This content originally appeared on Level Up Coding - Medium and was authored by Himayan Debnath
TECH
Not even a decade ago, JavaScript used to be just a front-end tool to make websites interactive and was actively used only by a small community of developers. However, a lot has changed since then.
The introduction of NodeJS, and the server-side solutions that come with it, along with the adoption of build tools like Webpack or Grunt made the prospect of learning JavaScript so immensely popular that it quickly became one of the most in-demand languages in the world.
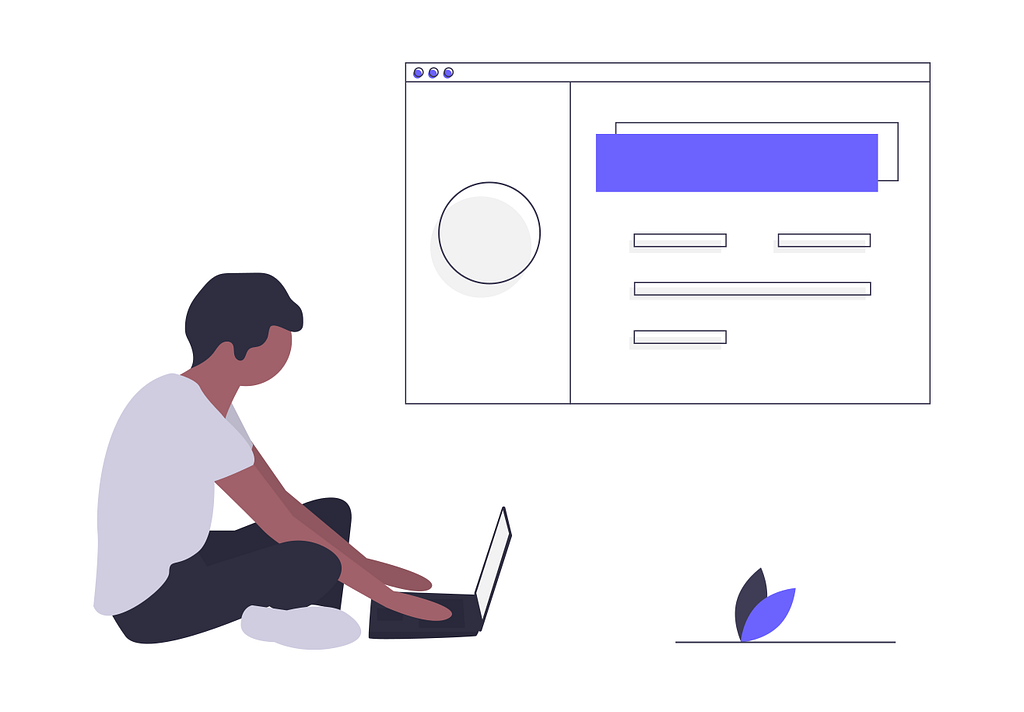
However, the rapid rise in popularity of JavaScript called for improved syntax and maintainable, easily understandable code. And not long after — ES6 arrived, a new look JavaScript which is graceful and compact in the way it is written.
A few more JavaScript versions have released since then, latest being the ES2020, adding a lot more developer-friendly features to the language. This article would try to focus on 10 such modern JavaScript syntax, which are useful, easy to adapt and do not need deep knowledge of the concept introduced.
1. String Padding
String.prototype.padEnd()
This method would append the current string with another given string, multiple times if required, until it reaches a particular length. Like in the example below, the hmm string will be appended with . multiple times, till the length of the final string is 6.
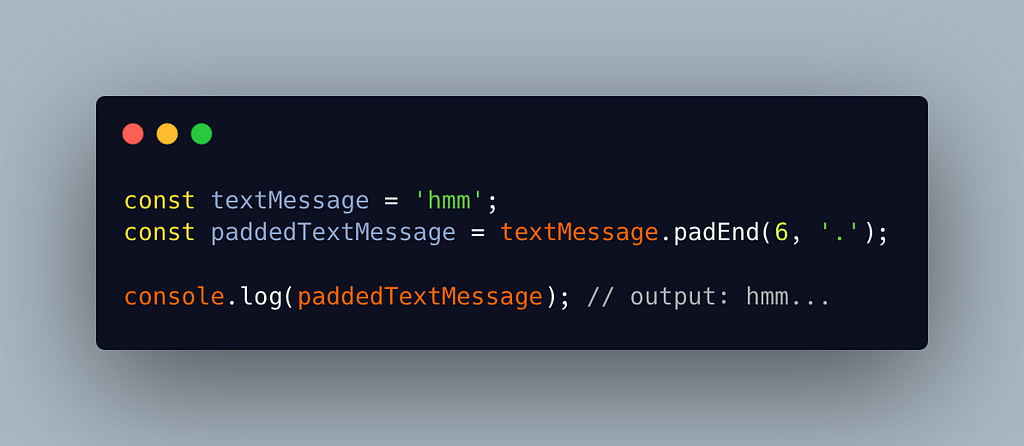
String.prototype.padStart()
This is exactly similar to String.prototype.padEnd(), other than the fact that now the string will be appended from the front, and not the end.
A popular scenario where this comes in handy, is for masking a phone number or an email address. In payment gateways or secure portals, it is very common to hide the contact information and show the last 3–4 characters, just enough for users to identify if it is meant for them.
