This content originally appeared on Bits and Pieces - Medium and was authored by Madushika Perera
Using Immer with React: a Simple Solutions for Immutable States
Why Immer works well for React State Immutability?

In React, using an Immutable state enables quick and cheap comparison of the state tree before and after a change. As a result, each component decides whether to re-rendered or not before performing any costly DOM operations.
And I hope you already know that;
JavaScript is mutable, and we have to implement Immutability ourselves.
Popular state management libraries like Redux also follow the same philosophy. When we use reduces, it expects us not to mutate state to avoid any side effects in the application. However, manually implementing Immutability might not be the best option for large-scale projects where it could become error-prone.
Luckily there are specialized JavaScript libraries like Immer, which enforces Immutability of the state tree by design.
What is Immer and How It Works
Immer is a small library created to help developers with immutable state based on a copy-on-write mechanism, a technique used to implement a copy operation on modifiable resources.
In Immer, there are 3 main states.
- Current State: Actual state data
- Draft State: All the changes will be applied to this state.
- Next State: This state is produced based on the mutations to the draft state.

Immer performs quite well from performance perspectives compared to Shallow copy using object.assign() or Spread operator in JavaScript. If you are interested to know more about performance comparisons, refer the article Immer vs Shallow copy vs Immutable Perf Test to understand the benchmarks.
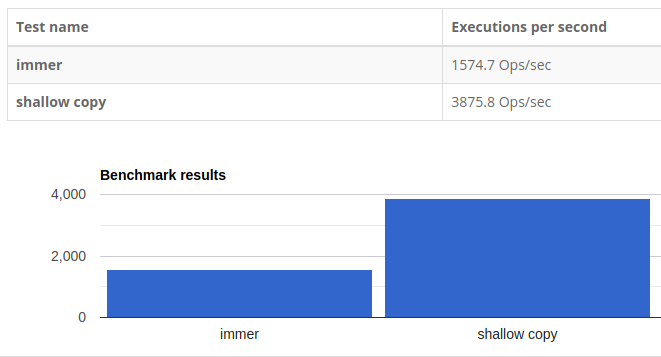
Immer also reduces the amount of code that you need to write to achieve the above benchmark results, and that is one reason why Immer stands out from the rest.
Since you have a basic understanding of Immer, let’s see why Immer is recognized as one of the best solutions for immutability.
Why Immer works well for React State Immutability?
You might get the feeling that Immer is complicating your code if you are working with simple states. But, Immer becomes very useful when it comes to handling more complex data.
To better understand that, let’s consider the famous React reducer example:
export default (state = {}, action) => {
switch (action.type) {
case GET_ITEMS:
return {
...state,
...action.items.reduce((obj, item) => {
obj[item.id] = item
return obj
}, {})
}
default:
return state
}
}
The code above shows a typical reducer of a React-Redux that uses ES6 spread operator to dive into the state tree object’s nested levels to update the values. We can easily reduce the complexity in the above code using Immer.
Let’s take an example how we can use Immer to reduce the complexity in practice.
import produce from "immer"
export default produce((draft, action) => {
switch (action.type) {
case GET_ITEMS:
action.items.forEach(item => {
draft[item.id] = item
})
}
}, {})
In this example, Immer simplifies the code used to spread the state. You can also see that it mutates the object by using a ForEach loop instead of an ES6 reduce function.
Let’s see another example where we could use Immer with React.
import produce from "immer";
this.state={
id: 14,
email: "stewie@familyguy.com",
profile: {
name: "Stewie Griffin",
bio: "You know, the... the novel you've been working on",
age:1
}
}
changeBioAge = () => {
this.setState(prevState => ({
profile: {
...prevState.profile,
age: prevState.profile.age + 1
}
}))
}
This code can be refactored by mutating the state like below
changeBioAge = () => {
this.setState(
produce(draft => {
draft.profile.age += 1
})
)
}
As you can see, Immer has reduced the number of code lines and your code’s complexity drastically.
Tip: Share your React components between projects using Bit (Github).
Bit components are independent modules that can be consumed, maintained, and developed independently. Use them to maximize code resue, keep a consistent design across apps, and collaborate more effectively.
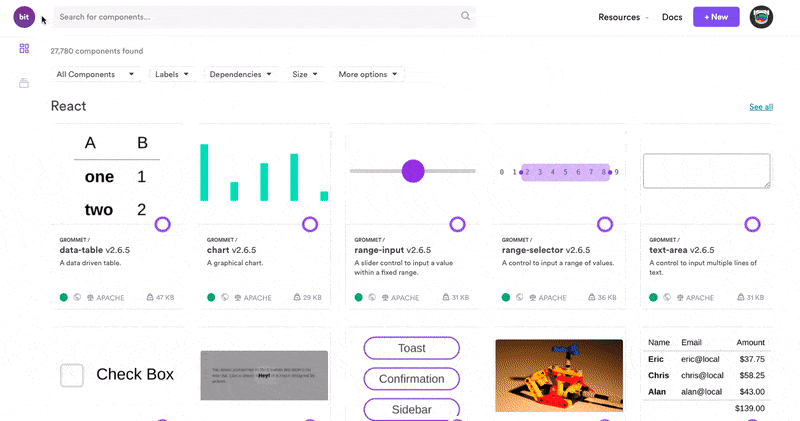
Can We Use it with Hooks?
