This content originally appeared on Bits and Pieces - Medium and was authored by Debbie O'Brien
We have almost all been in a situation where we need to share a component between one app and another. Often we just decide to copy and paste it because it really is the quickest option.
And sometimes it’s even ok, especially when it comes to those smaller components. But what if you wanted to share a big and more complex component? Take a booking engine for example.

You may have 2 apps that can book hotels and therefore require this component. You now have a few options. Copy and pasting works of course but any change you make in one you now have to repeat that work and do it again and let’s face it we all hate doing repetitive work.
Our time is much more valuable than that. So next option is to package it up and send it to npm. This is not a bad option at all. Both apps can install the package and use it and you only have to modify it once. Game over, happy days.
So what’s the problem with this option? The booking engine component is made up of several small components such as search input, calendar, button, input fields and so much more. These small components are now tightly coupled to this one big component.
If we change anything in our design system to give a new look and feel to our input components across our site we then need to update the booking engine too. Repetitive work again. But not just that, we have to also remember to do it as it is a very manual process. Now what if we had a contact form that we were also sharing between apps.
We would also have to manually update that too. We could just tell the designer to not make any changes, that would certainly be a great solution for us. But seriously all this updating, releasing new packages and managing all this is not easy and just not fun at all.
OK, there is always another solution. We could just move everything into a monorepo, one repo that holds several apps, and then we just have to modify the code once as all the form elements could be locally imported into the more complex components. It’s a great solution and will work pretty well.
Now what happens when your company scales and all of a sudden you now have 12 other brands that have just been created that need to use this booking engine? You are probably thinking, yeah that's probably never going to happen. And that’s what I thought until I was actually in that situation and had to provide a solution.
Now there are 13 apps inside my monorepo and build times are through the roof and every developer working on any of the projects is all sharing the same repo. Now this can be a good thing and some people like it but it can also be a nightmare.
We could use tools like NX, which will not rebuild everything on every comment, it only rebuilds what is necessary so that would help build times. Or we could use Learna which will optimize the workflow around managing multi-package repositories although we will have to manage a package.json for each of these packages.
There has to be a better solution. All we want is to be able to share our booking engine component across all our apps. We want it to consume smaller components that should be independent to our booking engine. We want to be able to update our components across our site and they will also update in our booking engine.
We don’t want to do repetitive tasks yet we want to keep everything in sync. We want to be able to work independently from other teams and brands using these components. Are we really asking for too much?
This is where Bit comes in. Bit is like git but for components.
Bit is a free and distributed version control system for components and dependencies. It helps build component-driven apps in a simple and scalable way. We can manage our components as individual building blocks which are created and tested in isolation.
These components can then be easily versioned and exported so they can be consumed in any application using npm or yarn. Bit takes care of all the versioning process so you don’t have to. As a developer you just want to build code and not have to worry about things you don’t want to worry about.
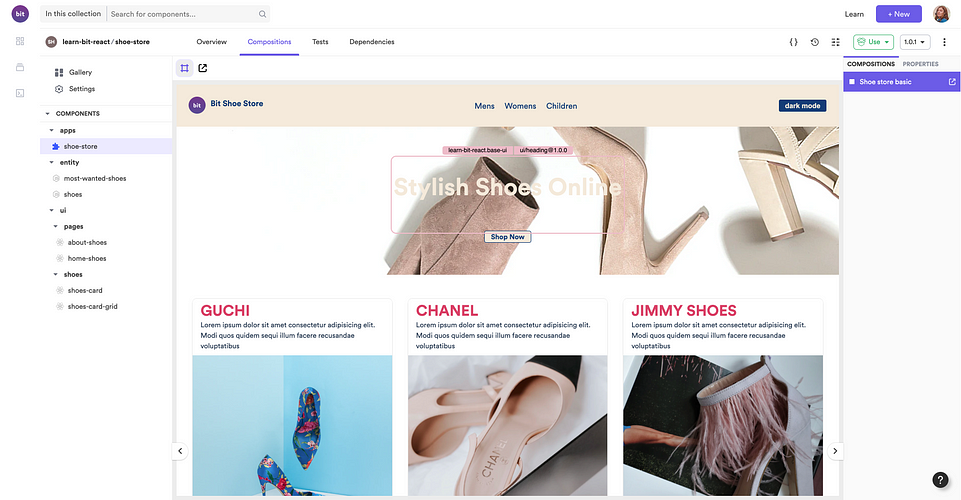
In the shoe store example above, built in a Bit workspace, you can see that the app does not contain any of the smaller components yet it uses them to build the more complex components. These smaller components such as buttons, headings, styles, entity types and mock data are actually used to create this app as well as many others. Just like how we wanted with our booking engine.
Ok seems like a good solution. But isn’t it the same as just adding all your components as individual npm packages you might ask? Well not really no. Bit not only takes care of the versioning of components for you but also the dependency management.
