This content originally appeared on flaviocopes.com and was authored by flaviocopes.com
This tutorial belongs to the Swift series
A protocol is a way to have different objects, of different types, have a common set of functionality.
A protocol is defined in this way:
protocol Mammal {
}
Structs and classes can adopt a protocol in this way:
struct Dog: Mammal {
}
class Cat: Mammal {
}
A protocol can define properties and methods, without providing values and implementations, and a struct/class must implement them:
protocol Mammal {
var age: Int { get set }
func walk()
}
The property can be defined as get
or get set
. If it’s get
, the property must be implemented as read only, with a getter.
Any type that adopts the protocol must conform to the protocol by implementing those methods or providing those properties:
struct Dog: Mammal {
var age: Int = 0
func walk() {
print("The dog is walking")
}
}
class Cat: Mammal {
var age: Int = 0
func walk() {
print("The cat is walking")
}
}
Structs and classes can adopt multiple protocols:
struct Dog: Mammal, Animal {
}
class Cat: Mammal, Animal {
}
Notice that for classes, this is the same syntax used to define a superclass. If there is a superclass, list it as the first item in the list, after the colon.
This content originally appeared on flaviocopes.com and was authored by flaviocopes.com
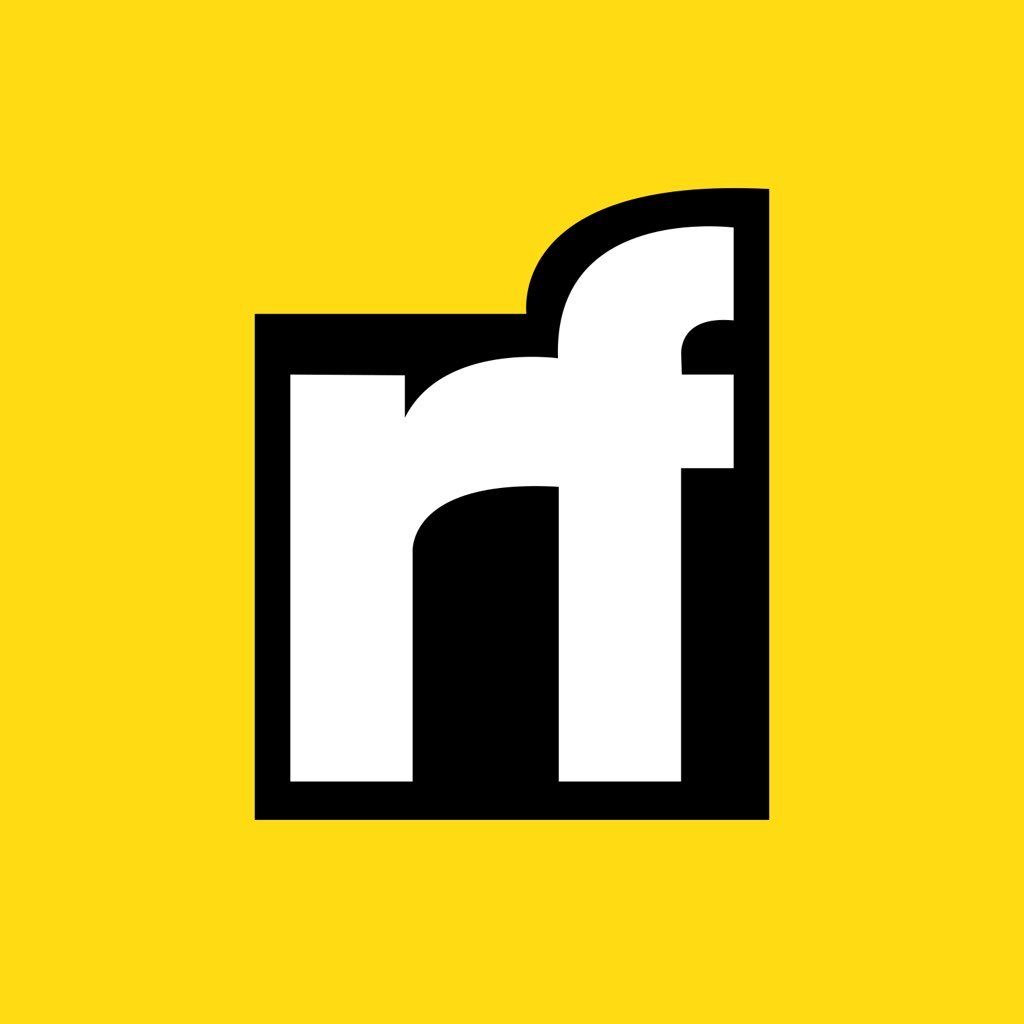
flaviocopes.com | Sciencx (2021-06-12T05:00:00+00:00) Swift Protocols. Retrieved from https://www.scien.cx/2021/06/12/swift-protocols/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.