This content originally appeared on DEV Community and was authored by Tapajyoti Bose
I came across the hover effect in the cover image at several sites, but just could not wrap my head around how to develop it. Thankfully after reading this blog, I finally got an idea on how to develop it.
Demo
Getting Started
Let's start coding!
index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Hover Effect</title>
<link rel="stylesheet" href="style.css" />
</head>
<body>
<div class="hover-container">
<img
src="https://images.unsplash.com/photo-1469474968028-56623f02e42e?auto=format&fit=crop&w=1953&q=80"
class="img"
/>
<div class="overlay"></div>
</div>
<script src="script.js"></script>
</body>
</html>
style.css
.hover-container {
height: max-content;
width: max-content;
position: relative;
margin: 12px 24px;
}
.img {
display: inline-block;
height: 200px;
}
.overlay,
.hover-container::after {
position: absolute;
top: 0;
bottom: 0;
left: 0;
right: 0;
}
.hover-container::after {
content: "";
}
NOTE: Without the ::after
pseudo-element
hovering the hover-container
would trigger .overlay:hover
instead of .hover-container:hover
Now to the meat of the hover effect. We would be adding the effect to all elements with hover-container
class. First the mouse position over the element will be tracked and the element would be styled accordingly (on mousemove
)
script.js
document.querySelectorAll(".hover-container").forEach((container) => {
// reseting styles for the element when the mouse exits the element
container.onmouseleave = (e) => {
const overlayChild = e.target.querySelector(".overlay");
e.target.style.transform = "rotateY(0) rotateX(0)";
overlayChild.style.background = "transparent";
};
// adding a listener to style the element when the mouse moves inside the element
container.addEventListener("mousemove", (e) => {
const rect = e.target.getBoundingClientRect();
const x = e.clientX - rect.left; //x position within the element.
const y = e.clientY - rect.top; //y position within the element.
const width = e.target.offsetWidth;
const height = e.target.offsetHeight;
const overlayChild = e.target.querySelector(".overlay");
// the values can be tweaked as per personal requirements
e.target.style.transform = `rotateY(${-(0.5 - x / width) * 30
}deg) rotateX(${(y / height - 0.5) * 30}deg)`;
overlayChild.style.background = `radial-gradient(
circle at ${x}px ${y}px,
rgba(255, 255, 255, 0.2),
rgba(0, 0, 0, 0.2)
)`;
});
})
NOTE: This is a simpler version of the effect shown in the cover image, with a few minor tweaks, that effect can be obtained.
Reference
Thanks for reading
Checkout my projects:
ruppysuppy
/
SmartsApp
?? An End to End Encrypted Cross Platform messenger app.
Smartsapp
A fully cross-platform messenger app with End to End Encryption (E2EE).
Demo
NOTE: The features shown in the demo is not exhaustive. Only the core features are showcased in the demo.
Platforms Supported
- Desktop: Windows, Linux, MacOS
- Mobile: Android, iOS
- Website: Any device with a browser
Back-end Setup
The back-end of the app is handled by Firebase
.
Basic Setup
- Go to firebase console and create a new project with the name
Smartsapp
- Enable
Google Analylitics
App Setup
- Create an
App
for the project from the overview page - Copy and paste the configurations in the required location (given in the readme of the respective apps)
Auth Setup
- Go to the project
Authentication
section - Select
Sign-in method
tab - Enable
Email/Password
andGoogle
sign in
Firestore Setup
- Go to the project
Firestore
section - Create firestore provisions for the project (choose the server nearest to your location)
- Go to the
Rules
…
ruppysuppy
/
Daily-Coding-Problem-Solutions
??️ Solutions for 350+ Interview Questions asked at FAANG and other top tech companies
Daily Coding Problem Solutions
This repository contains the Daily Coding Problem solutions in python.
Setup
Install the 3rd party packages, perform the following steps:
cd Daily-Coding-Problem-Solutions
- Run
pip install -r requirements.txt
Contribution Guidelines
Please go through Contribution Guidelines before you contribute.
Note:
- Modules
numpy
andmatplotlib
are used for presentation purpose only and hence are optional. - Some solutions require an additional Data Structures module.