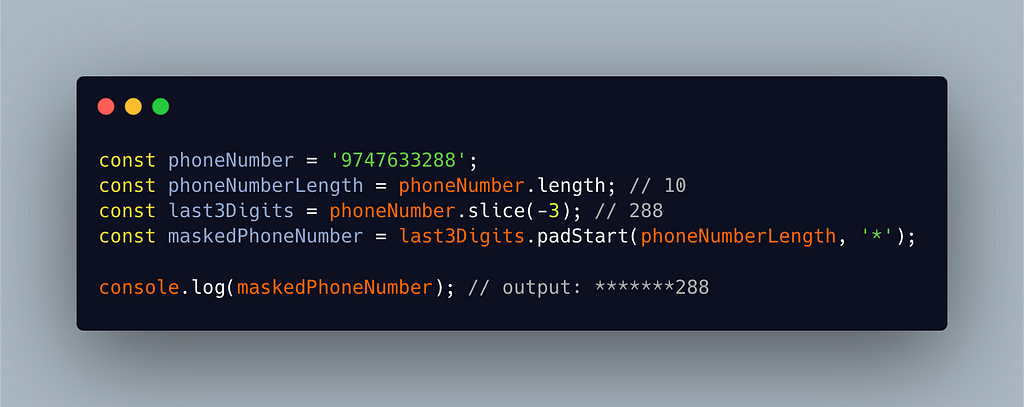
2. Array.prototype.includes()
The includes method will return true, if the element exists in the given array. If the method is supplied with a second argument fromIndex, the array will then be searched from that given index —
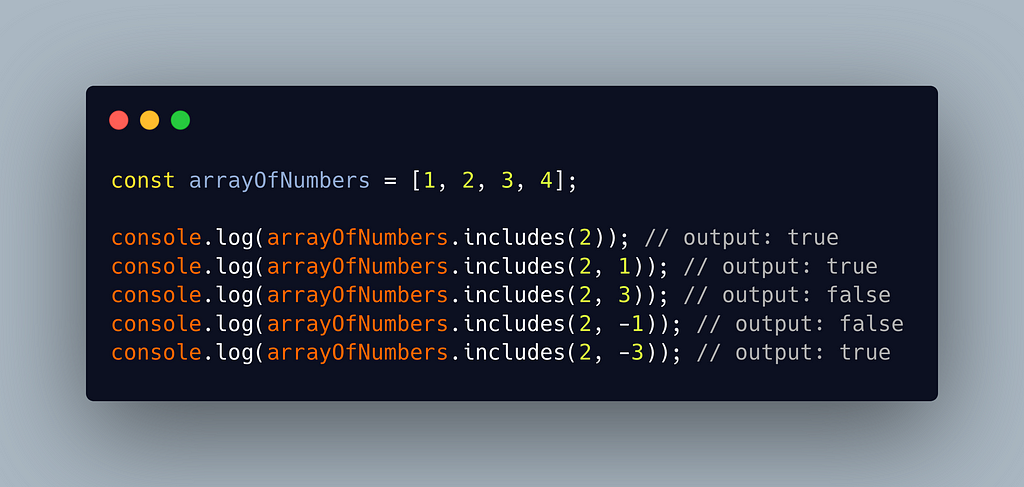
3. Rest parameters & Spread syntax
Rest parameters
Rest parameters are particularly useful when we are trying to create a function with indefinite number of arguments. Adding a ... in front of the name of a parameter during function definition, will create an array which will collect all the other arguments. Perhaps, the following example will make things clearer —
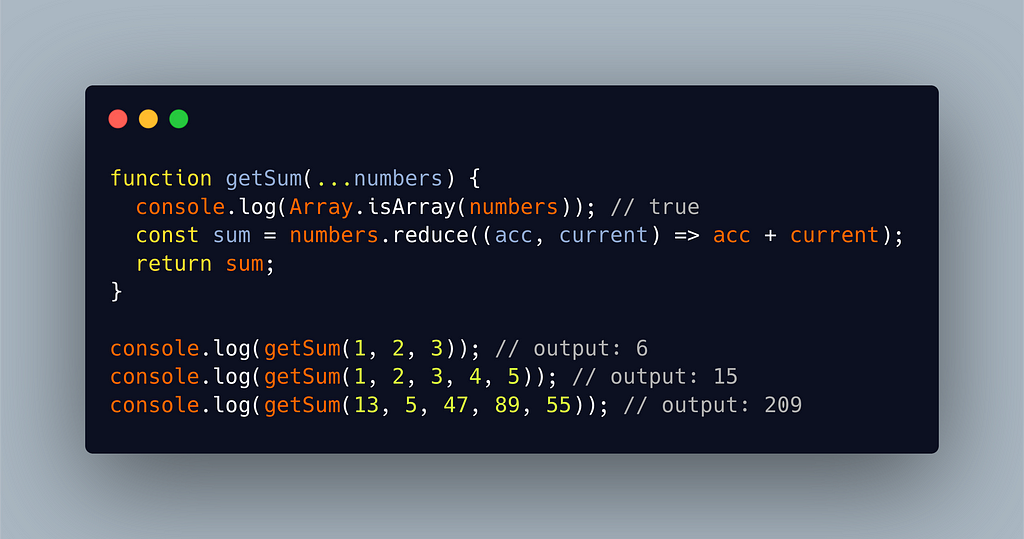
We can also choose to define the first few parameters, and let the rest of the arguments get collected in the array. But, it can only be done from the front, and not the other way around. In other words, only the last parameter can be a rest parameter —
