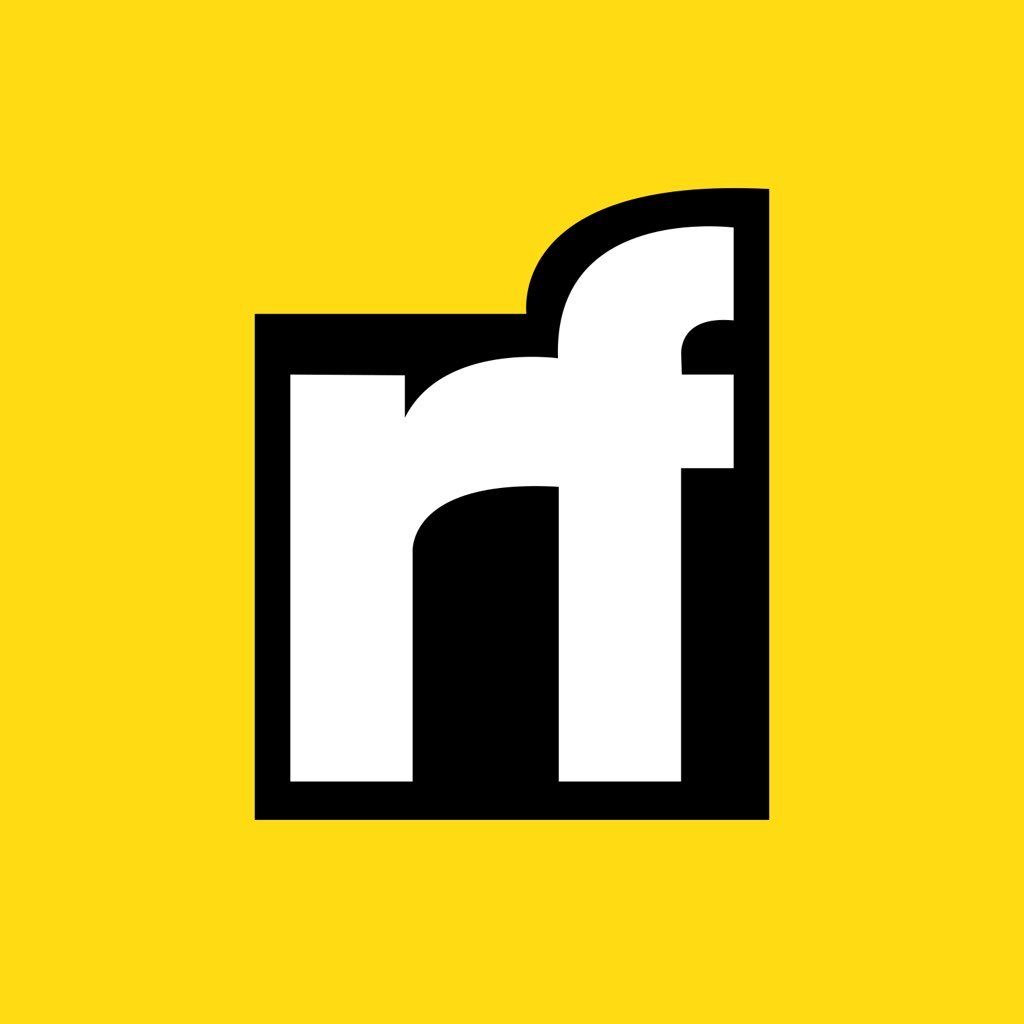
George Martsoukos | Sciencx (2019-10-27T14:12:38+00:00) CSS Hover Effects: Techniques for Creating a Text “Wipe Fill”. Retrieved from https://www.scien.cx/2019/10/27/css-hover-effects-techniques-for-creating-a-text-wipe-fill/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.