Problems
Problem 1
Given a list of numbers, return whether any two sums to k For example, given [10, 15, 3, 7] and k of 17, return true since 10 + 7 is 17.
Bonus: Can you do this in one pass?
Problem 2
This problem was asked by Uber.
Given an array of integers, return a new array such that each element at index i of the new array is the product of all the numbers in the original array except the one at…
Reach out to me on
This content originally appeared on DEV Community and was authored by Tapajyoti Bose
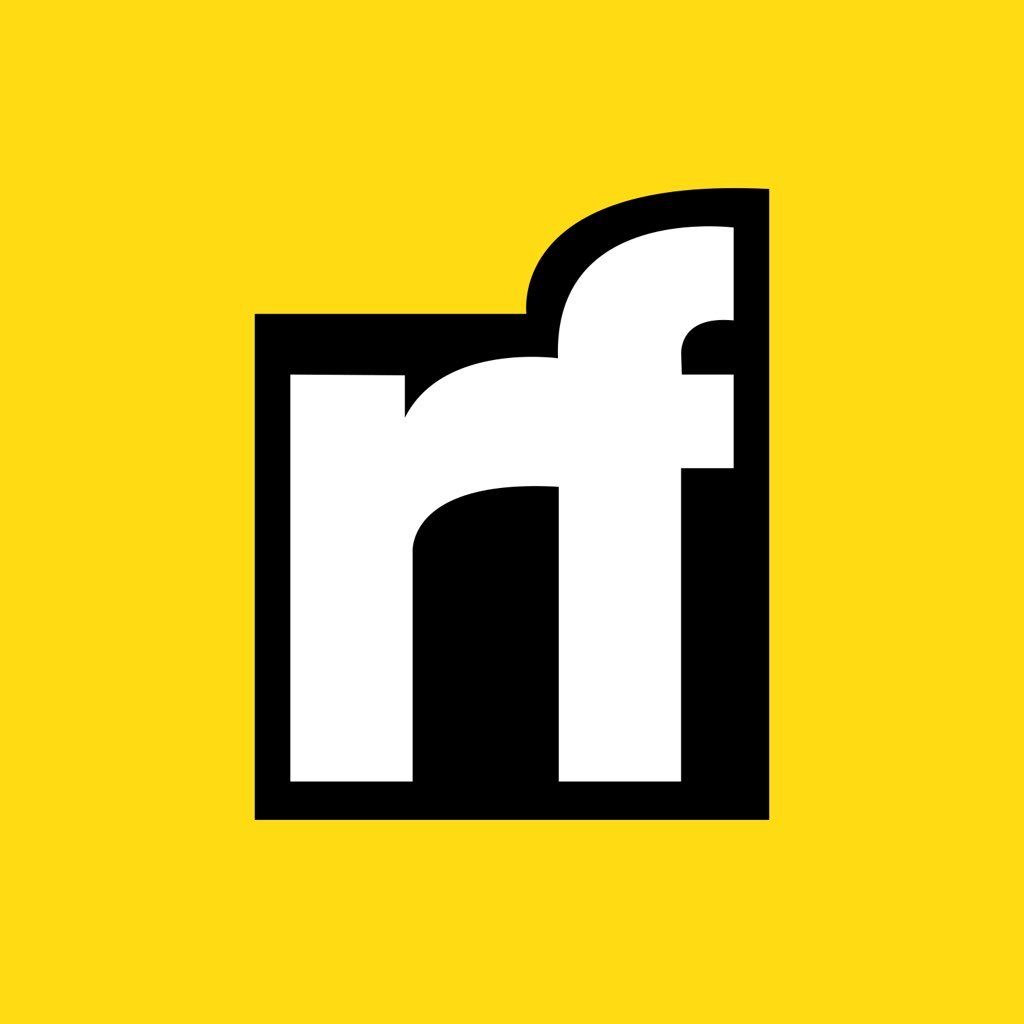
Tapajyoti Bose | Sciencx (2021-05-02T04:26:45+00:00) Advanced Hover Effect to WOW your visitors!. Retrieved from https://www.scien.cx/2021/05/02/advanced-hover-effect-to-wow-your-visitors/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.