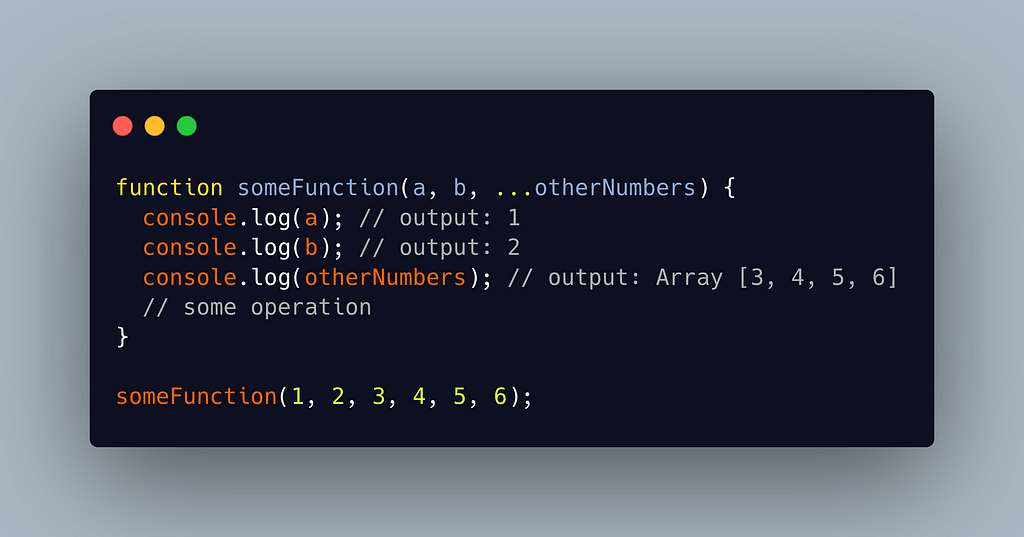
Spread Syntax
By the look of it, both rest and spread seem to be the same thing, but is quite the opposite. The syntax (i.e. ...), might look similar, but the way they are used is what differentiates them.
In a function call — if we pass an array with the spread syntax, it will essentially expand it into a list of arguments. Let us consider the getSum() example above, which takes in an indefinite number of arguments. If we have an array of numbers, and we would want to re-use that method, this is how we would do it—
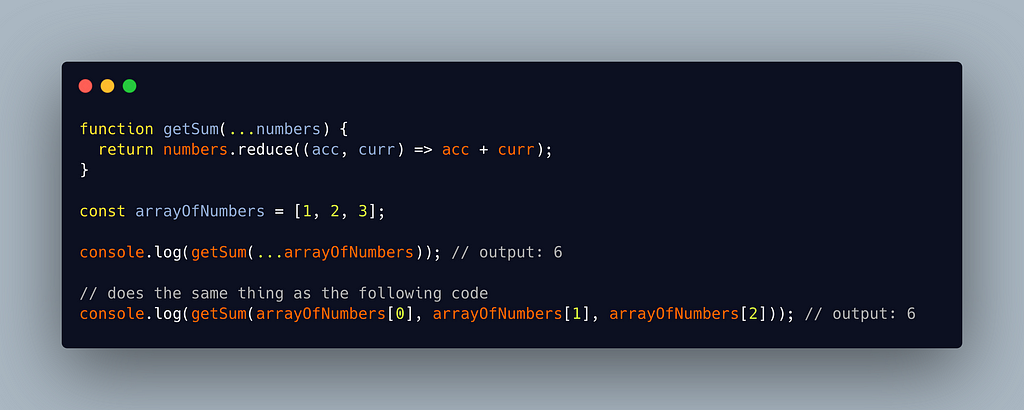
To shallow copy — we can use spread syntax, and any further operation on the cloned one will not affect the original array. This feature is also very useful in case of objects, but more on that later.
