This content originally appeared on DEV Community and was authored by rickavmaniac
An essential point to remember a training course is to take notes and discuss the subject with others. That's why every day I will post on dev.to the concepts and notes that I learned the day before.
If you want to miss nothing click follow and you are welcome to comments and discuss with me.
Without further ado here is a summary of my notes for day 5.
Array
An array is a data structure that contains a group of elements. Arrays are commonly used to organize data so that a related set of values can be easily sorted or searched.
// Data store one by one
name1 = 'Mike'
name2 = 'Paul'
name3 = 'John'
// More efficiently store in a array
names = ['Mike', 'Paul', 'John']
// Alternative declaration
names = new Array('Mike', 'Paul', 'John')
// Accessing a specific array element
console.log(names[0]) // Mike
console.log(names[1]) // Paul
console.log(names[2]) // John
// Number of entry in array
console.log(names.length)) // 3
// Get array last element
console.log(names[names.length-1])
// Modify a specific element
names[0] = 'Jack' // replace first entry (Mike) with Jack
//
Array basic methods
names = ['Mike', 'Paul', 'John']
// Add a element to array
names.push('Simon') // ['Mike', 'Paul', 'John', 'Simon']
// Add a element to the beginning
names.unshift('Jack') // ['Jack', 'Mike', 'Paul', 'John', 'Simon']
// Remove last element
names.pop() // ['Jack', 'Mike', 'Paul', 'John']
// Remove first element
names.shift() // ['Mike', 'Paul', 'John']
names = ['Mike', 'Paul', 'John']
// Find element index position
names.indexOf('Paul') // 1
// If elements includes in array
names.includes('Paul') // true
Objects
JavaScript objects are containers for named values, called properties and methods.
// Object declaration
const customer = {
firstName: 'Mike', // String
lastName: 'Tailor', // String
emails: ['mike@exemple.com', 'mike2@gmail.com'], //Array
creditLimit: 2500 // Number
}
// Access object property
console.log(customer.firstName) // Mike
// or
console.log(customer['firstName']) // Mike
// Add property
customer.phone = "800-828-1240"
// or
customer['phone'] = "800-828-1240"
Objects functions
const customer = {
firstName: 'Mike',
lastName: 'Tailor',
fullName: function() {
// this keyword reference the object calling the method
return this.firstName + ' ' + this.lastName
}
}
customer.firstName = 'Mike'
customer.lastName = 'Taylor'
console.log(customer.fullName()) // Mike Taylor
// or
console.log(customer['fullName']() // Mike Taylor
Loop
// For Loop keep running until condition is true
for (let i = 1;i <= 10; i++) {
console.log(i)
}
// For Loop : Break and Continue keyword
for (let i = 1;i <= 10; i++) {
if (i === 1) continue // go directly to next interation
if (i === 5) break // stop and exit loop
console.log(i)
}
// Loop within a Array
names = ['Mike', 'Paul', 'John']
for (let i = 0;names.length - 1; i++) {
console.log(names[i])
}
// While loop
let count = 1
while (count <= 10) {
count++
console.log(count)
}
Conclusion
That's it for part 5. Next day will cover DOM manipulation.
This content originally appeared on DEV Community and was authored by rickavmaniac
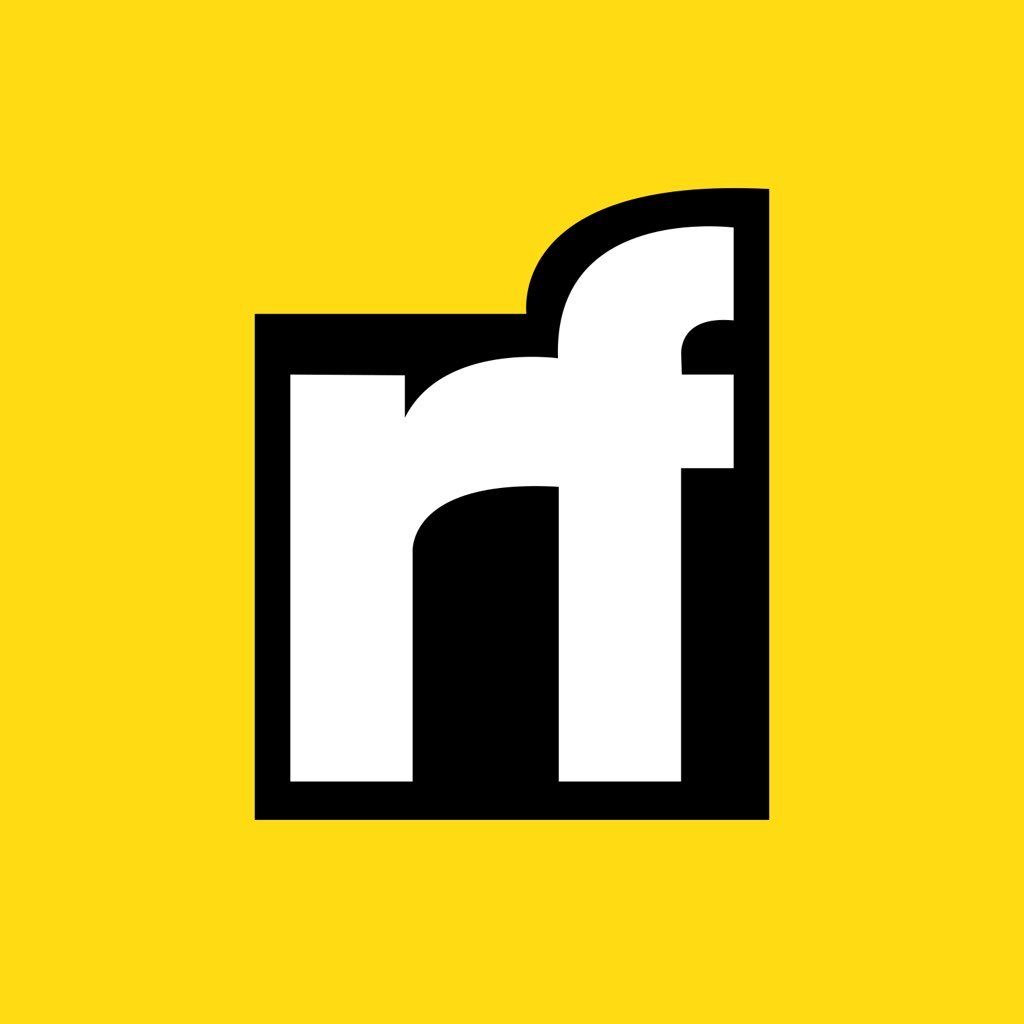
rickavmaniac | Sciencx (2021-02-23T19:45:34+00:00) (Javascript) My learning journey Part 5: Array, Object and loop. Retrieved from https://www.scien.cx/2021/02/23/javascript-my-learning-journey-part-5-array-object-and-loop/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.