What that means is that if I make a change to my input or my button component Bit knows which components are composed of these smaller components. In our case it is the booking engine and contact form. Bit will then create a new version of these components too with the updated input or button component. That means we don’t have to even think about or remember which components depend on other components.
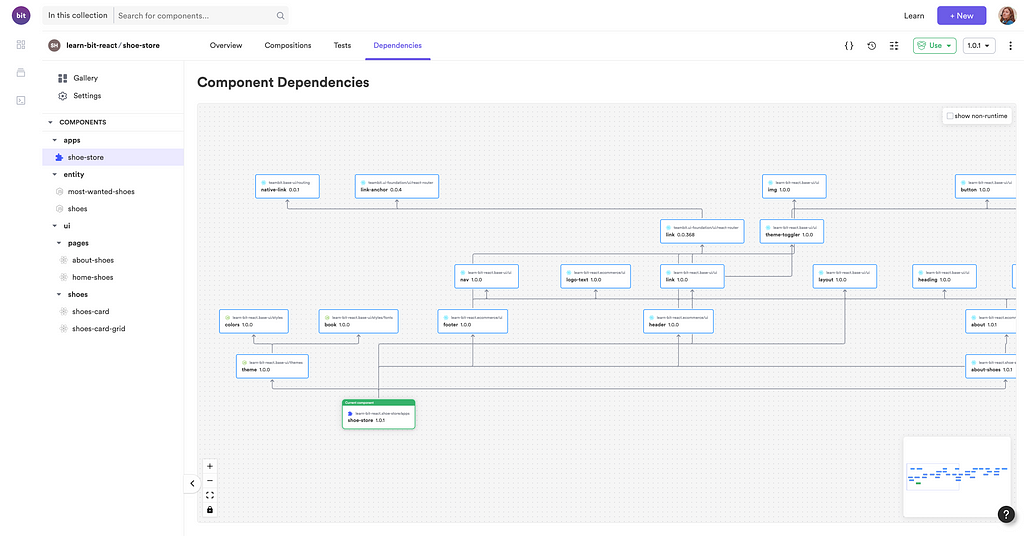
With Bit we can build our components in isolation, we can test our components in isolation. We can compose components from smaller components. We can version and publish these components and then consume these components across all our apps.
We can make changes to components and know that Bit will take care of updating any components that are using the changed component. This means we can just focus on building cool things.
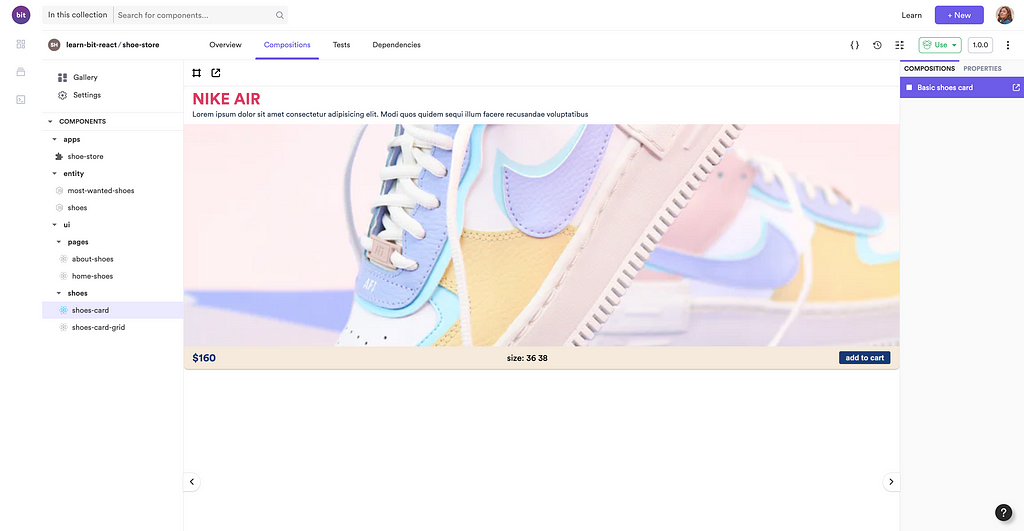
So what’s the catch? Well, you have to start building thinking in Component driven development. That means building components in isolation instead of building components that are tightly coupled together.
It means building components that have no relative links to other components. Luckily Bit helps you with this as it doesn’t allow relative imports and you therefore build your components with import links as if you were already linking to a package in your node modules folder, that’s cause you kinda are doing just that.
Bit gives you a workspace so you can build and test components in isolation complete with it’s own dev server and Hot Module Replacement. Each component has its own docs, compositions and test files.
Bit is 100% open source so it’s free to use. You can host your components yourself or take advantage of Bit’s cloud service. The choice is yours.