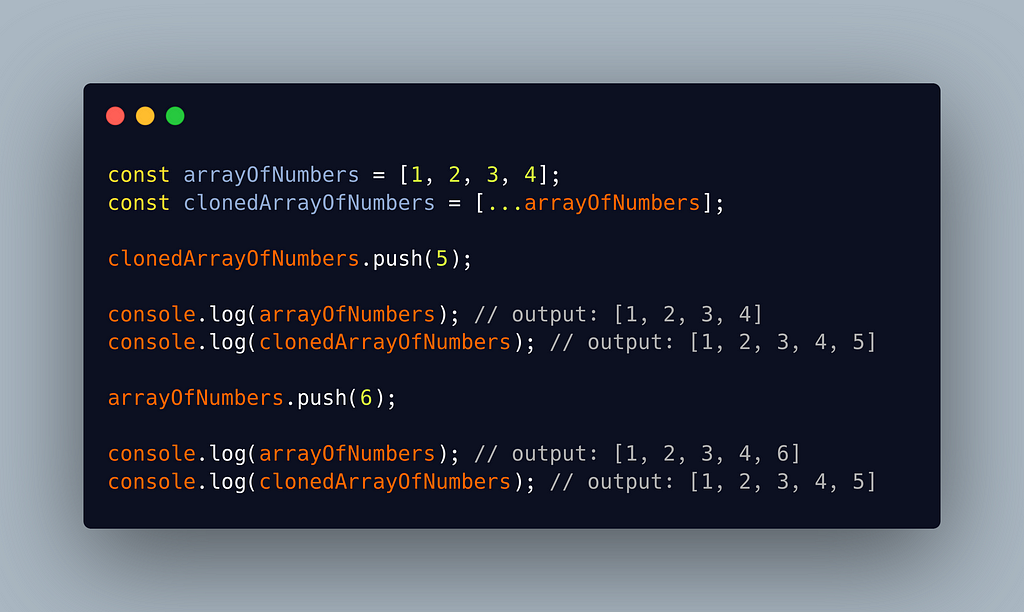
Array operations — Spread syntax provides an easy alternative to handle basic array operations like concatenation, adding elements at different positions and so on. This can be better explained with an example—
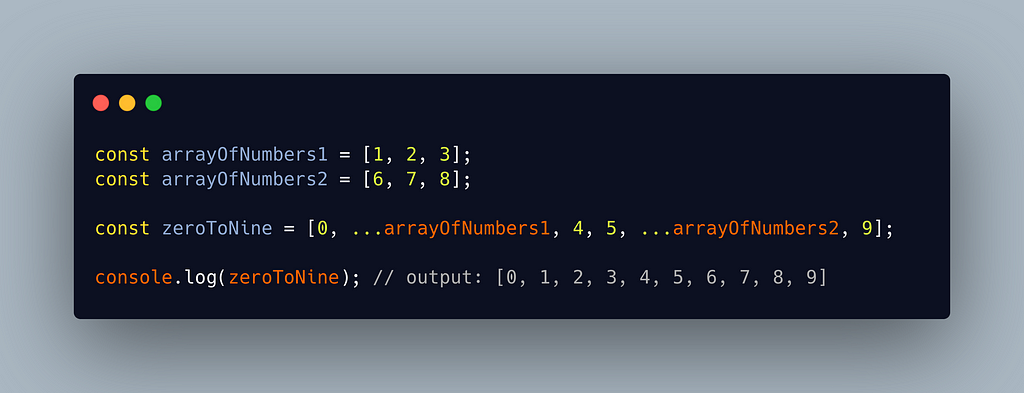
Moving on to objects — Spread / Rest properties got introduced in ES2018. It works very similar to the ones discussed before, with minor tweaks here and there. Here are a few examples of how to make use of it —
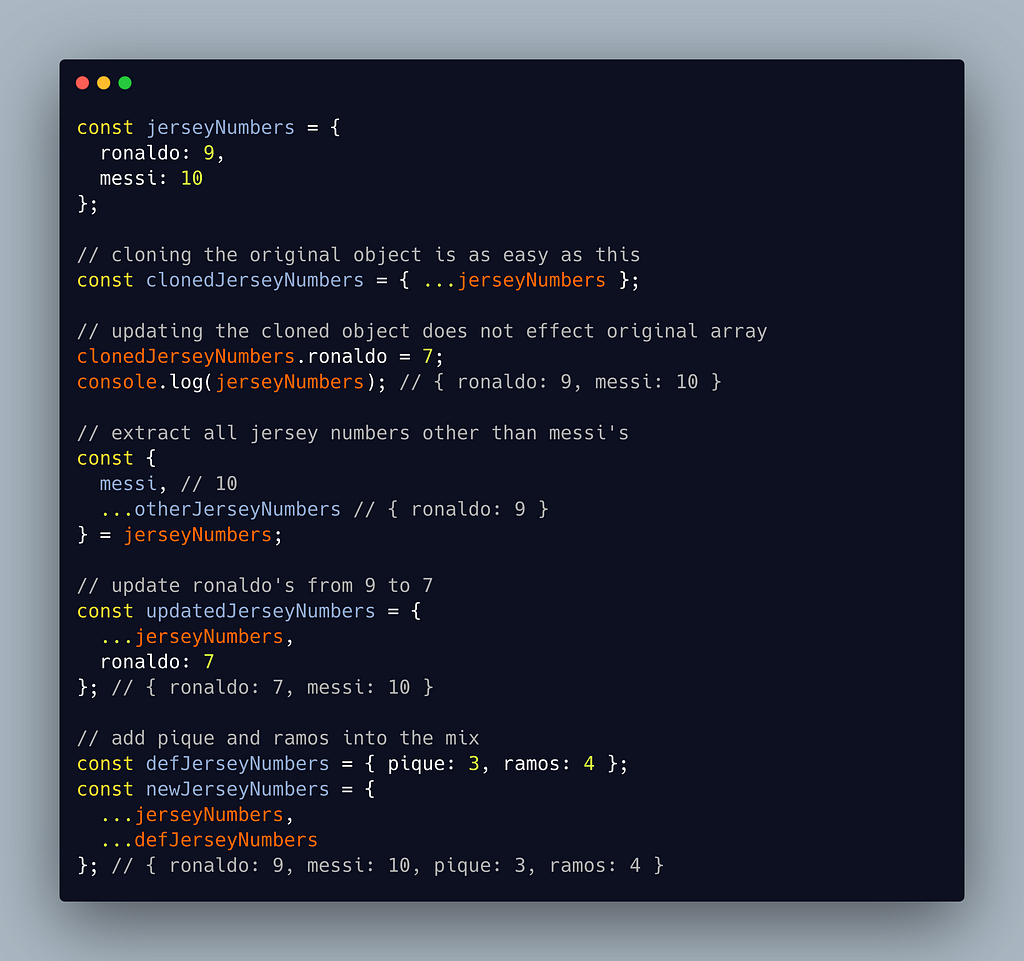
4. Object Iterators
Keys, values and entries —
Object.keys() returns an array of all the keys of an object, and similarly Object.values() returns an array of all the values. Object.entries(), on the other hand, returns an array of [key, value] pairs. As always, examples speak more than words—
