This content originally appeared on DEV Community and was authored by Francisco Inoque
Hello guys, in this post I will talk about the simplest and easiest way to validate form fields according to my point of view, please enjoy.
- First, let's create the validators.js file:
export const myValidators = {
isEmpty (string) {
if(string.trim() === '') {
return true;
} else {
return false
}
},
isEmail(email) {
const emailRegExp = /^(([^<>()[\]\\.,;:\s@\"]+(\.[^<>()[\]\\.,;:\s@\"]+)*)|(\".+\"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/;
if(email.match(emailRegExp)) {
return true;
} else {
return false;
}
},
isLength(string, value) {
if(string.length < value) {
return true
} else {
return false
}
},
}
- And finally, we will create the inputFields.js file:
import { myValidators } from './validators.js'
// TODO: testing isEmail() method
const isEmailMethod = () =>
{
let errors = {};
const email = "jaimeinoque20@gmail.com";
if (!myValidators.isEmail(email))
{
errors.email = "Email Field must be valid!"
} else
{
console.log(email)
}
if(Object.keys(errors).length > 0 ) {
return console.log(errors)
}
}
isEmailMethod()
// TODO: testing isLength method
const isLengthMethod = () =>
{
let errors = {};
const password = "849350920@sofala";
if (myValidators.isLength(password, 9))
{
errors.password = "your password is too short"
} else
{
console.log(password)
}
if(Object.keys(errors).length > 0 ) {
return console.log(errors)
}
}
isLengthMethod()
// TODO: testing isEmpty method
const isEmptyMethod = () =>
{
let errors = {};
const phone = "849350920";
if (myValidators.isEmpty(phone))
{
errors.phone = "Phone Field must not be empty!"
} else
{
console.log(phone)
}
if(Object.keys(errors).length > 0 ) {
return console.log(errors)
}
}
isEmptyMethod()
Thank you all
This content originally appeared on DEV Community and was authored by Francisco Inoque
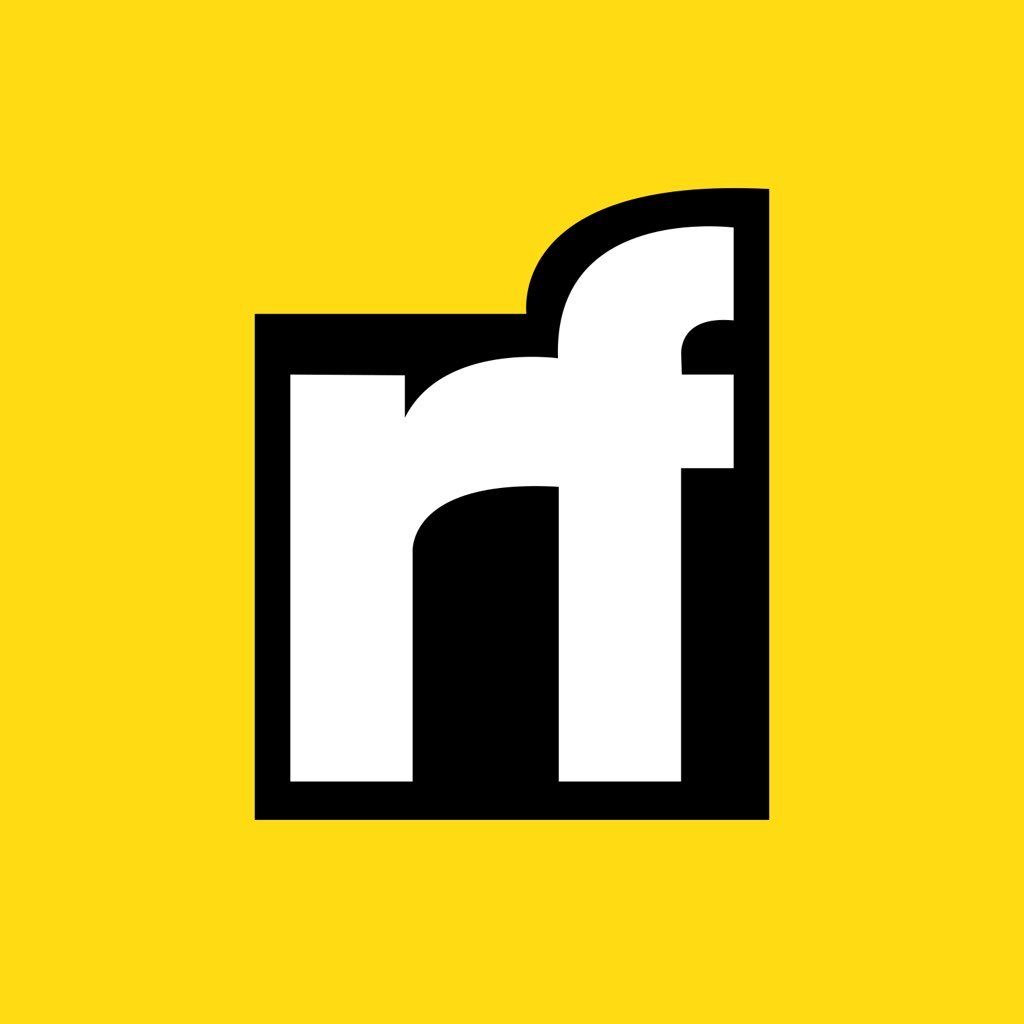
Francisco Inoque | Sciencx (2021-04-17T16:50:18+00:00) A simple and easy way to validate form fields. Retrieved from https://www.scien.cx/2021/04/17/a-simple-and-easy-way-to-validate-form-fields/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.