This content originally appeared on DEV Community and was authored by Bibek
Hey coders ?
If you are a JavaScript developer, I am pretty much sure that you have used the npm package UUID
at least once in your development journey.
For those who don't know what is
UUID
. It is an npm package to generate a unique string ID. Similar packages are available in other languages too.
But in this article I am not going to discuss UUID
, rather I will discuss another awesome npm package to generate a unique ID known as NanoID
.
What is NanoID?
A tiny, secure, URL-friendly, unique string ID generator for JavaScript.
Why NanoID?
- It is smaller in size as it has no dependencies.
- It is 60% faster than UUID.
- It uses cryptographically strong random APIs.
- It uses a larger alphabet than UUID (A-Za-z0-9_-).
We can control the behaviour of alphabets to be used.
NanoID is available in almost all the most used programming languages.
Disclaimer: All the above claims are picked from the package's docs itself. ?
Implementation
It is very easy to implement. Will write the code in a Node.js environment using CommonJS import.
Basic Way
It will generate the ID synchronously.
// Importing
const { nanoid } = require("nanoid");
// It will generate and return an ID with 21 characters
const id = nanoid();
Async way
It will generate the ID asynchronously.
// Importing async API
const { nanoid } = require("nanoid/async");
// It will generate and return an ID with 21 characters
const id = await nanoid();
Custom Size
You can also pass the required ID's size as an argument.
// Importing
const { nanoid } = require("nanoid");
// It will generate and return an ID with 10 characters
const id = nanoid(10);
Reducing the size will increase the collisions probability.
Non-Secure
If you want performance and not concerned with security, then you can use the non-secure way.
// Importing non-secure API
const { nanoid } = require("nanoid/non-secure");
const id = nanoid();
Custom Character or Size
You can control what characters you want to be included in your ID.
// Importing customAlphabet API
const { customAlphabet } = require("nanoid");
// First Param: Characters
// Second Param: ID size
const nanoid = customAlphabet("123456789qwerty", 8);
// Generated ID would be like: "q15y6e9r"
const id = nanoid();
You can also use the customAlphabet
with async way
and non-secure way
.
// Importing async API
const { customAlphabet} = require("nanoid/async");
// Importing non-secure API
const { customAlphabet} = require("nanoid/non-secure");
You can also check for the ID collision probability here.
Thank you for reading ?
If you enjoyed this article or found it helpful, give it a thumbs-up ?
Feel free to connect ?
Twitter | Instagram | LinkedIn
This content originally appeared on DEV Community and was authored by Bibek
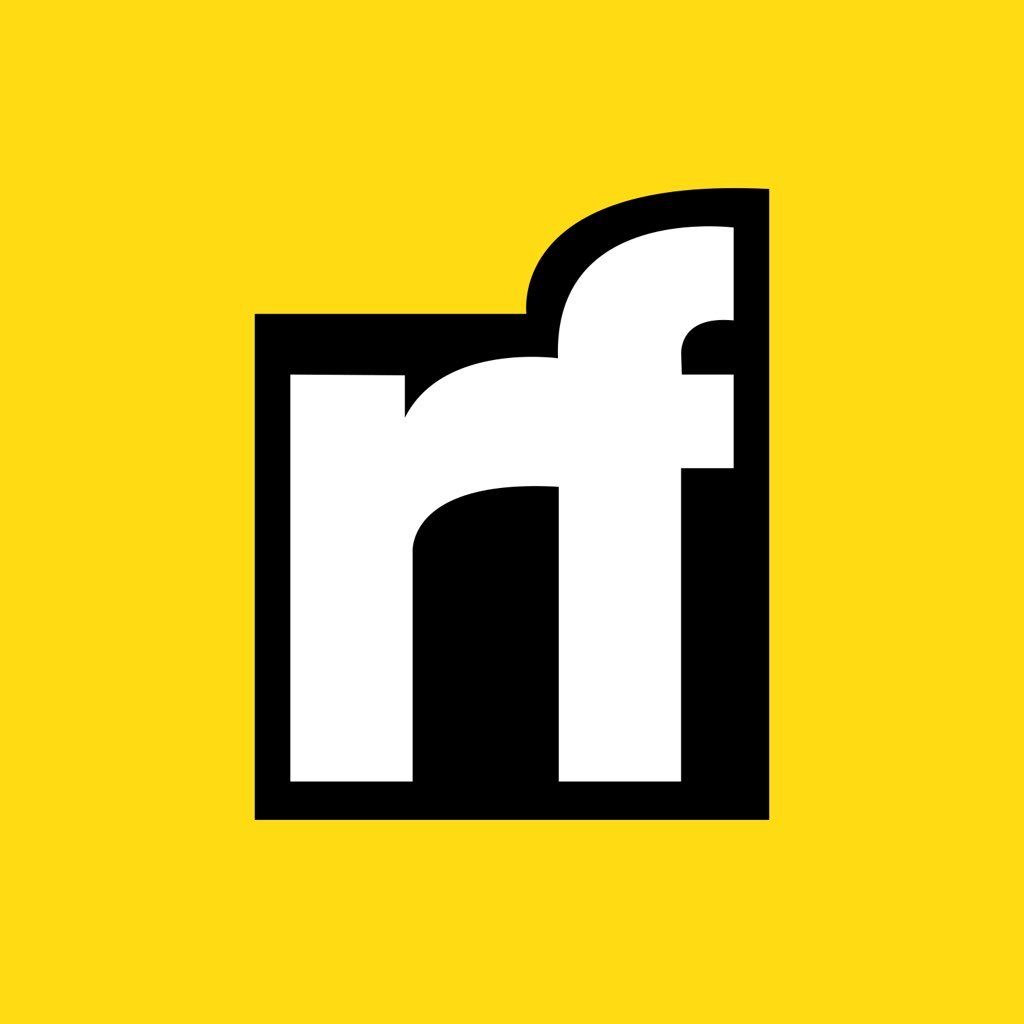
Bibek | Sciencx (2021-05-23T16:15:46+00:00) NanoID – Alternative To UUID. Retrieved from https://www.scien.cx/2021/05/23/nanoid-alternative-to-uuid/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.