Another significant feature of Immer is its ability to work with React Hooks. Immer uses an additional library called use-immer to achieve this functionality. Let's consider an example to get a better understanding.
const [state, setState] = useState({
id: 14,
email: "stewie@familyguy.com",
profile: {
name: "Stewie Griffin",
bio: "You know, the... the novel you've been working on",
age:1
}
});
function changeBio(newBio) {
setState(current => ({
...current,
profile: {
...current.profile,
bio: newBio
}
}));
}
We can further simplify the Hooks example by replacing useState with useImmer Hook. And we can also update the React component by mutating the component state.
import { useImmer } from 'use-immer';
const [state, setState] = useImmer({
id: 14,
email: "stewie@familyguy.com",
profile: {
name: "Stewie Griffin",
bio: "You know, the... the novel you've been working on",
age:1
}
});
function changeBio(newBio) {
setState(draft => {
draft.profile.bio = newBio;
});
}
Also, we can use Immer to convert arrays and sets to immutable objects as well. Maps, Sets created through Immer will throw errors when it is mutated, allowing the developers to be aware of the mistake of mutation.
Most importantly, Immer is not limited for React. You can easily use Immer with plain JavaScript as well.
Apart from Immutating, Immer helps maintain well written, readable codebase by reducing your codebase’s complexity.
Final Thoughts
Based on my experience with Immer, I believe it is a great option to use with React. It will simplify your code and help to manage immutability by design.
You can find more information on Immer by referring to their documentation.
Thank you for Reading !!!
Learn More
- Build Scalable React Apps by Sharing UIs and Hooks
- New JSX Enhancements in React 17
- Incremental vs Virtual DOM
Using Immer with React: a simple Solutions for Immutable States was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Madushika Perera
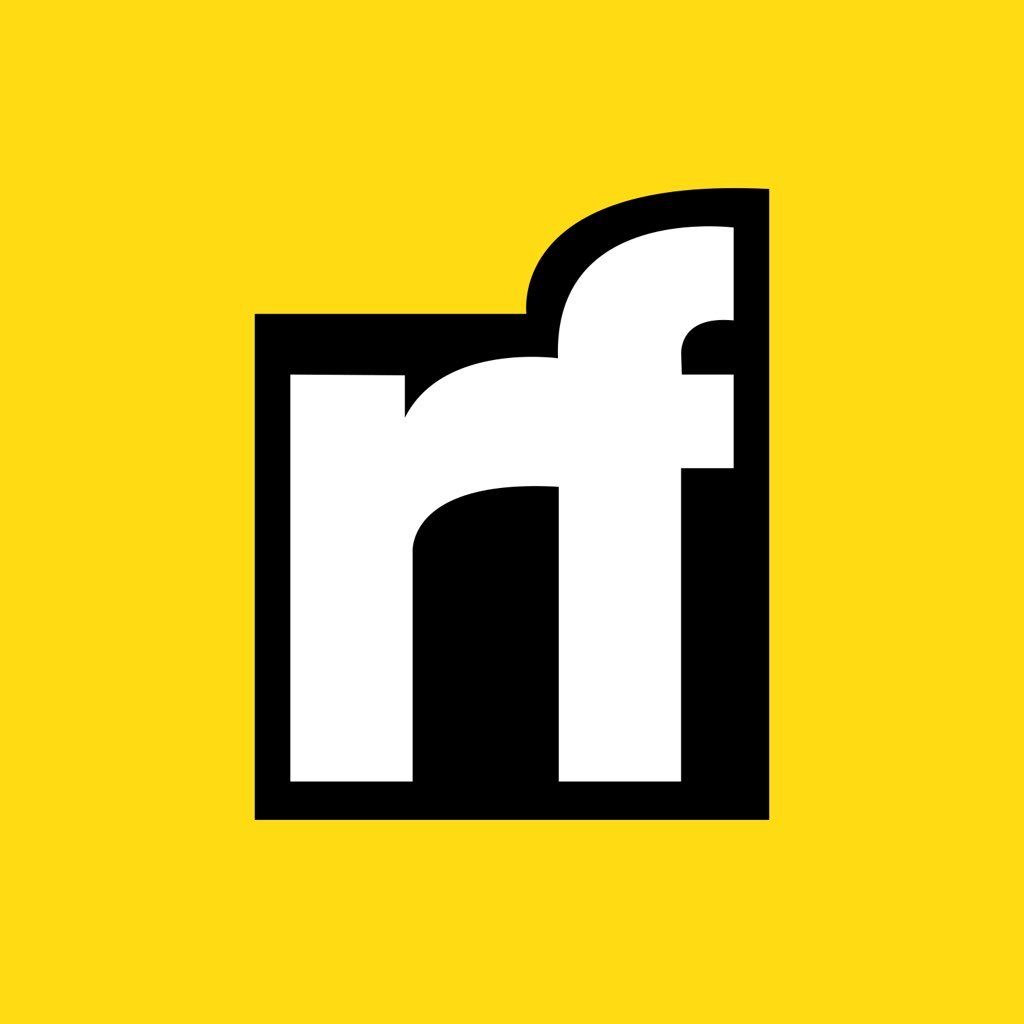
Madushika Perera | Sciencx (2021-02-24T19:14:43+00:00) Using Immer with React: a simple Solutions for Immutable States. Retrieved from https://www.scien.cx/2021/02/24/using-immer-with-react-a-simple-solutions-for-immutable-states/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.