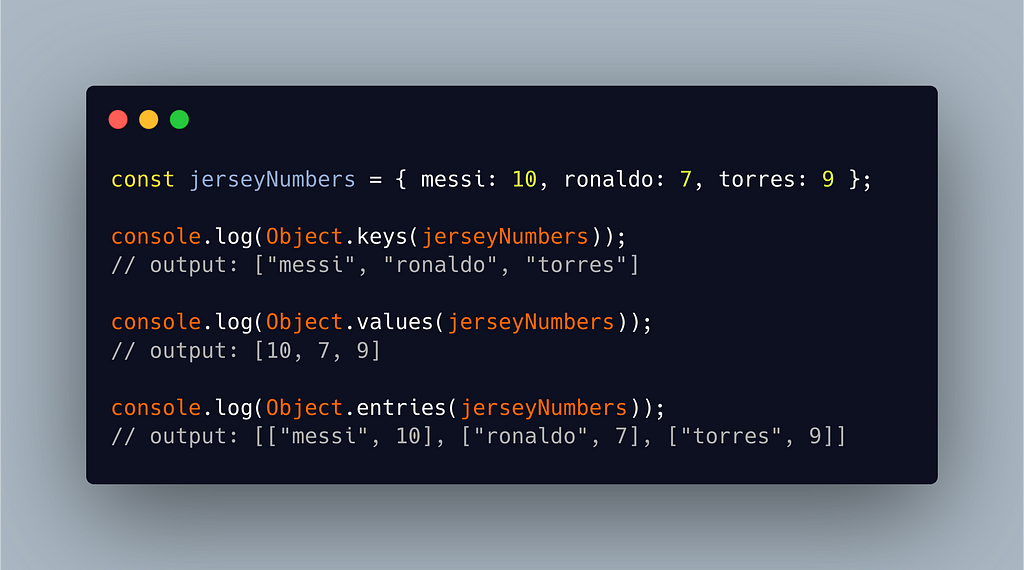
5. Optional Chaining
This feature comes in handy when you are dealing with unreliable, unpredictable data. As for example, I personally have faced multiple scenarios, where I need some deeply nested property and the whole application breaks with a message like —
Uncaught TypeError: Cannot read property someProperty of undefined / null
This is what optional chaining is meant to fix. The operator ?. is going to go one level deep, only if the chain is not nullish (i.e. not null or undefined).
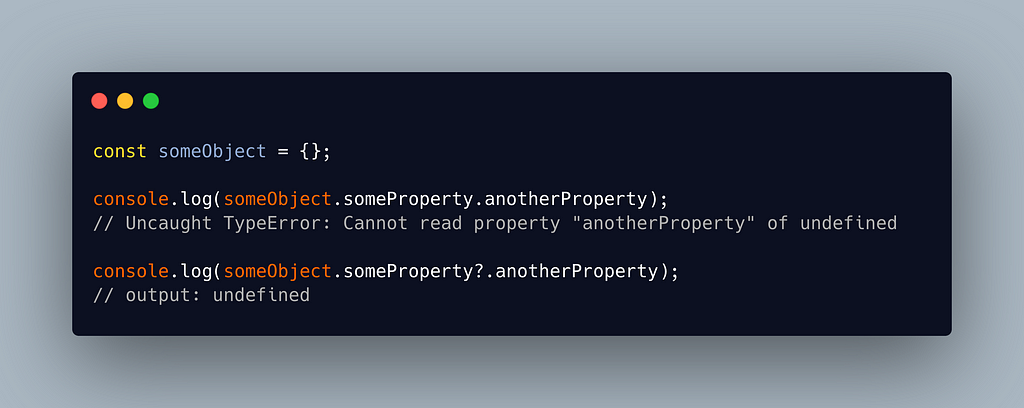
6. Nullish Coalescing Operator
If you have used the Logical OR ||, this would look familiar to you. If you haven’t, let’s discuss that first. The Logical OR would provide a default value if your variable is falsy (i.e. one of null, undefined, 0 and false).
