This content originally appeared on SitePoint and was authored by Ivaylo Gerchev
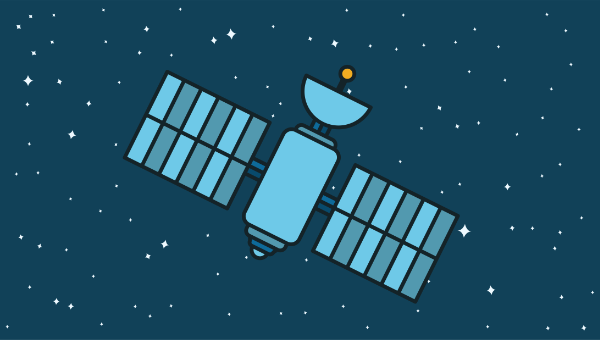
Redis is a fast and efficient in-memory key-value store. It is also known as a data structure server, as the keys can contain strings, lists, sets, hashes and other data structures. If you are using Node.js, you can use the node_redis
module to interact with Redis. This tutorial explains various ways of interacting with Redis from a Node.js app using the node_redis
library.
Installing node_redis
node_redis
, as you may have guessed, is the Redis client for Node.js. You can install it via npm using the following command.
[code]
npm install redis
[/code]
Getting Started
Once you have installed node_redis
module you are good to go. Let’s create a simple file, app.js
, and see how to connect with Redis from Node.js.
app.js
[js]
var redis = require(‘redis’);
var client = redis.createClient(); //creates a new client
[/js]
By default, redis.createClient()
will use 127.0.0.1
and 6379
as the hostname and port respectively. If you have a different host/port you can supply them as following:
[js]
var client = redis.createClient(port, host);
[/js]
Now, you can perform some action once a connection has been established. Basically, you just need to listen for connect
events as shown below.
[js]
client.on(‘connect’, function() {
console.log(‘connected’);
});
[/js]
So, the following snippet goes into app.js
:
[js]
var redis = require(‘redis’);
var client = redis.createClient();
client.on(‘connect’, function() {
console.log(‘connected’);
});
[/js]
Now, type node app
in the terminal to run the app. Make sure your Redis server is up and running before running this snippet.
Storing Key-Value Pairs
Now that you know how to connect with Redis from Node.js, let’s see how to store key-value pairs in Redis storage.
Storing Strings
All the Redis commands are exposed as different functions on the client
object. To store a simple string use the following syntax:
[js]
client.set(‘framework’, ‘AngularJS’);
[/js]
Or
[js]
client.set([‘framework’, ‘AngularJS’]);
[/js]
The above snippets store a simple string AngularJS
against the key framework
. You should note that both the snippets do the same thing. The only difference is that the first one passes a variable number of arguments while the later passes an args
array to client.set()
function. You can also pass an optional callback to get a notification when the operation is complete:
[js]
client.set(‘framework’, ‘AngularJS’, function(err, reply) {
console.log(reply);
});
[/js]
Continue reading Using Redis with Node.js on SitePoint.
This content originally appeared on SitePoint and was authored by Ivaylo Gerchev
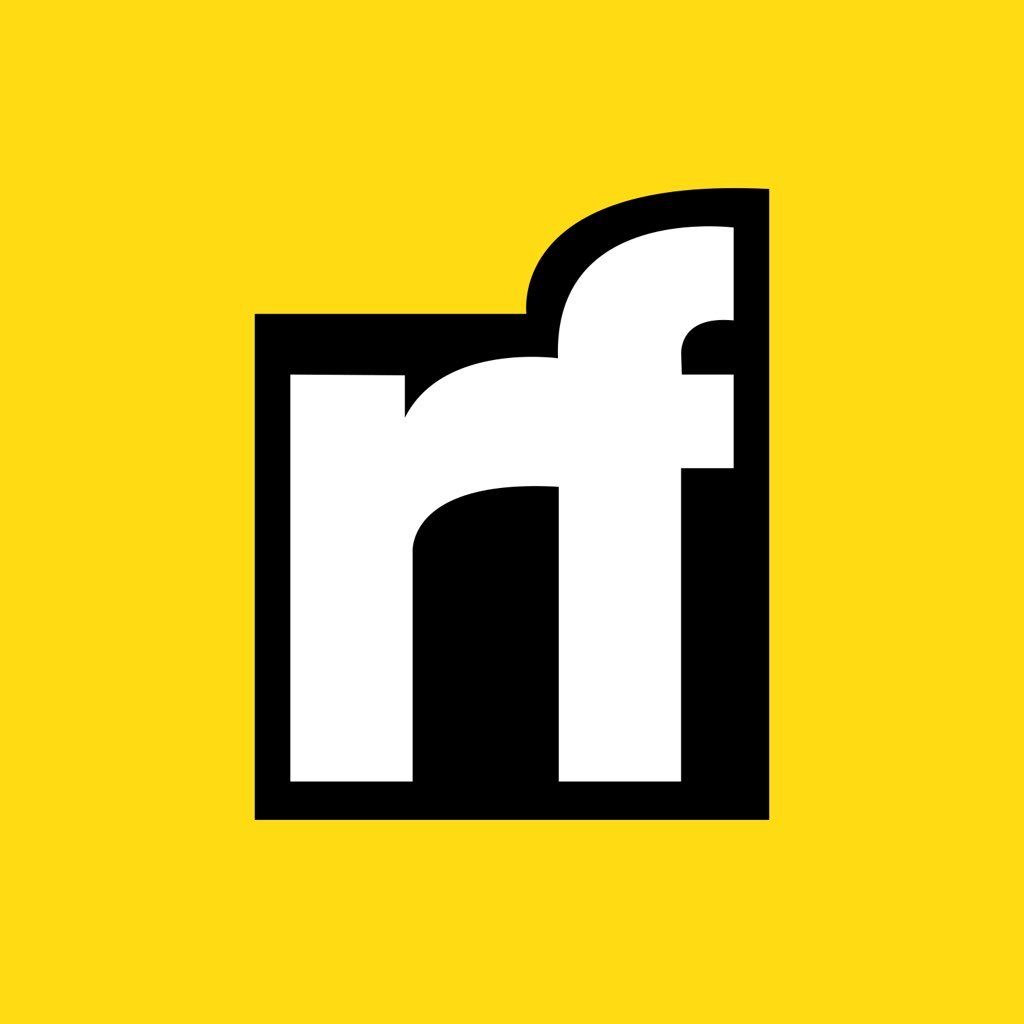
Ivaylo Gerchev | Sciencx (2021-07-12T15:00:03+00:00) Using Redis with Node.js. Retrieved from https://www.scien.cx/2021/07/12/using-redis-with-node-js/
Please log in to upload a file.
There are no updates yet.
Click the Upload button above to add an update.