This content originally appeared on CodeSource.io and was authored by Deven
In this article, you will learn to create a Custom Class in Javascript Using the Constructor Pattern. Constructor Pattern can also help you understand how JavaScript classes really work.
Consider the following example to create a Custom Class in Javascript Using the Constructor Pattern.
function Fruit(sweetFruit, sourFruit) {
// Store public data using 'this'
this.sweetFruit = sweetFruit;
this.sourFruit = sourFruit;
// Add a nested function to represent a method
this.swapFruits = function () {
[this.sweetFruit, this.sourFruit] = [this.sourFruit, this.sweetFruit];
};
}
// using a class with an identical constructor
const newFruit = new Fruit("mango", "pineapple");
console.log(newFruit.sweetFruit);
newFruit.swapFruits();
console.log(newFruit.sweetFruit);
In the code snippet above first, you write a constructor function that accepts parameters and initializes your object, and then you use the this
keyword to create publicly accessible fields.
The post Create a Custom Class in Javascript Using the Constructor Pattern appeared first on CodeSource.io.
This content originally appeared on CodeSource.io and was authored by Deven
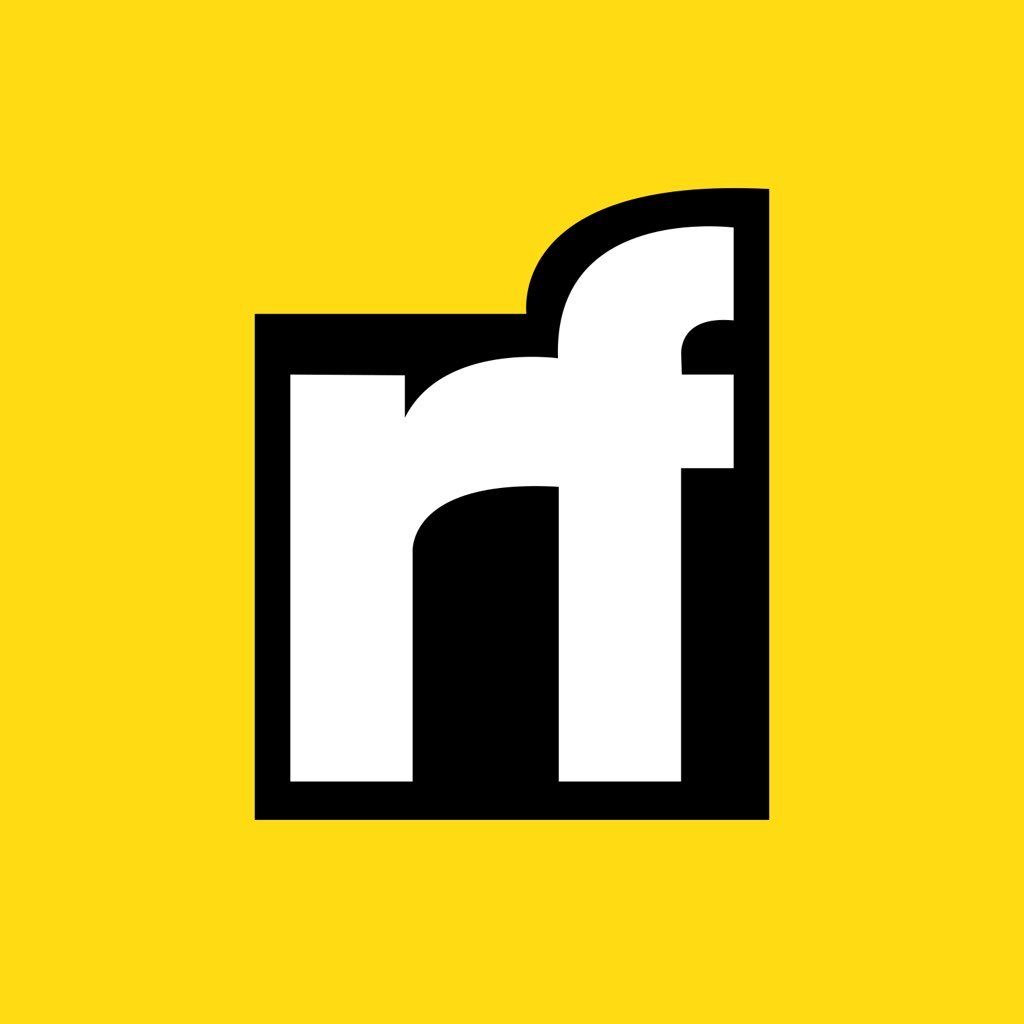
Deven | Sciencx (2021-02-10T15:54:00+00:00) Create a Custom Class in Javascript Using the Constructor Pattern. Retrieved from https://www.scien.cx/2021/02/10/create-a-custom-class-in-javascript-using-the-constructor-pattern/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.