Quite similarly, Nullish Coalescing ?? would provide a default value if the variable is nullish (i.e. either null or undefined). Let’s look at an example —
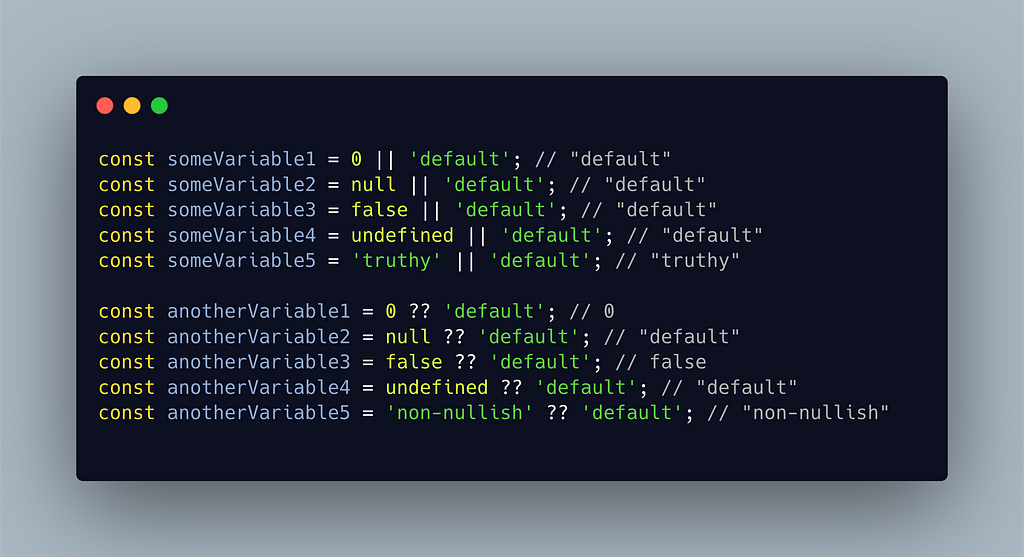
7. Logical Assignment Operator
The logical AND assignment (i.e. &&=) operator only assigns when a variable in truthy, while the logical OR assignment (i.e. ||=) operator would assign when the variable is falsy —
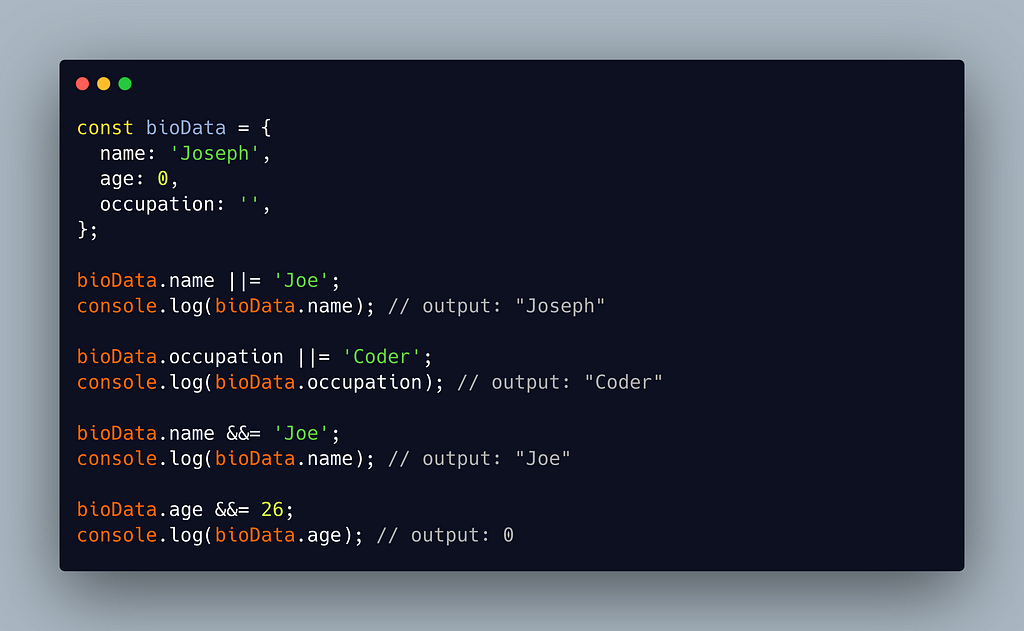
In scenarios where we are not sure if a property exists in an object, but we need to manipulate it somehow, the logical OR assignment provides a cleaner alternative to undefined checks.
Let’s say, we need to find out the number of times an element repeats itself in an array. The way I prefer to do this, is by iterating the array and storing the count of each element in an object —
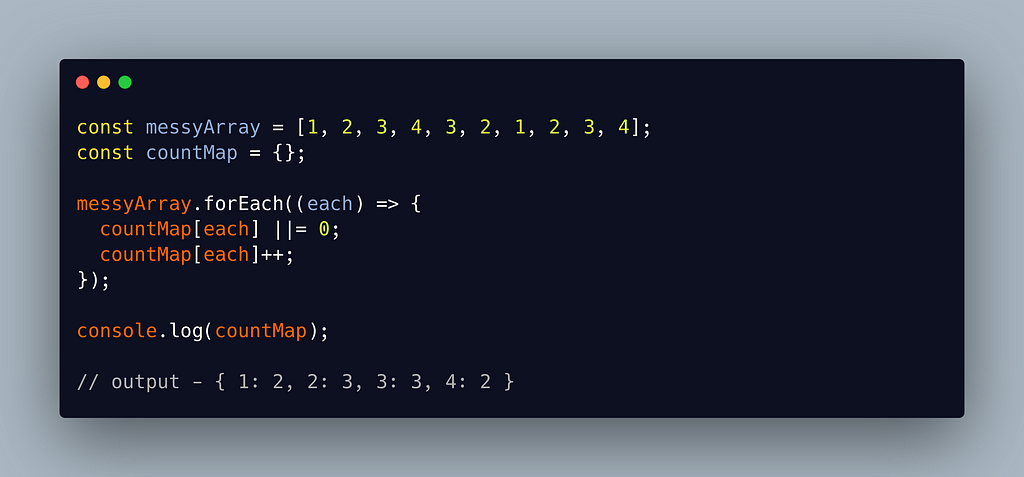
8. Numeric Separators
JavaScript has learnt to exclude underscores while reading numbers. This improves code readability while we are dealing with big numbers —
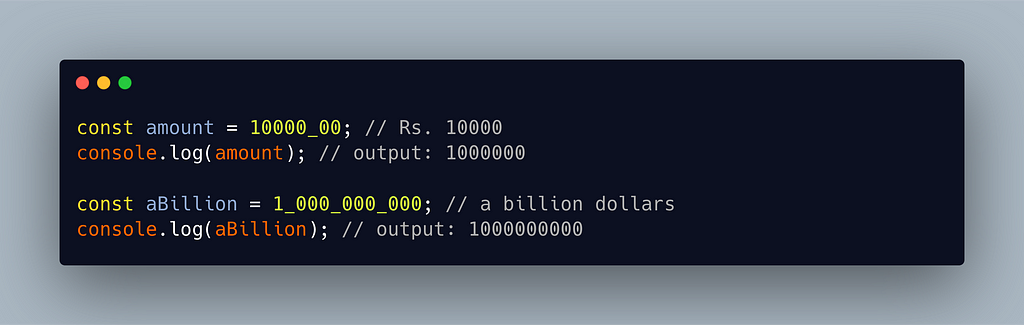