So how does it all work? I created a tutorial on how to get a component up and running in bit, consume it in another component, version and export it and then install it with Yarn in a Create React App. Don’t worry it doesn’t take long at all as we have built generator tools to get you setup quickly.
You can generate a basic component so you can test out what it is like to work and build with Bit and then later worry about making your components more useful and real.
If you don’t like reading then you can watch the video and code along instead.
Although we are a TypeScript first platform, if typescript still intimidates you or you simply just don’t want to use it then just choose the JavaScript version of the component and you can work without TypeScript. And of course if you are not a frontend developer and still want to try out Bit then feel free to create Node.js components instead.
Actually, the default environment for Bit is Node.js.
If you have any doubts or questions about Bit I would be happy to answer them so just reach out on twitter or better still join our slack channel and ask any of your technical questions there or just say hi. And remember Bit is open source so collaboration is welcome. Submit an issue or pr ;) Have fun and I look forward to seeing the cool things you will build with Bit.
Learn more
Sharing React Components across Multiple Applications was originally published in Bits and Pieces on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Bits and Pieces - Medium and was authored by Debbie O'Brien
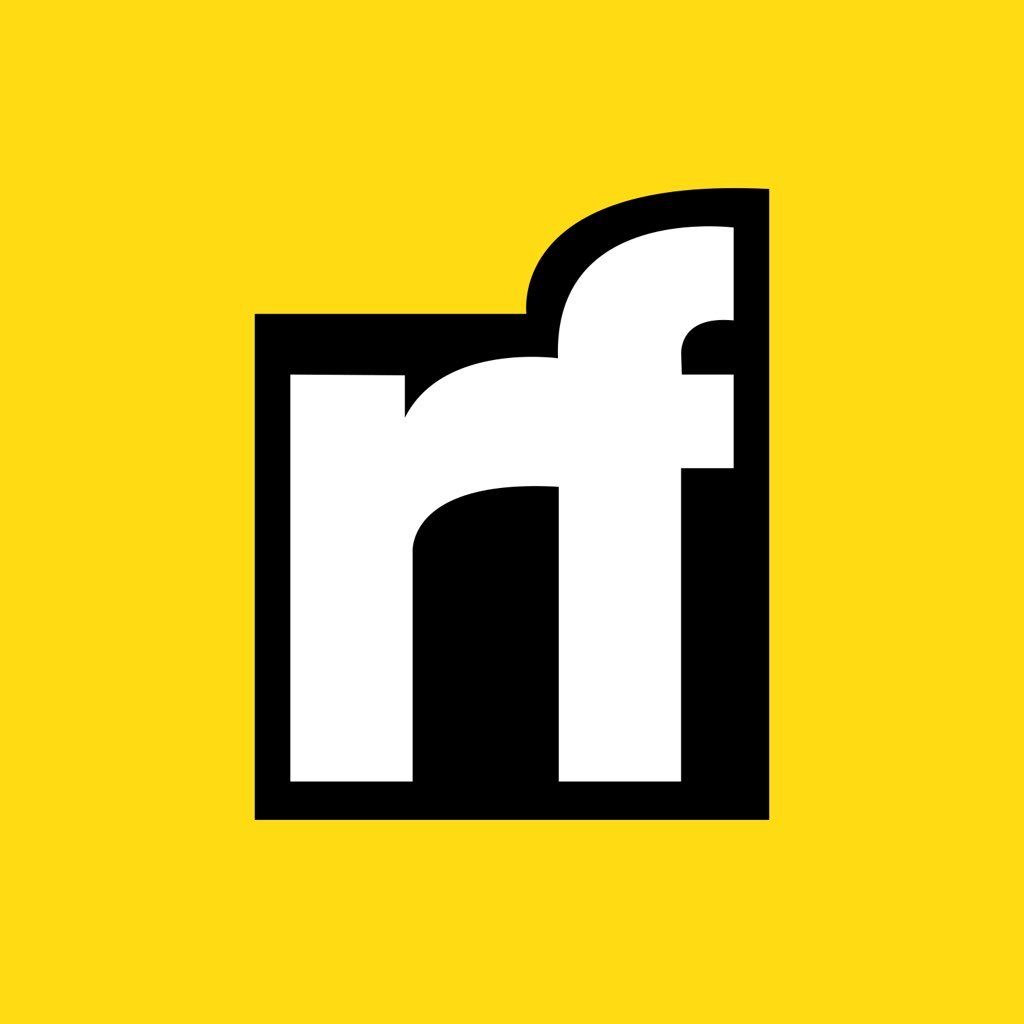
Debbie O'Brien | Sciencx (2021-06-16T16:04:53+00:00) Sharing React Components across Multiple Applications. Retrieved from https://www.scien.cx/2021/06/16/sharing-react-components-across-multiple-applications/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.