9. Template Literals
Template literals make it easier to deal with strings. We can get rid of the escape characters and get the output exactly as it is in the code, by wrapping it up with the back tick symbols (i.e. `) —
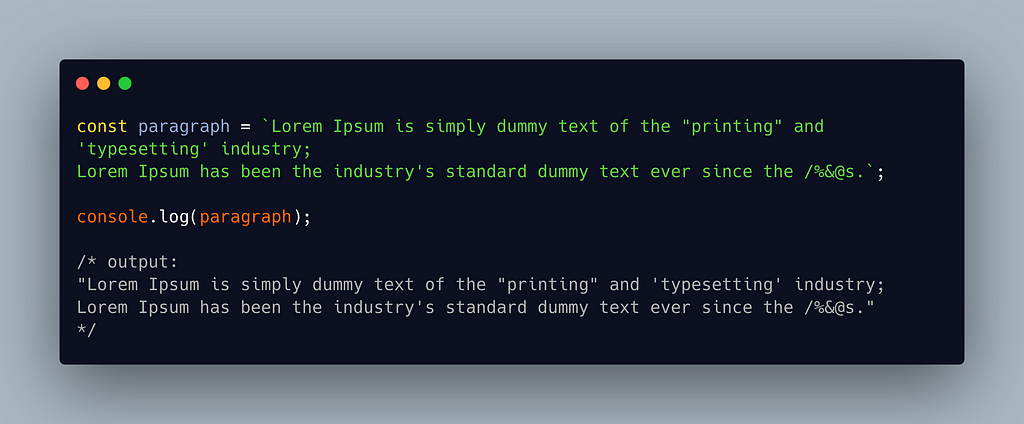
One other useful feature of template literals is what they call — expression interpolation. Most of the time, we would want to add some sort of expression sandwiched between strings, and code readability takes a big hit with all those + signs needed for string concatenation. A better way to do that is wrapping up the expression within ${expression} in a template literal —
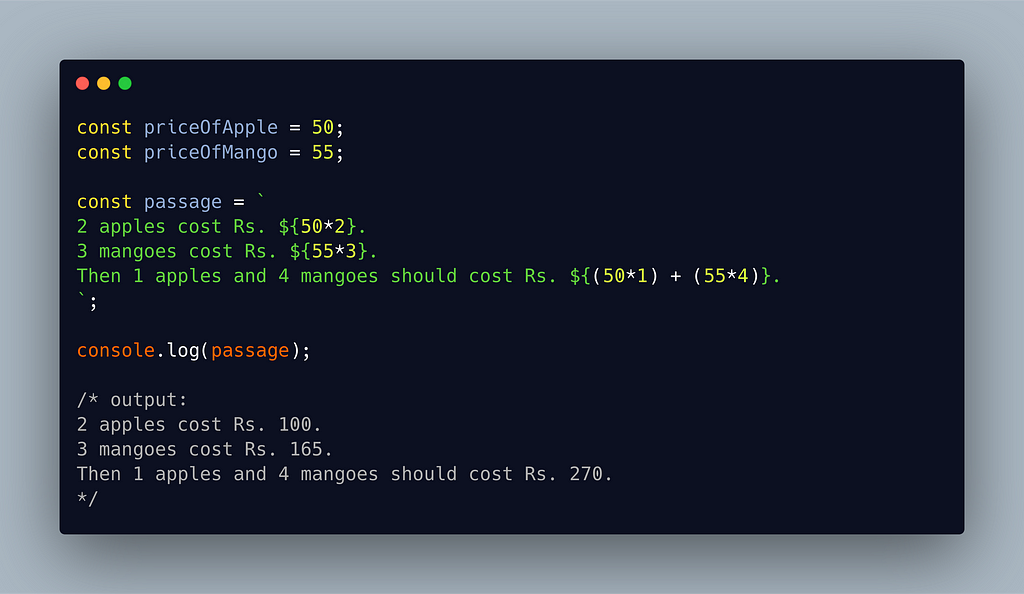
10. Default Parameters
This feature enables us to initialize a parameter with a default value in function definition, which would only be considered if the said parameter is not provided (i.e. is undefined). This is useful when we are required to make a function which can be called with or without any parameters —
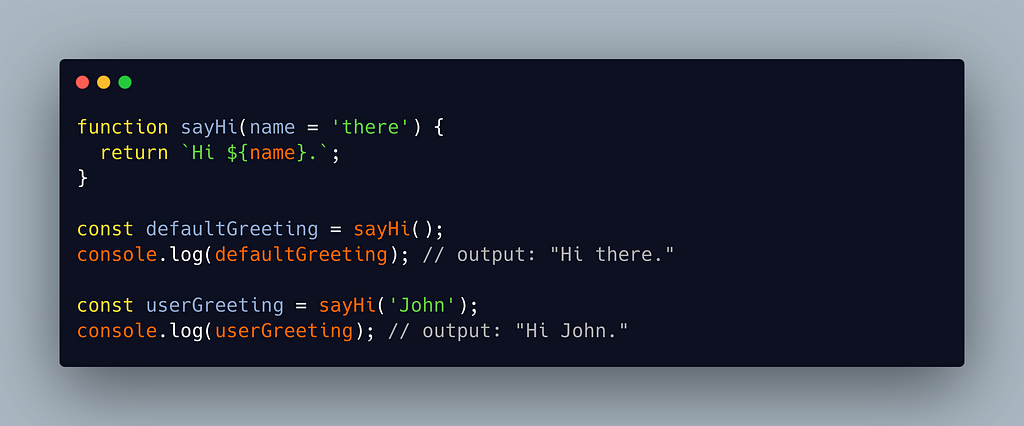
And that’s the list !
Thank you and congratulations on reaching the end of the article. I hope you find it useful.
Find me on LinkedIn, Github or Twitter.
Cheers !
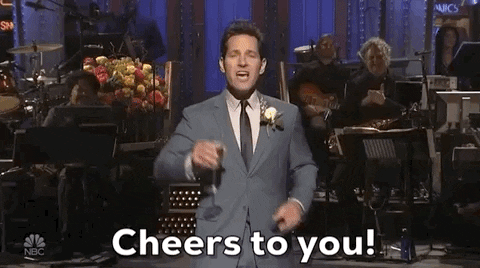
10 Modern JavaScript syntax to help you code faster was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding - Medium and was authored by Himayan Debnath
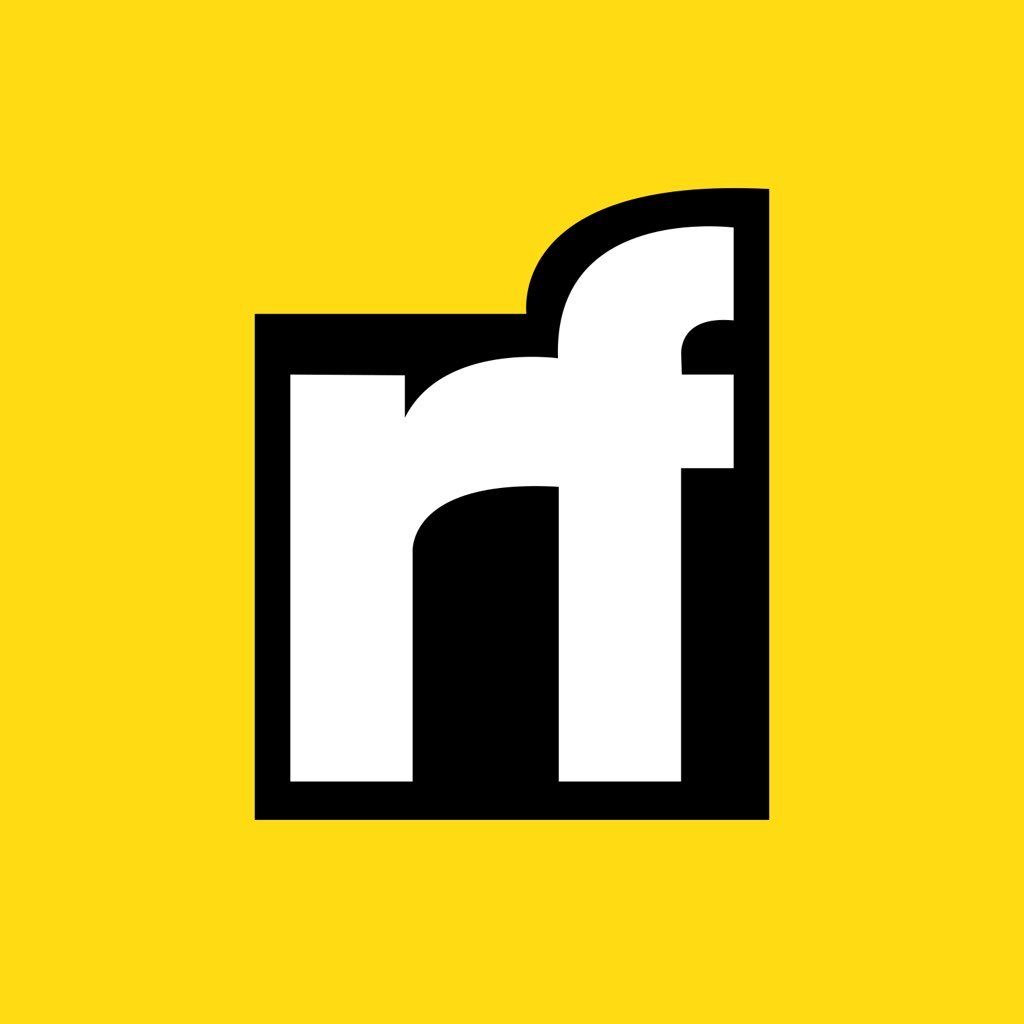
Himayan Debnath | Sciencx (2021-07-05T14:51:59+00:00) 10 Modern JavaScript syntax to help you code faster. Retrieved from https://www.scien.cx/2021/07/05/10-modern-javascript-syntax-to-help-you-code-faster/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.